Graphql types

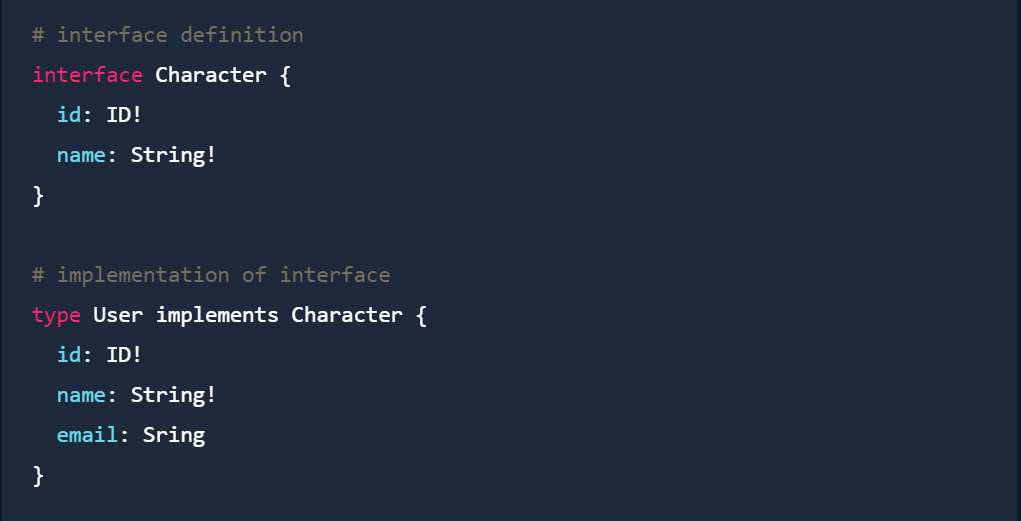
GraphQL is a strongly typed language. Type System defines various data types that can be used in a GraphQL application.
Types in GrapqhQL
Scalar:
Scalars aren't just data types; they're the raw materials that store a single value. GraphQL comes with a set of default scalar types out of the box:
Int
: A signed 32‐bit integer.Float
: A signed double-precision floating-point value.String
: A UTF‐8 character sequence.Boolean
:true
orfalse
.ID
:ID
signifies unique identifier
scalar Date
# We defined Date as a scalar type
Object:
Objects aren't merely concepts, they're the blueprints for structured data. You can fetch objects from your service, and what fields it has.
type User {
id: ID!
username: String!
email: String!
posts: [Post]
}
# User type object contains various scalar type like username is of String,
# id is of ID Scalar type
Query:
Entry point type to other specific types i.e. you can use them to fetch other types that are defined in the schema
type Query {
getUser(id: ID): User
posts:[Post]
}
# getUser query will be used to fetch User type based on some parameter
# posts query will return array of Post type
Mutation:
The entry point for data manipulation, we generally use mutation for updating the data. They work the same way as Query does
type Mutation{
updatePost(id: ID): Post
}
# updatePost Mutation type will update the Post object type and return the updated Pst type
Enumeration:
Also called Enums, enumeration types are a special kind of scalar that is restricted to a particular set of allowed values. This allows you to:
Validate that any arguments of this type are one of the allowed values
Communicate through the type system that a field will always be one of a finite set of values
enum POST_STATUS{
PUBLISHED
DRAFT
ARCHIVE
}
# POST_STATUS type defined that POST_STATUS can only be one of the given items
Interfaces:
An Interface is an abstract type that includes a certain set of fields that a type must include to implement the interface
# interface definition
interface Character {
id: ID!
name: String!
}
# implementation of interface
type User implements Character {
id: ID!
name: String!
email: Sring
}
Union:
Union is a combination of 2 or more types, it is used where the result can be any of the combination item types
union SearchResult = User| Post
# union definition
# now SearchResult can either be User or Post types
Subscribe to my newsletter
Read articles from Gaurav Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gaurav Kumar
Gaurav Kumar
Hey! I'm Gaurav, a tech writer who's all about MERN, Next.js, and GraphQL. I love breaking down complex concepts into easy-to-understand articles. I've got a solid grip on MongoDB, Express.js, React, Node.js, and Next.js to supercharge app performance and user experiences. And let me tell you, GraphQL is a game-changer for data retrieval and manipulation. I've had the opportunity to share my knowledge through tutorials and best practices in top tech publications. My mission? Empowering developers and building a supportive community. Let's level up together with my insightful articles that'll help you create top-notch applications!