How to setup your NodeJs project with TypeScript?

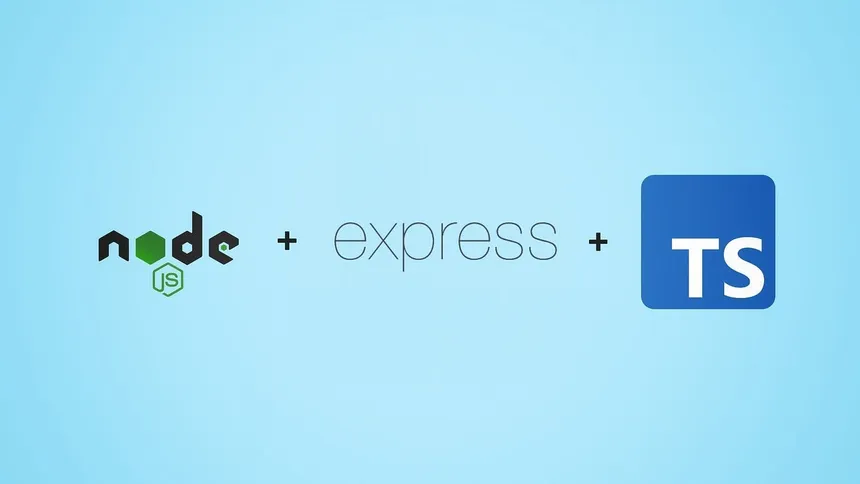
I am going to teach you how to setup your nodejs project with typescript.
Why TypeScript?
TypeScript is JavaScript with static typing, allowing you to define the type of variables at compile-time. This helps you to catch errors during development and reducing run-time bugs.
Other developers can understand the expected type of variables and function parameters making it easier to collaborate.
Excellent support for modern IDE's like VS Code give you features like autocompletion and real-time error checking.
Frameworks like nextjs, vite come with built in typescript compiler although you need to configure it in a node project.
How to?
First start with initializing a node project -
npm init -y
This will create a package.json file. Now install typescript as a dev dependency with the command
npm install -D typescript
In your package.json file add
{
"name": "server",
"version": "1.0.0",
"description": "",
"main": "index.ts",
"scripts": {
"build": "tsc" //This script object over here it converts the javascript code to typescript.
},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"typescript": "^5.3.3"
},
}
Now install the types for node since it won't be able to find it's type declaration. Run
npm install ts-node
Now create a src folder and create an index.ts file inside it.
Once done create a tsconfig.json file outside. In the file add
{
"compilerOptions": {
"module": "NodeNext",
"moduleResolution": "NodeNext",
"target": "ES2020",
"baseUrl": "src",
"rootDir": "src",//This is the root directory
"outDir": "dist", //This is the directory where the converted javascript code will live
"sourceMap": true,
"noImplicitAny": true,
},
"include": ["src/**/*"],
}
Now run
npm run build
This will create a dist folder where you can see the converted javascript code.
NOTE :
Whenever you install dependencies like express, cors etc. Do install their types as well
npm install express
npm install -D @types/express
Subscribe to my newsletter
Read articles from Nishant Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nishant Sharma
Nishant Sharma
I am web developer. A competitive Coder. Always Learning.