Exploring Isolates in Dart: Concurrency Made Easy
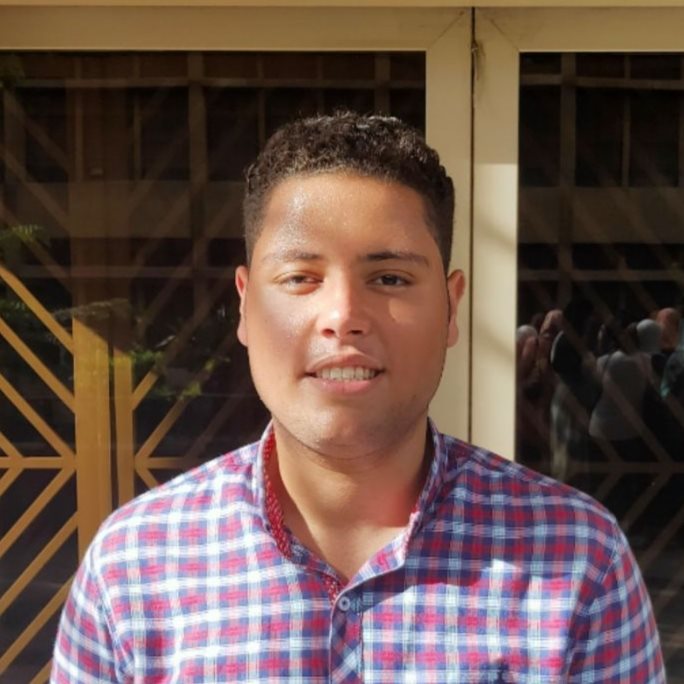
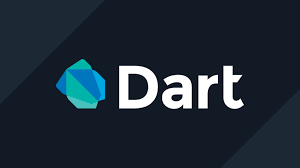
Introduction
Dart, Google's open-source programming language, is well-known for its simplicity and versatility. One of the features that sets Dart apart is its support for isolates, which allows developers to achieve concurrency without the complexity of managing threads directly. In this blog post, we'll delve into isolates in Dart, exploring what they are, why they matter, and how to leverage them for more efficient and responsive applications.
Understanding Isolates:
1. What are Isolates?
Dart isolates are lightweight, independent workers that run concurrently, but in a separate memory space. Unlike threads, isolates don't share memory, reducing the risk of race conditions and making concurrent programming more manageable.
2. Why Isolates?
Isolates are crucial for building responsive and scalable applications. By executing tasks in parallel, isolates enable better utilization of multi-core processors, leading to improved performance. Additionally, isolates help prevent one isolate's failure from affecting the others, enhancing the overall stability of the application.
Working with Isolates:
1. Creating Isolates:
In Dart, creating isolates is a straightforward process. You can spawn an isolate using the Isolate.spawn
function, providing a function that represents the entry point for the isolate's execution.
dartCopy codevoid myIsolateFunction() {
// Isolate logic goes here
}
void main() async {
final isolate = await Isolate.spawn(myIsolateFunction, 'Hello from Isolate!');
}
2. Communication between Isolates:
Isolates communicate by passing messages. Dart provides a communication channel through which isolates can send and receive messages. This allows for the exchange of data between isolates while maintaining the isolation of their memory.
dartCopy codevoid myIsolateFunction(SendPort sendPort) {
// Receiving a message
sendPort.listen((message) {
print('Isolate received: $message');
});
// Sending a message
sendPort.send('Hello from Isolate!');
}
void main() async {
final receivePort = ReceivePort();
final isolate = await Isolate.spawn(myIsolateFunction, receivePort.sendPort);
}
Use Cases for Isolates:
1. Parallel Processing:
Isolates are ideal for parallelizing tasks that can be divided into independent subtasks. Whether it's image processing, data manipulation, or other CPU-intensive operations, isolates can significantly boost performance.
2. Background Tasks:
Isolates are well-suited for executing background tasks, ensuring that the main application thread remains responsive. This is particularly valuable for tasks like file I/O, network requests, or other operations that might cause delays.
3. Isolation of Resources:
Isolates provide a clean way to isolate and manage resources, minimizing the risk of resource conflicts and improving the overall stability of the application.
Conclusion:
Dart isolates are a powerful tool for concurrent programming, offering developers a straightforward way to achieve parallelism without the complexity of managing threads directly. By embracing isolates, Dart developers can create more responsive, scalable, and stable applications. As you explore isolates in Dart, consider how they can enhance the performance and efficiency of your projects. Happy coding!
Subscribe to my newsletter
Read articles from Mohamed Said directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
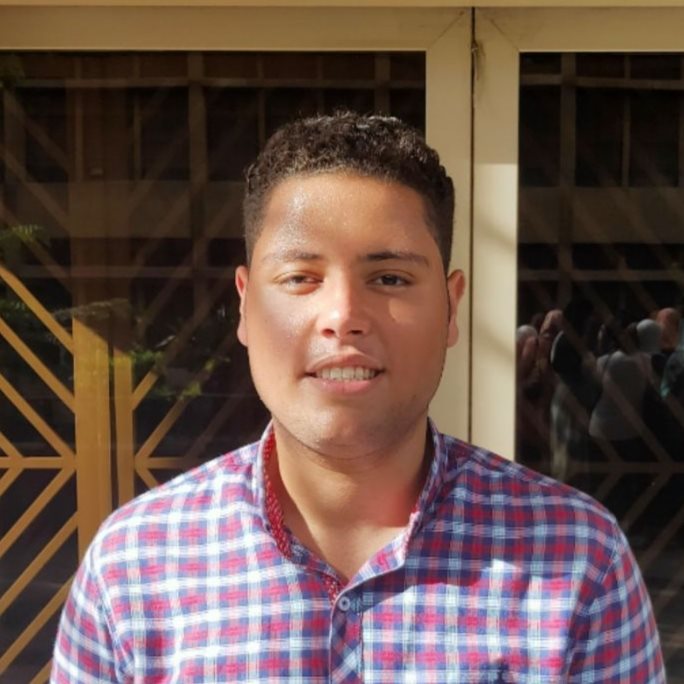
Mohamed Said
Mohamed Said
Software Engineer who is passionate about Mobile applications and backend development. With over three years of hands-on experience in developing mobile apps with Flutter. I'm looking forward to working in a challenging environment and building my knowledge about computer science.