Mastering the static Keyword in Java: A Beginner's Guide to Expert Understanding
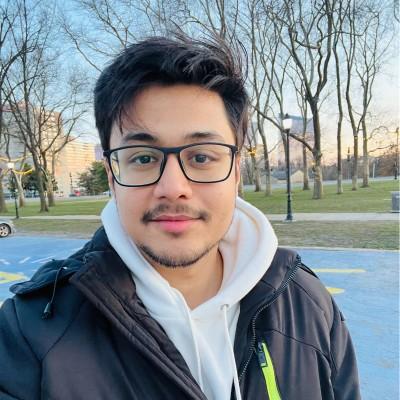
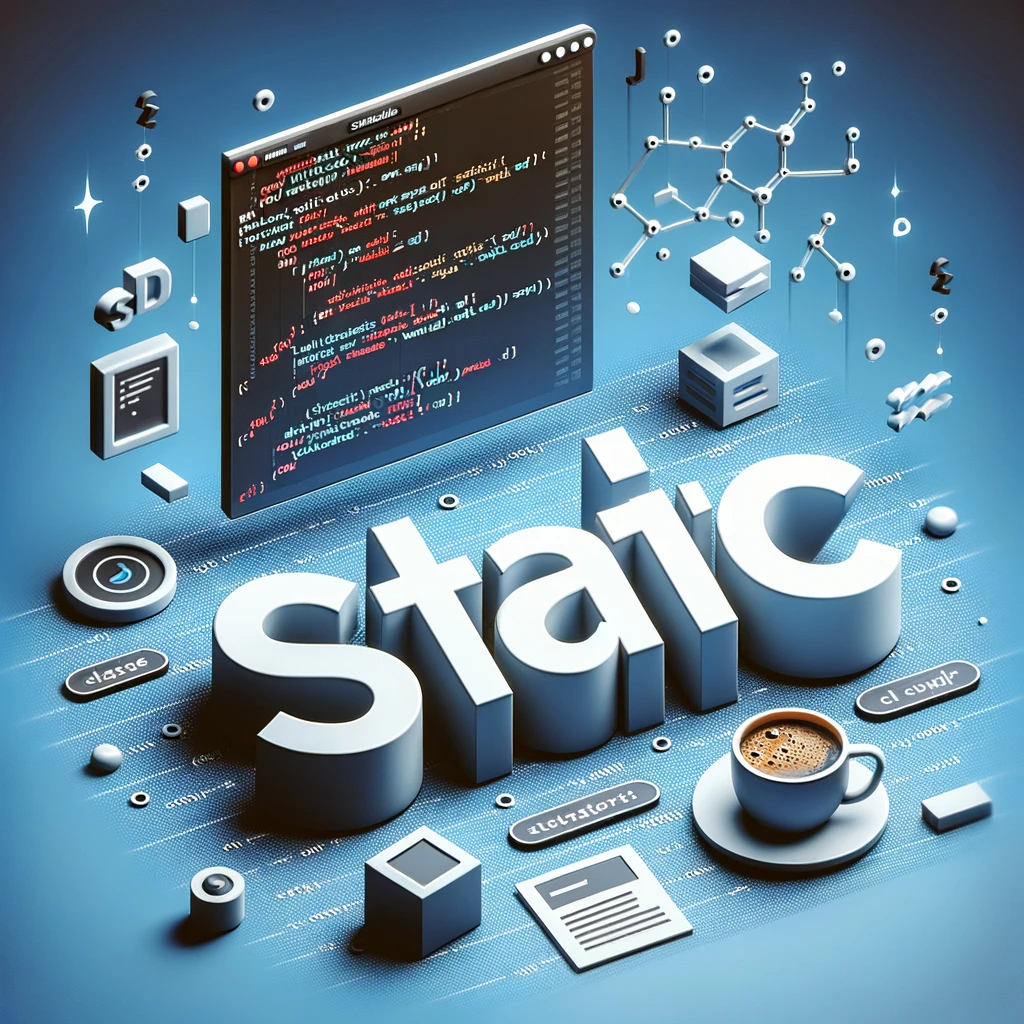
Introduction
Welcome to the world of Java programming! If you've been curious about what the static
keyword does in Java, you're in the right place. This guide is tailored for beginners but packed with enough detail to elevate your understanding to an expert level. We'll explore static
in a fun, intuitive way, with plenty of examples to solidify your learning.
Getting to Know static
in Java
Imagine you're building a robot. Every robot you build has a unique name, but they're all made in the same factory. In Java, each robot is an 'object,' the factory is a 'class,' and static
is a special word we use to say something belongs to the factory itself, not just one robot.
What Does static
Mean?
In Java, static
means that a particular variable or method belongs to the class as a whole, rather than to any individual object (or robot, in our analogy). It's like a shared resource or tool that any object created from that class can use.
Static Variables: Sharing Common Features
Let's dive into static variables with an example. Suppose we're coding a program that tracks how many robots we've made.
public class RobotFactory {
private static int robotCount = 0; // This is a static variable
public RobotFactory() {
robotCount++; // We increase the count for every new robot made
}
public static int getRobotCount() {
return robotCount; // This method lets us see how many robots we've made
}
}
In this example, robotCount
is a static variable. It's shared by all instances (or robots) created from the RobotFactory
class. Every time a new robot is made, robotCount
goes up, no matter which specific robot it is.
Why Use Static Variables?
Static variables are like a shared scoreboard or a common piece of data that every object created from the class can see and use. They're perfect for when you have information that isn't specific to one instance but is common to all instances.
Static Methods: Tools for Everyone
Now, let's talk about static methods. These are like tools in our factory that any robot can use, regardless of their individual features.
Consider a utility method that converts inches to centimeters. This is a common action and doesn't depend on individual robot characteristics.
public class MeasurementTool {
public static double inchesToCentimeters(double inches) {
return inches * 2.54; // Converts inches to centimeters
}
}
Here, inchesToCentimeters
is a static method. You don't need a specific instance to use it. Any part of your program can call MeasurementTool.inchesToCentimeters
and get the job done.
When to Use Static Methods
Static methods are fantastic for actions that are general-purpose and not tied to the state of an object. They're like communal tools or utilities that any instance, or even outside classes, can use.
Static Blocks: Special Setup Routines
Imagine you need to set up some common data or configurations before making any robots. This is where static blocks come in.
public class RobotFactory {
private static Map<String, String> configurations;
static {
configurations = new HashMap<>();
configurations.put("defaultColor", "Silver");
// Add more configurations
}
}
In this code snippet, the static block runs once when the class is first used. It's setting up common configurations for all robots.
Conclusion: Embracingstatic
The static
keyword is a powerful feature in Java, allowing shared resources and utilities to be efficiently managed at the class level. It's like having a set of tools and counters available to all instances created from a class, promoting efficiency and shared functionality.
Next Steps
Experiment with static
in your Java programs. Try creating static variables, methods, and blocks in a sample class. See how they behave differently from instance-level features. Remember, practice makes perfect in the world of programming!
Subscribe to my newsletter
Read articles from Pratik Upreti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
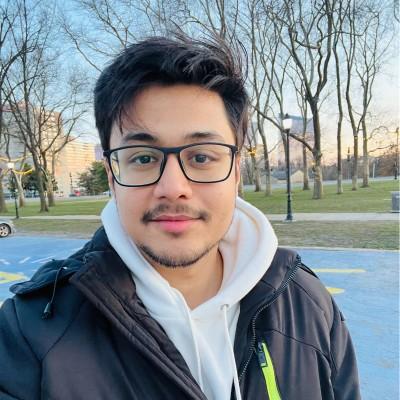
Pratik Upreti
Pratik Upreti
Hello! I’m Pratik Upreti, a seasoned software engineer with a rich background in languages like C#, Java, JavaScript, Python, SQL, and MySQL, I bring a diverse skill set to the table. I excel in backend and server technologies, including Node.js, Django, GraphQL, and Prisma ORM, and am adept in frontend development with Next.js, React.js and Tailwind CSS. Professionally, contributing at Brilliant Infotech.