Functions in JavaScript
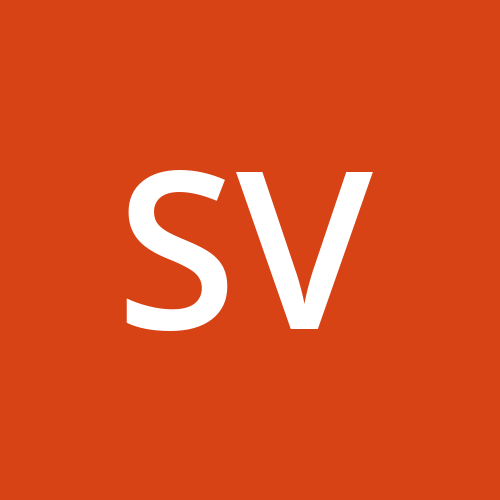
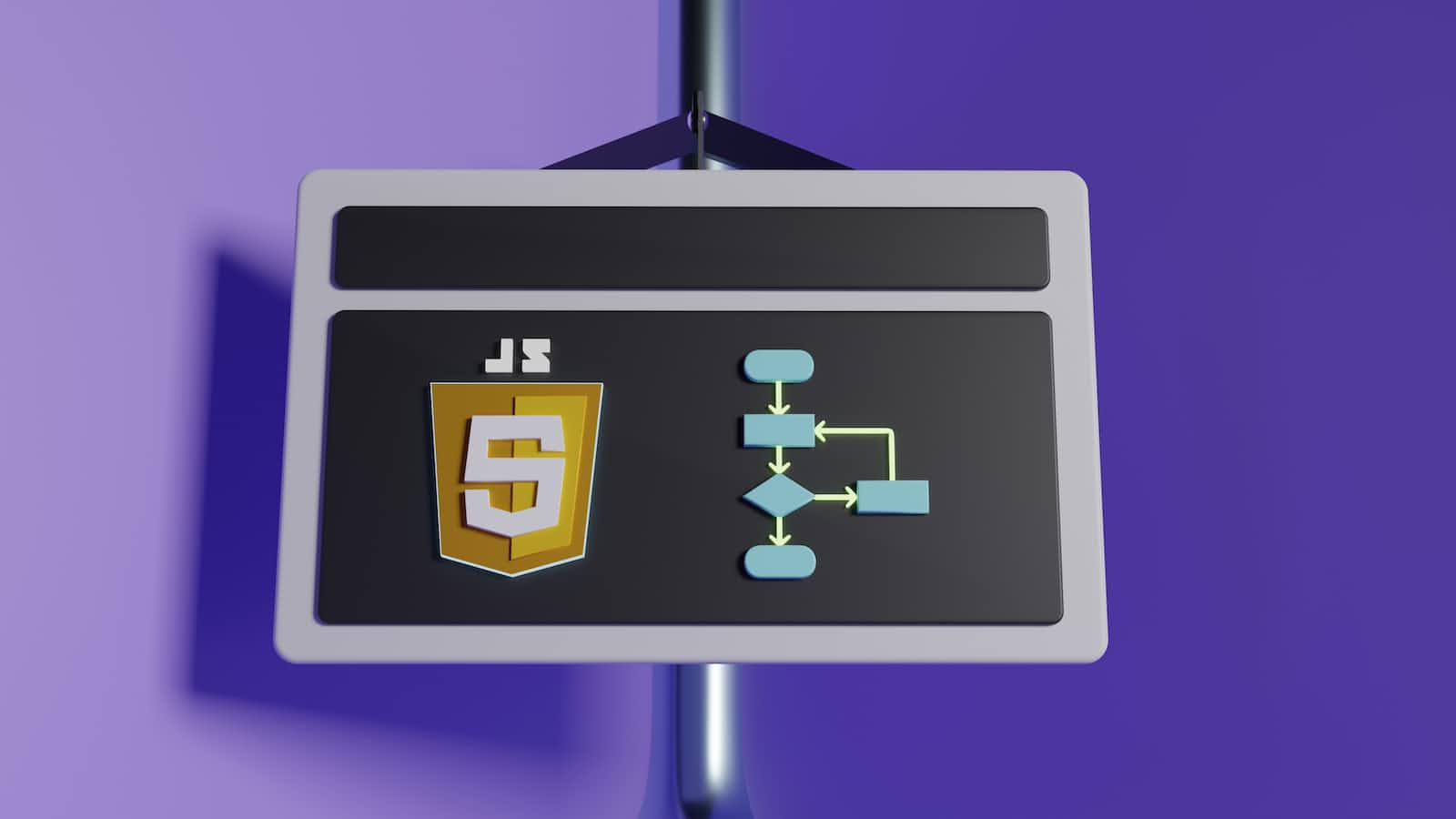
Functions are the bread and butter of JavaScript programming.
- ELOQUENT JAVASCRIPT
The " Functions " is a concept of wrapping a piece of program in a value which has many uses.
It helps us to structure the large chunk of a program in order to overcome repetition.
It helps to associate names with subprograms and to isolate these subprograms from each other.
DEFINING A FUNCTION
A Function definition is a regular binding where the value of the binding is a Function.
Example:-
const square = function(x)
{
return x * x;
};
console.log(square(12));
//144
The above code defines square which is the " function " that squares the given number. A function is created with an expression that starts with the keyword function.
Functions have a set of parameters and a body, which contains the statements that are to be executed when the function is called.
The function body of a function which is created this way must always be wrapped in braces, even when it consists of only a single statement.
A function can have multiple parameters or no parameters at all.
Example:-
-------------------------------------------------(no parameters) const makeNoise = function() { console.log("TingTing!"); }; makeNoise(); // TingTing! --------------------------------------------------(multipe parameters) const power = function(base, exponent) { let result = 1; for (let count = 0; count < exponent; count++) { result *=base; } return result; }; console.log(power(2, 10)); // 1024
In above example " makeNoise " does not list any parameter names.
Some functions produce a value such as power and square and some does not produce any value such as makeNoise.
A return statement determines the value the function which returns. when control comes across such a statement, it immediately jumps out of the current function and gives the returned value to the code which called the function.
A " return " keyword without an expression causes the function to return " undefined " .
Functions that don't have a " return " statement at all, such as " makeNoise ", returns undefined.
Parameters to a function behave like regular bindings, but their initial values are given by the " caller " of the function, not the code in the function itself.
Subscribe to my newsletter
Read articles from Samata Vijaykumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
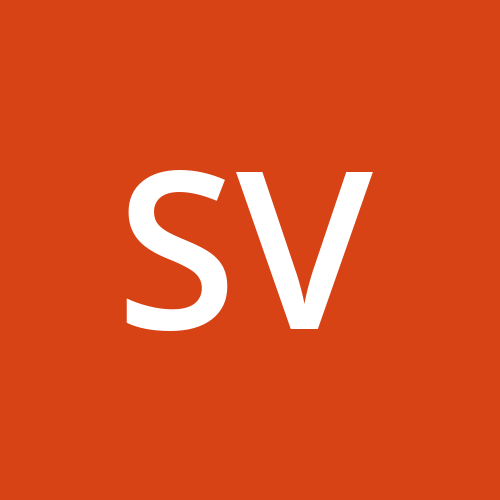
Samata Vijaykumar
Samata Vijaykumar
I am Computer Science Graduate looking forward to upskill my tech-stack through Networking and participating in the community. A self learner who looks for an opportunity to create change for the good.