Java Lambda Expression
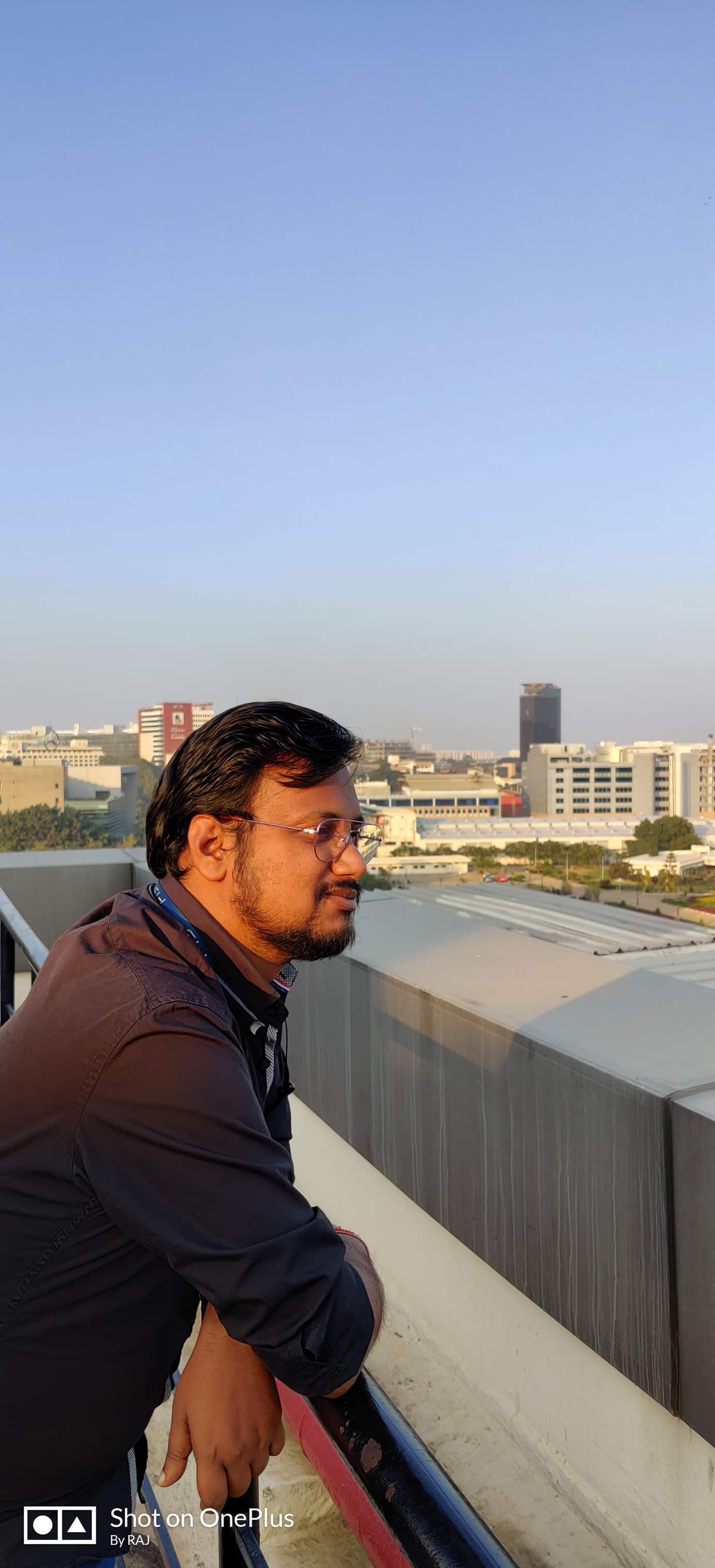
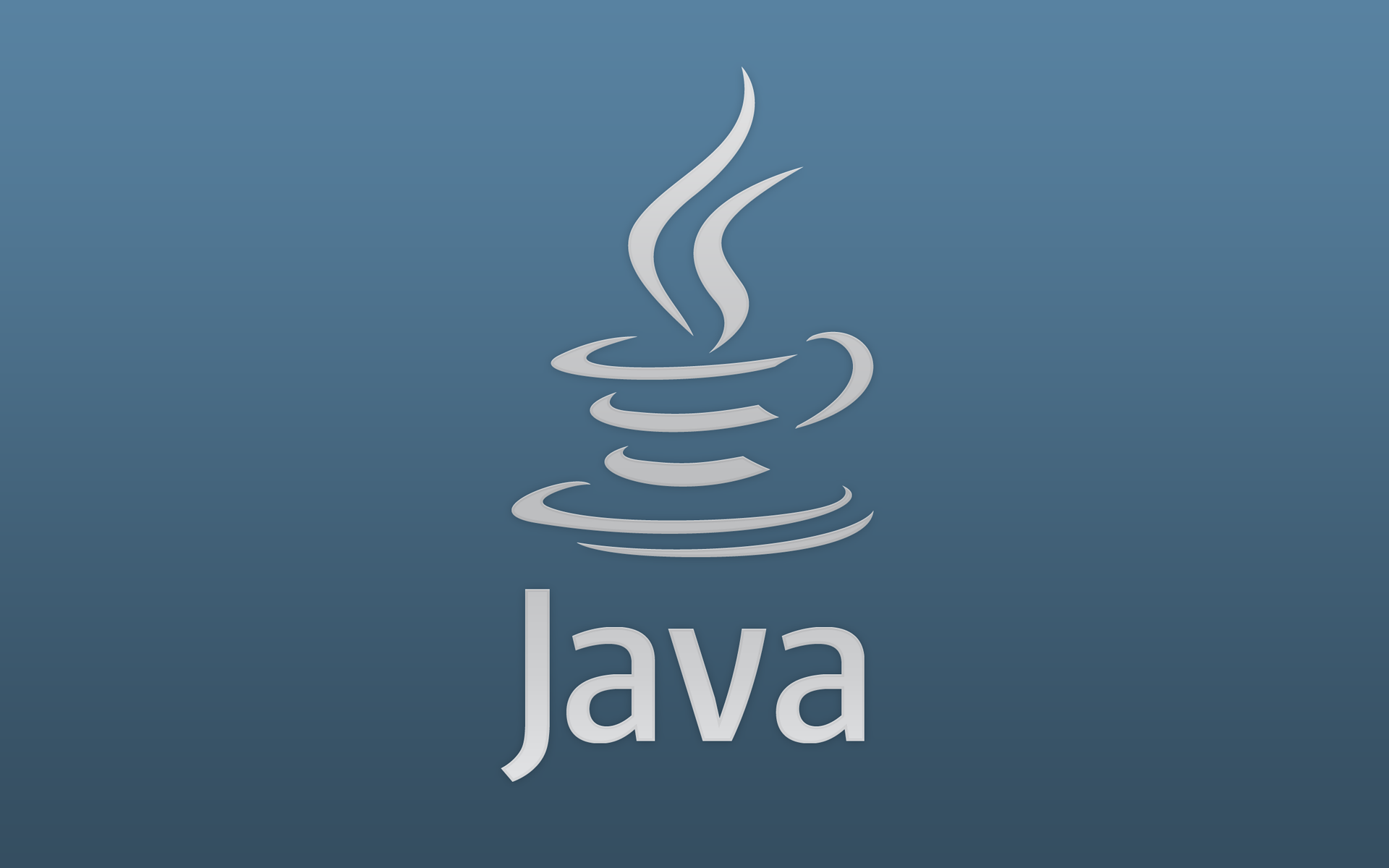
Lambda expressions in Java provide a concise and expressive way to write anonymous functions (implementations of functional interfaces). Lambda expressions streamline the syntax, making code more readable and allowing for more flexible use of functional programming concepts. Let's explore the syntax and usage with an example:
Lambda Expression Syntax:
The basic syntax of a lambda expression consists of parameters, an arrow (->
), and a body. It is defined as follows:
(parameters) -> expression
or
(parameters) -> { statements; }
Example: Sorting with Lambda Expressions:
Suppose you have a list of strings and you want to sort them using the Comparator
interface with a lambda expression.
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class LambdaExample {
public static void main(String[] args) {
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
names.add("David");
// Sorting with anonymous class (pre-Java 8)
Collections.sort(names, new Comparator<String>() {
@Override
public int compare(String s1, String s2) {
return s1.compareTo(s2);
}
});
System.out.println("Sorted (pre-Java 8): " + names);
// Sorting with lambda expression (Java 8)
Collections.sort(names, (String s1, String s2) -> s1.compareTo(s2));
System.out.println("Sorted with Lambda: " + names);
// Simplified lambda expression (type inference)
Collections.sort(names, (s1, s2) -> s1.compareTo(s2));
System.out.println("Sorted with Simplified Lambda: " + names);
}
}
In this example:
The lambda expression
(String s1, String s2) -> s1.compareTo(s2)
is used as an argument to theCollections.sort
method.The
Comparator
interface is a functional interface with a single abstract methodcompare
. The lambda expression provides a concise way to implement this method.In the simplified lambda expression
(s1, s2) -> s1.compareTo(s2)
, the type of parameters is inferred, making the code even more concise.
Lambda expressions are particularly useful in scenarios where you need to pass behavior as an argument to a method, such as with sorting, filtering, or iterating over collections. They promote a more functional style of programming in Java and contribute to cleaner and more expressive code.
Subscribe to my newsletter
Read articles from Navnit Raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
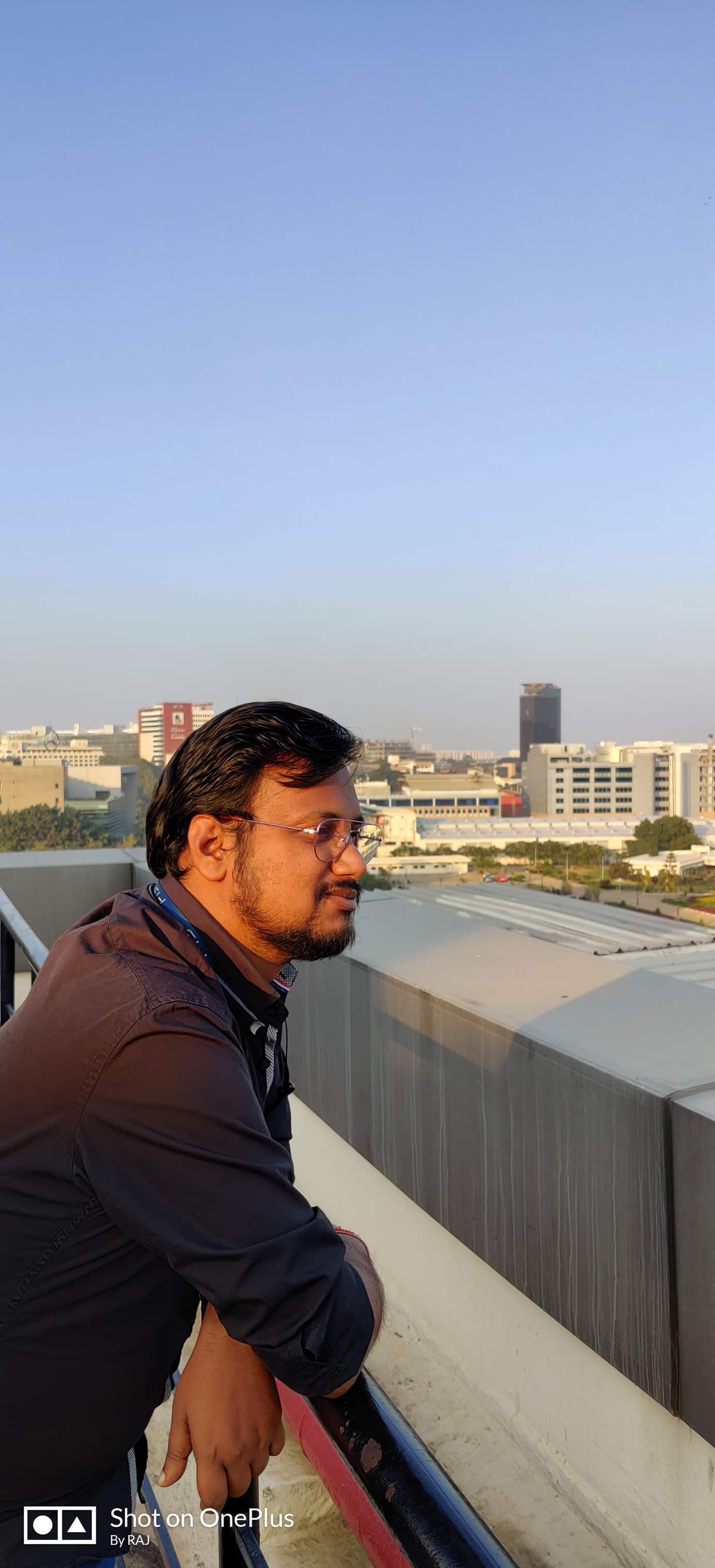