How To Fetch And Display Data From An Api In React Using Axios
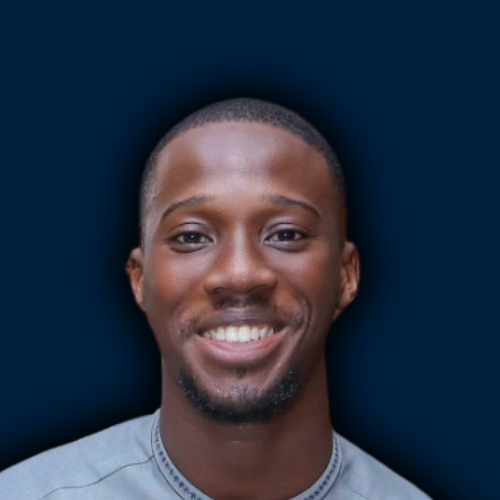
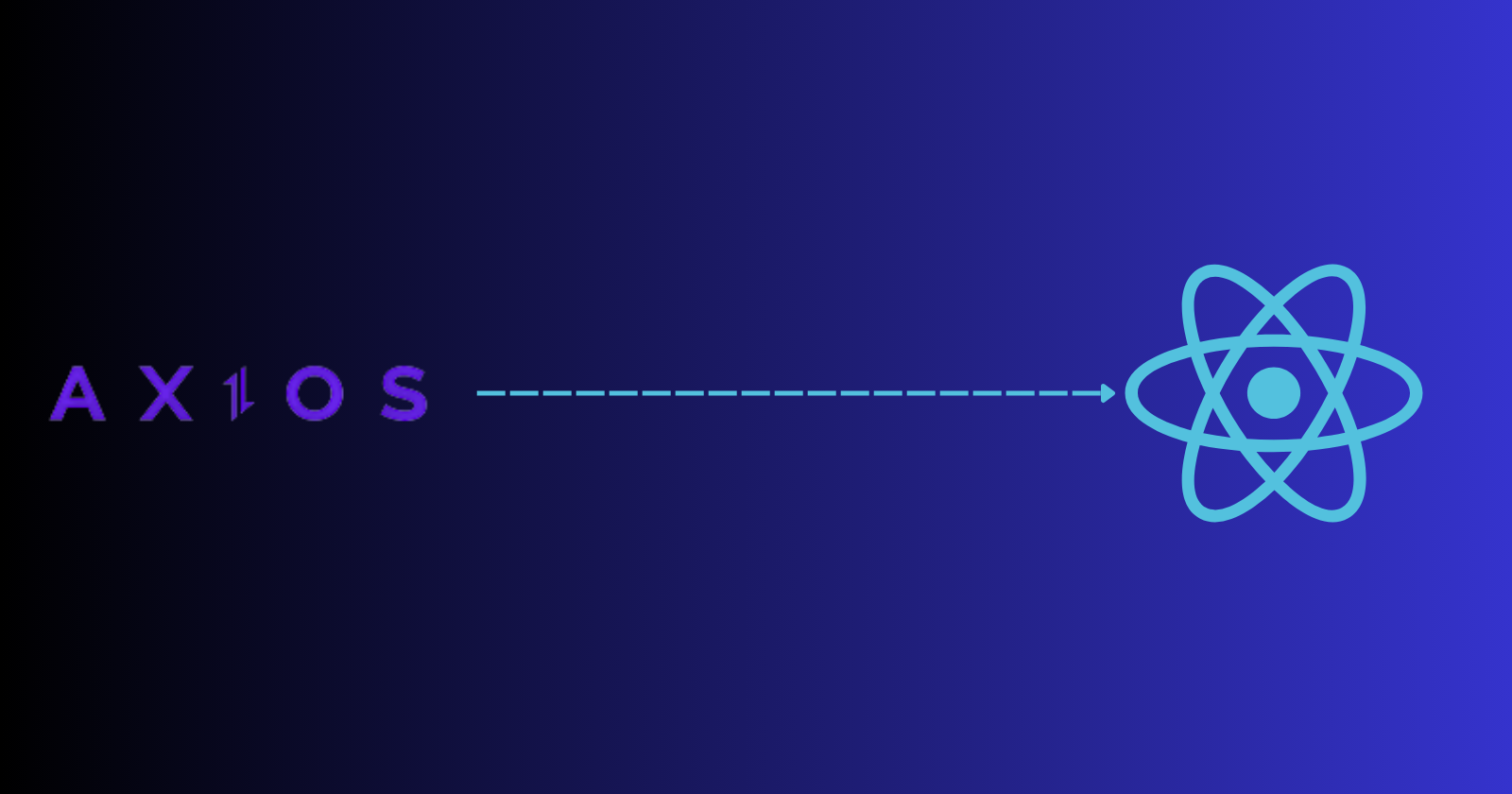
Data fetching is an important and common aspect of web development. In this modern day, more and more websites require data from a server to update their screens and user interfaces. Axios simplifies this process and is a very popular library for data fetching. In this tutorial, I’ll be showing you how to fetch and display data from an API in React, with the use of Axios.
REQUIREMENTS
Basic knowledge of React and its components.
Node js and a Package manager. In this tutorial, I’ll be using NPM as the package manager. Here’s a guide to installing both Node js and NPM.
API endpoint. I’ll be using a mock API endpoint from https://jsonplaceholder.typicode.com/ but you can use a live one.
STEPS
Setup a React app. There are various methods to do this but I’ll be setting it up with Vite. To set up a React app with vite, run the following command on your command line, in your preferred directory.
npm create vite@latest dummy-app -- --template react
Once this is done, go ahead and run the following commands
cd dummy-app
npm installOpen the project in an IDE and install Axios. The IDE I’ll be using is Visual Studio Code. Go to the terminal of the IDE and install Axios with the following command.
npm install axios
Create a file named Todo.jsx inside the components folder within the src directory. In this file, you’ll define a React component named Todo.
Make sure to render the Todo Component in your App.jsx or wherever you want to use it.
Import Axios in the Todo.jsx file by writing
import axios from ‘axios’
at the top of it.Make a GET REQUEST to the API using axios within a useEffect hook. See code snippet below for implementation details.
You should now see the data being fetched successfully in the console tab of the browser developer tools.
Display the fetched data on the screen. To do this, we will use the built-in map and arrow functions in Javascript. See the code snippet below for implementation details.
If you run the application now and check the browser, you should see the fetched todos displayed on the screen.
CONCLUSION
Using the Axios library to fetch and display data in React is a simple and straightforward process. It’s worth noting that Axios is versatile, supporting various HTTP methods such as POST, PATCH, and DELETE. You can read more about that here. Happy Coding!
Subscribe to my newsletter
Read articles from Adekoya Ireoluwatomiwa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
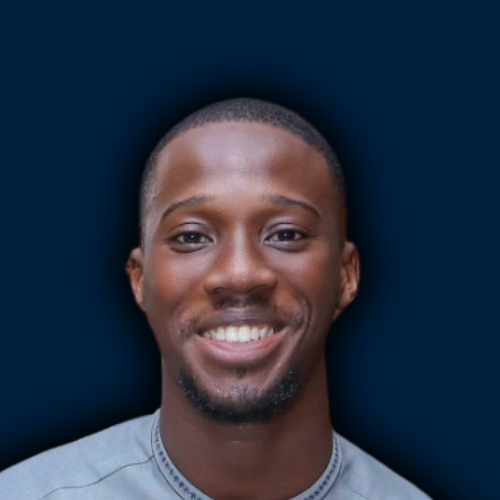