Adapter Design Pattern
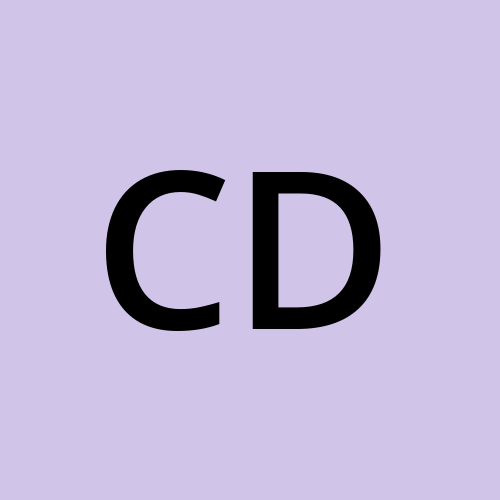
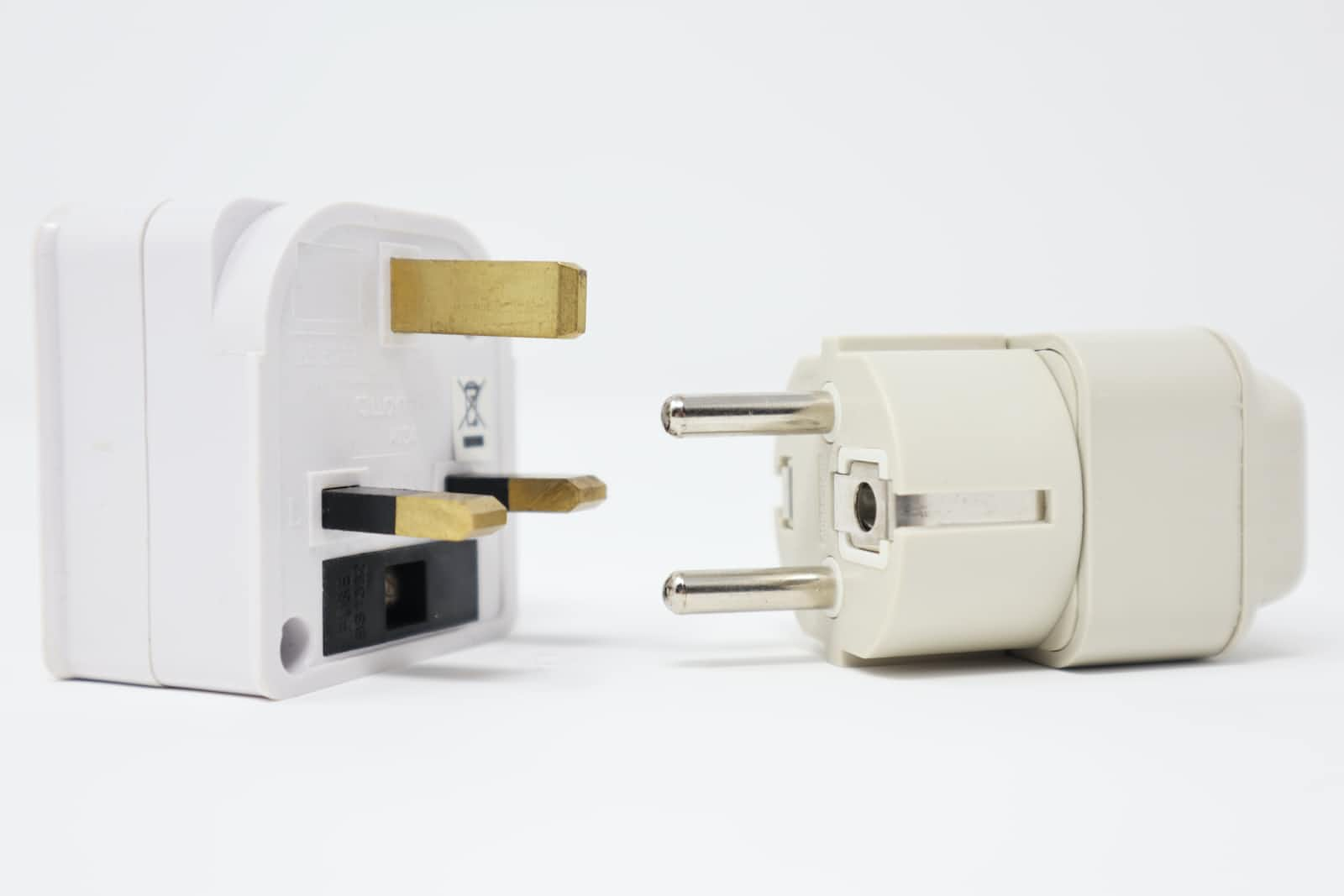
Introduction
This pattern acts as a bridge between the existing interface and the expected interface.
UML diagram
Real World Examples
I. Power Adapter and Socket Compatibility - When bringing a device from the USA to India, it's important to note that the USA power adapter cannot be directly used in India. This is because US appliances operate on 110V with flat pin plugs, whereas India uses 220V with round plugs. To connect the US power adapter to the Indian socket, a converter device, commonly referred to as the adapter, is required. This adapter accepts the flat input on one side and features a connector on the other side that seamlessly fits into the socket.
Socket - Original Interface (Adaptee)
Converter - Adapter
Power Adapter - Client
II. JSON to XML Converter - Imagine a scenario where our client systems prefer data in JSON format, yet our server responds with XML. Directly transmitting this XML to the client would result in a system malfunction. To prevent such format-related issues, we employ a converter that seamlessly transforms the source data (XML) into JSON format. This conversion tool is also referred to as an adapter.
III. Mobile Adapter - Our mobile devices are designed to receive direct current (dc) power with c-type inputs, yet the prevalent power sources in the real world provide alternating current (ac) with 3/2 pins output. To resolve this incongruity, we developed a converter that transforms the ac output into the required dc input, and this converter is essentially our mobile adapter.
Note: In the event that the client directly receives data from the source and if there are any future changes in the data format at the source, corresponding adjustments must be made on the client side as well. To prevent modifications on the client side, a converter is employed to manage and accommodate all such changes.
Weight Machine design and code
We possess a weight machine that provides weight measurements in pounds, but our client systems demand weights in kilograms. To address this discrepancy, we employ an adapter that facilitates the conversion process.
Code
Adaptee
package Adaptee;
public interface WeightMachine {
public int getWeightInPound();
}
package Adaptee;
public class WeightMachineForBabies implements WeightMachine{
@Override
public int getWeightInPound() {
return 28;
}
}
Adapter
package Adapter;
public interface WeightMachineAdapter {
public double getWeightInKg();
}
package Adapter;
import Adaptee.WeightMachine;
public class WeightMachineAdapterImpl implements WeightMachineAdapter{
private WeightMachine weightMachine;
public WeightMachineAdapterImpl(WeightMachine weightMachine){
this.weightMachine = weightMachine;
}
@Override
public double getWeightInKg() {
return weightMachine.getWeightInPound() * 0.45;
}
}
Client
package Client;
import Adaptee.WeightMachineForBabies;
import Adapter.WeightMachineAdapter;
import Adapter.WeightMachineAdapterImpl;
public class Main {
public static void main(String[] args) {
WeightMachineAdapter weightMachineAdapter = new WeightMachineAdapterImpl(new WeightMachineForBabies());
System.out.println(weightMachineAdapter.getWeightInKg());
}
}
Subscribe to my newsletter
Read articles from Chetan Datta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
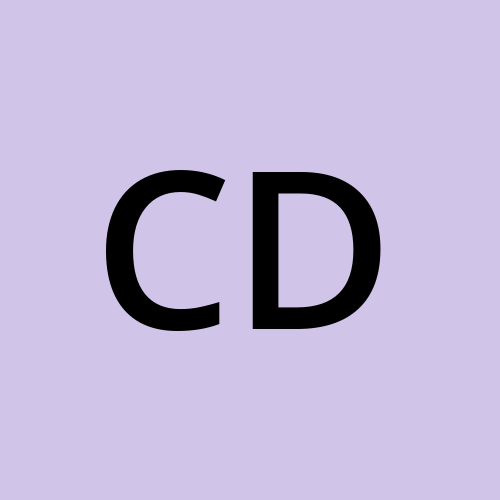
Chetan Datta
Chetan Datta
I'm someone deeply engrossed in the world of software developement, and I find joy in sharing my thoughts and insights on various topics. You can explore my exclusive content here, where I meticulously document all things tech-related that spark my curiosity. Stay connected for my latest discoveries and observations.