Implementing Stack Using an Array in JavaScript

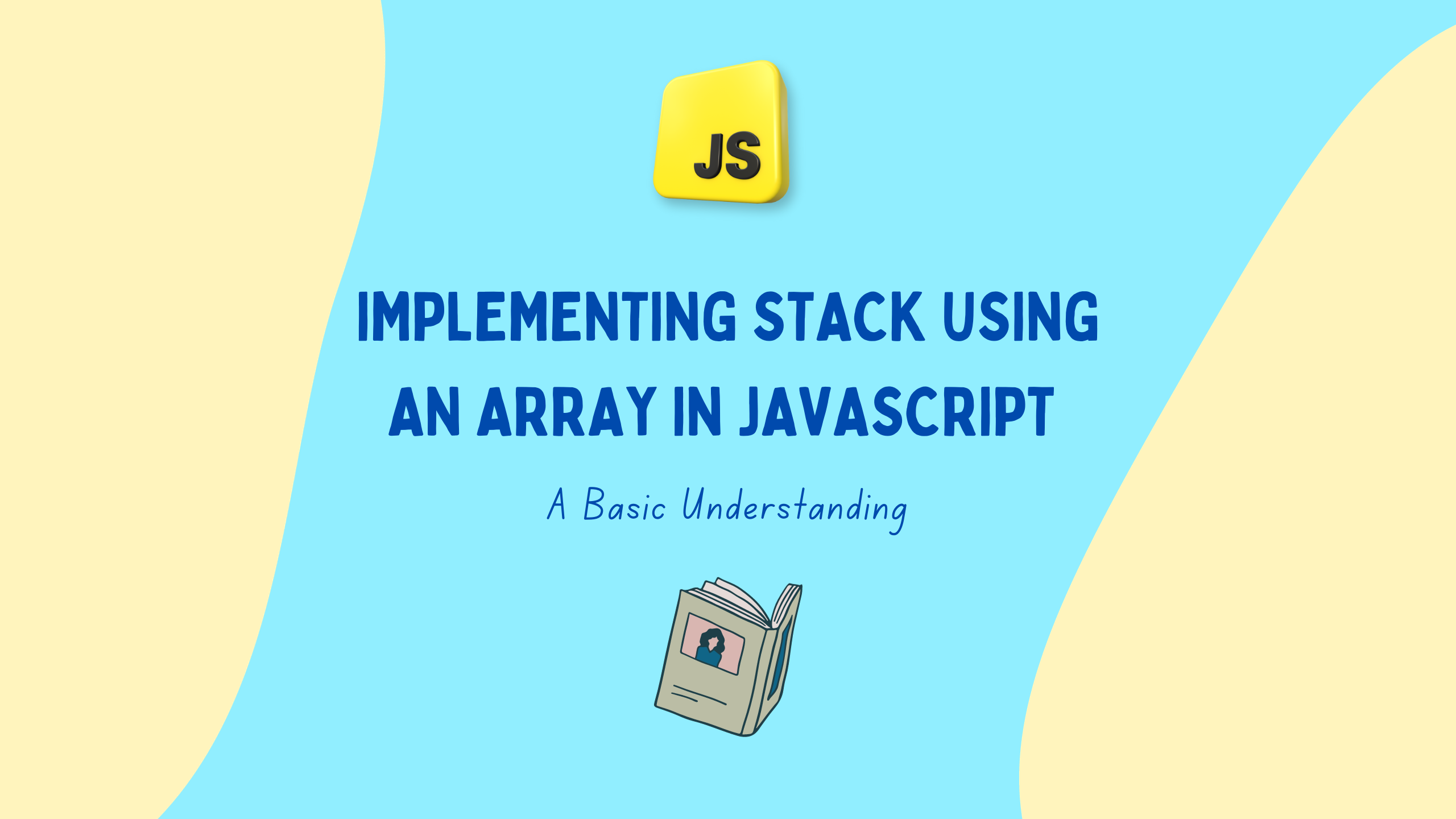
Stacks are a fundamental data structure in computer science, playing a crucial role in various algorithms and applications.
In this blog post, we'll explore the basics of stacks, why they are important, and demonstrate how to create a simple stack using JavaScript arrays.
What is a Stack?
A stack is a data structure that follows the Last In, First Out (LIFO) principle. This means that the last element added to the stack is the first one to be removed. Think of it as a stack of plates โ you add a plate to the top, and when you need to remove one, you take it from the top.
Importance of Stacks
Understanding stacks is essential for mastering algorithms and solving various problems in computer science. Stacks are used in a variety of scenarios, including:
Function Call Stack: In programming, function calls are managed using a stack. When a function is called, it is added to the stack, and when it completes execution, it is removed.
Undo Mechanisms: Many applications use stacks to implement undo mechanisms. Each action is pushed onto the stack, allowing users to undo the last operation.
Expression Evaluation: Stacks are commonly used to evaluate expressions, ensuring the correct order of operations.
Now, let's dive into implementing stacks in JavaScript.
Implementing a Stack Using Array Methods
You can simulate a stack using JavaScript arrays and their built-in methods. Here's a basic example:
// Initialize an empty stack
let stack = [];
// Push elements onto the stack
stack.push(1);
stack.push(2);
stack.push(3);
// Log the stack after pushing elements
console.log("Stack after pushing elements:", stack);
// Pop an element from the stack
let poppedElement = stack.pop();
// Log the popped element and the stack after popping
console.log("Popped element:", poppedElement);
console.log("Stack after popping element:", stack);
In this example, the push
method adds elements to the end of the array, simulating the process of pushing elements onto the stack. The pop
method removes the last element, simulating the process of popping an element off the stack.
Implementing a Stack Using a Stack Class
For a more structured approach, you can create a stack class:
class Stack {
constructor() {
// Initialize an empty array to store stack elements
this.items = [];
}
// Method to push an element onto the stack
push(element) {
this.items.push(element);
}
// Method to pop an element from the stack
pop() {
// Check if the stack is empty before popping
if (this.isEmpty()) {
return "Underflow"; // Indicates an empty stack
}
return this.items.pop(); // Remove and return the last element
}
// Method to get the top element of the stack without removing it
peek() {
return this.items[this.items.length - 1];
}
// Method to check if the stack is empty
isEmpty() {
return this.items.length === 0;
}
// Method to get the size of the stack
size() {
return this.items.length;
}
}
// Example usage of the Stack class
let myStack = new Stack();
myStack.push(10);
myStack.push(20);
myStack.push(30);
// Log various properties of the stack
console.log("Stack size:", myStack.size());
console.log("Top element:", myStack.peek());
console.log("Popped element:", myStack.pop());
console.log("Is the stack empty?", myStack.isEmpty());
In this Stack
class:
The class has a constructor that initializes an empty array (
items
) to store the stack elements.Methods like
push
,pop
,peek
,isEmpty
, andsize
are defined for manipulating and inspecting the stack.push
adds an element to the end of the array.pop
removes and returns the last element from the array (if the stack is not empty).peek
returns the top element of the stack without removing it.isEmpty
checks if the stack is empty.size
returns the number of elements in the stack.
Conclusion
Implementing stacks in JavaScript is straightforward and can greatly enhance your programming skills. Whether you choose to use array methods or create a dedicated class, understanding stacks is essential for mastering algorithms and solving various problems in computer science.
Feel free to experiment with the provided examples and explore more advanced use cases for stacks in your JavaScript projects.
~Happy coding!๐งโ๐ป
Hope you found this article helpful, don't forget to share & give your feedback in the comment section! ๐
Subscribe to my newsletter
Read articles from Pranab K.S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pranab K.S
Pranab K.S
I'm a passionate web developer with a love for coding challenges and a knack for making well-informed decisions. Proficient in a variety of web technologies, including HTML, CSS, JavaScript, jQuery, Node.js, Express.js, MongoDB and React. I'm an avid open source enthusiast dedicated to both learning and contributing to the developer community.