Higher-Order Array Methods (map(), filter(), and reduce() ) in JavaScript in-depth
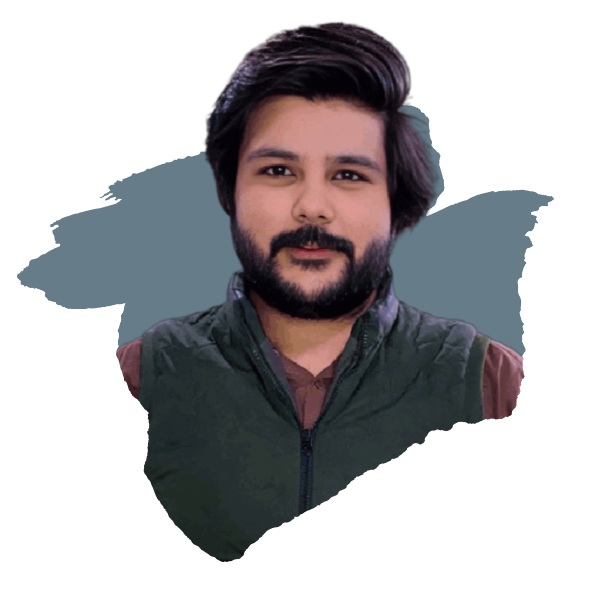
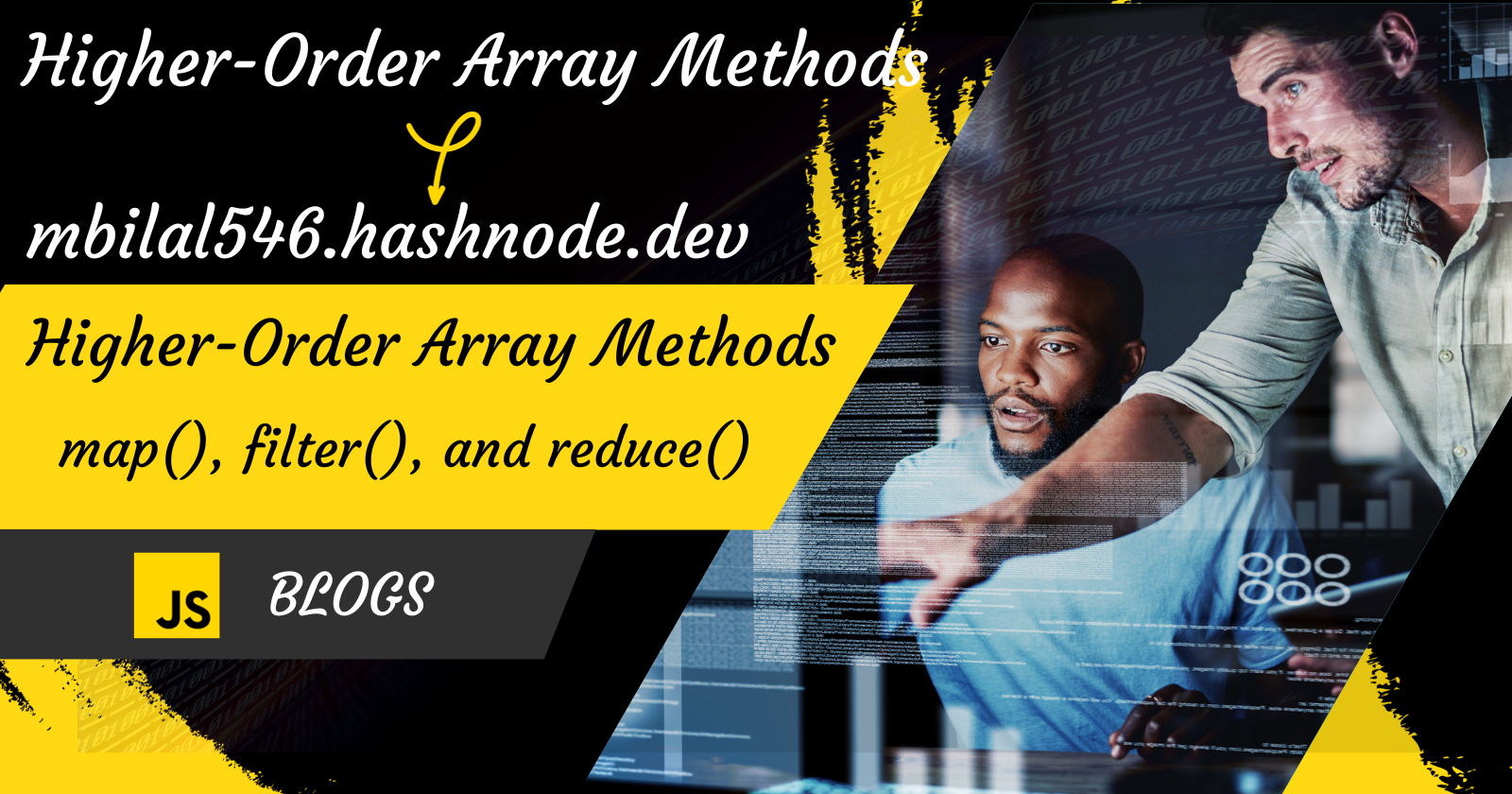
1. map()
The map()
method in JavaScript is a powerful and widely used array method. It is used to create a new array by applying a function to each element of an existing array. The original array remains unchanged. This will return the value and allow us to store it in a variable that we further use in the program. It will also allow chaining.
For Example:
let myArr = [1, 2, 3, 4, 5, 6, 7];
newNums = myArr.map( function (num){
return num * 2
} );
console.log(newNums);
Output:
[
2, 4, 6, 8,
10, 12, 14
]
// With arrow function
let myArr = [1, 2, 3, 4, 5, 6, 7];
newNums = myArr.map( (num)=>{ // arrow function
return num * 2
} );
Output:
[
2, 4, 6, 8,
10, 12, 14
]
Let's see a practical example of map()
method:
const users = [
{firstName: 'Muhammad', lastName: 'Bilal', age: 21},
{firstName: 'Tahir', lastName: 'Munir', age: 23},
{firstName: 'Usama', lastName: 'Arif', age: 22},
{firstName: 'Hamid', lastName: 'Roman', age: 25},
];
// Here in map() we call every object in users as user
// so we can access them by dot notation
const fullName = users.map( (user) => user.firstName + " " + user.lastName);
console.log(fullName);
Output: [ 'Muhammad Bilal', 'Tahir Munir', 'Usama Arif', 'Hamid Roman' ]
2. filter()
The filter()
method in JavaScript is an array method that creates a new array with all elements that pass a certain condition provided by a callback function. This method does not modify the original array; instead, it returns a new array containing only the elements that satisfy the specified condition. This will return the value and allow us to store it in a variable that we further use in the program. It will also allow chaining.
For Example:
let myArr = [1, 2, 3, 4, 5, 6, 7];
newNums = myArr.filter( (num) => num > 4 ); // condition in callback
console.log(newNums);
Output: [ 5, 6, 7 ]
You can also do it with foreach
.
For Example:
let myArr = [1, 2, 3, 4, 5, 6, 7];
let newNums = [];
myArr.forEach( (num) =>{
if (num > 4) {
newNums.push(num)
}
});
console.log(newNums);
Output: [ 5, 6, 7 ]
As you can see it is also possible by foreach
but here you have to make it logical and filter()
is easier than foreach
because this will return the value and allow us to store it in a variable that we further use in the program and also it will allow chaining. You used the filter()
many times.
Let's see a practical example of how we use map()
and filter()
in chaining.
let myArr = [1, 2, 3, 4, 5, 6, 7];
let newNums = myArr.map( (num)=> num + 2).map( (num) => (num * 2));
// first map() +2 with every element in myArr and then store it in newNums.
// Now the newNums have values [ 3, 4, 5, 6, 7, 8, 9 ]
// Second map() *2 with every element of previous map() result
// Now the newNums have values [ 6, 8, 10, 12, 14, 16, 18 ]
console.log(newNums);
Output:
[
6, 8, 10, 12,
14, 16, 18
]
Now chain the filter()
with the above example. You can write it in multiline for better readability.
For Example:
let myArr = [1, 2, 3, 4, 5, 6, 7];
let newNums = myArr.map( (num)=> num + 2)
.map( (num) => (num * 2))
.filter( (num) => num >= 14 );
// first map() +2 with every element in myArr and then store it in newNums.
// Now the newNums have values [ 3, 4, 5, 6, 7, 8, 9 ]
// Second map() *2 with every element of previous map() result
// Now the newNums have values [ 6, 8, 10, 12, 14, 16, 18 ]
// in third you chain filter() which filter the values of second map()
console.log(newNums);
Output: [ 14, 16, 18 ]
Let's see a practical example of filter()
method:
const books = [
{title: 'Book1', genre: 'Fiction', publish: 1981, edition: 2004},
{title: 'Book2', genre: 'Non-Fiction', publish: 1982, edition: 2008},
{title: 'Book3', genre: 'History', publish: 1989, edition: 2007},
{title: 'Book4', genre: 'Non-Fiction', publish: 1999, edition: 2010},
{title: 'Book5', genre: 'Science', publish: 2002, edition: 2014},
{title: 'Book6', genre: 'Fiction', publish: 1986, edition: 2010},
{title: 'Book7', genre: 'History', publish: 1987, edition: 1996},
{title: 'Book8', genre: 'Science', publish: 2009, edition: 1992},
]
// Here in filter() we call every object in books as bk
// so we can access them by dot notation
const userBooks = books.filter( (bk)=> bk.genre === "History");
console.log(userBooks);
Output:
[
{ title: 'Book3', genre: 'History', publish: 1989, edition: 2007 },
{ title: 'Book7', genre: 'History', publish: 1987, edition: 1996 }
]
// Let's filter with edition
const userBooks = books.filter( (bk)=> bk.edition >= 2010);
console.log(userBooks);
Output:
[
{ title: 'Book4', genre: 'Non-Fiction', publish: 1999, edition: 2010 },
{ title: 'Book5', genre: 'Science', publish: 2002, edition: 2014 },
{ title: 'Book6', genre: 'Fiction', publish: 1986, edition: 2020 }
]
3. reduce()
In JavaScript, the reduce()
method is used to iterate over an array and accumulate the values into a single result. It is a powerful and versatile method that can be used for a variety of tasks, including summing up values, flattening arrays, filtering elements, and more. This will return the value and allow us to store it in a variable that we further use in the program. It will also allow chaining. This has two parameters (accumulator
and currentvalue
). After the callback, we have to give an initial value
from which the accumulator has to start.
For Example:
let myArr = [1, 2, 3, 4, 5, 6, 7];
let myVal = myArr.reduce((acc, curr) => {
// Here acc used for accumulator and curr used for currentValue
return acc + curr;
}, 0);
// Here zero is the initial value to tell accumulator where to start with.
console.log(myVal);
Output: 28
Let's see console
how it proceeds.
let myArr = [1, 2, 3, 4, 5, 6, 7];
let myVal = myArr.reduce((acc, curr) => {
console.log(`Now accumulator is: ${acc} and currentValue is ${curr}`);
return acc + curr;
}, 0);
// Here zero is the initial value to tell accumulator where to start with.
console.log(myVal);
Output:
Now accumulator is: 0 and currentValue is 1
Now accumulator is: 1 and currentValue is 2
Now accumulator is: 3 and currentValue is 3
Now accumulator is: 6 and currentValue is 4
Now accumulator is: 10 and currentValue is 5
Now accumulator is: 15 and currentValue is 6
Now accumulator is: 21 and currentValue is 7
28
// With implicit return
let myVal = myArr.reduce((acc, curr) => acc + curr, 0);
console.log(myVal);
Output: 28
Let's see a practical example of reduce()
method:
const shoppingCart = [
{
itemName: 'School Bag',
price: 999,
},
{
itemName: 'Shopping Bag',
price: 1999,
},
{
itemName: 'Shoes',
price: 1500,
},
{
itemName: 'Shirt',
price: 1800,
},
]
const total = shoppingCart.reduce( (acc, items)=> acc + items.price, 0 );
// Here in reduce() we call every object in shoppingCart as items
// so we can access them by dot notation
console.log(total);
Output: 6298
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
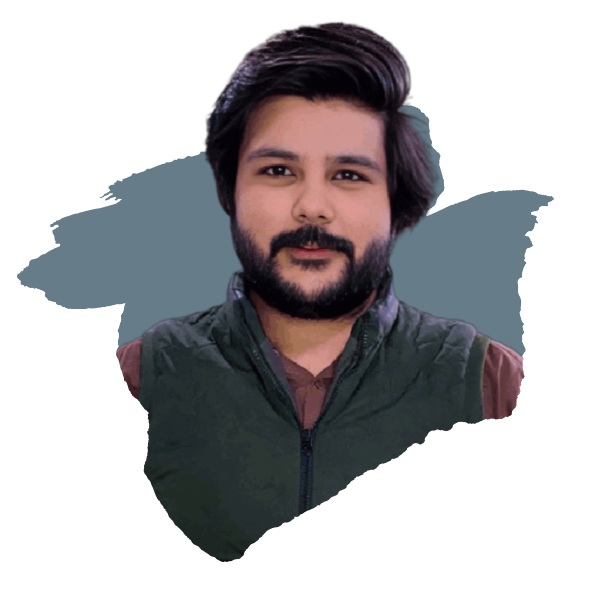
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. ๐ Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. ๐ Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub ๐ฅ Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. โจ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. ๐ Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. ๐ฅ Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.