Higher-Order Array Loops (for in, for of, and foreach loop) in JavaScript in-depth
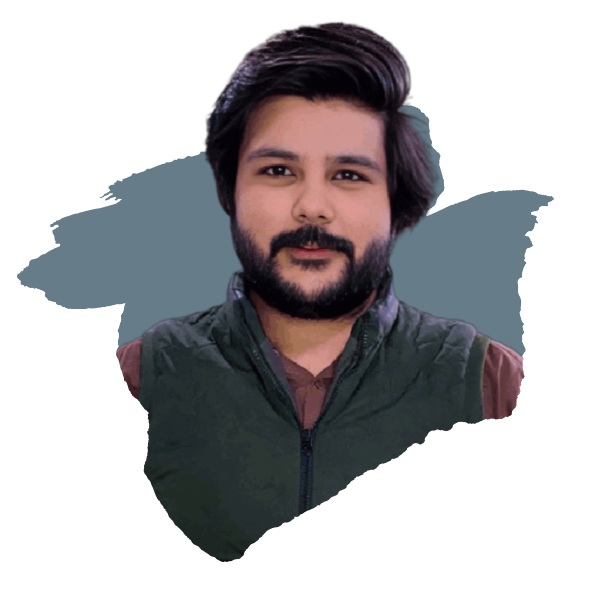
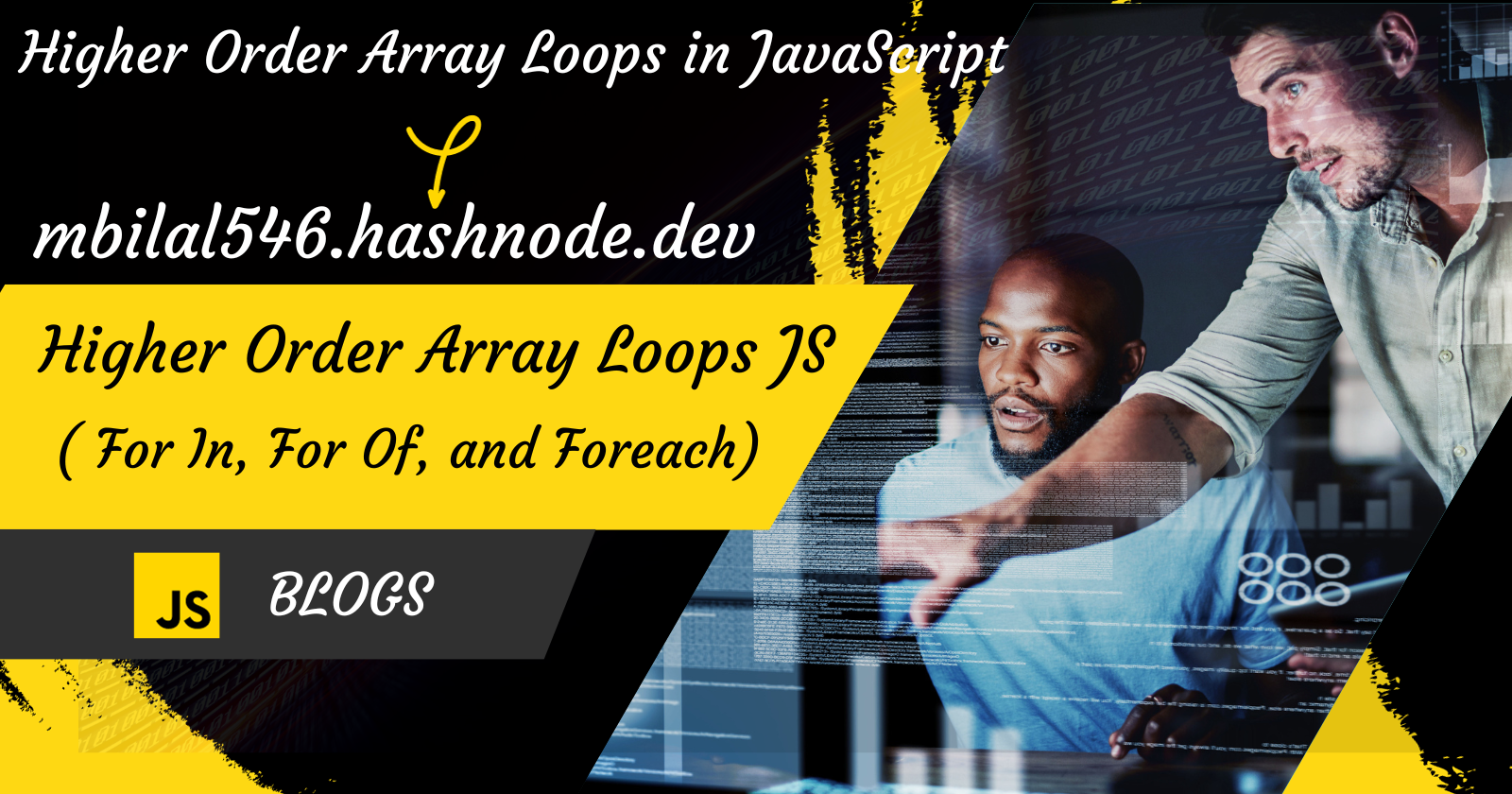
There are several types of array loops. Let's discuss them one by one with an example:
For-In Loop
In JavaScript, the for...in
loop is used to iterate over the properties of an object. It can be used with both arrays and objects, but it's important to understand its behavior and limitations.
For Arrays:
When used with arrays, for...in
iterates over the enumerable properties of an object, including the array indices.
For Example:
let arr = ["JavaScript", "Blog", "Best", "In-depth"];
for (let i in arr) {
console.log(i); // This will print array indexes
}
OutPut:
0
1
2
3
// If you want to print array values then
let arr = ["JavaScript", "Blog", "Best", "In-depth"];
for (let i in arr) {
console.log(arr[i]);
}
OutPut:
JavaScript
Blog
Best
In-depth
However, it's generally not recommended for iterating over arrays, as it may not always produce the expected results. While possible, it's not the ideal choice for arrays due to the issues that It might include non-index properties from the array's prototype. and its traversal orders might not be consistent.
For Objects:
for...in
is commonly used to iterate over the properties of an object. It iterates over the object's enumerable properties, including inherited ones.
For Example:
let obj = {
JS: "JavaScript",
cpp: "C++",
rb: "Ruby",
swift: "Swift",
}
for (const val in obj) {
console.log(val); // If print the values of the objects
}
Output:
JS
cpp
rb
swift
// If you want to print both keys and values then
for (const val in obj) {
console.log(`${val} Shortcut written for ${obj[val]}`);
}
Output:
JS Shortcut written for JavaScript
cpp Shortcut written for C++
rb Shortcut written for Ruby
swift Shortcut written for Swift
For-Of Loop
In JavaScript, the for...of
loop is used to iterate over iterable objects, such as arrays, strings, maps, sets, and more. It provides a simpler syntax compared to traditional for
loops and is particularly useful for iterating over the values of an iterable.
For Arrays:
It is suitable for arrays to print elements directly
For Example:
let arr = ["JavaScript", "Blog", "Best", "In-depth"];
for (let elem of arr) {
console.log(elem); // It will print elements directly
}
OutPut:
JavaScript
Blog
Best
In-depth
For Objects:
When it comes to objects, the for...of
loop is not suitable because it works with iterable objects, and objects in JavaScript are not inherently iterable.
If you specifically want to iterate over the values of an object, you can use Object.values()
:
For Example:
let obj = {
JS: "JavaScript",
cpp: "C++",
rb: "Ruby",
swift: "Swift",
}
// Iterating over values of an object using Object.values()
for (const val of Object.values(obj)) {
console.log(val);
}
Output:
JavaScript
C++
Ruby
Swift
Foreach Loop
In JavaScript, the forEach
method is used to iterate over elements in an array or key-value pairs in an object. It mostly uses a loop because it is used for both arrays and objects. It takes a callback function in it as an argument. A callback function is an anonymous (without name) function.
For Example:
For Arrays:
let arr = ["JavaScript", "Blog", "Best", "In-depth"];
arr.forEach(function (values) {
console.log(values);
})
OutPut:
JavaScript
Blog
Best
In-depth
// You can also do this task through arrow function
let arr = ["JavaScript", "Blog", "Best", "In-depth"];
arr.forEach( (values)=> {
console.log(values);
})
OutPut:
JavaScript
Blog
Best
In-depth
If you want to declare the function separately then you can pass its reference in foreach
loop. For this, you will have to provide the name of the function for its reference.
For Example:
let arr = ["JavaScript", "Blog", "Best", "In-depth"];
// creating function separately
let arrElements = (values)=> {
console.log(values);
}
// Passing the reference of the function
arr.forEach(arrElements)
OutPut:
JavaScript
Blog
Best
In-depth
Note:
foreach
not have only the parameter of elements only. It has the parameters of index and complete array also.
For Example:
let arr = ["JavaScript", "Blog", "Best", "In-depth"];
arr.forEach( (values, index, arr)=> {
console.log(values, index, arr);
});
OutPut:
JavaScript 0 [ 'JavaScript', 'Blog', 'Best', 'In-depth' ]
Blog 1 [ 'JavaScript', 'Blog', 'Best', 'In-depth' ]
Best 2 [ 'JavaScript', 'Blog', 'Best', 'In-depth' ]
In-depth 3 [ 'JavaScript', 'Blog', 'Best', 'In-depth' ]
For Objects:
let obj = {
JS: "JavaScript",
cpp: "C++",
rb: "Ruby",
swift: "Swift",
};
// accessing only keys
Object.keys(obj).forEach( (keys)=>{
console.log(keys);
});
Output:
JS
cpp
rb
swift
// accessing only values
Object.values(obj).forEach( (val)=>{
console.log(val);
});
// Output:
JavaScript
C++
Ruby
Swift
// accessing both keys and values
Object.entries(obj).forEach( ([keys, val])=>{
console.log(keys, ":" , val);
});
// Output:
JS : JavaScript
cpp : C++
rb : Ruby
swift : Swift
The scenario of an array that we use mostly foreach
in JavaScript:
The data came from the database in the format of an array. We have to iterate that through foreach
loop.
Let's see an example:
const data = [
{
languageName: "JavaScript",
languageFileName: "js",
},
{
languageName: "Python",
languageFileName: "py",
},
{
languageName: "Java",
languageFileName: "java",
},
];
// Suppose you want to access the language names
data.forEach((item)=>{
console.log(item.languageName); // Prints the values
});
Output:
Output:
JavaScript
Python
Java
But sometimes it happens that we don't always print the value we have to return
them and store them in a variable to use them further in the program, we can't do that type of task with foreach
loop. Because it doesn't return the values.
Let's see with an example:
const data = [
{
languageName: "JavaScript",
languageFileName: "js",
},
{
languageName: "Python",
languageFileName: "py",
},
{
languageName: "Java",
languageFileName: "java",
},
];
// Suppose you want to return the languageName
const newValues = data.forEach((item)=>{
return item.languageName;
});
Output: undefined
For this purpose, we have some higher-order array methods (map()
, filter()
, and reduce()
). We will discuss them in the next blog.
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
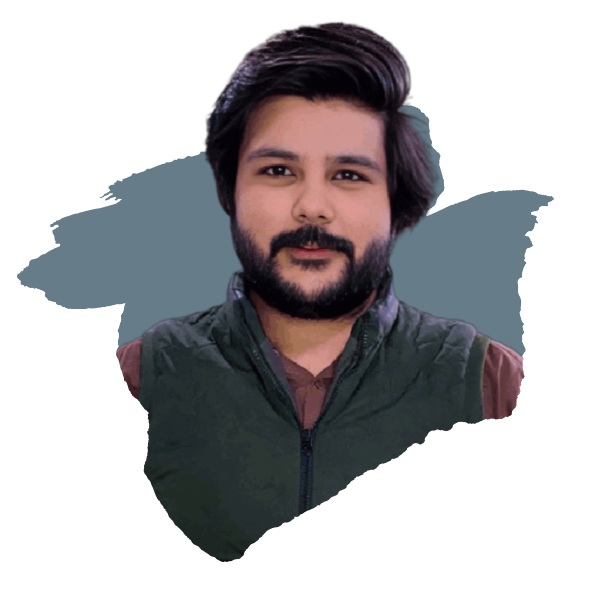
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. ๐ Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. ๐ Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub ๐ฅ Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. โจ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. ๐ Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. ๐ฅ Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.