Mastering the "Final" Touch in Java
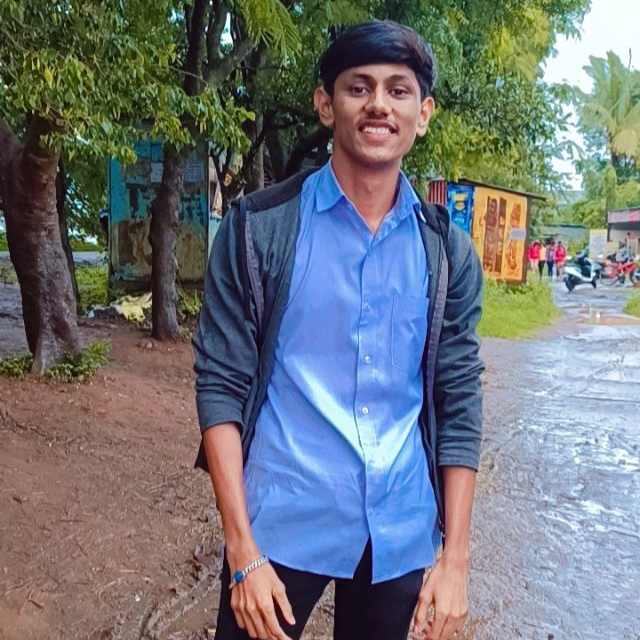
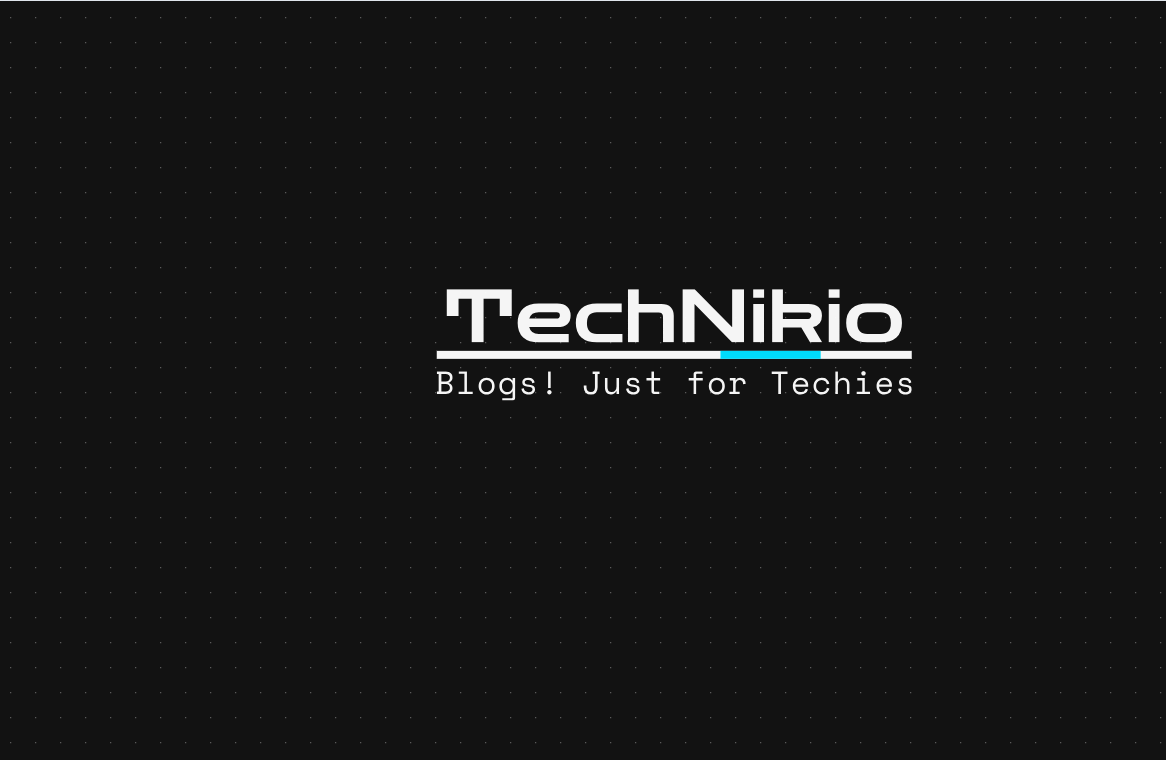
1. Introduction:
Greetings, Java enthusiasts! Today, let's unravel the power of the "final" keyword a versatile gem that adds precision and clarity to your Java code. Buckle up as we explore how this keyword, whether applied to variables, methods, or classes, can elevate your coding finesse.
#CodeMagicLaughs🦸♂️
2. The "Final" Keyword Unveiled
The final keyword is used in :
Variable
Method
Class
3. Final Variables: Constants Redefined
In Java, marking a variable as "final" transforms it into a constant. Once assigned, the value cannot be altered. This not only enhances code readability but also provides a safeguard against inadvertent changes.
If you make any variable as final, you cannot change the value of final variable(It will be constant).
Final Variables must be initialised.
You can reinitialise the final variable using constructors.
You can mark local variable as a final.
class Circle {
final double PI = 3.14; // A final variable
void calculateArea(final double radius) {
final double area = PI * radius * radius; // A final variable in a method
System.out.println("Area: " + area);
}//End calculateArea
}//End class
4. Final Methods: Immutable Blueprints
Declaring a method as "final" in a class prevents it from being overridden by subclasses. This enforces immutability in the method's behavior, promoting robust and predictable code.
Final method is instance method.
Final method cannot be overidden.
- Preventing Method Overriding:
A final method in a class cannot be overridden by subclasses. This ensures that the behavior of the method remains constant across the class hierarchy. Any attempt to override a final method will result in a compilation error.
class Parent {
final void performOperation() {
// Final method implementation
}
}
class Child extends Parent {
// Attempting to override the final method will result in a compilation error
}
- Ensuring Method Immutability:
Marking a method as final indicates that its implementation should not be changed by subclasses. This enforces method immutability, providing a clear and unmodifiable blueprint. This is particularly useful when certain behaviors need to be fixed and not altered in subclasses.
class ImmutableClass {
final void processInput(String input) {
// Final method ensures that the processing logic remains constant
}
}
- Compiler and Runtime Optimization:
The "final" keyword provides opportunities for compiler and runtime optimizations. Since the method cannot be overridden, the compiler may perform optimizations, and the JVM can make certain assumptions about the method's behavior, potentially leading to improved performance.
final class UtilityClass {
final int performCalculation(int a, int b) {
// Final method allows potential compiler and runtime optimizations
return a + b;
}
}
class Parent {
final void performOperation() {
// Final method implementation
}
}
class Child extends Parent {
// Attempting to override the final method will result in a compilation error
}
5. Final Classes: Unmodifiable Entities
When a class is marked as "final," it signifies that the class itself cannot be extended or subclassed. This is particularly useful when you want to create unmodifiable entities.
Final Class cannot be extended.
Final Class cannot be subclassed.
Final inner Class is allowed.
We cannot use final and abstract keywords together in methods. Want to know Why?
final class UtilityClass {
// Class with final keyword
}//End class
6. Embracing the Benefits
Code Safety and Readability
The "final" keyword acts as a guardian, ensuring that values, methods, or entire classes remain unaltered where intended. This enhances code safety and readability, making your intentions clear to fellow developers.
Performance Optimization
In certain scenarios, the JVM can optimize code more effectively when variables or methods are marked as "final." This can result in improved performance.
7. Best Practices and Considerations
Choose Wisely:
- Apply the "final" keyword judiciously. While it brings benefits, excessive use may lead to rigid code.
Constants Convention:
- For constants, consider using uppercase letters with underscores (e.g.,
MAX_SIZE
).Immutable Objects:
- Combine "final" with other practices to create immutable objects for enhanced code robustness.
8. How To Create A CONSTANT In Java?
Constants are always decided in upper case.
Conclusion:
The "final" keyword in Java is a silent hero, ensuring code stability and reinforcing design choices. Embrace it wisely, and witness the transformation of your code into a robust and reliable masterpiece.
Exciting times lie ahead! Get ready to unravel the secrets of JDK, JVM, and JIT—the powerhouse trio shaping Java's magic. 🌐💻 Brace for the next chapter! #JavaMagic #StayTuned 🚀✨
#CodeMagicLaughs🦸♂️
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Nikhil Abhiman Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
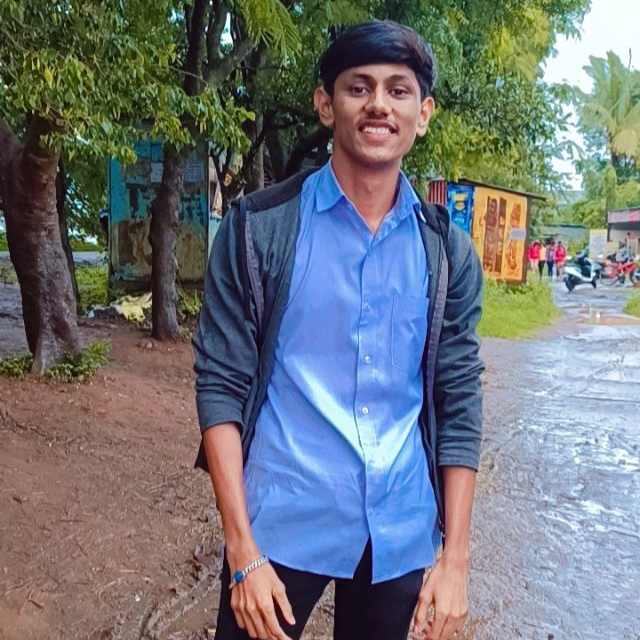
Nikhil Abhiman Jadhav
Nikhil Abhiman Jadhav
Hey there 👋🏻, I'm Nikhil Jadhav, a passionate tech enthusiast and aspiring writer on a mission to demystify the complexities of coding and technology. By day, I'm immersed in lines of code, and by night, I'm weaving words to make tech more accessible and enjoyable for everyone. 📬 Get in touch Twitter: https://twitter.com/Technikio LinkedIn:linkedin.com/in/nikhil-7571nik GitHub:github.com/jadhavnikhil2624