jQuery Basics: A Simple Guide to Getting Started with JavaScript Enhancement
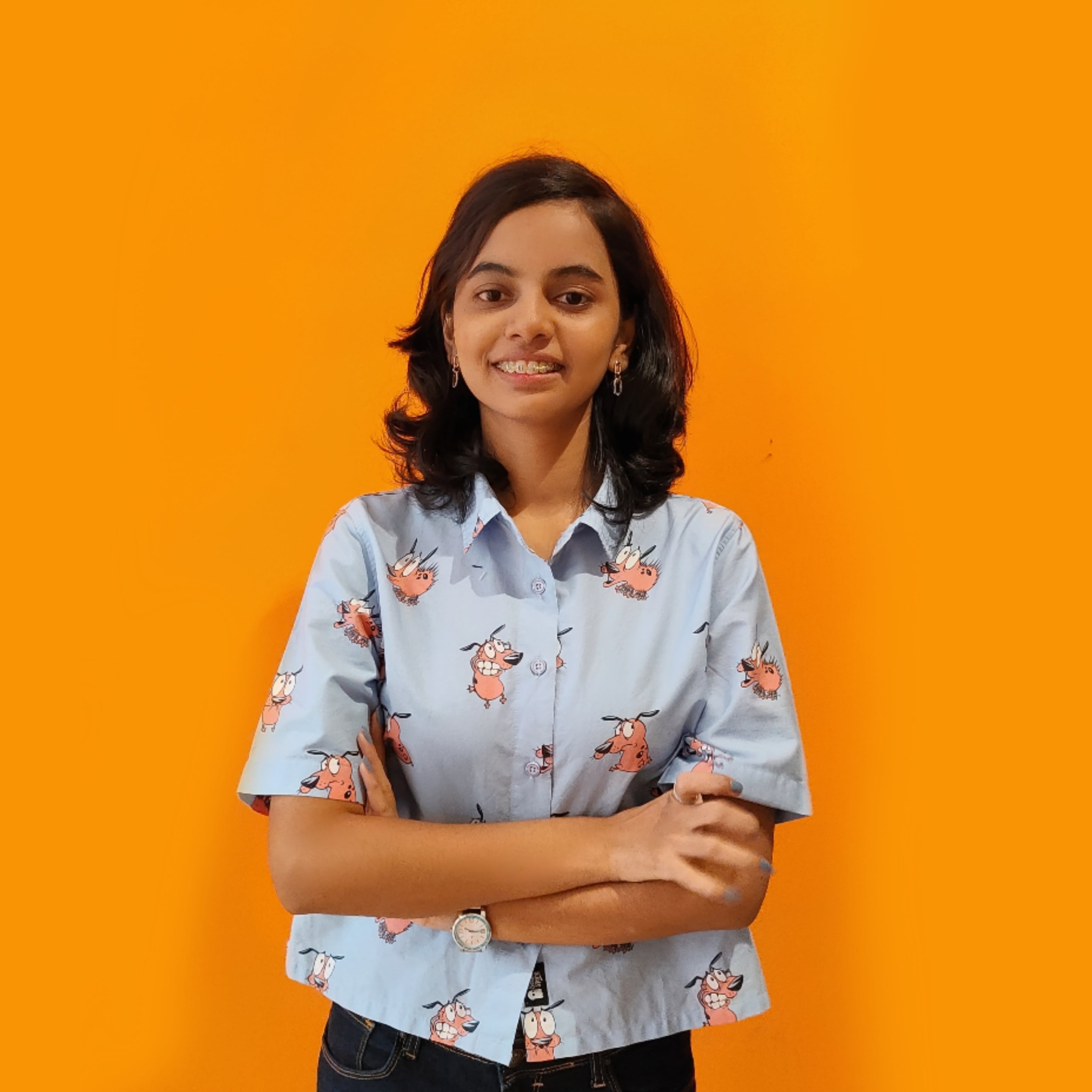
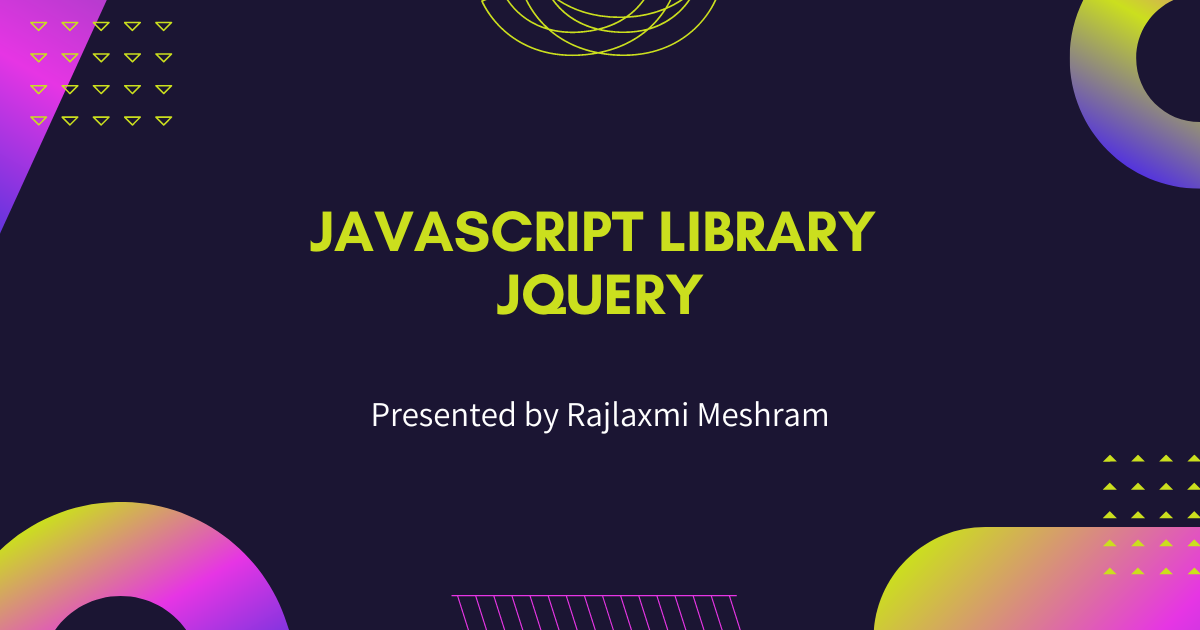
jQuery is indeed a popular JavaScript library that simplifies and streamlines the process of writing JavaScript code. It was created by John Resig in 2006, and its primary purpose is to make it easier to work with HTML documents, handle events, create animations, and perform AJAX interactions.
There are 2 ways to include JQuery into your project:
Download jQuery File
Visit the official jQuery website.
Choose the desired version, considering compressed for production and uncompressed for development.
Download the file and include it in your project.
2. Using CDN (Content Delivery Network):
Go to http://code.jquery.com/
Copy the relevant CDN link for the jQuery version you want.
Paste the link in your HTML file to fetch jQuery directly from the CDN.
3. GitHub
- jQuery is also available on GitHub at http://github.com/jquery/
Writing Code in jQuery:
Include the jQuery file before your JavaScript file in your HTML, allowing the browser to download and render jQuery before executing your code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Jquery Practice</title>
</head>
<body>
<script src="jquery-3.7.1.js"></script>
<script src="script.js"></script>
</body>
</html>
Manipulating Elements:
Use the $
(dollar sign) to fetch elements and manipulate their properties.
$(document).ready(function(){
// Your jQuery code here
});
Exploring More jQuery:
http://learn.jquery.com is a valuable resource for learning jQuery.
Creating a Fun Ball-in-the-Basket Project with jQuery
Our goal is to build a "Ball-in-the-Basket" project where users can click a button to add colorful balls to a virtual basket. To add a touch of flair, the balls will fall into the basket.
Setting Up the Basics:
Created a button labeled "Add Ball" and a container div that simulates our basket. Additionally, we include a script tag to link the jQuery library.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Jquery Practice</title>
</head>
<body>
<button id="addBallBtn">Add Ball</button>
<div id="basket"></div>
<script src="https://code.jquery.com/jquery-3.7.1.min.js"></script>
<script src="script.js"></script>
</body>
</html>
Adding Style:
To make our project visually appealing, I apply some basic styles. I define a flex container for the basket and style each ball with a circular shape and a random color.
#basket {
display: flex;
flex-wrap: wrap;
background-color: aqua;
min-height: 50px;
}
.ball {
width: 30px;
height: 30px;
margin: 5px;
border-radius: 50%;
}
jQuery Magic:
Now, I introduce jQuery to our project. I set up an event listener to detect when the "Add Ball" button is clicked. When triggered, it calls the addBallToBasket()
function. Inside this function, a random color is chosen from an array, and a new ball element is created using jQuery. This ball is then appended to the basket, dynamically updating the content of our web page.
$(document).ready(function () {
$('#addBallBtn').on('click', function () {
addBallToBasket();
});
function addBallToBasket() {
var colors = ['red', 'blue', 'yellow','black', 'orange', 'pink', 'green', 'purple'];
// Randomly pick a color from the array
var randomColor = colors[Math.floor(Math.random() * colors.length)];
// Create a ball element and append it to the basket
var $ball = $('<div>').addClass('ball').css('background-color', randomColor);
$('#basket').append($ball);
}
});
Additional jQuery Libraries:
jQuery UI:
Used to create different UI elements, enhancing the user interface of web applications.
jQuery Mobile:
Specifically designed for building mobile applications, providing a mobile-friendly framework.
Conclusion
By Following these steps and utilizing jQuery, you can enhance the development process ensure cross-browser compatibility, and create more interactive and dynamic web pages.
Subscribe to my newsletter
Read articles from rajlaxmi meshram directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
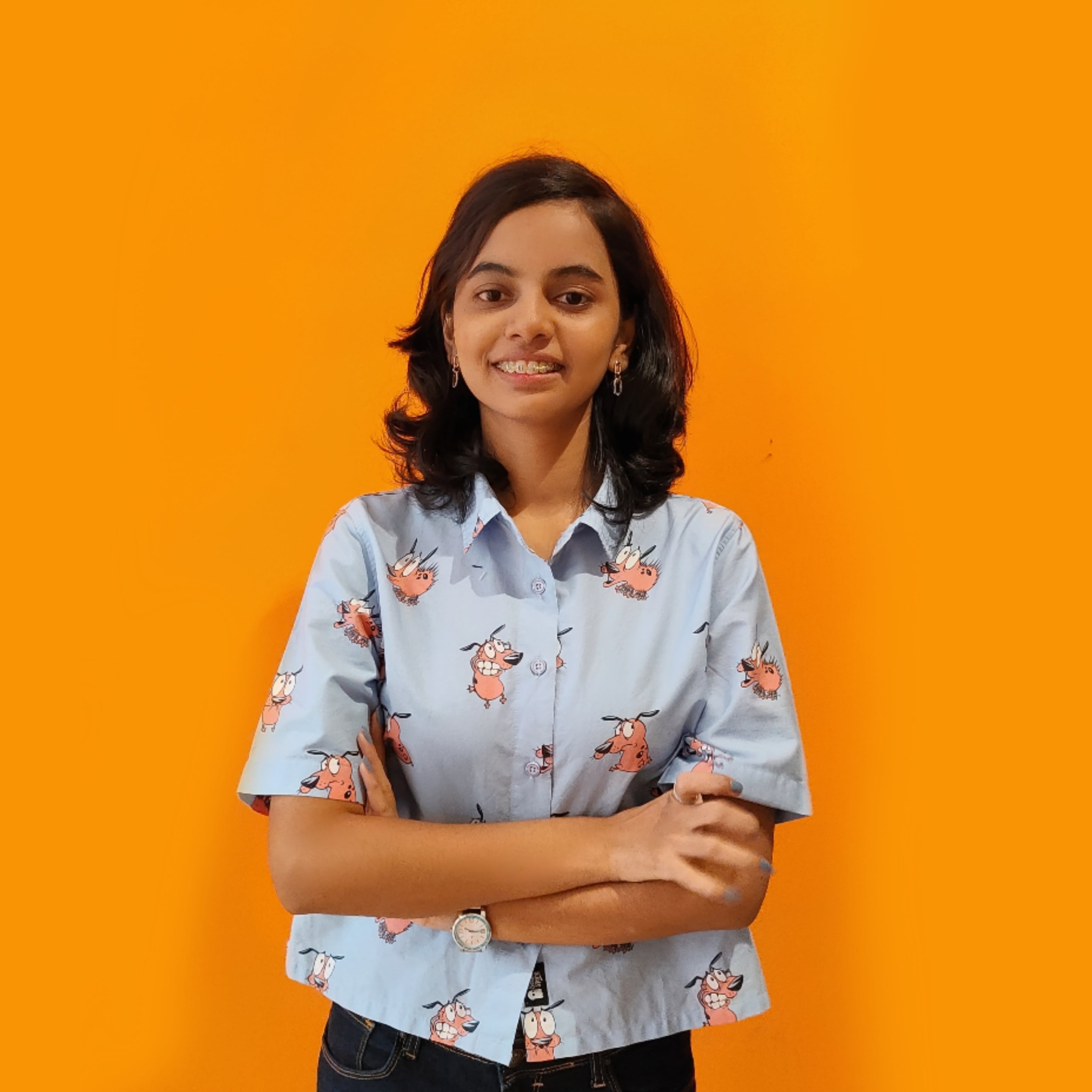
rajlaxmi meshram
rajlaxmi meshram
As a graduate student with a B.E. degree in Information Technology Engineering, I have specialized in Full Stack Development and Design. My goal is to excel in software development, both frontend and backend, and I am eager to work in a competitive environment to enhance my indispensable skill-set, essential for success in any industry. I am determined to put forth abundant effort to achieve my aspirations and soar high in my career.