Advanced Docker
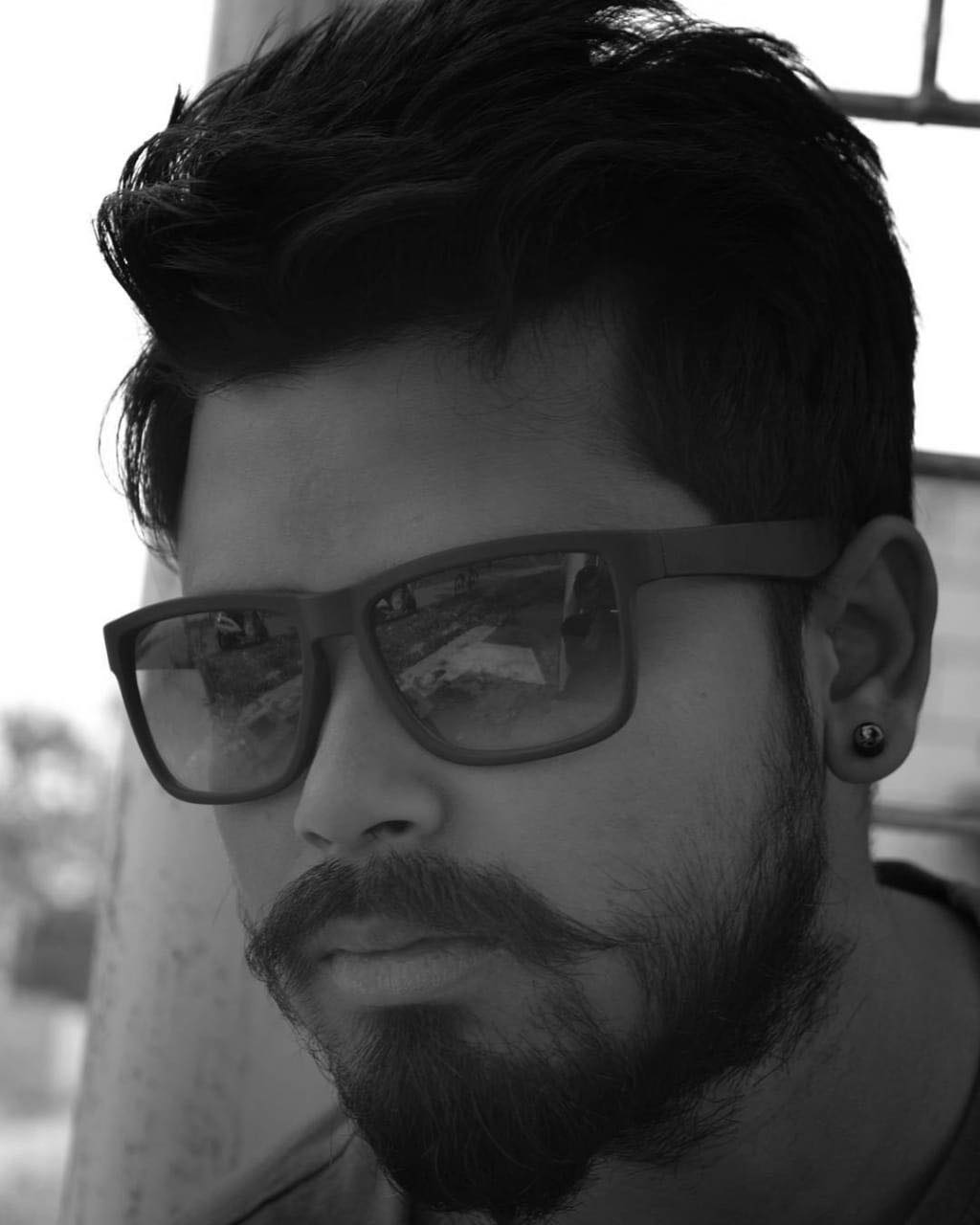
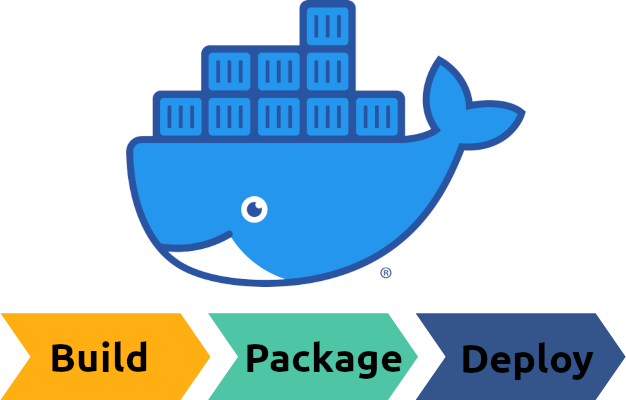
Today in this blog we are going to discuss about some advanced docker concepts such as:-
Docker volume
Docker networking
Docker compose
Multistage docker
Docker Volume
Let's break down Docker volumes in a simple and understandable way:
Imagine a Container as a Box:
- Container: Think of it as a box that holds everything your application needs to run - the code, libraries, and dependencies.
Now, Think About Data:
- Inside the Container: The box is great, but what if your application needs to store data? Like user uploads, database files, or logs?
Here Comes the Challenge:
- Container's Lifespan: Containers are designed to be ephemeral. If they die or get replaced, the data inside them might be lost.
Enter Docker Volumes - A Special Compartment:
- Docker Volume: It's like an extra compartment you attach to your container. This compartment exists outside the container, so even if the container goes away, the data in this compartment stays safe.
Let's Draw a Parallel:
- Think of a USB Drive: Your container is like a computer, and the Docker volume is like a USB drive. You plug in the USB drive when you need to store something important. Even if your computer crashes, the data on the USB drive is still safe.
Practical Examples:
Database Storage: If your container runs a database, you might use a Docker volume to store the database files. This way, even if you replace or update the container, your data remains intact.
File Uploads: If your application allows users to upload files, you can use a Docker volume to store these files. This ensures that even if you deploy a new version of your app, the user uploads are preserved.
Summing Up:
- Docker Volume = External Storage: It's an external storage space attached to your container, making sure that important data survives the life cycle of your containers.
In a nutshell, Docker volumes provide a way for your containers to have a persistent and separate space for data, keeping it safe and accessible even as containers come and go.
Docker Networking
Let's break down Docker networking in simple terms:
Imagine Containers as Houses:
- Containers: Picture them as houses where your applications live. Each house (container) has its own things to do.
Containers Need to Talk:
- Communication: Sometimes, these houses need to talk to each other. For example, one house might have a piece of information that another house needs.
Docker Networking - Building Roads:
- Docker Networking: It's like building roads or pathways between these houses so they can communicate. Without roads, the houses are isolated.
Different Types of Roads:
Bridge Networks: Imagine big bridges connecting many houses. Containers in a bridge network can easily talk to each other.
Host Networks: Think of this like all houses on the same street. They share the same network space, making communication quick.
Overlay Networks: Picture tunnels connecting houses in different neighborhoods. Even if they're far apart, containers can communicate seamlessly.
IP Addresses - House Numbers:
- IP Addresses: Each house (container) has a unique address, just like a house number. This helps them find and talk to each other.
Ports - Open Windows:
- Ports: Imagine windows in the houses. Containers talk through these open windows, known as ports. Each window (port) serves a different purpose.
Example: Web Server and Database:
Scenario: One house (container) is a web server, and another is a database. They need to talk to each other.
Docker Networking: It's like creating a road between them. The web server can find the database easily, like sending a letter to a neighboring house.
IP Addresses: Each house has a unique address (IP), so they know where to send and receive messages.
Ports: The web server may have a window (port) for receiving web requests, and the database may have a window (port) for data exchange. They communicate through these open windows.
Summing Up:
- Docker Networking = Communication Roads: It's like building roads or tunnels between containers (houses), ensuring they can easily talk and share information.
In essence, Docker networking is all about creating pathways for containers to communicate, allowing them to work together smoothly, just like houses in a neighborhood connected by roads.
Docker compose and dockerfile
Let's simplify Docker Compose and Dockerfile:
Docker Compose - Planning a Party:
Scenario: Imagine you're planning a party. You need a venue, music, food, and decorations.
Docker Compose: It's like your party planning list. You write down what you need and how everything fits together.
Services: Each thing you need (venue, music, food) is a "service" in Docker Compose. You describe them in a simple file.
Easy Setup: With Docker Compose, you just follow your list, and everything is set up without much hassle.
Dockerfile - Building a Cake:
Scenario: Now, think of making a cake. You need ingredients and steps to follow.
Dockerfile: It's your cake recipe. You list the ingredients (software, configurations) and steps (instructions) to build your application.
Layers: Dockerfile is like building a cake in layers. Each step adds a new layer, making the final cake (your application).
Reproducibility: Anyone with your recipe (Dockerfile) can make the same cake (run your application). It ensures consistency.
Putting It Together:
Party Planning Analogy: Docker Compose is like your party planning checklist, defining what you need (services) for a smooth event.
Cake Baking Analogy: Dockerfile is like your cake recipe, providing the instructions and ingredients to build your application.
Real-world Example:
Web App Party:
Docker Compose: You list services like the web app, database, and maybe a cache system.
Dockerfile: You write a recipe for the web app, specifying the programming language, dependencies, and how to start the app.
Summing Up:
Docker Compose = Party Planner: It's your plan for multiple services to work together smoothly, like planning a party with various elements.
Dockerfile = Cake Recipe: It's your step-by-step guide to build your application, making it easy for anyone to replicate the same setup.
In essence, Docker Compose helps you orchestrate different services, and Dockerfile provides a recipe to build your application, making development and deployment more organized and reproducible.
Let's create a simple example of a Dockerfile for a basic web application using Node.js. This example assumes you have a Node.js web application with a file named app.js
and a package file named package.json
.
# Use an official Node.js runtime as a base image
FROM node:14
# Set the working directory inside the container
WORKDIR /usr/src/app
# Copy package.json and package-lock.json to the working directory
COPY package*.json ./
# Install application dependencies
RUN npm install
# Copy the rest of the application code to the working directory
COPY . .
# Expose a port that the application will run on
EXPOSE 3000
# Define the command to run the application
CMD ["node", "app.js"]
Explanation:
FROM node:14
: This line sets the base image for the Dockerfile. Here, we're using an official Node.js image with version 14.WORKDIR /usr/src/app
: Sets the working directory inside the container.COPY package*.json ./
: Copiespackage.json
andpackage-lock.json
to the working directory.RUN npm install
: Installs the application dependencies.COPY . .
: Copies the rest of the application code to the working directory.EXPOSE 3000
: Informs Docker that the application inside the container will use port 3000.CMD ["node", "app.js"]
: Specifies the command to run the application when the container starts.
This is a basic example for a Node.js application. Depending on your application and requirements, you might need additional configuration or steps in your Dockerfile.
Multistage Docker
Multistage builds in Docker allow you to create a more efficient and smaller final image by using multiple stages in the build process. Each stage is like a temporary container where you perform specific tasks, and only the necessary artifacts are carried over to the final stage. This is particularly useful for reducing the size of the resulting Docker image.
Here's a simple example of a multistage Dockerfile for a Node.js application:
# Stage 1: Build Stage
FROM node:14 as builder
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
# Stage 2: Production Stage
FROM node:14-alpine
WORKDIR /usr/src/app
COPY --from=builder /usr/src/app/dist ./dist
COPY --from=builder /usr/src/app/package*.json ./
RUN npm install --only=production
CMD ["node", "dist/app.js"]
Explanation:
Stage 1 (Build Stage):
FROM node:14 as builder
: Starts with a Node.js image and names it "builder."WORKDIR /usr/src/app
: Sets the working directory for the build stage.COPY package*.json ./
: Copies the package files for dependency installation.RUN npm install
: Installs dependencies.COPY . .
: Copies the application code.RUN npm run build
: Builds the application. This could be any build process relevant to your application.
Stage 2 (Production Stage):
FROM node:14-alpine
: Starts with a minimal Node.js image for production.WORKDIR /usr/src/app
: Sets the working directory for the production stage.COPY --from=builder /usr/src/app/dist ./dist
: Copies only the necessary artifacts (built code) from the build stage.COPY --from=builder /usr/src/app/package*.json ./
: Copies the package files.RUN npm install --only=production
: Installs production dependencies only.CMD ["node", "dist/app.js"]
: Specifies the command to run the application in the production stage.
The multistage Dockerfile reduces the size of the final image by excluding unnecessary build dependencies and files from the production image. It improves security and efficiency in the production environment.
Summary:
Volume: External storage for persistent data.
Networking: Communication pathways between containers.
Multistage: Optimization for smaller, efficient Docker images.
Compose: Tool for defining and running multi-container applications.
These advanced Docker features enhance flexibility, scalability, and efficiency in managing and deploying containerized applications.
Subscribe to my newsletter
Read articles from Avik Dutta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
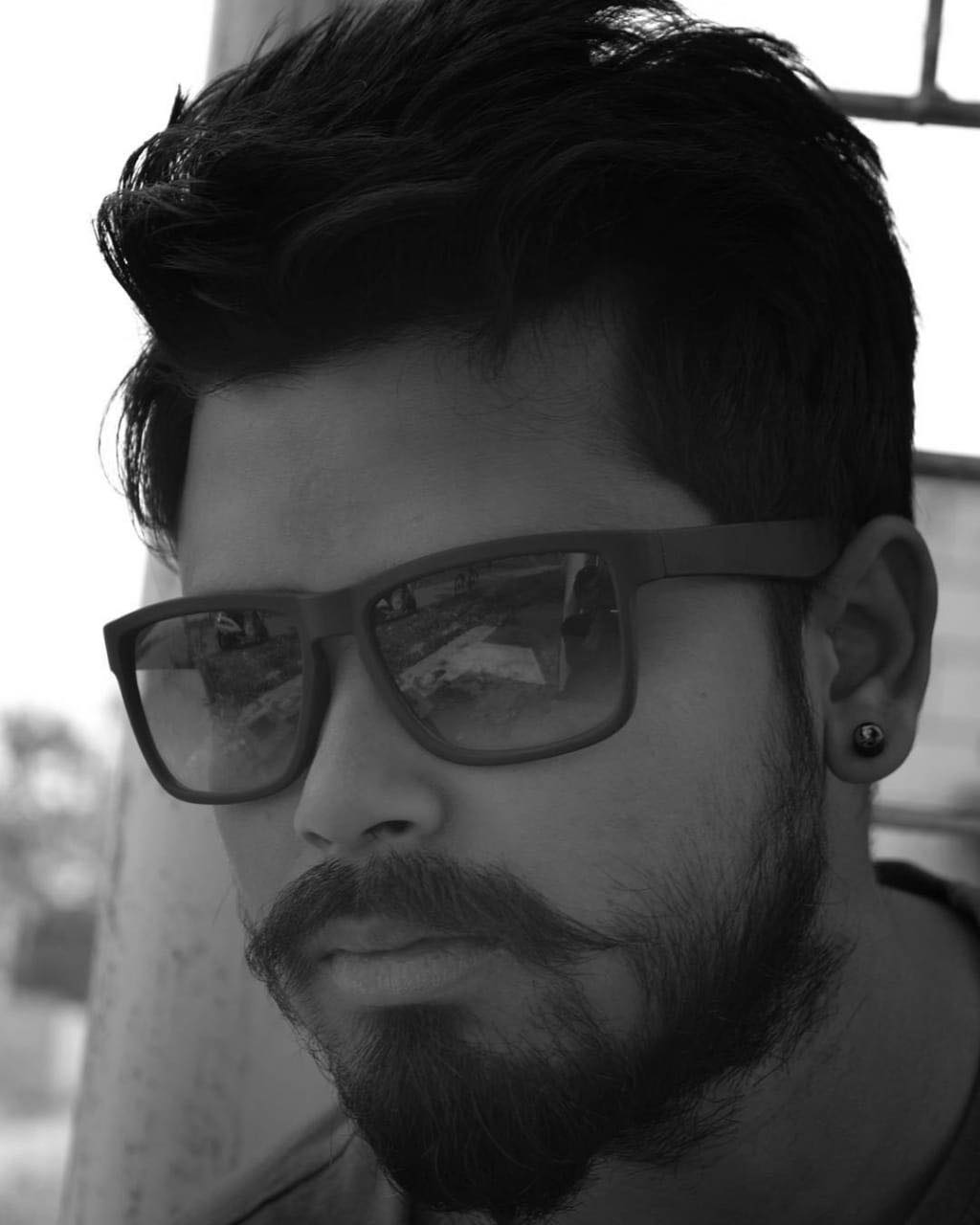
Avik Dutta
Avik Dutta
Experienced with a demonstrated history of working in the information technology and services industry. Strong engineering professional skilled in Linux and Cloud platform, AWS, Azure. Passionate about DevOps....