Setting tsconfig alias paths
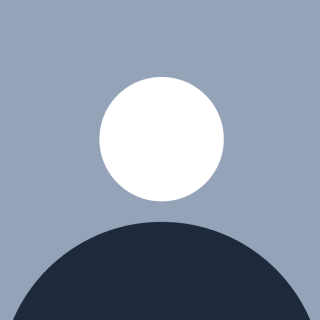
Introduction
When importing certain components or files, the deeper the path goes, the longer the relative path can be, depending on the location of the file you want to import.
// e.g. sample
import deep from '../../../../../../../A/B/C/file.js';
// e.g. too many paths
import deep1 from '../../../../../../../A/B/C/file1.js';
import deep2 from '../../../../../../../A/B/C/file2.js';
import deep3 from '../../../../../../../A/B/C/file3.js';
import deep4 from '../../../../../../../A/B/C/file4.js';
import deep5 from '../../../../../../../A/B/C/file5.js';
...
...
import deep999 from '../../../../../../../A/B/C/file999.js';
Longer relative paths like this not only make it harder to find the files to import, but they're also less readable.
You can set an absolute path in tsconfig.json
or jsconfig.json
.
What is tsconfig.json?
It's a file that allows you to configure settings for typescript, and if your repository is in javascript, use jsconfig.json
.
If the repository is using Typescript, tsconfig.json
must exist in the root directory and is loaded at compile time via the tsc
command.
In addition to setting options for the compiler, it provides a number of other features.
// e.g. sample tsconfig.json
{
"compilerOptions": {
...
},
"include": [...],
"exclude": [...]
}
Setting an absolute path
In tsconig.json
set the absolute path using baseUrl and paths in the compilerOptions option.
baseUrl is an option that must be set in order to set paths.
In the case of paths, it is a key-value object. Enter the name of the path you want to define as an absolute path in key, and enter the path relative to baseUrl
in value.
The key of paths usually starts with @
, followed by a special character such as /
or _
, because there are often packages that start with @
.
// tsconfig.json
{
"compilerOptions": {
"baseUrl": ".", // In this case, the reference is to the root directory where the tsconfig.json file exists.
"paths": {
"@_components/*": ["src/components/*"],
"@_apis/*": ["src/api/*"],
"@_types/*": ["src/types/*"],
"@_icons/*": ["public/assets/icons/*"],
"@_images/*": ["public/assets/images/*"],
"@_emojis/*": ["public/assets/emoji/*"]
}
}
}
If you specify paths as above, the actual structure relative to the root directory will look like this.
Root
├─ public
│ └─ assets
│ ├─ icons
│ ├─ images
│ └─ emojis
└─ src
├─ components
├─ api
└─ types\
Let's see an example in action.
import path1 from '@_components/home/main.tsx';
import path2 from '@_icons/sample.png';
import path2 from '@_apis/member'
You can specify modules as absolute paths as well as specific files.
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"jquery": ["node_modules/jquery/dist/jquery"]
}
}
}
Tip
If you want to add more absolute paths, you can use the extends option in tsconfig.json
to remove them.
The default config file is read and overridden by the files specified in extends. For files defined in include or exclude, the default config file is overwritten (overwrite).
The extends option is only accepted as a string. If you want to extend multiple config files, you need to create a line-by-line structure.
// tsconfig.a.json
{
"compilerOptions": {
...
},
}
// tsconfig.b.json
{
"compilerOptions": {
...
},
"extends": "./tsconfig.a.json"
}
// tsconfig.json
{
"compilerOptions": {
...
},
"extends": "./tsconfig.b.json"
}
How to use.
Create and save the file you set up above.
The file can be named anything and can be in any directory. It just needs to be in json format.
In the following example, we create tsconfig.path.json in the root directory.
// tsconfig.paths.json
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"@_components/*": ["src/components/*"],
"@_apis/*": ["src/api/*"],
"@_types/*": ["src/types/*"],
"@_icons/*": ["public/assets/icons/*"],
"@_images/*": ["public/assets/images/*"],
"@_emojis/*": ["public/assets/emoji/*"]
}
}
}
Add the extends
option to tsconfig.json
and enter the json you want to extend as a relative path to the file tsconifg.json
.
// tsconifg.json
{
"compilerOptions": {
...
},
"extends": "./tsconfig.paths.json"
}
References
Subscribe to my newsletter
Read articles from le jack directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
le jack
le jack
Web Front end Engineer