Dart Decision Making

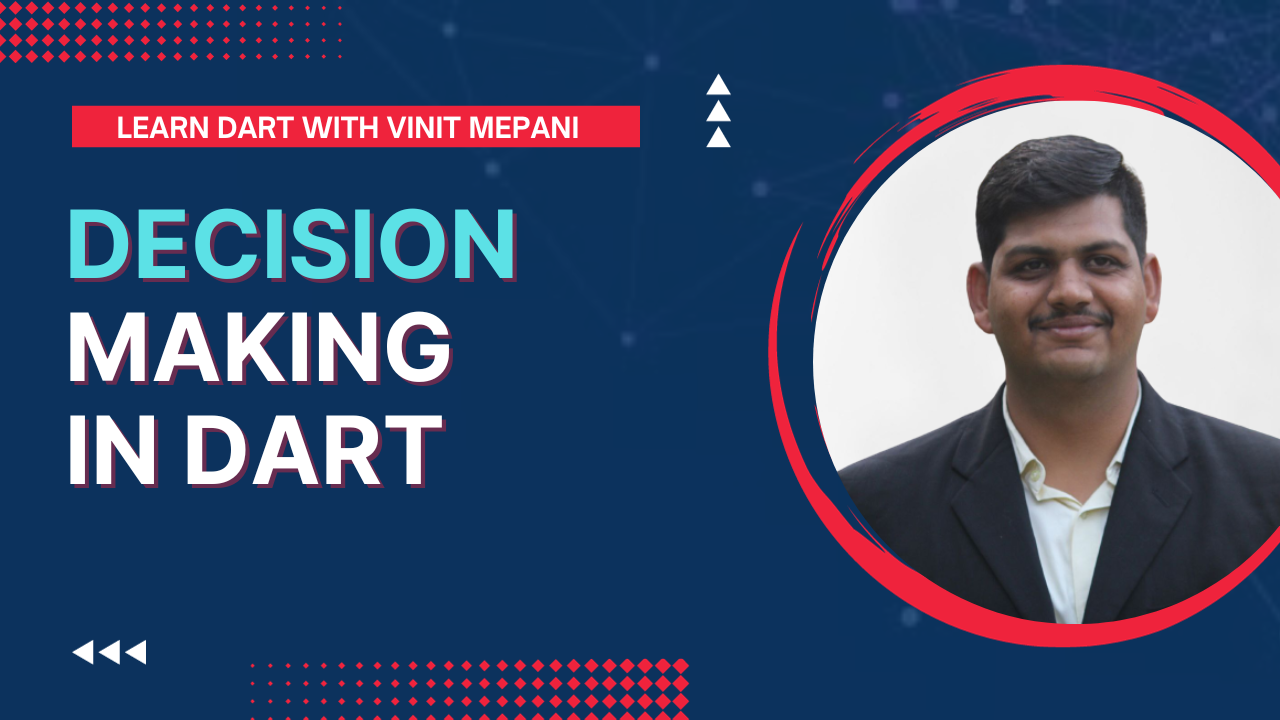
The Decision-making statements allow us to determine that which statement will be execute on based on the condition during run time.
There are many decision making statement such as
If Statement
If-else Statements
If else if Statement
Switch Case Statement
Let's see all the statement in details with example.
If statement
In this decision making we only write the if statement and when it's condition is true than it show output of the condition otherwise we can see blank output or we have to write other statement outside the if block.
syntax of if block:
If (condition) { //statement(s) }
void main () { // define a variable which holds a numeric value var age = 16; // if statement check the given condition if (age>18){ print("You are eligible for voting"); } print("You are not eligible for voting"); }
if .... else statement
In this decision making we write two block one is if and other is else. In this scenario we have two option like if condition is true then print first statement otherwise other statement .
Syntax of if.... else block:
if(condition) {
// statement(s);
} else {
// statement(s);
}
void main() {
var x = 20;
var y = 30;
if(x > y){
print("x is greater than y");
} else {
print("y is greater than x"); //This is the output
}
}
- in this we can see that we put condition of that x is greater than y in if block and other statement is else block, and here y value is greater than x , so as an output else block will executed.
If else if Statement
In this we can run multiple condition at the same time , we can use else if block between if else block and there is no limit to use else if block.
For the execution time complier start debug from the if block ad than else if block and else, if in between compiler find true condition than they provide output and after that execution will stop form there , complier will not check other block after that.
Syntax of if else if block:
if (condition1) { // statement(s) } else if(condition2) { // statement(s) } else if (conditionN) { // statement(s) } . . else { // statement(s) }
void main() { var marks = 74; if(marks > 85) { print("Excellent"); } else if(marks>75) { print("Very Good"); } else if(marks>65) { print("Good"); //Output } else { print("Average"); } }
In this we have declare 74 value as marks so when compiler come to second else if block where condition is true than we will receive output as Good and now compiler will not check the else block due to already condition is true.
Switch case statement
We can use this as alternative option as If else if Statement , because in " If else if Statement " in this compiler check all block from start to end until condition is true which is slow and very time consuming while in switch case compiler one check the case which one met with the condition and providing output faster and run code efficiently.
Syntax of Switch case
switch( expression ) { case value-1:{ //statement(s) Block-1; } break; case value-2:{ //statement(s) Block-2; } break; case value-N:{ //statement(s) Block-N; } break; default: { //statement(s); } }
void main() { int n = 3; switch (n) { case 1: print("Value is 1"); break; case 2: print("Value is 2"); break; case 3: print("Value is 3"); // Output break; case 4: print("Value is 4"); break; default: print("Out of range"); break; } }
- in this we have declare one variable n and store the value of 3 in this variable . In this compiler direct check the case 3 and give output and if any case are not matching than default block will executed .
Subscribe to my newsletter
Read articles from Vinit Mepani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinit Mepani
Vinit Mepani
"Hello World, I'm Vinit Mepani, a coding virtuoso driven by passion, fueled by curiosity, and always poised to conquer challenges. Picture me as a digital explorer, navigating through the vast realms of code, forever in pursuit of innovation. In the enchanting kingdom of algorithms and syntax, I wield my keyboard as a magical wand, casting spells of logic and crafting solutions to digital enigmas. With each line of code, I embark on an odyssey of learning, embracing the ever-evolving landscape of technology. Eager to decode the secrets of the programming universe, I see challenges not as obstacles but as thrilling quests, opportunities to push boundaries and uncover new dimensions in the realm of possibilities. In this symphony of zeros and ones, I am Vinit Mepani, a coder by passion, an adventurer in the digital wilderness, and a seeker of knowledge in the enchanting world of code. Join me on this quest, and let's create digital wonders together!"