How to create a Slack Bot using Python?
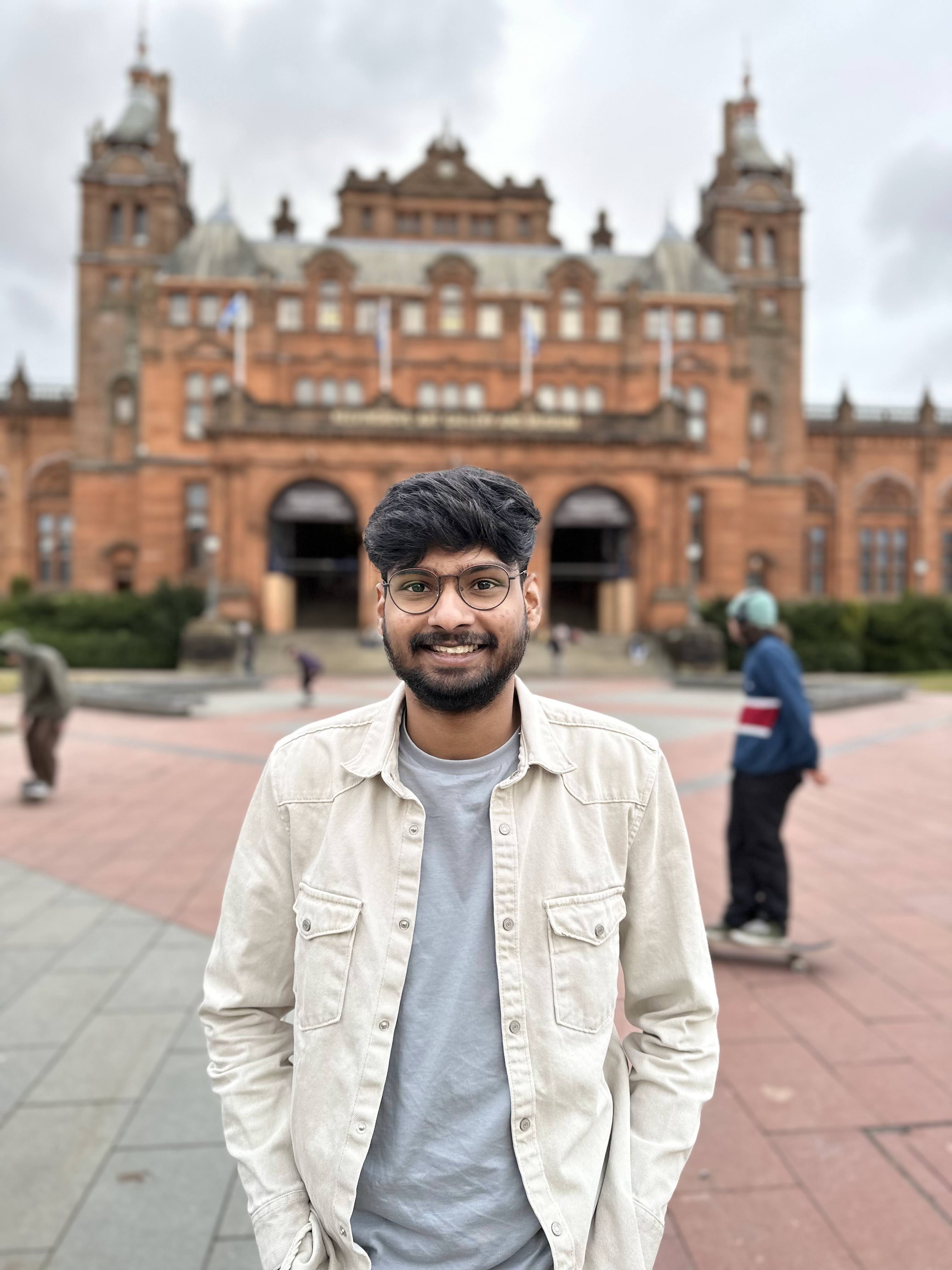
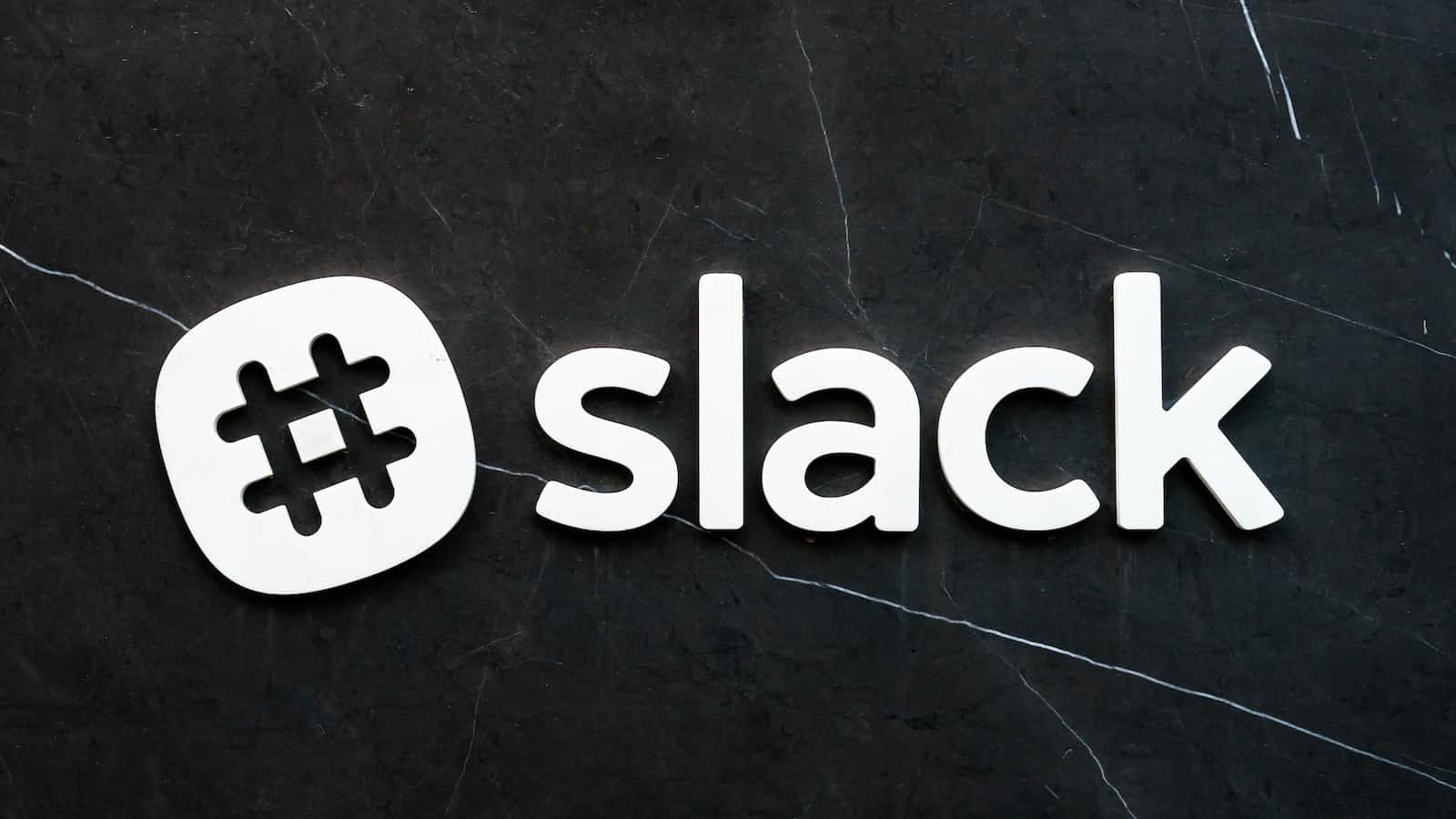
About Slack and Slack Apps 💬
Slack is like a super helpful chatroom for teams to work together. Slack apps (or bots) make it even better by doing things automatically, sharing information, and connecting with other tools. These apps help teams work smarter, get things done faster, and make Slack fit their needs perfectly.
Prerequisites
Before diving into creating your Slack bot, make sure you have a few things ready:
Python: You'll be using Python to build your bot.
Pip: This tool helps install additional components, like the Slack library.
Slack Account, Workspace, and Channel: If you don’t have a Slack account, create one. Also, create a Slack workspace and a channel in that workspace to test your new bot.
Let's begin ↗️
Head over to https://api.slack.com/apps and start by creating a new app. Name your app whatever you like and connect it to your desired workspace.
I am naming my app “JD buddy”
After creating the bot, you will find a dashboard similar to this →
Slack App permissions 🔑
To set up what your bot can do, it needs permissions. These could include things like reading and writing messages or accessing channel details.
To add permissions:
Click on OAuth & Permissions from the left menu.
Scroll down to Scopes. Inside, find Bot Token Scopes to add permissions for your bot.
Add scopes, like the ones shown below:
Here, you need to add scopes, as shown below:
Now simply install the app to your workspace. You can do this by clicking on the Install to your workspace
button at the top of this page.
Once installed, you will get a Bot User OAuth Token, Take note of this.
Let’s code 🧑💻
Go to your favorite IDE (I will be using VS Code) and create a Python file (I am naming it bot.py
).
Add the below code to your bot.py file: (I will explain the code in a bit.)
import slack
slack_token = "FILL_YOUR_SLACK_TOKEN_HERE"
slack_channel = "FILL_YOUR_SLACK_CHANNEL_HERE"
my_text = "HELLO WORLD!"
slack_client = slack.WebClient(token = slack_token)
slack_client.chat_postMessage(channel = slack_channel, text = my_text)
Now, install a library called slackclient
pip install slackclient
Note: If you are on Linux or Mac, you might need pip3 instead of pip
Create a channel and add your app to it ➕
Even though you have installed the app in your Slack workspace, you need to add the app to your channel as well. If you haven’t created a channel in your workspace, create one now. (My channel name is testing-slack-bot
)
Now add the app to your channel →
Option 1: You might see an option to “Connect your app” inside the channel. Through this, add your app to the channel
Option 2: If you can’t see the above option, right-click on the channel name and click “View channel details” (check the below image). There, inside the “Integrations” tab, you will find an option for apps. Go ahead and add your app.
Code Explanation 🗣️
This code uses the slack
library in Python to send a message to a Slack channel using a Slack bot or app.
Here's what each part does:
import slack
: This line imports the necessary functionality from the Slack library, allowing you to use Slack's features in your Python script.slack_token = "FILL_YOUR_SLACK_TOKEN_HERE"
: This variable holds the authentication token required to connect your Python script to your Slack workspace. You need to replace"FILL_YOUR_SLACK_TOKEN_HERE"
with the actual token obtained when setting up your Slack app or bot.slack_channel = "FILL_YOUR_SLACK_CHANNEL_HERE"
: Here, you specify the channel in your Slack workspace where you want to send the message. Replace"FILL_YOUR_SLACK_CHANNEL_HERE"
with the ID or name of the channel you wish to send the message to.my_text = "HELLO WORLD!"
: This variable holds the text that you want to send to the Slack channel. In this case, it's set to"HELLO WORLD!"
, but you can change it to any message you want to send.slack_client = slack.WebClient(token=slack_token)
: This line creates aWebClient
object from theslack
library and authenticates it using the Slack token provided. Thisslack_client
object will be used to interact with Slack's API.slack_
client.chat
_postMessage(channel=slack_channel, text=my_text)
: Finally, this line uses thechat_postMessage
method of theslack_client
object to send a message to the specified channel. It includes the channel ID or name (slack_channel
) where the message will be posted and the text (my_text
) that you want to send.
Remember to replace "FILL_YOUR_SLACK_TOKEN_HERE"
and "FILL_YOUR_SLACK_CHANNEL_HERE"
with your actual Slack token (Bot User OAuth Token) and channel information for the code to work properly!
Run your code🏃
Once you've set up your code, RUN IT.
Now, check your Slack channel, and you'll see your bot's message pop up!
“ModuleNotFoundError: No Module named ‘slack’”
then reinstall slackclient. For this first uninstall (pip uninstall slackclient
) then install (pip install slackclient
)Congratulations! You've successfully set up your Slack bot to send messages. Get ready to explore more functionalities in the upcoming sections!
If you face any errors, feel free to contact me at: www.twitter.com/vedantbothikar
Subscribe to my newsletter
Read articles from Vedant Bothikar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
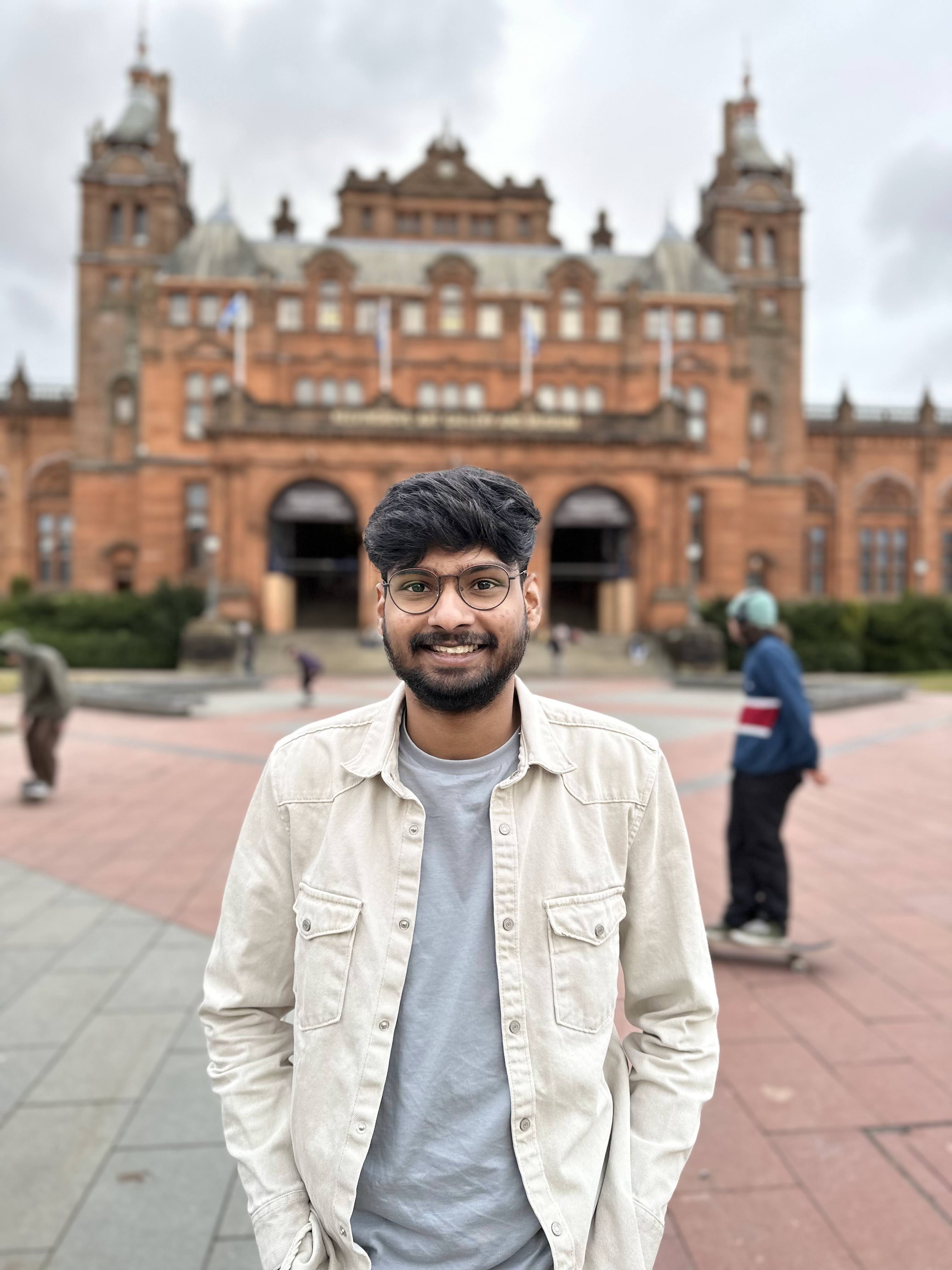
Vedant Bothikar
Vedant Bothikar
Software Engineer