Callback Function Props: Navigating Non-Linear Data Flows in React
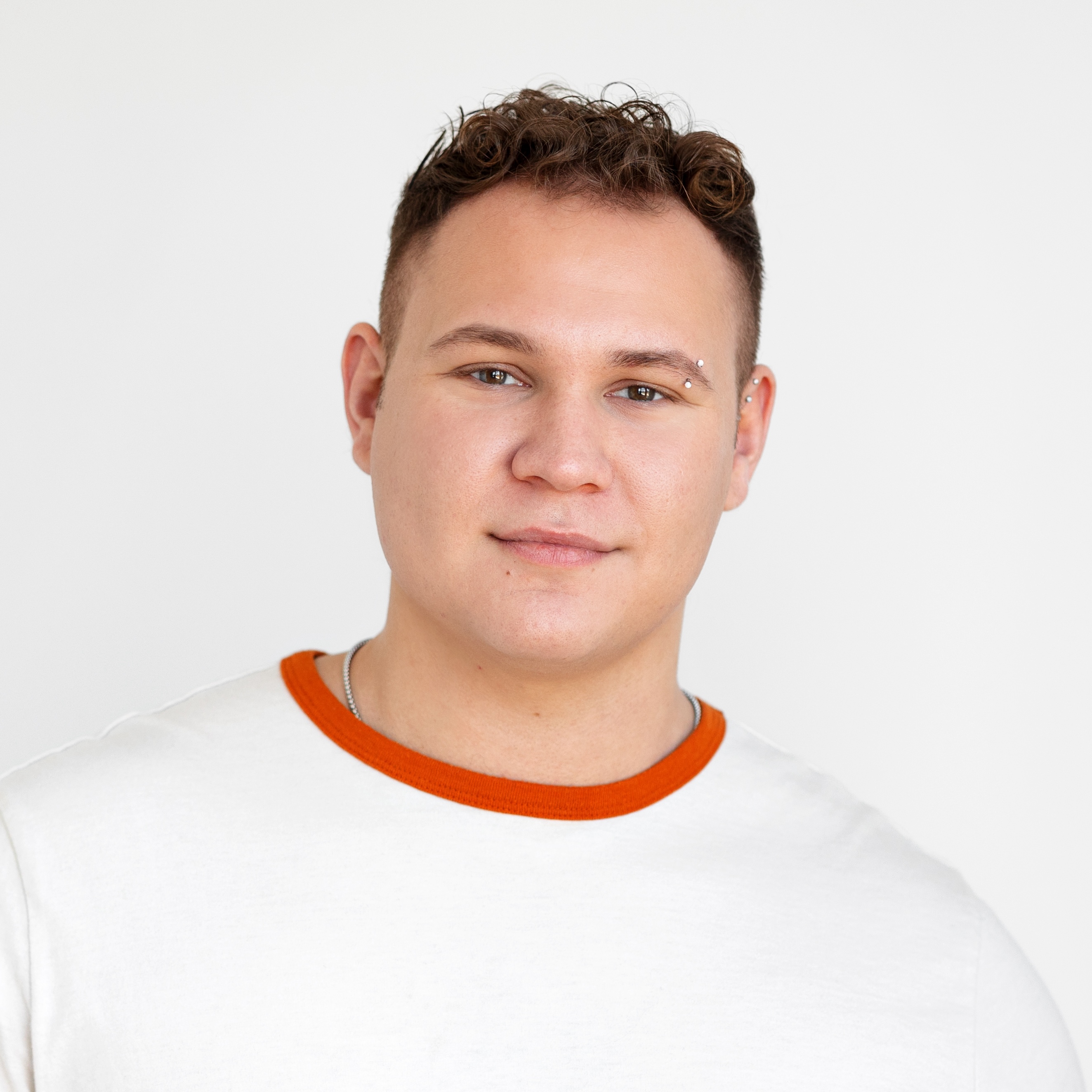
React, renowned for its component-based architecture, offers developers the flexibility to create reusable and modular UI elements. While a component typically receives data through props from its parent, the relationship isn't limited to a one-to-one connection. In this blog post, we'll explore the powerful concept of React components having multiple parent components and how each parent can pass distinct callback functions as props to achieve diverse functionality within the same component.
The Foundation: Callback Functions in React
Before diving into the multi-parent scenario, let's revisit the fundamental concept of callback functions in React. A callback function is a function passed as a prop from a parent component to a child component, allowing the child to communicate with its parent. This enables dynamic interactivity, as events within the child component can trigger actions in the parent component.
// ParentComponent1.jsx
import React from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent1() {
const handleEvent1 = () => {
console.log('Event 1 occurred!');
};
return (
<ChildComponent onEvent={handleEvent1} />
);
}
// ParentComponent2.jsx
import React from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent2() {
const handleEvent2 = () => {
console.log('Event 2 occurred!');
};
return (
<ChildComponent onEvent={handleEvent2} />
);
}
Above are ParentComponent's 1 & 2
they both have a respective handleEvent
function that is passed down to the ChildComponent
which will always display the text 'click me'. This demonstrates that componentns design and styling can remain consistant despite function changing. Functionality such as this is helpful when you want to display the same information on different webpages, but may require different functionality for the user to interact with.
// ChildComponent.jsx
import React from 'react';
function ChildComponent({ onEvent }) {
const handleClick = () => {
onEvent();
};
return (
<button onClick={handleClick}>Click me</button>
);
}
Here, ChildComponent
receives a different callback function (onEvent
) from each parent (ParentComponent1
and ParentComponent2
). Clicking the button triggers the respective event for each parent, showcasing the typical use of callback functions.
Practical Benefits: Reusability and Customization
The ability of a React component to receive different callback functions from multiple parents introduces powerful patterns for reusability and customization. Components can be designed to offer a core functionality while allowing parents to tailor their behavior as needed.
Imagine a scenario where a shared UI component, such as a modal or a form, is used across various sections of an application. Each section may require different actions to be taken when interacting with the shared component. By employing the multiple-parent, multiple-callback approach, developers can ensure that the shared component remains versatile and adaptable to diverse requirements.
Considerations and Best Practices
While this approach provides flexibility, it's essential to consider a few best practices:
Clear Documentation: Clearly document the expected callback functions for each parent component to make it easy for other developers (or even yourself) to understand how to use the shared component.
Consistent Interfaces: Maintain consistency in the interfaces of callback functions to create a predictable pattern. This makes it easier for developers to work with your components.
Keep It Simple: While multiple parents and callbacks offer flexibility, avoid overcomplicating your component hierarchy. Keep it simple and maintain a clear structure.
Abstraction is Key: When reusing components you want to make sure the ChildComponent is as abstract as possible so that the change in functionality comes solely from the ParentComponent.
Conclusion
React's component-based architecture empowers developers to create modular, reusable UI elements. By allowing components to have multiple parents, each passing different callback functions, React enables dynamic and customizable behavior. This pattern is especially valuable when building shared components that need to adapt to various contexts within an application. As you explore the possibilities of multiple parents and callbacks, keep in mind the balance between flexibility and simplicity to create robust and maintainable React applications.
Subscribe to my newsletter
Read articles from Steven Mentzer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
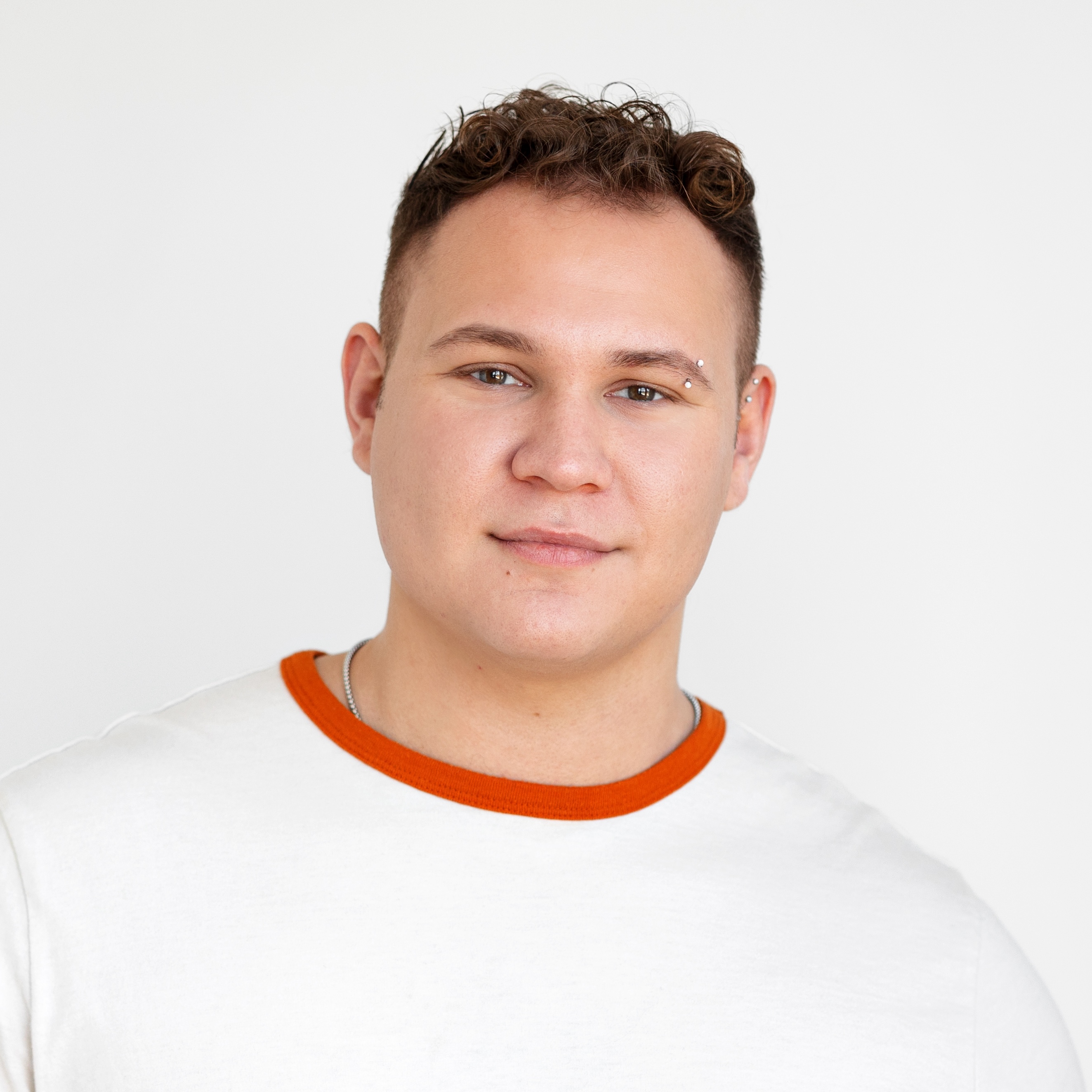
Steven Mentzer
Steven Mentzer
Hey there! ๐ Full-stack software engineer passionate about art and tech, crafting innovative solutions for today's dynamic landscape. BA in Studio Art ๐จ & cert. in Software Engineering ๐ป. Love blending creativity with precision! Currently honing skills in Swift ๐ฑ & mobile design. Let's collaborate on cool projects! ๐ก Also into weightlifting ๐๏ธโโ๏ธ, queer history ๐ณ๏ธโ๐, and board games ๐งฉ. Writing this blog to chronicle my journey into software engineering, aiming to both track my own growth and provide insights for fellow learners. Let's embark on this coding adventure together, learning and growing every step of the way!