Stream API in Java
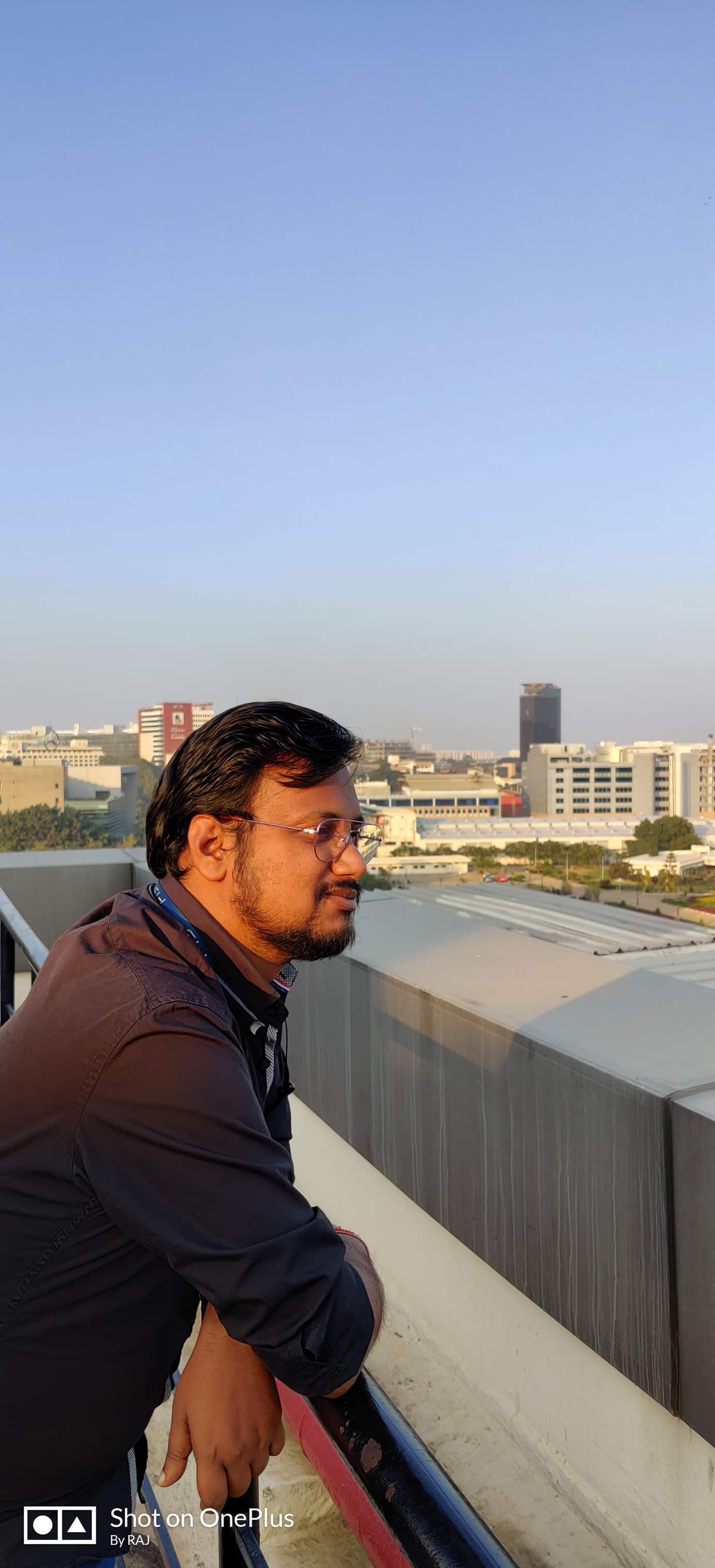
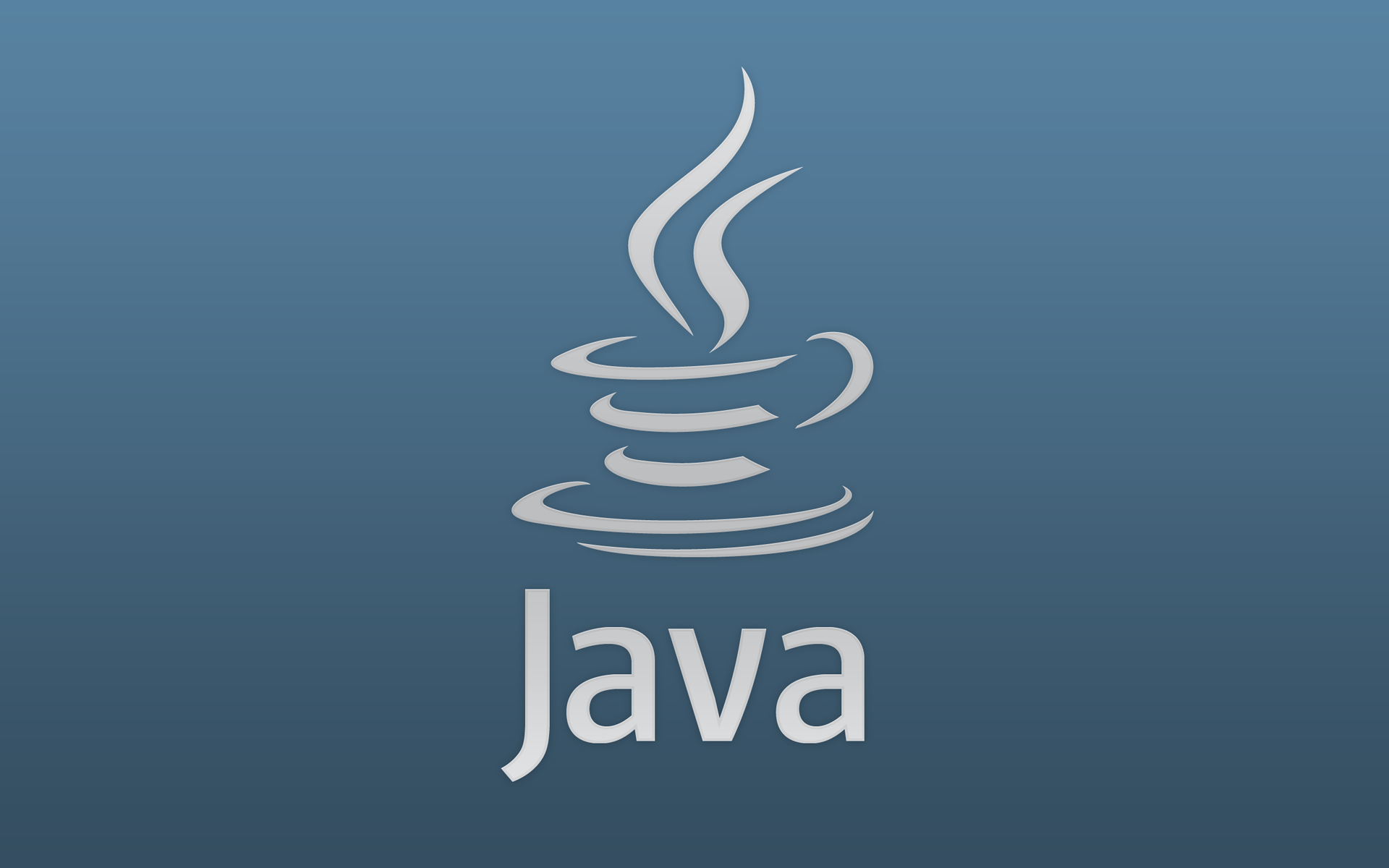
Java Stream API, introduced in Java 8, provides a powerful and expressive way to process collections of data in a functional and declarative manner. Streams enable you to perform operations like filtering, mapping, and reducing on data sets with concise and readable code. Let's explore the Stream API with examples:
Basics of Stream API:
1. Creating Streams:
- Streams can be created from collections, arrays, or by using
Stream
methods.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Creating a stream from a list
Stream<String> nameStream = names.stream();
// Creating a stream directly
Stream<String> directStream = Stream.of("Apple", "Banana", "Orange");
2. Intermediate Operations:
Intermediate operations are operations that transform a stream into another stream. They are lazy and do not execute until a terminal operation is invoked.
// Filter names starting with 'A'
Stream<String> filteredStream = nameStream.filter(name -> name.startsWith("A"));
// Map each name to its length
Stream<Integer> lengthStream = filteredStream.map(String::length);
3. Terminal Operations:
Terminal operations are operations that produce a result or a side-effect. They trigger the execution of the stream pipeline.
// Print lengths
lengthStream.forEach(System.out::println);
// Collect names starting with 'A' into a list
List<String> aNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
Examples:
Example 1: Filtering and Mapping
List<String> fruits = Arrays.asList("Apple", "Banana", "Orange", "Grapes");
// Using Stream API to filter and print
fruits.stream()
.filter(fruit -> fruit.startsWith("A"))
.map(String::toUpperCase)
.forEach(System.out::println);
Example 2: Sum of Squares
javaCopy codeList<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Using Stream API to find the sum of squares
int sumOfSquares = numbers.stream()
.map(x -> x * x)
.reduce(0, Integer::sum);
System.out.println("Sum of squares: " + sumOfSquares);
Example 3: Collecting to List
List<String> words = Arrays.asList("Java", "is", "fun", "and", "powerful");
// Using Stream API to collect words with length greater than 3 into a list
List<String> longWords = words.stream()
.filter(word -> word.length() > 3)
.collect(Collectors.toList());
System.out.println("Long words: " + longWords);
Benefits of Stream API:
Conciseness: Stream API allows you to express complex data processing tasks more concisely and readably.
Parallelism: Streams can leverage parallel processing, making it easier to perform parallel computations on large data sets.
Declarative Style: Stream operations follow a declarative style, where you specify what you want to achieve rather than how to achieve it.
Java Stream API simplifies the processing of collections, promoting a functional and declarative programming style. It is particularly useful when dealing with large data sets and complex data processing tasks.
Subscribe to my newsletter
Read articles from Navnit Raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
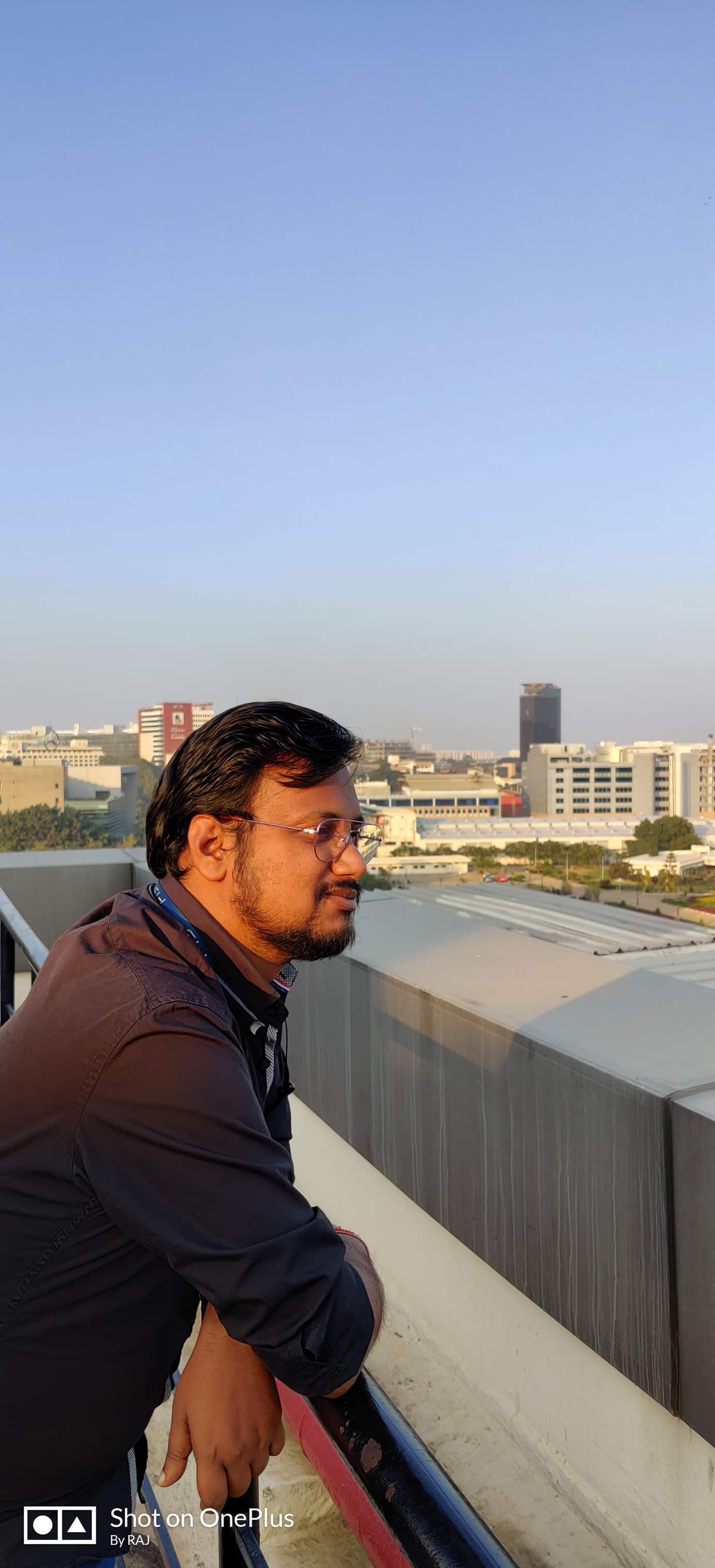