Building Stylish Node.js CLI Applications
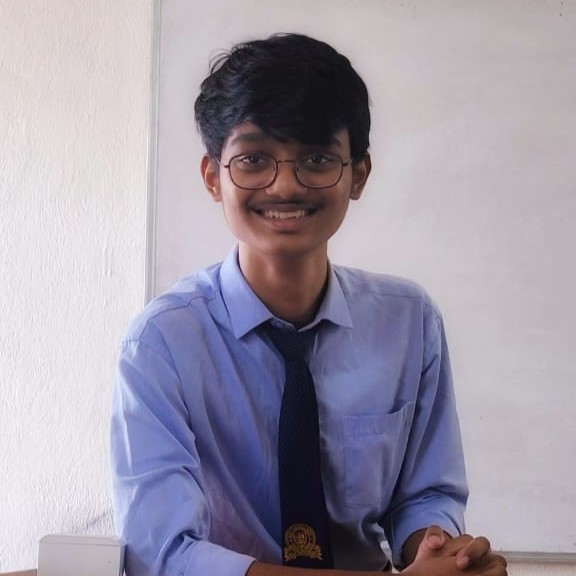
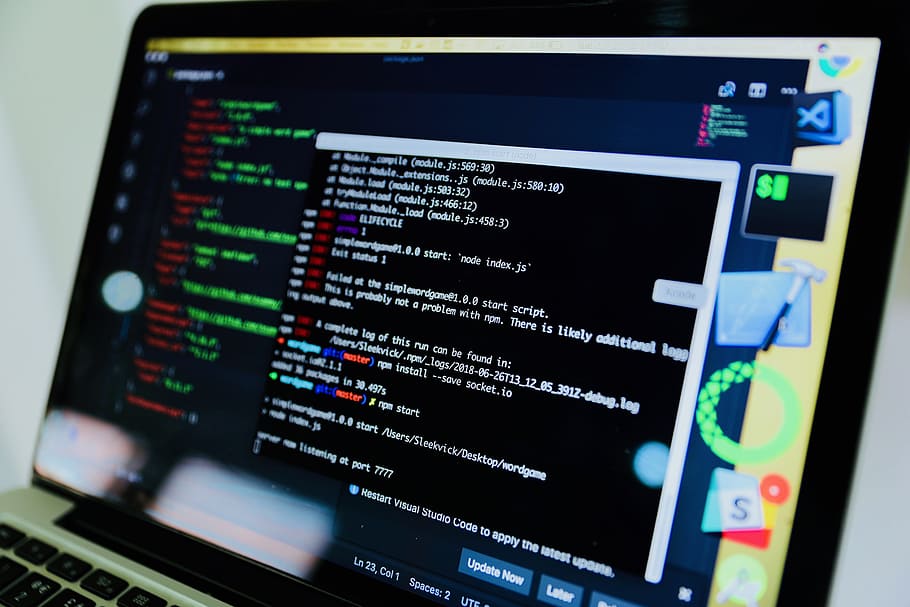
Welcome to the wonderful world of Command Line Interface (CLI) ... where you can make powerful and versatile tools as a developer. One can use CLI tools for easy debugging, making custom scripts, and presentable logging in the console, and also for showing off your cool terminal to your friends ๐ง๐ฝโ๐ป๐โจ.
So let's begin our journey ... using npm modules like Inquirer, Figlet, and Chalk.
Getting Started
Setting up the Project
Let's set up a npm project named cli-project
mkdir cli-project
cd cli-project
npm init -y
Make sure that your generated package.json looks some think like this:
{
"name": "cli-project",
"version": "1.0.0",
"main": "index.js",
"type": "module",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "node index.js"
},
"keywords": [],
"author": "",
"license": "ISC",
"description": ""
}
Install the necessary packages:
npm install inquirer figlet chalk
Now, let's dive into the main components of our CLI application. We are making a Dictionary Application using**:**
Inquirer Module: used for taking custom user input in different forms like checkboxes, inputs, password inputs, etc.
Chalk Module: used for styling and adding color to the text
Figlet Module: used for making large letters out of ordinary text
We are gonna be making this...๐
index.js file
First, let's import all the modules:
#!/usr/bin/env node
import inquirer from "inquirer";
import chalk from "chalk";
import figlet from "figlet";
Now we have to determine how to make a dictionary application... this code will fetch the meaning and the phonetic details of a word.
We are also using chalk to set the text color to green in this case.
figlet("Dictionary", { font: "Banner3-D" },
(err, result) => {
if (err) {
console.log("Hi I am Vishnu Prasad Korada");
} else {
console.log("\n\n" + chalk.green(result));
}
});
We will write the rest of the code in this function.
async function main() {
// we will write the rest of the code in this function
// ask the user for word
// fetch the data using API
// display the details
}
We will use the inquirer module to input the word in this way. We can also check for invalid inputs. We will write the rest of the code in this function.
// ask the user for word
const answer = await inquirer.prompt({
name: "word",
type: "input",
message: "\nEnter the word:"
});
if (["", " "].some((e) => e === answer.word)) {
throw new Error(chalk.red("I need a word to find its meaning ๐๐"));
}
We will use a free dictionary API to fetch the details of the word, notice that the error texts will be logged in red. To get the exact data like meaning and any other details we can access the properties from the response data we got from fetch.
We also used chalk to customize the output.
console.log(chalk.cyan(`\nFetching ${answer.word} Meaning....`));
// accessing the input word as answer.word
let res = await fetch(`https://api.dictionaryapi.dev/api/v2/entries/en/${answer.word}`);
let data = await response.json();
// if any error arises
if (!response.ok) {
throw new Error(chalk.red("Word not Found"))
}
// getting the things we want from data
const meaning = data[0]["meanings"][0]["definitions"][0]["definition"];
const phonetics = data[0]["phonetic"];
// printing the
console.log(chalk.bold.yellow(`Fetched ${answer.word} meaning..`));
console.log(chalk.bold.magenta("Meaning: ") + chalk.cyan(meaning));
console.log(chalk.bold.magenta("Phonetics: ") + chalk.cyan(phonetics));
Finally, just a call to the main() function is required. We call it after 1 second to let the ASCII art out and then the main() starts.
setTimeout(main, 1000);
Our dictionary Application is done...!!๐๐
To execute this script anywhere add a bin section in your package.json
file and run npm link
. The command will be the name of the project in the package.json
.
{
"name": "cli-project",
"version": "1.0.0",
"description": "",
"main": "index.js",
"type": "module",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"chalk": "^5.3.0",
"figlet": "^1.7.0",
"inquirer": "^9.2.12",
},
"bin": {
"cli-project": "./index.js"
}
}
Some more ideas for CLI Applications:
Password Manager using the fs module
Custom Scripts for initializing a project using shelljs module
Happy coding... ๐โจ
Subscribe to my newsletter
Read articles from Vishnu Prasad Korada directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
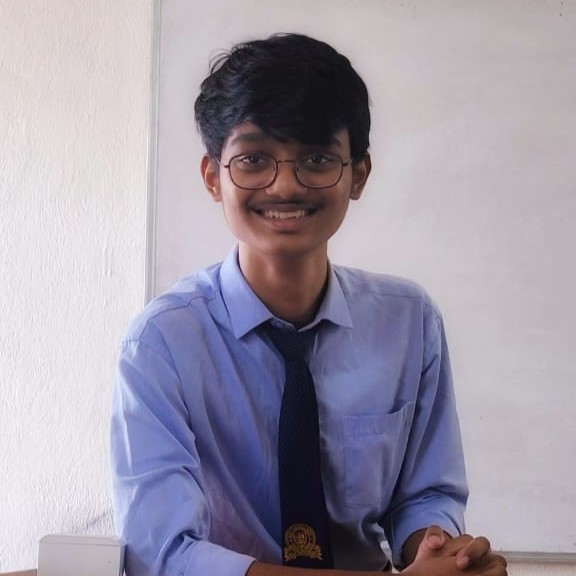