"The Silent Dynamo: Exploring the Impact of 'Static' in Java's Code Symphony"
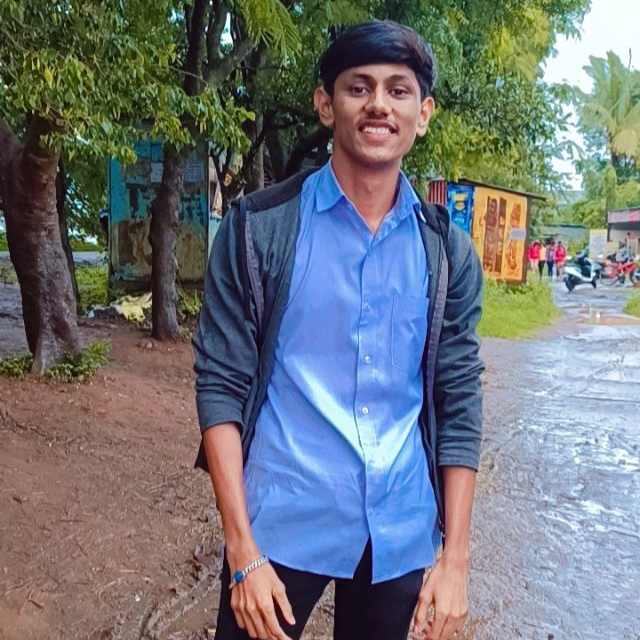
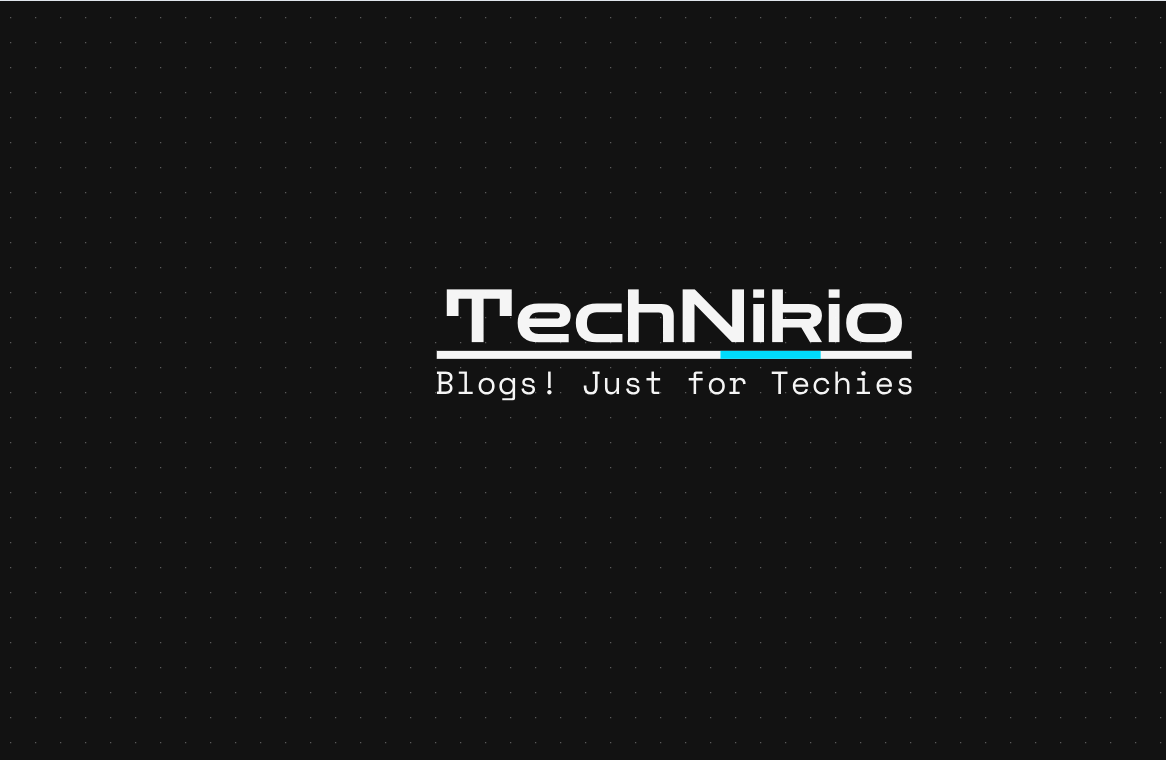
1. Introduction:
Greetings Java enthusiasts! Today, we're venturing into the realm of the "static" keyword—a versatile tool that adds a touch of magic to your code. Buckle up as we unravel the mysteries and potential of this keyword in the Java programming language.
#CodeMagicLaughs🦸♂️
2. Understanding the Static Realm
Static is the keyword that always gives you the latest value.
Static keywords create their self-memory only once.
Static keywords reserve single-copy storage
The Static keyword is used in :
Variables
Methods
Blocks
Inner Class
Static Variables: The Shared Memory Lane
In Java, a static variable is shared among all instances of a class. It's like a memory lane where all instances stroll together, sharing a common piece of information. This not only conserves memory but also facilitates consistent data across objects.
Important points for static variables:
We can create static variables at the class level only. See here
static block and static variables are executed in the order they are present in a program.
public class Main { // static variable static int myStaticVariable = 42; public static void main(String[] args) { // Accessing the static variable directly without creating an object System.out.println("Static Variable Value: " + myStaticVariable); } }
Static Methods: Beyond Object Boundaries
Static methods, unlike instance methods, don't require an object to be instantiated. They belong to the class, not to instances. This makes them accessible directly through the class, allowing for utility methods and operations that transcend the boundaries of objects.
They can only directly call other static methods.
They can only directly access static data.
class StaticMethodExample { static void greet(String name) { System.out.println("Hello, " + name + "!"); } public static void main(String[] args) { StaticMethodExample.greet("Java Guru"); // Outputs: Hello, Java Guru! } }
Static Blocks: The Class Warm-Up Act
Static blocks in Java are like warm-up acts before the main performance. They are executed when the class is loaded into memory
Static Blocks are executes only once when they are loaded into the memory.
A class can have any number of static initialization blocks, and they can appear anywhere in the class body. The runtime system guarantees that static initialization blocks are called in the order that they appear in the source code.
Static Inner Classes in Java:
Definition:
- A static inner class is a nested class that is declared with the
static
keyword. It is associated with its outer class but doesn't require an instance of the outer class for instantiation.
Access Modifiers:
- A static inner class can have its access modifiers (
public
,private
,protected
, default), independent of the outer class.
- A static inner class can have its access modifiers (
Accessing Outer Class Members:
- A static inner class can access static members of its outer class directly. However, it cannot access non-static (instance) members without creating an instance of the outer class.
Static Members:
- A static inner class can contain both static and non-static members. It can have static methods, variables, and instance methods.
Instantiation:
- Unlike non-static inner classes, a static inner class can be instantiated without creating an instance of the outer class. It is associated with the class itself rather than a specific instance.
class OuterClass {
// Outer class member
static int outerStaticVariable = 42;
// Static inner class
static class StaticInnerClass {
// Inner class member
static int innerStaticVariable = 10;
// Inner class method
static void printInnerMessage() {
System.out.println("Hello from Static Inner Class!");
}
}
}
public class Main {
public static void main(String[] args) {
// Accessing static inner class members without creating an instance
System.out.println("Outer Static Variable: " + OuterClass.outerStaticVariable);
System.out.println("Inner Static Variable: " + OuterClass.StaticInnerClass.innerStaticVariable);
// Calling static inner class method
OuterClass.StaticInnerClass.printInnerMessage();
}
}
3. Calling of static block in Java?
Now comes the point of how to call this static block. So to call any static block, there is no specified way as a static block executes automatically when the class is loaded in memory. Refer to the below illustration for an understanding how what the static block is called.
Illustration:
class Technikio {
Technikio() {
// Constructor
}
// Method of this class
public static void print() { }
static{
// static block content
// execute only once, when class is loaded into the memory
}
public static void main(String[] args) {
// Calling of method inside main()
Technikio geeks = new Technikio();
// Calling of constructor inside main()
new Technikio();
}
}
Example:
class Technikio {
// Static block
static
{
// Print statement
System.out.print("Static block can be printed without main method");
}
}
Output:
Static block can be printed without main method
4. Unleashing the Potential
Singleton Design Pattern: One and Only One
The static keyword plays a crucial role in implementing the Singleton design pattern, ensuring that a class has only one instance globally.
class Singleton {
private static Singleton instance;
private Singleton() {
// Private constructor to prevent instantiatio
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Math Utility Classes: No Objects Required
Java's Math
class is a prime example of the static keyword in action. With static methods for common mathematical operations, you can use them directly without creating a Math object.
javaCopy codedouble result = Math.sqrt(25); // No Math object needed
5. Caveats and Considerations
While the static keyword opens doors to efficiency and global accessibility, it comes with responsibilities:
Thread Safety: Shared static variables can lead to thread safety concerns.
Testing Challenges: Static methods can pose challenges in unit testing due to their global nature.
Conclusion
The static keyword in Java isn't just a keyword; it's a powerful enabler of efficient code, shared resources, and unique design patterns. Embrace its potential wisely, and it will add a dynamic layer to your Java programming journey.
So, fellow coders, venture forth and wield the "static" keyword with purpose! 🚀✨
🔐 Exciting News! The next stop in our Java journey is all about the "final" keyword. Brace for impact as we delve into locking down variables and adding that final touch to our code. Stay tuned for code excellence! 🚀✨ #JavaFinalKeyword #StayTuned 💻🔒
#CodeMagicLaughs🦸♂️
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Nikhil Abhiman Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
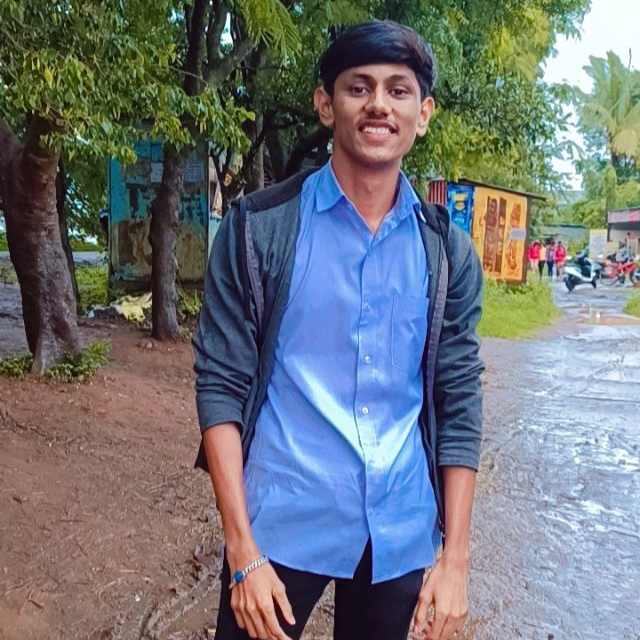
Nikhil Abhiman Jadhav
Nikhil Abhiman Jadhav
Hey there 👋🏻, I'm Nikhil Jadhav, a passionate tech enthusiast and aspiring writer on a mission to demystify the complexities of coding and technology. By day, I'm immersed in lines of code, and by night, I'm weaving words to make tech more accessible and enjoyable for everyone. 📬 Get in touch Twitter: https://twitter.com/Technikio LinkedIn:linkedin.com/in/nikhil-7571nik GitHub:github.com/jadhavnikhil2624