Day 2
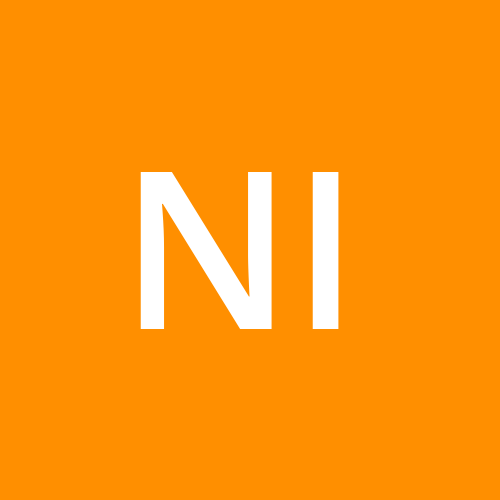
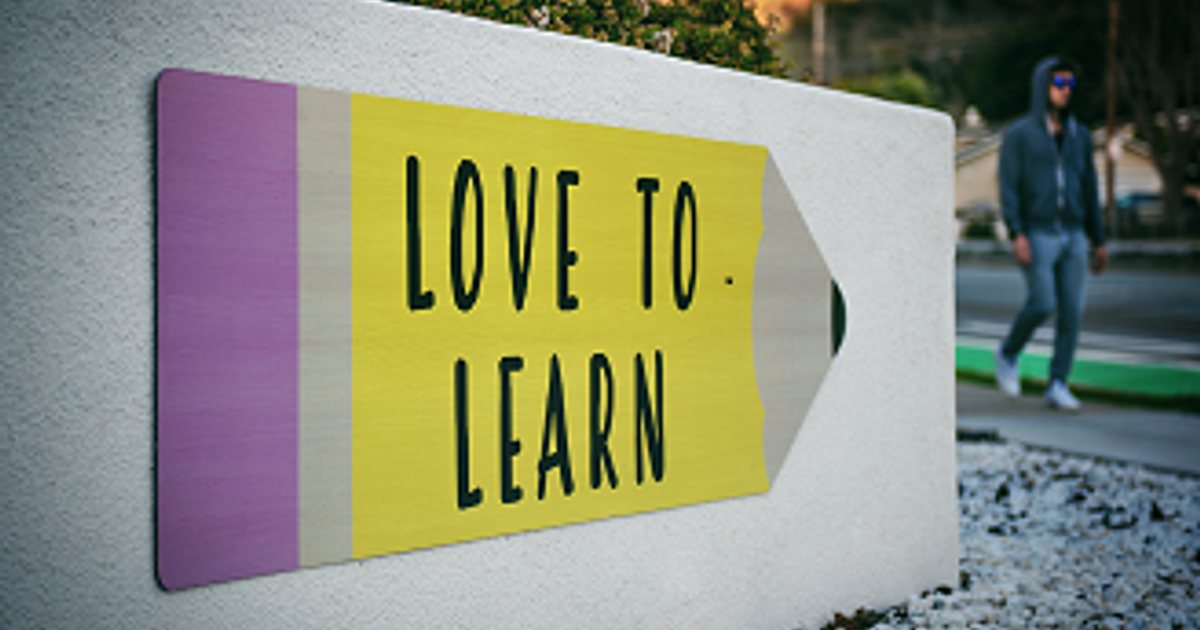
On Day 2 I did some 3 easy question on string questions with the PODT in geeksforgeeks.
PODT question (easy)
Given an integer K and a queue of integers, we need to reverse the order of the first K elements of the queue, leaving the other elements in the same relative order.
Only following standard operations are allowed on queue.
enqueue(x) : Add an item x to rear of queue
dequeue() : Remove an item from front of queue
size() : Returns number of elements in queue.
front() : Finds front item.
Note: The above operations represent the general processings. In-built functions of the respective languages can be used to solve the problem.
Input:
5 3
1 2 3 4 5
Output:
3 2 1 4 5
Explanation:
After reversing the given
input from the 3rd position the resultant
output will be 3 2 1 4 5.
queue<int> modifyQueue(queue<int> q, int k) {
queue<int>ans;
stack<int>st;
for(int i=0;i<k;i++)
{
st.push(q.front());
q.pop();
}
while(st.empty()==false)
{
ans.push(st.top());
st.pop();
}
while(q.size()!=0)
{
ans.push(q.front());
q.pop();
}
return ans;
}
In this question we use stack for reversing the k part of the queue.
Next question (easy but needed hint)
Given two strings of lowercase alphabets and a value K, your task is to complete the given function which tells if two strings are K-anagrams of each other or not.
Two strings are called K-anagrams if both of the below conditions are true.
1. Both have same number of characters.
2. Two strings can become anagram by changing at most K characters in a string.
Input:
str1 = "fodr", str2="gork"
k = 2
Output:
1
Explanation: Can change fd to gk
For this question we use map where we run 2 for loops serially, first for loop adds the element with it's frequency, then we with another for loop taking str2 we start removing the element , like this the common elements will be removed , now remaining elements we divide it by two because we have to convert 1 to another , so after dividing by 2 if the answer is less than or equal to k then it is k-anagram.
bool areKAnagrams(string str1, string str2, int k) {
int s1=str1.size();
int s2=str2.size();
if (s1!=s2) return 0;
unordered_map<char, int> mpp;
for(auto it: str1) mpp[it]++;
for(auto it: str2 ) mpp[it]--;
int add=0;
for(auto it: mpp) add+=abs(it.second);
return (add/2)<=k;
}
Next question(easy but learnt new thing in string)
Given a string Str which may contains lowercase and uppercase chracters. The task is to remove all duplicate characters from the string and find the resultant string. The order of remaining characters in the output should be same as in the original string.
Input:
Str = geeksforgeeks
Output: geksfor
Explanation: After removing duplicate
characters such as e, k, g, s, we have
string as "geksfor".
This is a easy question and I solved it by my own but when I saw other's solution I learnt a new thing in the string topic which I wanted to note it down, here I have only given solution which is not mine.
string removeDuplicates(string str) {
// code here
string s = "";
s.push_back(str[0]);
for(int i = 1; i<str.length(); i++){
if(s.find(str[i]) == string::npos){ //this is important
s.push_back(str[i]);
}
}
return s;
}
I could not start Dynamic programming today as I didn't get time but might start from tomorrow. And today I did 30mins of walk as an outdoor activity.
Subscribe to my newsletter
Read articles from Nitishkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
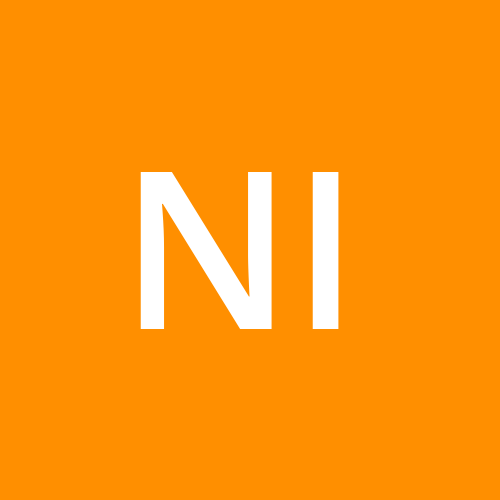