Create Scroll Reveal Effect on Websites with just JavaScript!

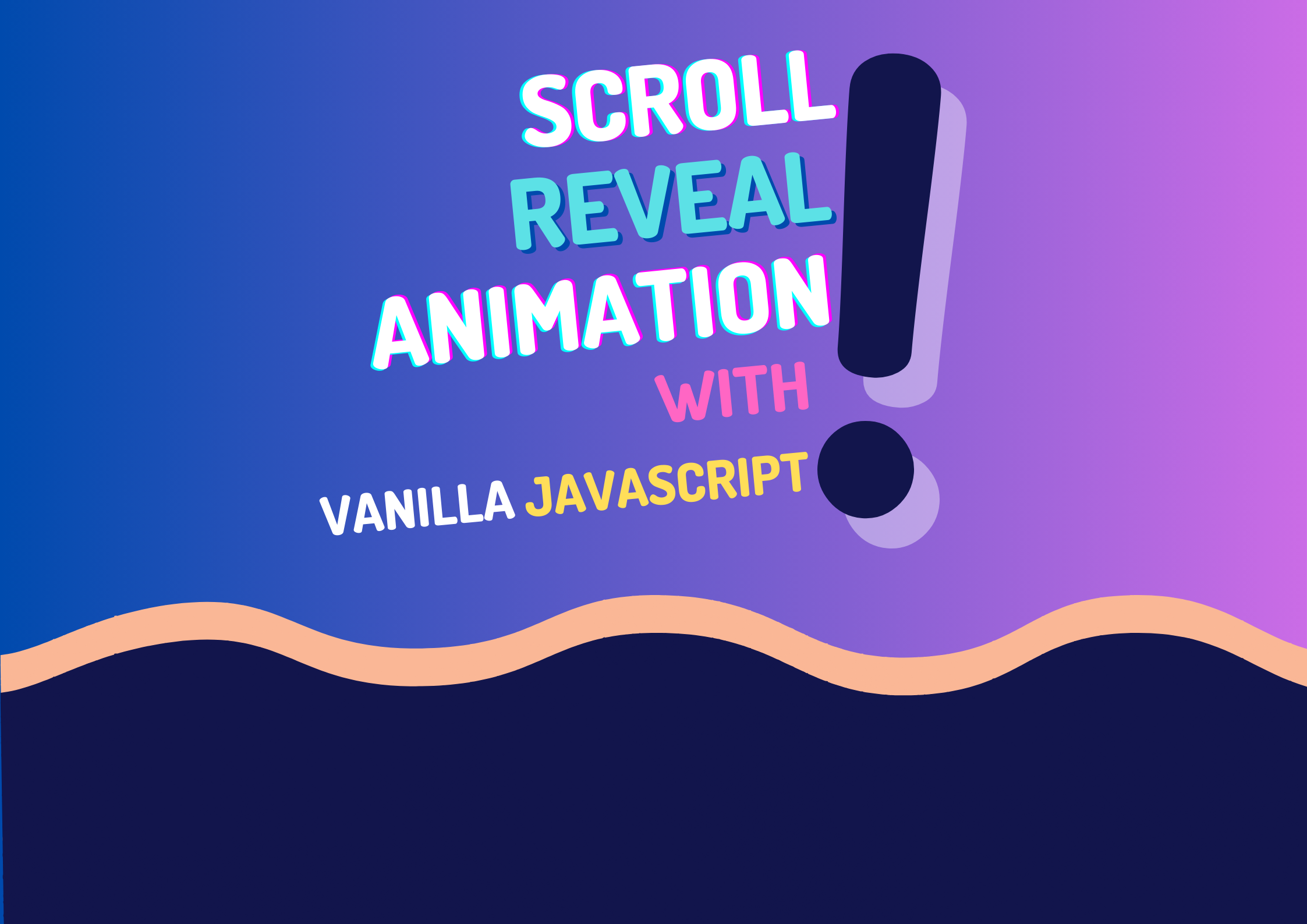
Welcome to my JavaScript tutorial, where I dive into the world of web development and explore the captivating realm of scroll reveal effects using vanilla JavaScript. As a modern website strives to deliver engaging and immersive user experiences, incorporating subtle animations and dynamic elements has become a hallmark of effective design.
In this guide, I'll walk you through the steps of creating scroll reveal animations without relying on external libraries or frameworks. Whether you're a beginner eager to enhance your JavaScript skills or a seasoned developer looking to add an extra layer of interactivity to your projects, join me on this journey as I unravel the magic behind scroll-based animations.
Demo:
Initialize a webpage with basic HTML and few elements.
Here, I have created a webpage which has basic HTML elements containing, services with product cards.
<div class="wrapper">
<div class="heading">Our Services</div>
<div class="products">
<div class="product fade-up">
<div class="product-title">Product 1</div>
<div class="product-desc">
Aliquip officia aliqua voluptate anim duis proident.Est do dolore
ullamco elit culpa Lorem tempor esse id amet pariatur sunt.
</div>
<button class="product-button">Read More</button>
</div>
<!-- code for more product list -->
</div>
</div>
You can see, I have added fade-up class for products. We will use this class to identify elements to be animated.
Let's start animating in JavaScript!
First, we need to get all elements with fade-up class. We will be using querySelectorAll selector to select all elements with fade-up class.
const fadeUpElements = document.querySelectorAll(".fade-up");
Now, we need Scroll event listener to trigger our scrollRevealCheck() function. This function will iterate through all scroll reveal elements that are in fadeUpElements and check if they are visible on screen.
document.addEventListener("scroll", (e) => {
scrollRevealCheck();
checkCounts();
});
//triggering this function once on startup to prevent glitches.
scrollRevealCheck();
To check, whether the element is inside view box or visible on screen, we will use getBoundingClientRect() method to get position relative to the viewport. If the position relative to the viewport is greater than height of the screen, the element is not visible on the screen.
Therefore, Let's create a function isElementOutOfView to check if the element is outside the viewport. isElementOutOfView will return true if an element is outside the viewport.
function isElementOutOfView(element) {
return (
element.getBoundingClientRect().top >
(window.innerHeight - 100 || document.documentElement.clientHeight - 100)
);
}
Note: 100 is a threshold for scroll reveal. Which means, the function will return false (element is visible) only when at least 100px of the element is visible on the screen.
Let's define scrollRevealCheck() function.
function scrollRevealCheck() {
//fade up
fadeUpElements.forEach((element) => {
if (!isElementOutOfView(element)) {
displayFadeUpElement(element);
} else {
hideFadeUpElement(element);
}
});
}
displayFadeUpElement() is triggered when the element is visible on the screen. In this function, we will add fade-up-scrolled class on the element. Likewise, hideFadeUpElement will remove the fade-up-scrolled class.
function displayFadeUpElement(element) {
element.classList.add("fade-up-scrolled");
}
function hideFadeUpElement(element) {
element.classList.remove("fade-up-scrolled");
}
Now, we just need to define CSS classes.
fade-up class translates the element 100px (y-axis) to the bottom and fade-up-scrolled class translates the element back to 0px (y-axis). Scale and Opacity are set to give that extra smooth animation effect.
.fade-up {
transform: translateY(100px);
opacity: 0;
scale: 0.9;
transition: 0.5s ease-out;
}
.fade-up-scrolled {
transform: translateY(0px);
opacity: 1;
scale: 1;
}
That's it! We have successfully made a Scroll Reveal Effect using Vanilla JavaScript.
Full Source code:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
<link rel="stylesheet" href="./css/main.css" />
</head>
<body>
<div class="wrapper">
<div class="space">Scroll Down!!</div>
<div class="heading">Our Services</div>
<div class="products">
<div class="product fade-up">
<div class="product-title">Product 1</div>
<div class="product-desc">
Aliquip officia aliqua voluptate anim duis proident.Est do dolore
ullamco elit culpa Lorem tempor esse id amet pariatur sunt.
</div>
<button class="product-button">Read More</button>
</div>
<div class="product fade-up">
<div class="product-title">Product 2</div>
<div class="product-desc">
Aliquip officia aliqua voluptate anim duis proident.Est do dolore
ullamco elit culpa Lorem tempor esse id amet pariatur sunt.
</div>
<button class="product-button">Read More</button>
</div>
<div class="product fade-up">
<div class="product-title">Product 3</div>
<div class="product-desc">
Aliquip officia aliqua voluptate anim duis proident.Est do dolore
ullamco elit culpa Lorem tempor esse id amet pariatur sunt.
</div>
<button class="product-button">Read More</button>
</div>
<div class="product fade-up">
<div class="product-title">Product 4</div>
<div class="product-desc">
Aliquip officia aliqua voluptate anim duis proident.Est do dolore
ullamco elit culpa Lorem tempor esse id amet pariatur sunt.
</div>
<button class="product-button">Read More</button>
</div>
<div class="product fade-up">
<div class="product-title">Product 5</div>
<div class="product-desc">
Aliquip officia aliqua voluptate anim duis proident.Est do dolore
ullamco elit culpa Lorem tempor esse id amet pariatur sunt.
</div>
<button class="product-button">Read More</button>
</div>
</div>
</div>
<script src="./js/scroll.js"></script>
</body>
</html>
JavaScript:
const fadeUpElements = document.querySelectorAll(".fade-up");
function isElementOutOfView(element) {
return (
element.getBoundingClientRect().top >
(window.innerHeight - 100 || document.documentElement.clientHeight - 100)
);
}
function displayFadeUpElement(element) {
element.classList.add("fade-up-scrolled");
}
function hideFadeUpElement(element) {
element.classList.remove("fade-up-scrolled");
}
function scrollRevealCheck() {
fadeUpElements.forEach((element) => {
if (!isElementOutOfView(element)) {
displayFadeUpElement(element);
} else {
hideFadeUpElement(element);
}
});
}
document.addEventListener("scroll", (e) => {
scrollRevealCheck();
});
//triggering this function once on startup to prevent glitches.
scrollRevealCheck();
CSS:
body {
width: 100%;
overflow-x: hidden;
font-family: sans-serif;
}
.space {
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
font-size: 30px;
}
.wrapper {
margin: 0 auto;
max-width: 1000px;
}
.heading {
font-size: 50px;
color: grey;
font-weight: bold;
text-align: center;
margin-bottom: 70px;
margin-top: 70px;
}
.products {
display: flex;
justify-content: center;
align-items: center;
flex-wrap: wrap;
gap: 30px;
}
.product {
width: 200px;
height: 200px;
padding: 15px;
background-color: white;
box-shadow: rgb(217, 217, 217) 0px 0px 20px;
border-radius: 15px;
display: flex;
flex-direction: column;
}
.product-title {
font-size: 20px;
font-weight: bold;
flex: 1;
color: rgb(57, 57, 57);
}
.product-desc {
font-size: 15px;
flex: 3;
}
.product-button {
height: 30px;
border: none;
outline: none;
border-radius: 10px;
background-color: black;
color: white;
}
/* /////////////////// FadeUP Animation //////////////////////// */
.fade-up {
transform: translateY(50px);
opacity: 0;
scale: 0.9;
transition: 0.5s ease-out;
}
.fade-up-scrolled {
transform: translateY(0px);
opacity: 1;
scale: 1;
}
Thank you for joining me on this exploration of scroll reveal effects using vanilla JavaScript. I hope this tutorial has equipped you with the knowledge and skills to add engaging animations to your web projects. Remember, the beauty of coding lies in the endless possibilities for creativity and innovation. As you embark on your coding journey, feel free to experiment, tweak, and personalize these techniques to suit your unique vision. If you found this guide helpful, I'm genuinely grateful for your time and engagement. Happy coding, and may your future projects be filled with captivating scroll-based animations. Thank you again, and until next time!
Subscribe to my newsletter
Read articles from SUBU directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
