JavaScript program to find Compound Interest
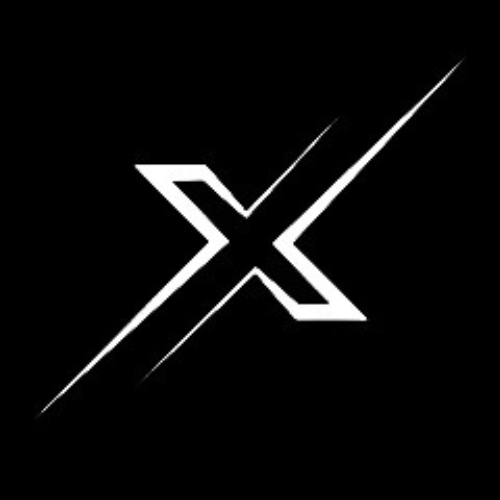
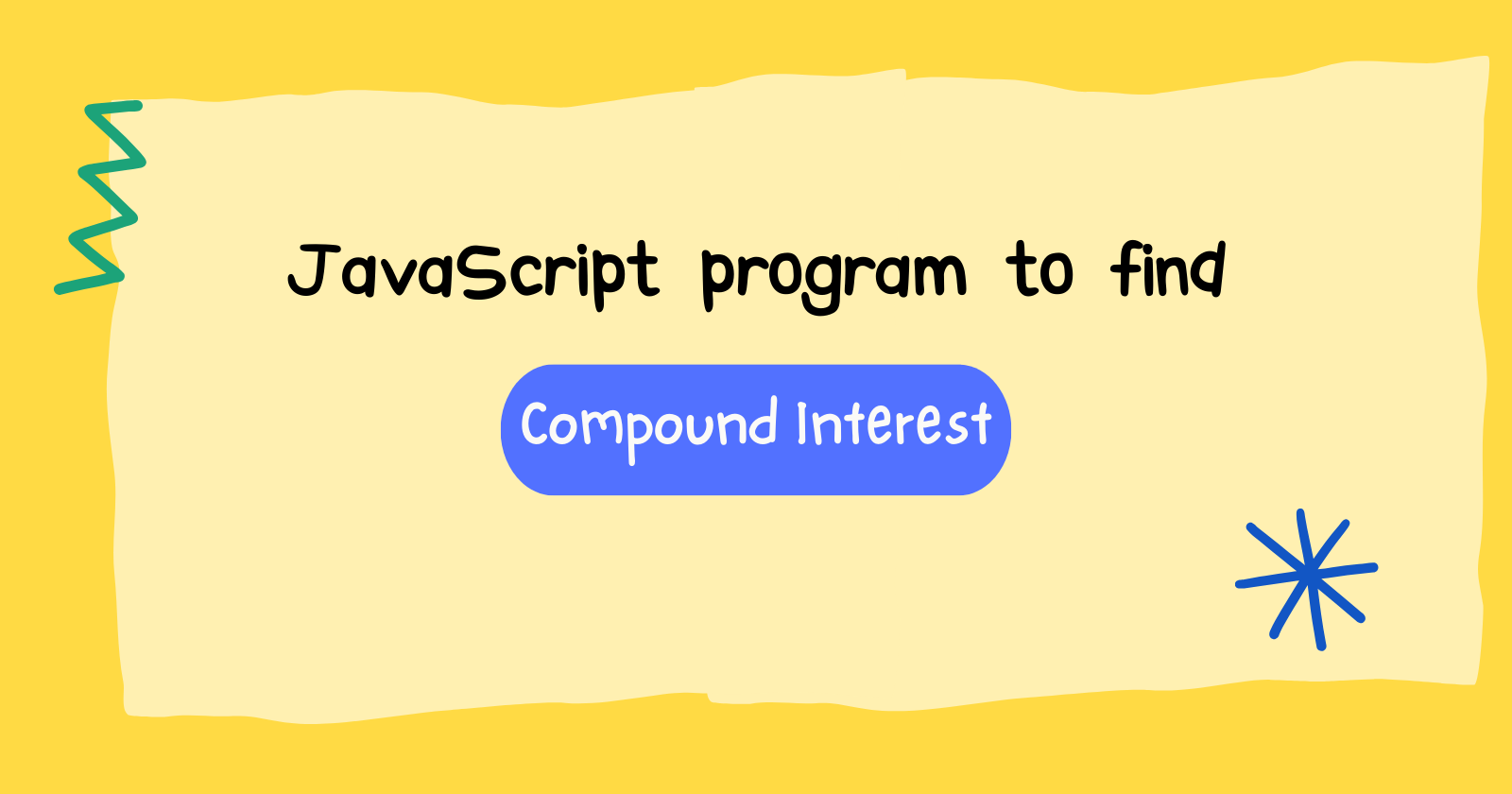
Hi guys, today I was solving some aptitude problems and suddenly an idea struck to my mind, why not create programs that'll solve aptitude problems. So letting my intrusive thoughts win, I created this basic compound interest calculator using our beloved JavaScript. So let's begin without delay....
Compound Interest:
Compound interest is the interest paid on both principal and existing interest. Hence, it is usually termed "interest over the interest". Here, the interest so far accumulated is added to the principal and the resulting amount becomes the new principal for the next interval. i.e., Compound Interest = Interest on principal + Interest over existing interest.
Compound Interest formula: Formula to calculate compound interest annually is given by:
Amount= P(1 + R/100)t
Compound Interest = Amount - P
Where,
P is the principal amount
R is the rate and
T is the time span
Pseudo Code:
Input principal amount. Store it in some variable say principal.
Input time in some variable say time.
Input rate in some variable say rate.
Calculate Amount using the formula,
Amount = principal * (1 + rate / 100) time).
Calculate Compound Interest using Formula.
Finally, print the resultant value of CI.
Example:
function calculateCompoundInterest(principal, rate, time) {
// Assuming interest is compounded annually (n = 1)
const n = 1;
// Convert the annual interest rate to a decimal
const r = rate / 100;
// Calculate the compound interest
const compoundInterest = principal * Math.pow((1 + r / n), n * time) - principal;
return compoundInterest;
}
const principalAmount = 1000; // Initial investment
const annualInterestRate = 5; // Annual interest rate (5%)
const numberOfYears = 3; // Time in years
const interest = calculateCompoundInterest(principalAmount, annualInterestRate, numberOfYears);
console.log(`Compound Interest after ${numberOfYears} years: $${interest.toFixed(2)}`);
Output:
Compound Interest after 3 years: $157.63
In this program, the calculateCompoundInterest
function takes three parameters: principal
(initial investment), rate
(annual interest rate), and time
(time in years). The function then calculates the compound interest using the compound interest formula.
Example with UI:
Here is an example using HTML, CSS & JavaScript that'll do the job -
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Compound Interest</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="content">
<h3>Compound Interest Calculator</h3>
<label for="principal">Principal Amount ($):</label>
<input type="number" id="principal" placeholder="Enter principal amount">
<label for="rate">Annual Interest Rate (%):</label>
<input type="number" id="rate" placeholder="Enter annual interest rate">
<label for="time">Time (Years):</label>
<input type="number" id="time" placeholder="Enter time in years">
<button onclick="calculateCompoundInterest()">Calculate</button>
<div id="result"></div>
</div>
<script src="script.js"></script>
</body>
</html>
Styling:
body {
font-family: 'Arial', sans-serif;
background-color: #f4f4f4;
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
.content {
width: 300px;
padding: 20px;
background-color: #fff;
border-radius: 8px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
}
h3 {
color: #333;
text-align: center;
}
label {
display: block;
margin: 10px 0 5px;
color: #555;
}
input {
width: 100%;
padding: 8px;
margin-bottom: 15px;
box-sizing: border-box;
}
button {
width: 100%;
padding: 10px;
background-color: #4caf50;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
#result {
margin-top: 15px;
font-weight: bold;
color: #333;
}
JavaScript :
function calculateCompoundInterest() {
const principal = parseFloat(document.getElementById('principal').value);
const rate = parseFloat(document.getElementById('rate').value);
const time = parseFloat(document.getElementById('time').value);
const compoundInterest = principal * Math.pow((1 + rate / 100), time) - principal;
document.getElementById('result').innerHTML = `Compound Interest: $${compoundInterest.toFixed(2)}`;
}
Output :
Time Complexity: O(1), as there is no loop used so constant time.
Auxiliary Space: O(1).
Conclusion:
Creating Compound Interest Calculator using JavaScript is both fun and creative way to hone your programming skills, you get to build UI, interact with DOM, and use mathematical formula to get output. This program is must recommended if you're a beginner in JavaScript and want to try out something new.
If you found this blog useful ,please follow and do share this post. It'll motivate me to create more posts like this.
Subscribe to my newsletter
Read articles from Aditya Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
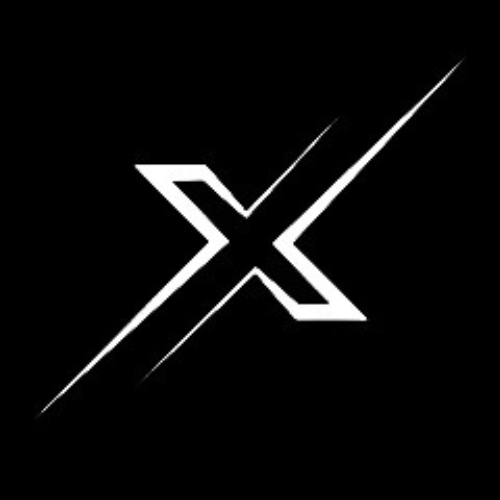
Aditya Sharma
Aditya Sharma
I am a web developer from Bengaluru, India. My mission is to turn your vision into a compelling digital experience. I'm here to collaborate and bring your ideas to life. Let's build something amazing together!