Django Views and Templates: Crafting Dynamic Web Experiences
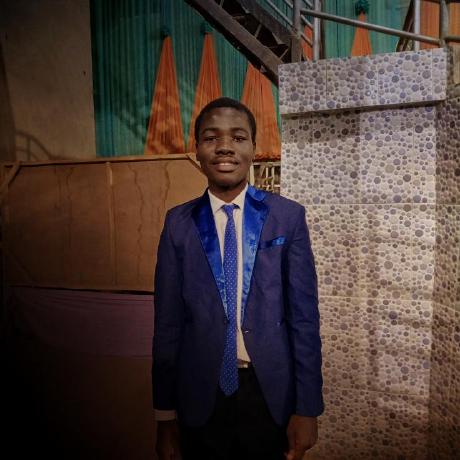
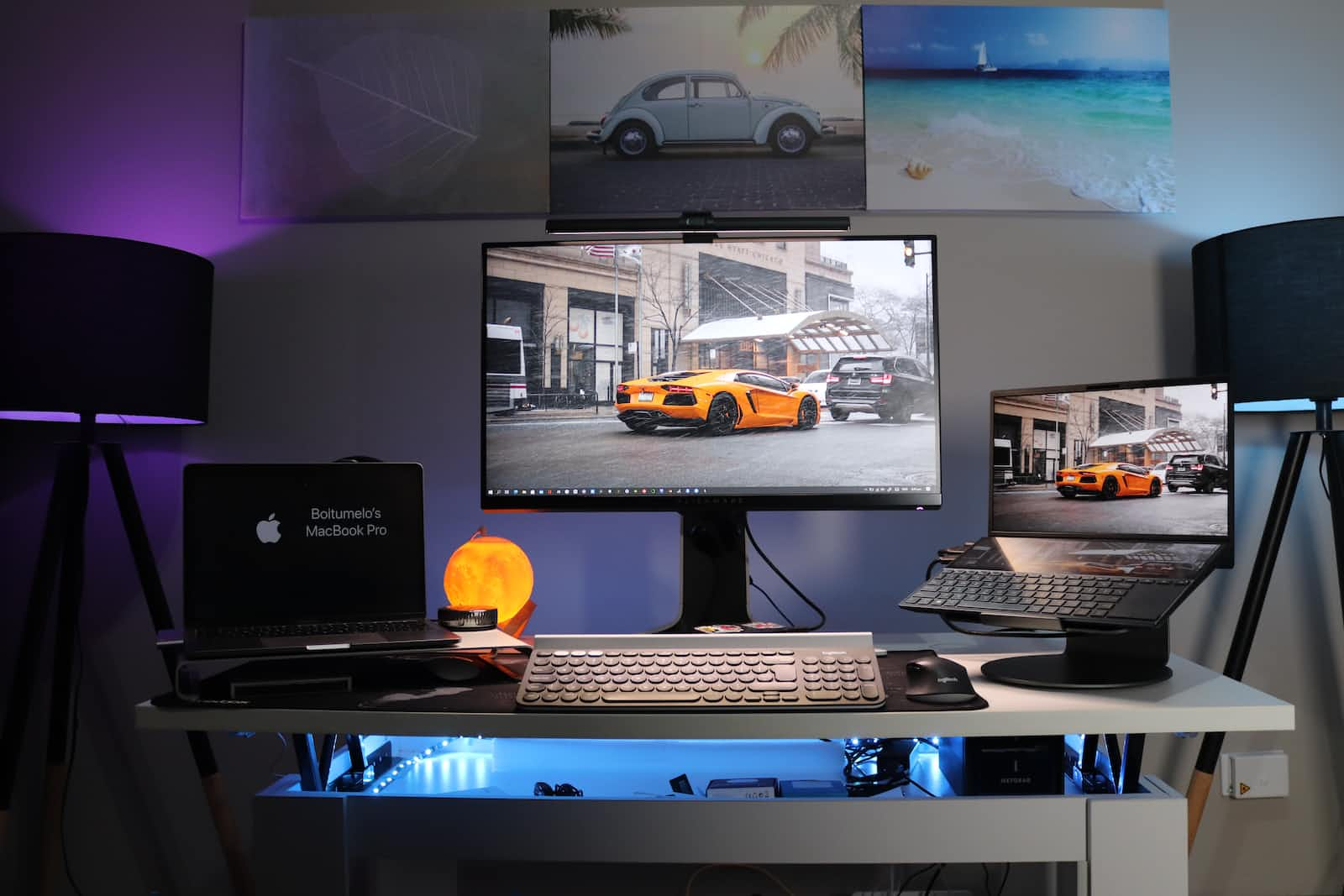
In Django web framework , Views and Templates are responsible for shaping how your web application looks and behaves. In this article let's unravel the intricacies of Views—which helps with request processing—and Templates—with renders the dynamic and artistic feel of your application.
Understanding Django Views: The Brain Behind the Operation
At the core of every web application lies the View—a function or class that processes an incoming request and returns an appropriate response. Here's a breakdown of key concepts:
Function-Based Views
#Views can be simple functions that
# take a request as input and return a response.
from django.shortcuts import render
def index(request):
return render(request, 'index.html')
#For more complex functionality, Django provides
#class-based views with reusable mixins.
from django.views import View
from django.shortcuts import render
class IndexView(View):
def get(self, request):
return render(request, 'index.html')
Request and Response
Views handle the incoming request and decide what response to send back based on the codes.
Views also interact with the models to fetch data (if necessary) and utilize django templates to render the HTML
Generic Class-Based Views:
- Leverage Django's built-in generic views for common patterns like displaying a list or detail view.
from django.views.generic import ListView, DetailView
from .models import Article
class ArticleListView(ListView):
model = Article
template_name = 'article_list.html'
class ArticleDetailView(DetailView):
model = Article
template_name = 'article_detail.html'
How about Templates?
Templates are the design blueprints for your web pages. They mix HTML with Django template language to create dynamic and data-driven content. Let's explore the key components:
Django templates help Inject dynamic data into HTML using template variables.
<h1>{{ article.title }}</h1>
Use control structures to create conditional logic and loops in templates.
{% if user.is_authenticated %}
<p>Welcome, {{ user.username }}!</p>
{% else %}
<p>Please log in.</p>
{% endif %}
Django Templates help reduce boiler plates through Inheritance
<!-- base.html -->
<!DOCTYPE html>
<html>
<head>
<title>{% block title %}Default Title{% endblock %}</title>
</head>
<body>
{% block content %}{% endblock %}
</body>
</html>
<!-- page.html -->
{% extends 'base.html' %}
{% block title %}Page Title{% endblock %}
{% block content %}
<h1>Hello, World!</h1>
{% endblock %}
Views and Templates work in tandem to create seamless web experiences. Here's how they collaborate:
Passing Data to Templates
Views pass data to templates, making it accessible for rendering.
def article_detail(request, article_id): article = get_object_or_404(Article, pk=article_id) return render(request, 'article_detail.html', {'article': article})
Use template tags to perform logic within templates, such as looping through a list.
{% for article in latest_articles %} <h2>{{ article.title }}</h2> <p>{{ article.summary }}</p> {% endfor %}
Access variables and attributes within templates.
<h1>{{ user.username }}'s Profile</h1>
Templates are used to also modify variablesusing filters
<p>{{ article.content|linebreaks }}</p>
It also allows for more logic and control structures
{% if user.is_authenticated %} <p>Welcome, {{ user.username }}!</p> {% else %} .... {% endif %}
Feel free to comment, ask questions and am open to collaboration.
Subscribe to my newsletter
Read articles from Olumide Adeola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
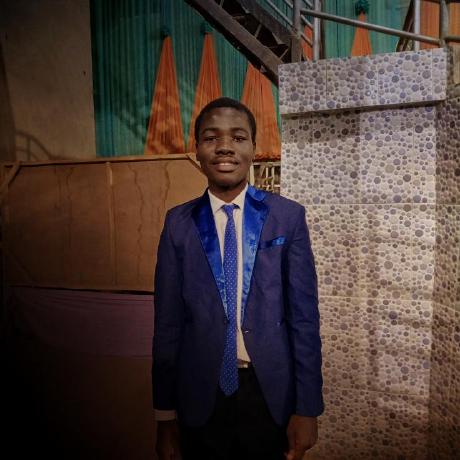
Olumide Adeola
Olumide Adeola
Classical | Health | Academics | Services et Solutions | Finance | Agriculture | Technology This is what my passion revolves round....