Working with JavaScriptExecutor in Selenium
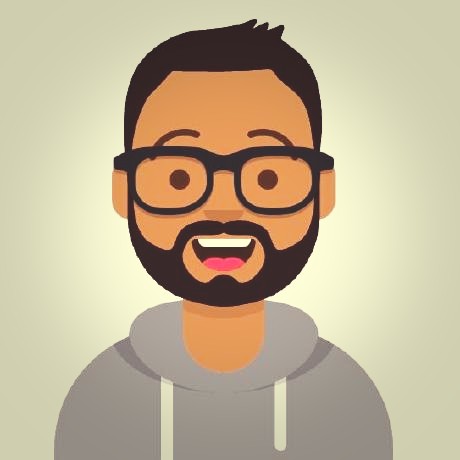
Introduction
Selenium is a powerful tool for automated testing of web applications, but there are scenarios where interacting with the browser using traditional Selenium commands may not be sufficient.
For instance, the user opens a URL and there is an unexpected pop-up that will prevent the WebDriver from locating a specific element and produce inaccurate results. This is where JavaScriptExecutor comes into play. In this blog, we'll explore what JavaScriptExecutor is and how it can be utilized in Selenium with Java.
What is JavaScriptExecutor?
In simple words, JavascriptExecutor is an interface that is used to execute JavaScript with Selenium. To simplify the usage of JavascriptExecutor in Selenium, think of it as a medium that enables the WebDriver to interact with HTML elements within the browser. JavaScript is a programming language that interacts with HTML in a browser, and to use this function in Selenium, JavascriptExecutor is required.
Methods provided by JavaScriptExecutor
executeScript
This method executes JavaScript in the currently selected window or frame. The script runs as an anonymous function, and it can return various data types such as WebElement, List, String, Long, or Boolean.
// Import the package import org.openqa.selenium.JavascriptExecutor; // Create a reference JavascriptExecutor js = (JavascriptExecutor) driver; // Execute the script js.executeScript(script, args);
executeAsyncScript
This method is used to execute asynchronous JavaScript in the current window or frame. Asynchronous JavaScript executes in a single thread while the rest of the page continues parsing, enhancing performance.
Getting Started with JavaScriptExecutor
To use JavaScriptExecutor in Selenium:
Import the package:
Create a reference:
Call the JavaScriptExecutor method:
//importing the package import org.openqa.selenium.JavascriptExecutor; //creating a reference JavascriptExecutor js = (JavascriptExecutor) driver; //calling the method js.executeScript(script, args);
Examples of JavaScriptExecutor in Selenium
Example 1: Refresh the browser window
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("location.reload()");
Example 2: Send text to an element
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("document.getElementById('elementId').value = 'xyz';");
Example 3: Generate an Alert Pop Window
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("alert('hello world');");
Example 4: Get InnerText of a Webpage
JavascriptExecutor js = (JavascriptExecutor) driver;
String sText = (String) js.executeScript("return document.documentElement.innerText;");
Example 5: Get Title of a WebPage
JavascriptExecutor js = (JavascriptExecutor) driver;
String sText = (String) js.executeScript("return document.title;");
Example 6: Scroll Page
JavascriptExecutor js = (JavascriptExecutor) driver;
// Vertical scroll – down by 150 pixels
js.executeScript("window.scrollBy(0,150)");
Example 7: Click a button
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("document.getElementByID(‘element id ’).click();");
Example 8: Interact with checkbox
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("document.getElementByID(‘element id ’).checked=false;");
Best Practices for Using JavaScriptExecutor
Avoid Using Hard Waits: JavaScriptExecutor can execute asynchronously, so prefer using it along with WebDriver's wait mechanism to ensure synchronization.
Error Handling: Wrap your JavaScriptExecutor calls in try-catch blocks to handle any potential exceptions gracefully.
Document Your Scripts: Clearly document the purpose and functionality of your JavaScriptExecutor scripts for better code maintainability.
Testing in Multiple Browsers: Test your JavaScriptExecutor scripts across different browsers to ensure cross-browser compatibility.
Conclusion
JavaScriptExecutor in Selenium provides a powerful way to interact with web elements using JavaScript, giving testers more flexibility and control over their automated tests. Utilizing JavaScriptExecutor, you can perform actions and retrieve information that may not be possible with traditional Selenium WebDriver commands.
By following best practices and understanding the nuances of JavaScriptExecutor, you can enhance the robustness and reliability of your Selenium test scripts. As you explore and incorporate JavaScriptExecutor into your testing strategy, you'll find that it opens up a world of possibilities for automating complex scenarios in web applications.
Subscribe to my newsletter
Read articles from Rinaldo Badigar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
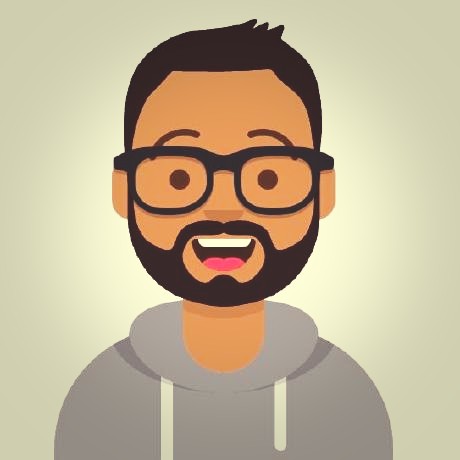
Rinaldo Badigar
Rinaldo Badigar
Software Engineer with 3+ years of experience in Automation, with a strong foundation in Manual, API, and Performance testing, ensuring high-quality software delivery for Ecommerce and Banking applications.