Day 15: GitHub-JIRA Integration Project - (Project-4) - (Part 2)
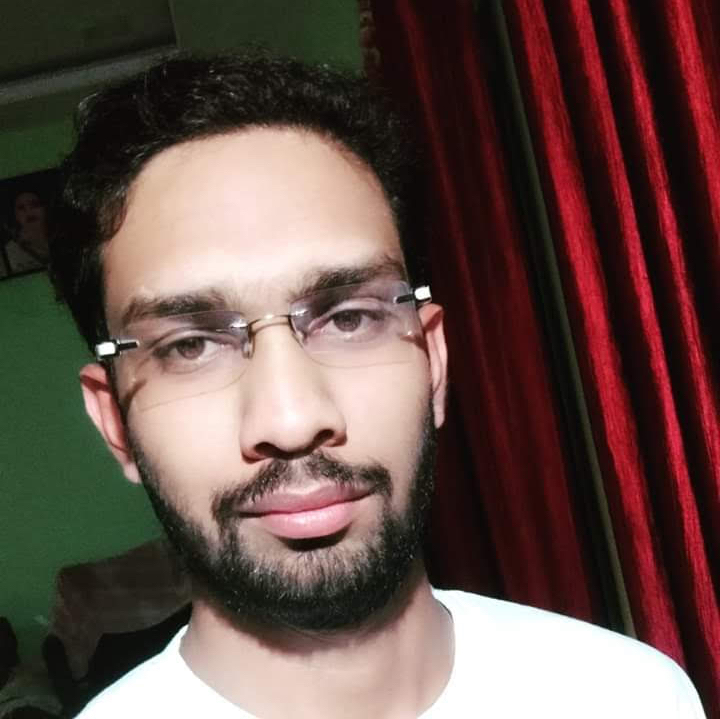
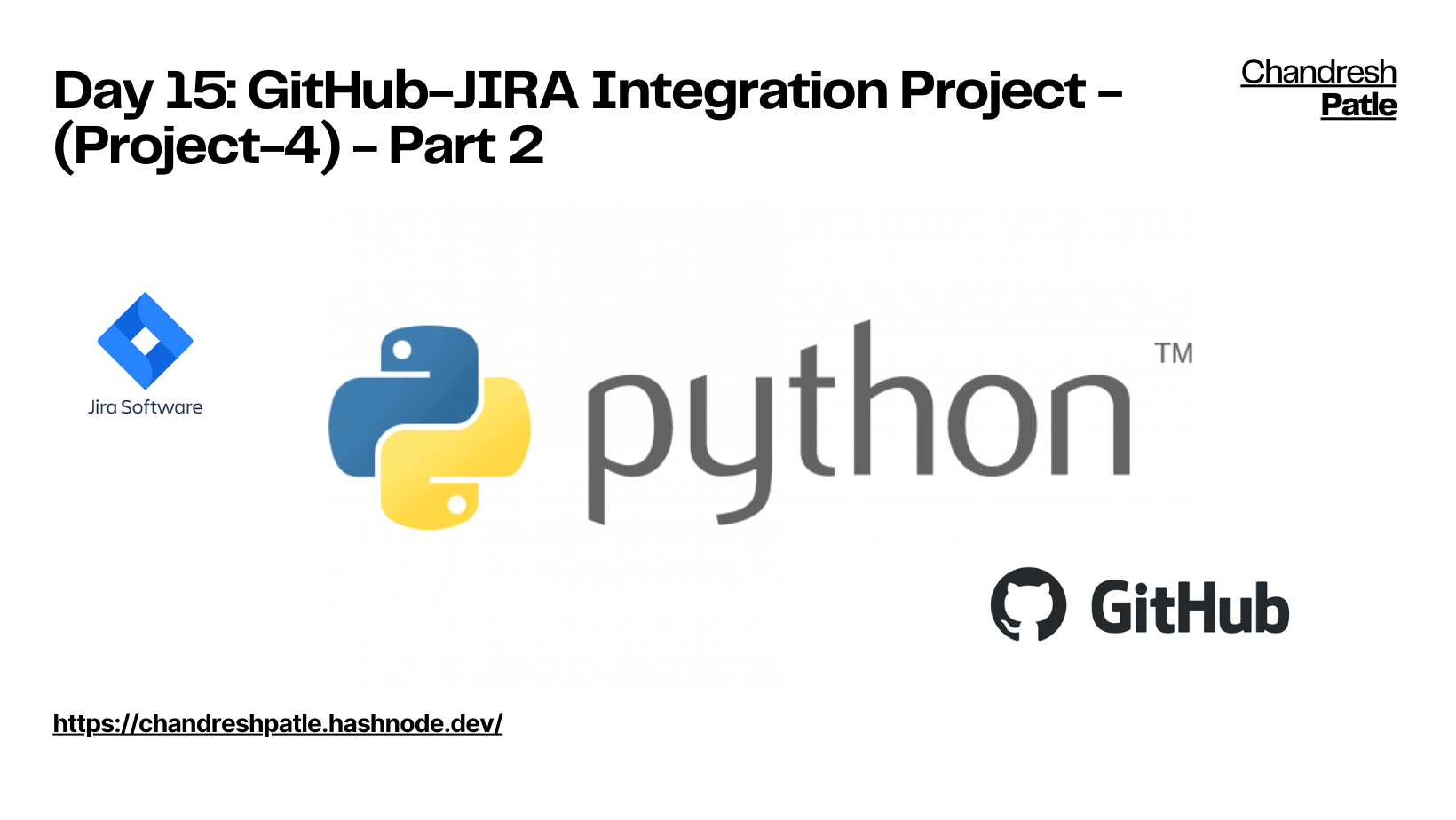
Welcome back to our Python for DevOps series! Today, we're taking our GitHub-JIRA integration project to the next level in Part 2. We'll delve into Flask, a powerful web framework for Python, and explore the process of writing your first API in Python. By the end, we'll have a fully functional Python API that listens for GitHub comments and creates JIRA tickets. Let's get started!
π Introduction to Flask
Flask is a lightweight and flexible web framework for Python. It's designed to be easy to use and scale up to complex applications. Flask allows you to build web applications, including APIs, with minimal boilerplate code.
π Writing Your First API in Python
Let's start by writing a simple API using Flask. In your Python environment, install Flask using:
pip install Flask
Now, create a file named app.py
and write the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/api', methods=['GET'])
def hello_world():
return 'Hello, this is your first Flask API!'
if __name__ == '__main__':
app.run(debug=True)
This code defines a basic Flask application with a single API endpoint /api
that returns a greeting.
π Handling API Calls and Deployment
To handle API calls, we need to enhance our Flask application. Let's modify app.py
to accept GitHub comments and trigger the creation of JIRA tickets. We'll use the GitHub Webhooks to achieve this.
For deployment, platforms like Heroku, AWS, or your server can host Flask applications. Deploying on Heroku is straightforward:
- Create a
Procfile
with the following content:
python app.py
Commit your changes and push them to a GitHub repository.
Create a new app on Heroku, connect it to your GitHub repository, and deploy it.
π Practice Exercise and Example
Now, let's implement the GitHub-JIRA integration in our Flask app. Modify the /api
endpoint to listen for GitHub comments, extract relevant information, and create a JIRA ticket.
from flask import Flask, request
app = Flask(__name__)
@app.route('/api', methods=['POST'])
def github_webhook():
data = request.get_json()
# Extract relevant information from GitHub comment
github_comment = data['comment']['body']
# TODO: Implement JIRA ticket creation based on the comment
return 'Webhook received successfully!'
if __name__ == '__main__':
app.run(debug=True)
In this example, we've added a GitHub Webhook endpoint that listens for POST requests. It extracts the comment body, and your task is to implement the logic for creating a JIRA ticket based on the GitHub comment.
π Conclusion
Congratulations! You've expanded your GitHub-JIRA integration project by incorporating Flask and creating a Python API. Practice handling API calls and explore deployment options. In the next part, we'll enhance our integration with more advanced features.
Feel free to adapt this project to your specific needs, and stay tuned for more exciting Python for DevOps sessions!
Stay tuned for more advanced topics as we continue our Python for DevOps journey!
Note: I am following Abhishek Verraamalla's YouTube playlist for learning.
GitHub Repo: https://github.com/Chandreshpatle28/python-for-devops-av
Happy Learning :)
Stay in the loop with my latest insights and articles on cloud βοΈ and DevOps βΎοΈ by following me on Hashnode, LinkedIn (https://www.linkedin.com/in/chandreshpatle28/), and GitHub (https://github.com/Chandreshpatle28).
Thank you for reading! Your support means the world to me. Let's keep learning, growing, and making a positive impact in the tech world together.
#Git #Linux Devops #Devopscommunity #PythonforDevOps #python
Subscribe to my newsletter
Read articles from CHANDRESH PATLE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
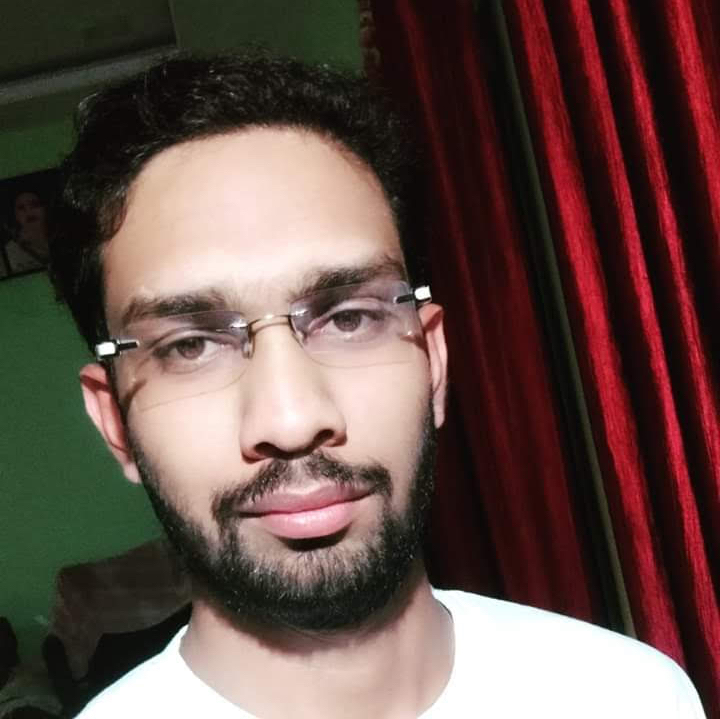
CHANDRESH PATLE
CHANDRESH PATLE
Hi, I'm Chandresh Patle, an aspiring DevOps Engineer with a diverse background in field supervision, manufacturing, and service consulting. With a strong foundation in engineering and project management, I bring a unique perspective to my work. I recently completed a Post Graduate Diploma in Advanced Computing (PG-DAC), where I honed my skills in web development, frontend and backend technologies, databases, and DevOps practices. My proficiency extends to Core Java, Oracle, MySQL, SDLC, AWS, Docker, Kubernetes, Ansible, Linux, GitHub, Terraform, Grafana, Selenium, and Jira. I am passionate about leveraging technology to drive efficient and reliable software delivery. With a focus on DevOps principles and automation, I strive to optimize workflows and enhance collaboration among teams. I am constantly seeking new opportunities to expand my knowledge and stay up-to-date with the latest industry trends. If you have any questions, collaboration ideas, or professional opportunities, feel free to reach out to me at patle269@gmail.com. I'm always open to connecting with fellow tech enthusiasts and exploring ways to contribute to the DevOps community. Let's build a better future through innovation and continuous improvement!