Handling Alerts & Popups in Seleniumπ¨
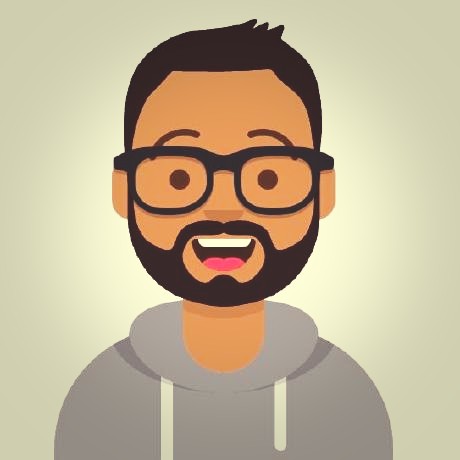
Alerts, also known as pop-ups or dialog boxes, are common elements encountered during web testing. Selenium WebDriver provides a robust set of commands to handle these alerts effectively. In this guide, we'll explore the ins and outs of handling Java Alerts using Selenium WebDriver.
Understanding Alerts π€
Java Alerts are used to convey important information, and warnings, or to prompt user interaction. They can appear as simple informational messages, confirmations, or input prompts.
Types of Alerts:
Alerts: Simple notifications that require acknowledgement.
Confirmations: Alerts with options to confirm or cancel.
Prompts: Alerts that prompt the user to enter input.
Selenium Commands for Alerts using Java πΉοΈ
Alert Interface:
Selenium provides the Alert
interface to interact with Java Alerts. Here are some essential methods:
Switch to Alert:
Alert alert = driver.switchTo().alert();
Accept Alert (OK/Yes):
alert.accept();
Dismiss Alert (Cancel/No):
alert.dismiss();
Get Alert Text:
String alertText = alert.getText();
Enter Text in Prompt:
alert.sendKeys("YourInput");
Handling Different Alert Types π
1. Simple Alerts:
Simple alerts require only acknowledgement. Use accept()
to close them.
Alert simpleAlert = driver.switchTo().alert();
simpleAlert.accept();
2. Confirm Alerts:
Confirm alerts have options to confirm or cancel. Use accept()
for confirmation and dismiss()
for cancellation.
Alert confirmAlert = driver.switchTo().alert();
confirmAlert.accept(); // Confirm
// OR
confirmAlert.dismiss(); // Cancel
3. Prompt Alerts:
Prompt alerts prompt the user to enter input. Use sendKeys()
to enter text and accept()
to confirm.
Alert promptAlert = driver.switchTo().alert();
promptAlert.sendKeys("YourInput");
promptAlert.accept();
Example: Handling a Login Alert π
Consider a scenario where a website prompts an alert upon incorrect login credentials. You can handle it like this:
// Locate login elements and trigger login attempt
driver.findElement(By.id("username")).sendKeys("yourUsername");
driver.findElement(By.id("password")).sendKeys("wrongPassword");
driver.findElement(By.id("loginBtn")).click();
// Handle alert
Alert loginAlert = driver.switchTo().alert();
String alertText = loginAlert.getText();
System.out.println("Alert Text: " + alertText);
loginAlert.accept();
Conclusion π
Mastering Alerts is essential for robust Selenium test automation. With the Alert
interface, you can seamlessly handle alerts of different types, ensuring your automated tests handle pop-ups gracefully. Happy testing! ππ¦
Subscribe to my newsletter
Read articles from Rinaldo Badigar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
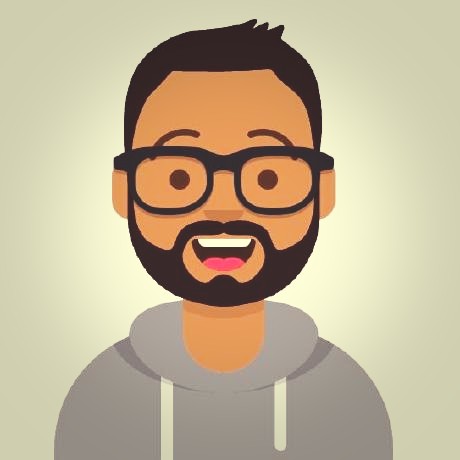
Rinaldo Badigar
Rinaldo Badigar
Software Engineer with 3+ years of experience in Automation, with a strong foundation in Manual, API, and Performance testing, ensuring high-quality software delivery for Ecommerce and Banking applications.