Roman to Integer
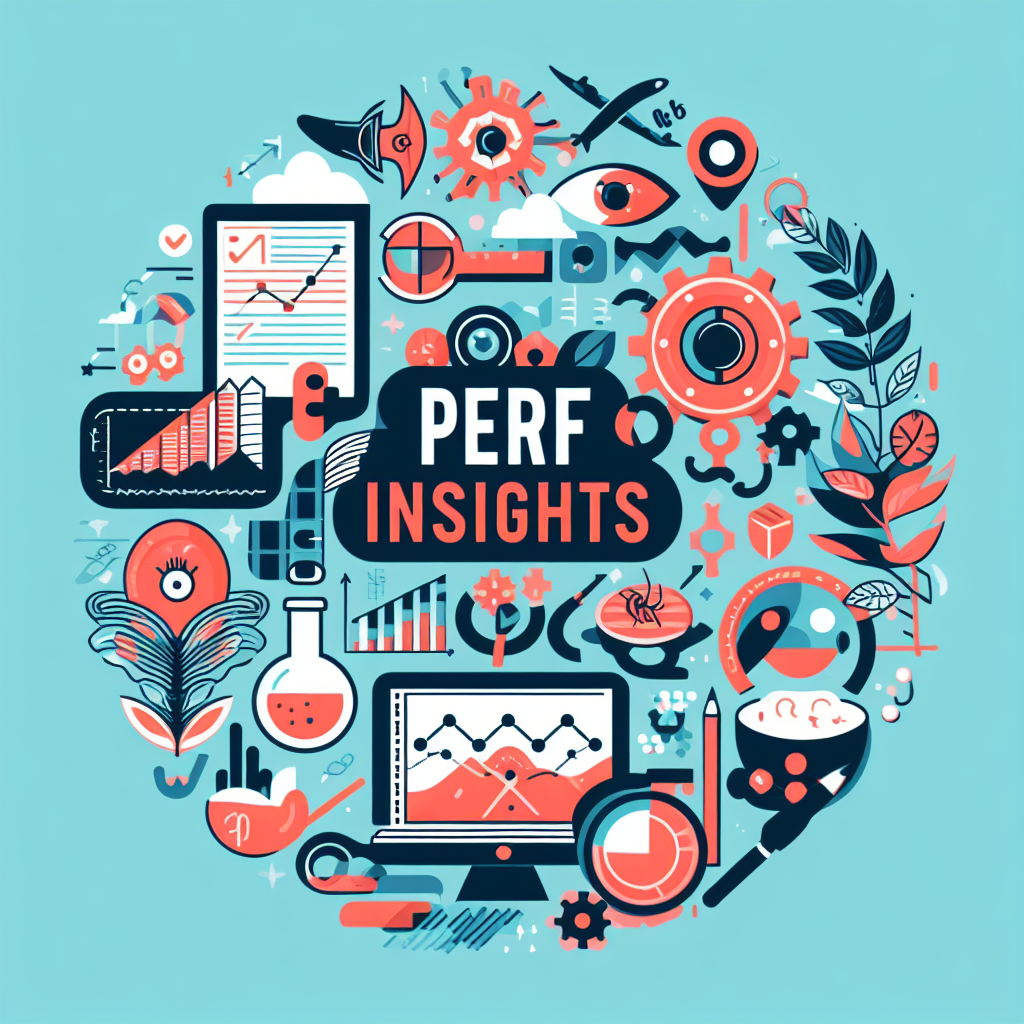
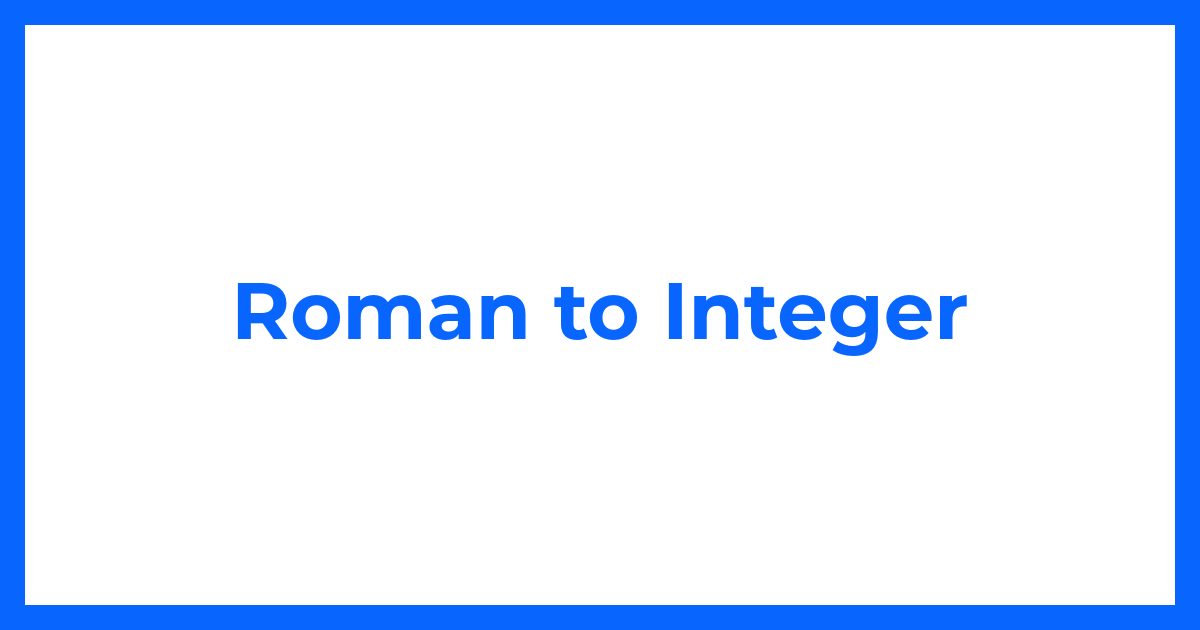
Roman numerals are represented by seven different symbols: I
, V
, X
, L
, C
, D
and M
.
Symbol Value
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, 2
is written as II
in Roman numeral, just two ones added together. 12
is written as XII
, which is simply X + II
. The number 27
is written as XXVII
, which is XX + V + II
.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not IIII
. Instead, the number four is written as IV
. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as IX
. There are six instances where subtraction is used:
I
can be placed beforeV
(5) andX
(10) to make 4 and 9.X
can be placed beforeL
(50) andC
(100) to make 40 and 90.C
can be placed beforeD
(500) andM
(1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
LeetCode Problem: Link | Click Here
class Solution {
public int romanToInt(String s) {
int sum = 0;
// Iterate through the Roman numeral string
for (int i = 0; i < s.length(); i++) {
// Get the current character
char currentChar = s.charAt(i);
// Get the next character if it exists, else set it to '\0'
char nextChar = (i + 1 < s.length() ? s.charAt(i + 1) : '\0');
// Check for special cases like IV, IX, XL, XC, CD, CM
if (currentChar == 'I' && nextChar == 'V') {
sum = sum + 4; // When 'IV' is encountered, add 4 to sum and skip the next character
i++;
} else if (currentChar == 'I' && nextChar == 'X') {
sum = sum + 9; // When 'IX' is encountered, add 9 to sum and skip the next character
i++;
} else if (currentChar == 'X' && nextChar == 'L') {
sum = sum + 40; // When 'XL' is encountered, add 40 to sum and skip the next character
i++;
} else if (currentChar == 'X' && nextChar == 'C') {
sum = sum + 90; // When 'XC' is encountered, add 90 to sum and skip the next character
i++;
} else if (currentChar == 'C' && nextChar == 'D') {
sum = sum + 400; // When 'CD' is encountered, add 400 to sum and skip the next character
i++;
} else if (currentChar == 'C' && nextChar == 'M') {
sum = sum + 900; // When 'CM' is encountered, add 900 to sum and skip the next character
i++;
} else {
// For other cases like 'I', 'V', 'X', 'L', 'C', 'D', 'M', add their respective values to sum
if (s.charAt(i) == 'I') {
sum = sum + 1;
} else if (s.charAt(i) == 'V') {
sum = sum + 5;
} else if (s.charAt(i) == 'X') {
sum = sum + 10;
} else if (s.charAt(i) == 'L') {
sum = sum + 50;
} else if (s.charAt(i) == 'C') {
sum = sum + 100;
} else if (s.charAt(i) == 'D') {
sum = sum + 500;
} else if (s.charAt(i) == 'M') {
sum = sum + 1000;
}
}
}
return sum;
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
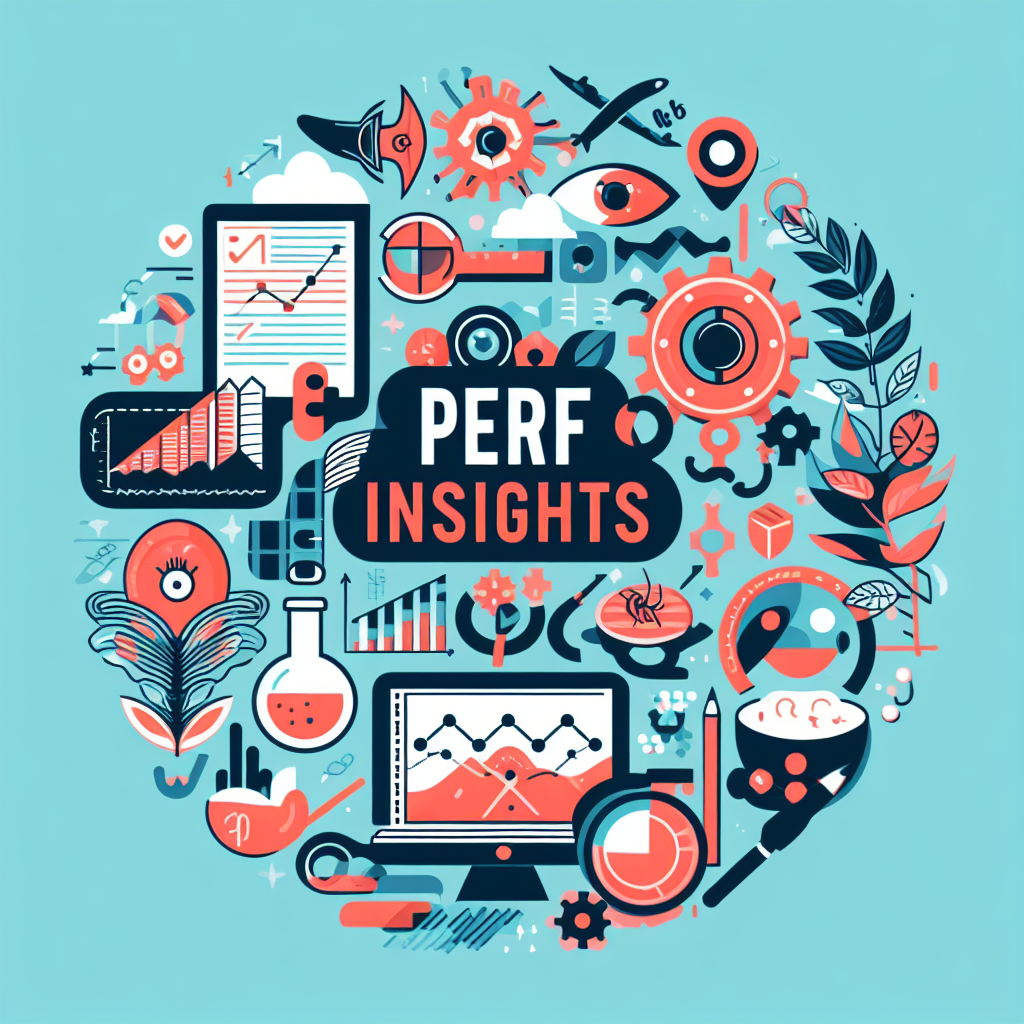
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.