JavaScript trim() method: Simply Explained
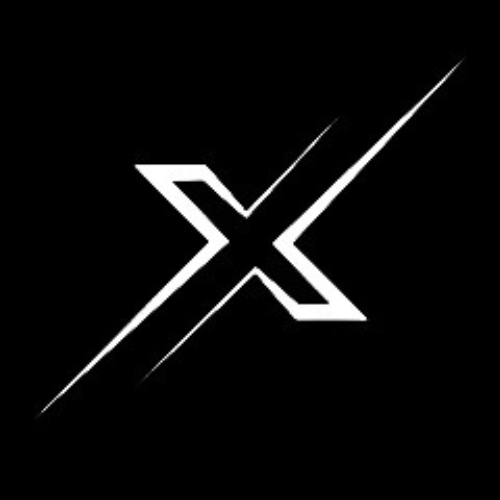
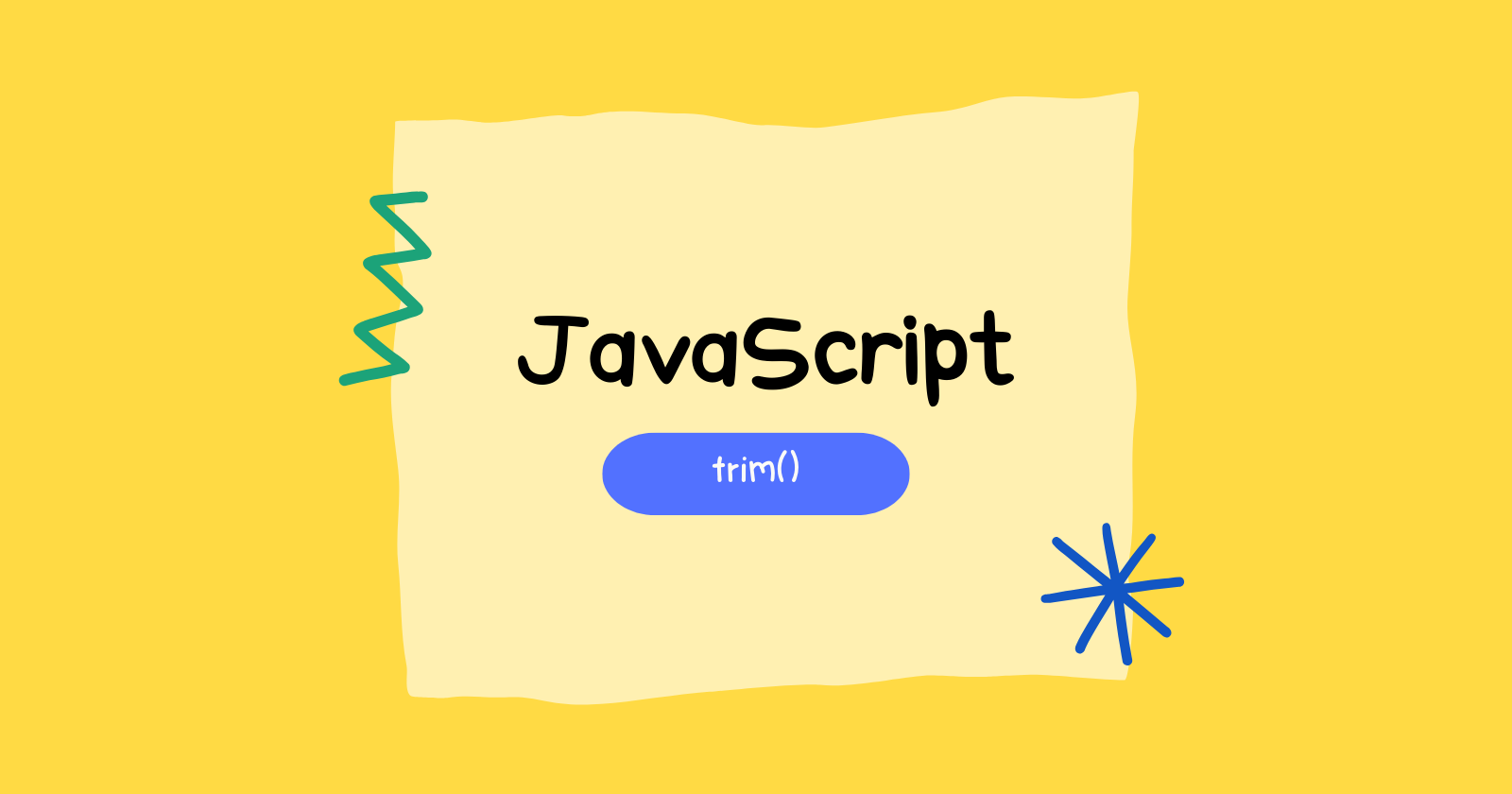
In JavaScript, the trim()
method is a built-in method for strings. It is used to remove whitespace (spaces, tabs, and line breaks) from both ends of a string. The trim()
method does not modify the original string; instead, it returns a new string with the leading and trailing whitespace removed.
Here's a simple example:
let originalString = " Hello, World! ";
let trimmedString = originalString.trim();
console.log(trimmedString); // Output: "Hello, World!"
In this example, the trim()
method is called on the originalString
, which contains extra spaces at both ends. The resulting trimmedString
is a new string without those leading and trailing spaces.
It's important to note that the trim()
method doesn't remove whitespace within the string itself, only at the beginning and end. If you need to remove all whitespace, including spaces within the string, you might consider combining trim()
with a regular expression or another method, such as replace()
.
let stringWithSpaces = " This is a string with spaces ";
let stringWithoutSpaces = stringWithSpaces.trim().replace(/\s/g, '');
console.log(stringWithoutSpaces); // Output: "Thisisastringwithspaces"
In this example, the replace(/\s/g, '')
part of the code uses a regular expression (/\s/g
) to match all whitespace characters globally (g
flag) and replace them with an empty string. This effectively removes all spaces from the string, both at the beginning and within the string.
Subscribe to my newsletter
Read articles from Aditya Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
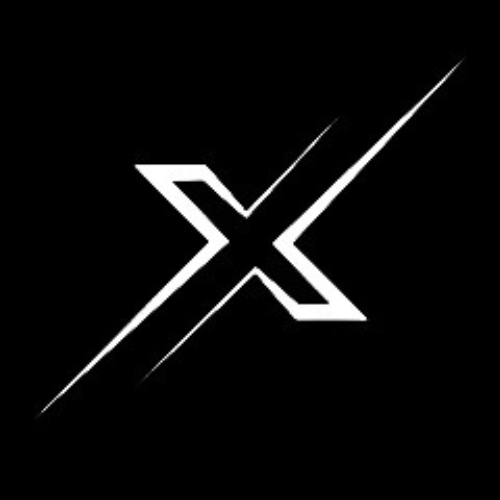
Aditya Sharma
Aditya Sharma
I am a web developer from Bengaluru, India. My mission is to turn your vision into a compelling digital experience. I'm here to collaborate and bring your ideas to life. Let's build something amazing together!