Safe Password Hashing For PHP
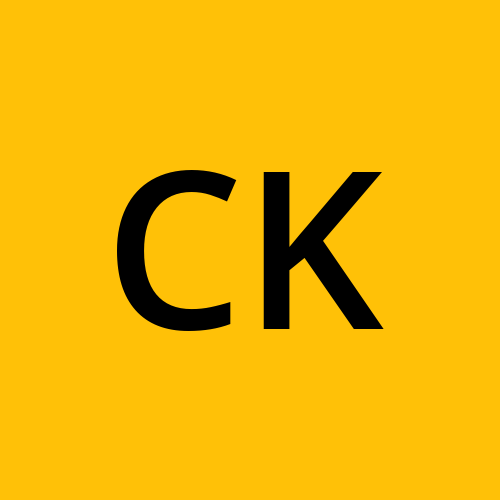
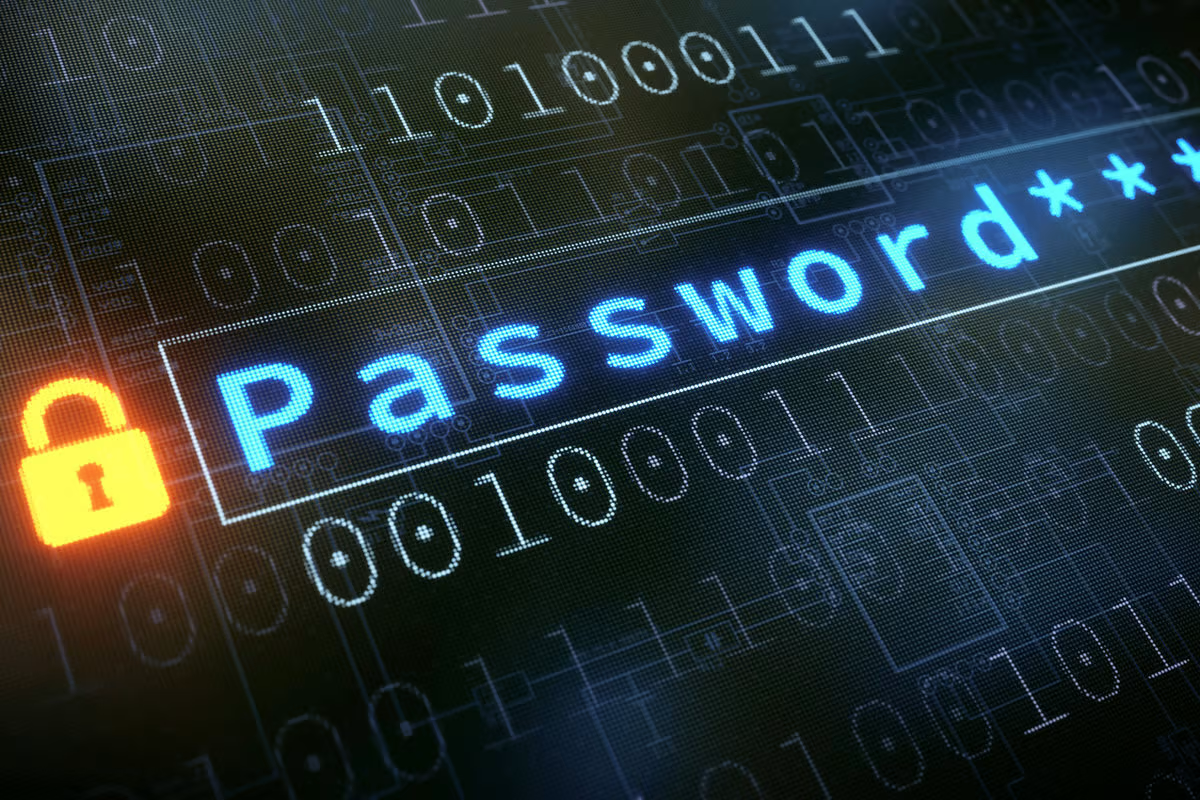
When it comes to password hashing in PHP, it is crucial to use a secure and up-to-date method to protect user credentials. PHP provides a built-in function called password_hash()
that is designed for secure password hashing using bcrypt.
Here's a basic example of how to use password_hash()
:
// User's password
$userPassword = "user123";
// Hash the password
$hashedPassword = password_hash($userPassword, PASSWORD_BCRYPT);
// Store $hashedPassword in the database
In this example, PASSWORD_BCRYPT
is the algorithm used for hashing. The password_hash()
function automatically generates a random salt and incorporates it into the hash. The resulting hash includes information about the algorithm used, cost parameter, and the actual hashed value, making it self-contained and easy to store in a database.
To verify a user's password during login, you can use the password_verify()
function:
// User's entered password during login
$enteredPassword = "user123";
// Retrieve the hashed password from the database based on the username or email
// For example: $hashedPassword = getHashedPasswordFromDatabase($usernameOrEmail);
// Verify the entered password against the stored hash
if (password_verify($enteredPassword, $hashedPassword)) {
// Password is correct
echo "Login successful!";
} else {
// Password is incorrect
echo "Login failed. Invalid password.";
}
Make sure to use prepared statements or an ORM (Object-Relational Mapping) library when interacting with the database to prevent SQL injection attacks.
Additionally, consider using the password_needs_rehash()
function periodically to check if a password needs to be rehashed. This is important because it allows you to update the hash with a stronger algorithm or cost parameter without requiring the user to change their password:
if (password_needs_rehash($hashedPassword, PASSWORD_BCRYPT)) {
// Generate a new hash and update it in the database
$newHashedPassword = password_hash($enteredPassword, PASSWORD_BCRYPT);
// Update $newHashedPassword in the database
}
Remember to stay informed about the latest best practices for password hashing and adjust your implementation accordingly. As of my knowledge cutoff in January 2022, using password_hash()
with bcrypt is a secure choice, but it's always good to check for any updates or changes in best practices.
Subscribe to my newsletter
Read articles from Cafer Kara directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
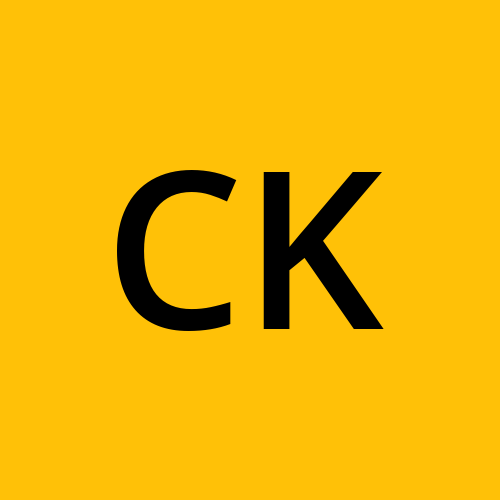