π Demystifying JavaScript Lexical Scope
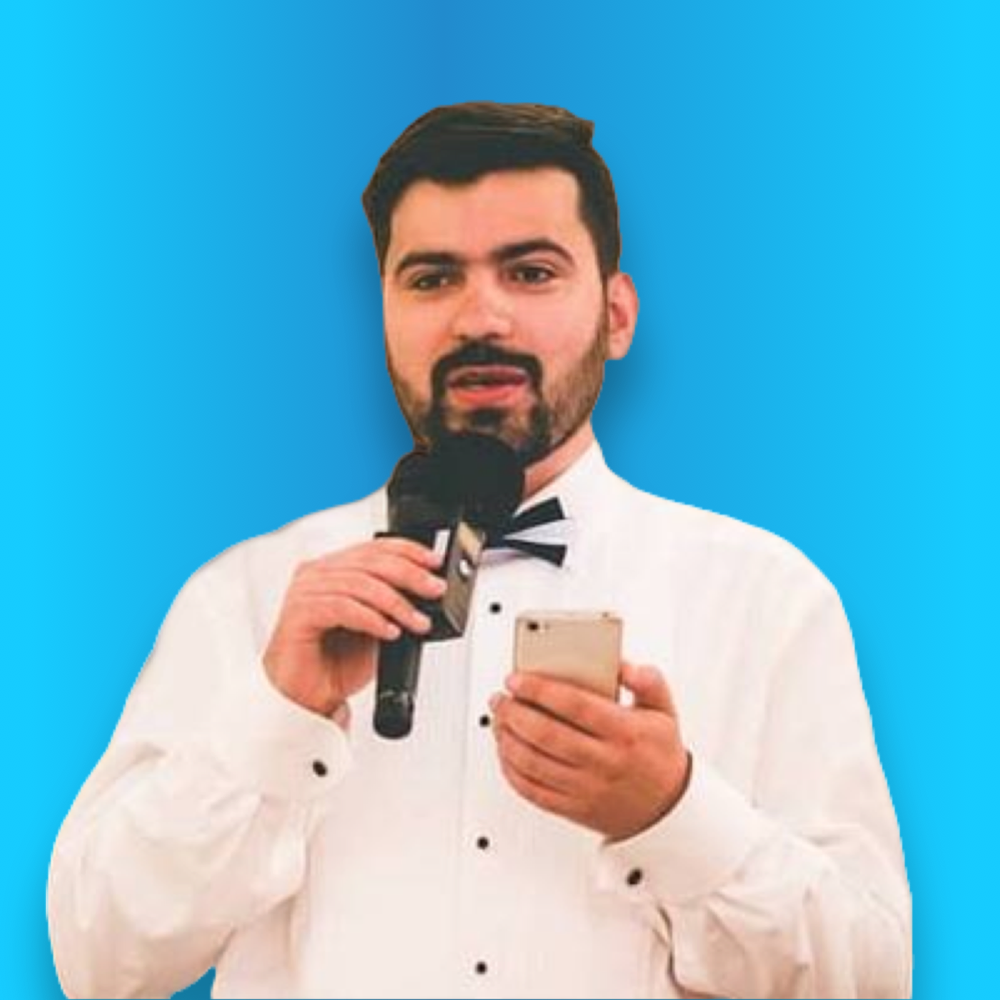
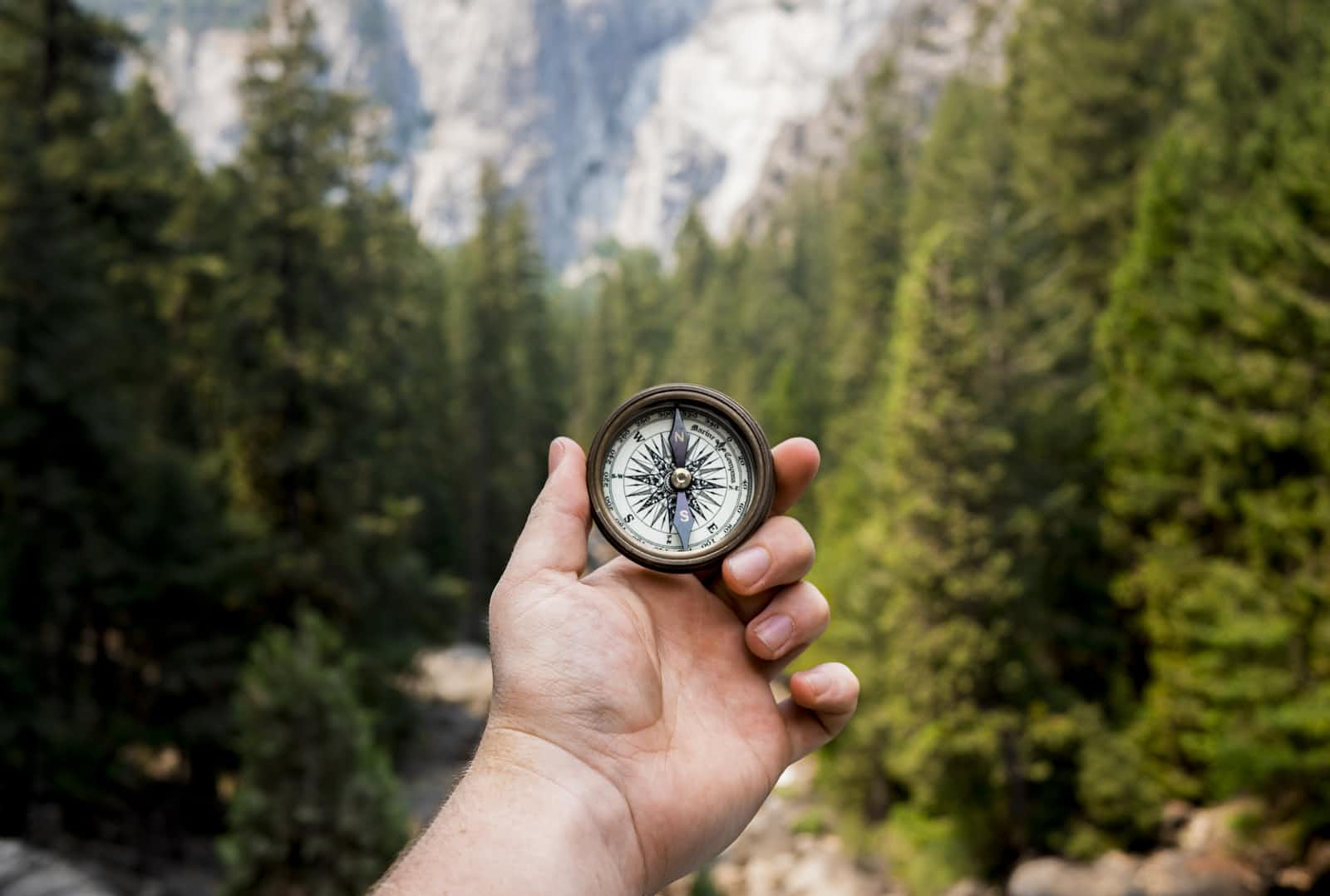
Hey fellow coders! π Let's talk about something cool in the JavaScript world β lexical scope! π€
So, what's the deal with lexical scope, you ask? Well, buckle up because it's a concept that can make your coding life a whole lot easier.
What is the lexical scope? π€
In plain English, the lexical scope is like the GPS of your variables in JavaScript. It figures out where a variable belongs based on where it's hanging out in your code. It's all about location, location, location π!
Imagine this: you've got a bunch of variables and functions doing their thing in your code. Lexical Scope steps in and says, "Hey, I know where you belong, buddy!". It does this magic during code compilation, not when the code is running. That's right β it's like having a superhero organize your variables before the action begins.
Letβs check out some examples π!
Global Scope π vs. Local Scope π
In the following example, globalVar
is accessible inside and outside the function showScope
because it's declared in the global scope. However, localVar
is only accessible within the showScope
function because it's declared in the local scope.
// Global variable π
let globalVar = "I'm global!";
function showScope() {
// Local variable π
let localVar = "I'm local!";
console.log(globalVar); // Accessing global variable π
console.log(localVar); // Accessing local variable π
}
showScope(); // Output: "I'm global!" and "I'm local!"
console.log(globalVar); // β
Output: "I'm global!"
console.log(localVar); // β ReferenceError: localVar is not defined
Nested Lexical Scope πͺ
In this example, innerFunction
has access to both its own local variable innerVar
and the variable outerVar
declared in the outer function outerFunction
. However, these variables are not accessible outside their respective scopes.
function outerFunction() {
let outerVar = "I'm from outer!";
function innerFunction() {
let innerVar = "I'm from inner!";
console.log(outerVar); // Accessing outer variable
console.log(innerVar); // Accessing inner variable
}
innerFunction();
}
outerFunction(); // Output: "I'm from outer!" and "I'm from inner!"
console.log(outerVar); // β ReferenceError: outerVar is not defined
console.log(innerVar); // βReferenceError: innerVar is not defined
Final thoughts π
Understanding lexical scope is crucial for writing clean and organized code in JavaScript. It's like knowing the neighborhood of your variables and functions, making your code more predictable and easier to debug. Happy coding! ππ
References β»
Subscribe to my newsletter
Read articles from Ricardo Rocha // π¨βπ» directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
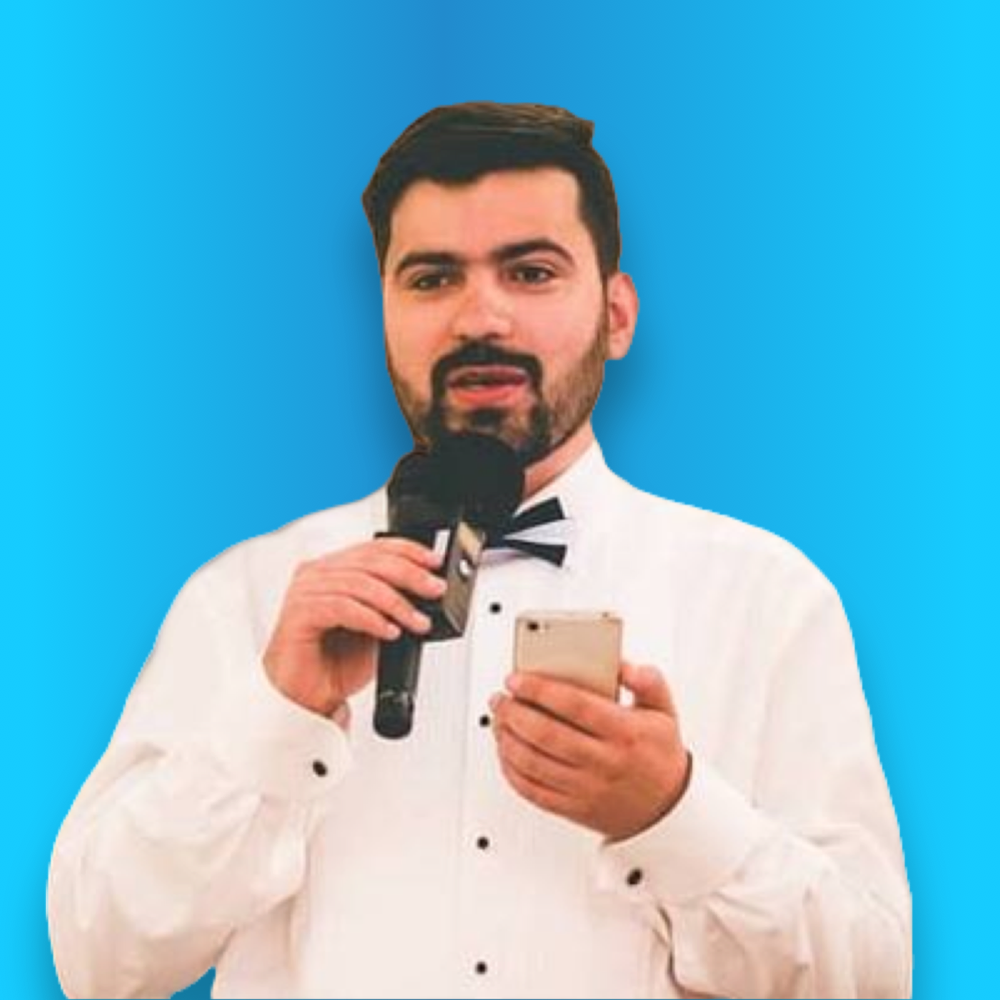
Ricardo Rocha // π¨βπ»
Ricardo Rocha // π¨βπ»
Hey there π! π So, here's the lowdown β I'm a full-stack web dev with a serious crush on front-end development. Armed with a master's in Software Engineering, I've been rocking the programming scene for a solid decade. I've got this knack for software architecture, team and project management, and even dabble in the magical realm of deep learning (yeah, AI, baby!). My coding toolbox π§° is stacked β JavaScript, TypeScript, React, Angular, C#, SQL, NoSQL - you name it. Nevertheless, learning is my best tool π! But here's the thing β I'm not just about the code. My soft skills game is strong β think big-picture pondering, critical thinking, and communication skills sharper than a ninja's blade. Leading, mentoring, and rocking successful projects? Yeah, that's my jam as well. Now, outside the coding dojo, I'm a music lover. Saxophone and piano are my instruments of choice, teaching me the art of teamwork and staying cool under pressure. I've got a soft spot for giving back too π₯°. I've lent a hand to the Jacksonville Human Society (dog shelter). And speaking of sharing wisdom, I also write blogs and buzz around on Twitter, LinkedIn, Stackoverflow and my own Blog. Go ahead and check me out: Linkedin (https://www.linkedin.com/in/ricardogomesrocha/) Stackoverflow (https://stackoverflow.com/users/5148197/ricardo-rocha) Twitter (https://twitter.com/RochaDaRicardo) Github (https://github.com/RicardoGomesRocha) Blog (https://ricardo-tech-lover.hashnode.dev/) Let's connect and dive into the exciting world of web development! Cheers π₯, Ricardo π