Demystifying Ruby's State Machine: Managing User Authentication States

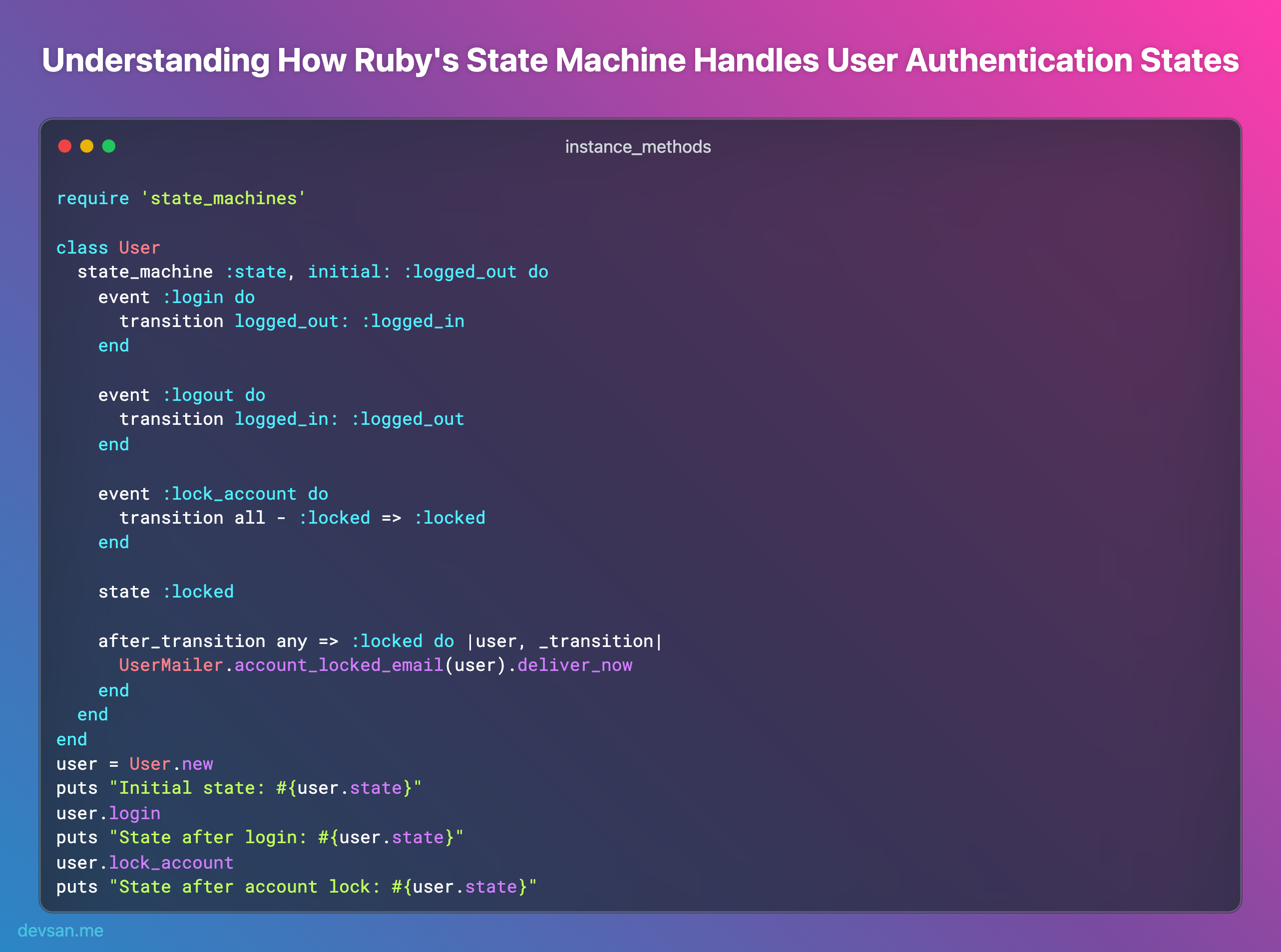
Welcome to an exploration of Ruby's state machine gem! Today, we're unraveling the power of state management in a straightforward scenario: user authentication states.
Setting the Stage: User Authentication States
Imagine a simplified user authentication system. We're interested in managing the authentication states a user goes through—logged out, logged in, and locked—using Ruby's state machine gem.
The Ruby Magic: Implementing State Transitions
Let's delve into the code snippet to illustrate how the state machine handles user authentication states:
require 'state_machines'
class User
state_machine :state, initial: :logged_out do
event :login do
transition logged_out: :logged_in
end
event :logout do
transition logged_in: :logged_out
end
event :lock_account do
transition all - :locked => :locked
end
state :locked
after_transition any => :locked do |user, _transition|
UserMailer.account_locked_email(user).deliver_now
end
end
end
Understanding the User Authentication States
In this code snippet, the User
class employs Ruby's state machine gem to manage authentication states:
Events and Transitions: Events like
:login
,:logout
, and:lock_account
facilitate transitions between user states.State-Specific Behavior: The
:locked
state triggers an email notification usingUserMailer
when a user's account is locked.Simplified Logic: The state machine simplifies the logic governing state transitions in the user authentication process.
A Sneak Peek into the Code Execution
Let's envision a scenario where a user interacts with this simplified authentication system:
user = User.new
puts "Initial state: #{user.state}"
user.login
puts "State after login: #{user.state}"
user.lock_account
puts "State after account lock: #{user.state}"
Conclusion: Simplifying State Management
Ruby's state machine gem offers an elegant way to manage and visualize state transitions in user authentication. By encapsulating state logic, it simplifies the handling of complex state changes.
Feel free to explore and adapt this simplified example! Experiment with different transitions to understand how the state machine manages user authentication states effortlessly.
Tags: #Ruby #StateMachine #UserAuthentication #CodeExploration LinkedIn
Subscribe to my newsletter
Read articles from sandip parida directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

sandip parida
sandip parida
Hi there! 👋 I'm sandip parida, a passionate fullstack software developer who loves to learn and work with new technologies. #ruby #rails #nodejs #aiapps #openai #ai #iot