ReactJS: Understanding the Difference between Props and State

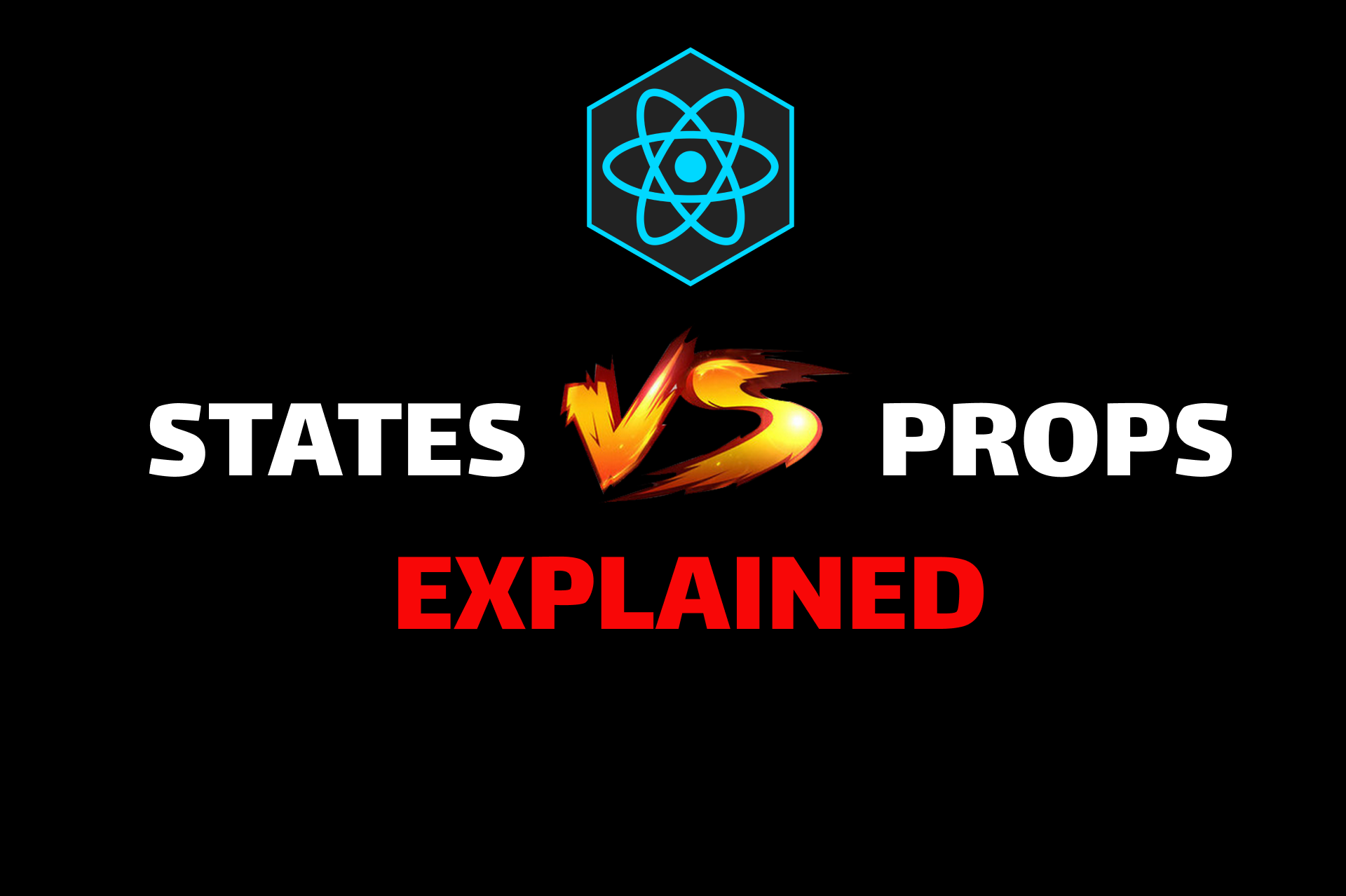
Introduction
When working with ReactJS, it's important to understand the difference between props and state. Both props and state are used to manage data within a component, but they serve different purposes. In this article, we'll explore the differences between props and state and when to use each one.
Props are short for "properties" and are used to pass data from a parent component to a child component. When a parent component renders a child component, it can pass data to that child component through props. Props are read-only, meaning that the child component cannot modify the data passed to it through props. Instead, the child component can use the data to render its own content or pass it down to its own child components.
State, on the other hand, is used to manage data within a component itself. State is mutable, meaning that the component can modify its own state. When a component's state changes, React will automatically re-render the component to reflect the new state. State is often used to manage user input, such as form data or the current state of a dropdown menu. By understanding the differences between props and state, you can make better decisions about how to manage data within your React components.
Understanding Props in ReactJS
In ReactJS, props are short for "properties" and they are used to pass data between components. Props are immutable, meaning they cannot be changed by the component that receives them. Instead, they are passed down from a parent component to a child component.
Props are commonly used to customize the appearance or behavior of a component. For example, you can pass a prop to a button component to change its color or label. You can also pass a prop to a form component to specify the initial values of its fields.
To pass a prop to a child component, you simply include it as an attribute when you render the child component. For example, if you have a child component called Button
and you want to pass it a prop called color
, you would render it like this:
<Button color="red" />
In the Button
component, you can access the color
prop by using the props
object. For example, you can use the color
prop to set the background color of the button:
function Button(props) {
return (
<button style={{ backgroundColor: props.color }}>
{props.children}
</button>
);
}
In this example, the style
attribute is used to set the background color of the button to the value of the color
prop. The children
prop is used to render the contents of the button.
Overall, props are a powerful feature of ReactJS that allow you to pass data between components and customize their appearance and behavior.
Understanding State in ReactJS
In ReactJS, state is a built-in feature that allows you to store and manage data within a component. It represents the current state of the component and can be updated over time. The state is an object that holds data that can be modified by the component itself.
One of the key features of state is that when it changes, the component will re-render, updating the view to reflect the new state. This makes state an important tool for creating dynamic and interactive user interfaces.
To define state in a React component, you need to use the useState
hook. This hook takes an initial value as a parameter and returns an array with two elements: the current state value and a function to update it.
const [count, setCount] = useState(0);
In this example, count
is the current state value, and setCount
is the function to update it. You can use setCount
to update the state value whenever you need to.
It's important to note that state should only be used for data that can change within a component. If the data is static, it should be passed down as props from a parent component.
State is a powerful feature in ReactJS that allows you to manage and update data within a component. By using the useState
hook, you can define and modify state values, which trigger re-renders and update the view.
Props vs. State: Key Differences
When building a React application, it's important to understand the differences between props and state. Both props and state are used to pass data between components, but they have some key differences.
Props
Props are short for "properties" and are used to pass data from a parent component to a child component. Props are read-only, meaning that they cannot be modified by the child component. Instead, the parent component must update the props and pass them down again.
Props are passed down as an object and can contain any type of data, including strings, numbers, arrays, and objects. Props are also used to pass down functions that can be called by the child component.
State
State is used to manage data within a component. Unlike props, state can be modified by the component itself. When state is updated, React will re-render the component and any child components that rely on that state.
State is initialized in the constructor of a component and can be updated using the setState
method. When updating state, it's important to remember that it should never be modified directly. Instead, use the setState
method to update the state.
Key Differences
The key differences between props and state are:
Props are read-only, while state can be modified by the component itself.
Props are passed down from a parent component to a child component, while state is managed within a component.
Props are used to pass data and functions from a parent component to a child component, while state is used to manage data within a component.
Understanding the differences between props and state is crucial for building React applications. By using props and state correctly, you can create reusable and maintainable components that are easy to work with.
When to Use Props
Props are used to pass data from one component to another in React. They are immutable and cannot be changed by the component that receives them. As a result, props are best used when you have data that will not change throughout the lifecycle of the component.
Props are also useful when you want to pass data down from a parent component to a child component. This is because props can only be passed down the component tree, not up. This makes it easy to manage data flow in your application.
Another use case for props is when you want to reuse a component in multiple places with different data. By passing in different props, you can reuse the same component and display different data.
When using props, it's important to keep in mind that they should only be used for data that is needed by the component to render. If a component needs to change its state based on user input or some other event, then state should be used instead.
When to Use State
In ReactJS, state is used to store and manage data within a component. It is used when the data needs to be updated and rendered dynamically based on user interaction or other events.
State is best used for data that is local to a specific component and does not need to be shared with other components. For example, if you have a form component that needs to store user input, you would use state to store that data.
State can also be used to manage the state of UI elements, such as toggling a button on and off or displaying a modal window.
When using state, it is important to remember that it should only be updated using the setState
method provided by React. Directly modifying the state object can cause unexpected behavior and should be avoided.
Additionally, because state is local to a component, it is not recommended to use it for data that needs to be shared between multiple components. In these cases, it is better to use props to pass data down from a parent component to its children.
Managing State and Props in Component Hierarchies
When building a React application, it's common to have multiple components that need to share data with one another. This is where the concepts of state and props come into play.
State refers to the internal data of a component that can change over time. It's managed within the component and can only be updated by calling the setState()
method.
Props, on the other hand, are passed down from a parent component to a child component and are read-only. They cannot be modified by the child component.
When managing state and props in component hierarchies, it's important to keep in mind the flow of data. Data should flow from parent components to child components via props, and changes to that data should be communicated back up the hierarchy via callback functions.
It's also important to avoid overuse of state and props, as this can lead to a complex and difficult-to-manage application. Instead, consider using a state management library like Redux to simplify the management of data across components.
Immutable Nature of Props
In ReactJS, props are used to pass data from a parent component to a child component. They are immutable, meaning that once they are passed down to a child component, they cannot be changed by that component. This is because React is designed to be a one-way data flow, with data flowing from parent to child.
This immutability of props ensures that the data passed down to a child component remains consistent and predictable. It also makes it easier to debug code, as you can be sure that the data passed down to a child component will not change unexpectedly.
When working with props, it is important to remember that they should not be modified directly. Instead, you should use state to manage any changes to the data. This is because state is mutable, meaning that it can be changed within a component.
Props are immutable and should not be modified directly. They are used to pass data from a parent component to a child component, and any changes to the data should be managed through state. By following these guidelines, you can ensure that your React code is consistent, predictable, and easy to debug.
Stateful vs. Stateless Components
In ReactJS, components can be classified as either stateful or stateless. Stateful components are those that have a state object, which can be updated during the component's lifecycle. On the other hand, stateless components do not have a state object and rely only on the props that are passed to them.
Stateful components are useful when you need to manage and update the state of the component. They can be used to store information that changes over time, such as user input or data fetched from an API. Stateful components use the setState()
method to update their state, which triggers a re-render of the component.
Stateless components, on the other hand, are simpler and more lightweight than stateful components. They are useful when you need to render static content or when the content is determined solely by the props passed to the component. Stateless components are also known as "dumb" components, as they do not have any logic or state of their own.
Here is a summary of the differences between stateful and stateless components:
Stateful Components | Stateless Components |
Have a state object | Do not have a state object |
Use setState() to update state | Rely only on props |
Used for managing dynamic data | Used for rendering static content |
Can be more complex and heavier | Are simpler and more lightweight |
Can have their own logic and methods | Do not have any logic or methods of their own |
Best Practices for Props and State
When working with ReactJS, it is important to follow best practices for using props and state. Here are some guidelines to keep in mind:
Props
Always pass props down from parent to child components. This makes it easier to manage and maintain the data flow in your application.
Avoid modifying props directly. Instead, create a copy of the props object and modify the copy. This helps prevent unexpected side effects.
Use PropTypes to define the expected type and shape of the props. This can help catch potential bugs early on and make your code more robust.
Keep your props simple and focused. Avoid passing down large, complex objects or arrays if possible.
State
Only use state when you need to manage data that changes over time. If the data doesn't change, it should be a prop instead.
Avoid modifying state directly. Instead, use the setState method provided by React. This ensures that the component will re-render when the state changes.
Keep your state as minimal as possible. Don't store unnecessary data in state, as it can lead to performance issues.
Use functional setState when updating state based on previous state. This ensures that the state is updated correctly, even if multiple updates happen in quick succession.
By following these best practices, you can write cleaner, more maintainable code in your ReactJS applications.
Conclusion
Props and state are fundamental concepts in React that allow you to pass data between components and manage state within components. By understanding the difference between props and state, and when to use each one, you can build React apps that are scalable, maintainable, and performant.
Key takeaways include:
Props allow one-way data flow from parent to child components. They are immutable and should not be modified directly.
State is used to manage data within a component that changes over time. State should be updated via setState() to trigger re-renders.
Use props to pass data that doesn't change, and state to manage data that does change.
Follow best practices like keeping state minimal, avoiding prop mutations, and using PropTypes for type checking.
Mastering the use of props and state is critical for building robust React applications. By adhering to unidirectional data flow and separating mutable state from immutable props, you can create well-structured components that are optimized for performance. Keep these key principles in mind as you advance your React skills.
Subscribe to my newsletter
Read articles from Sikiru directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
