Optional Class in Java8
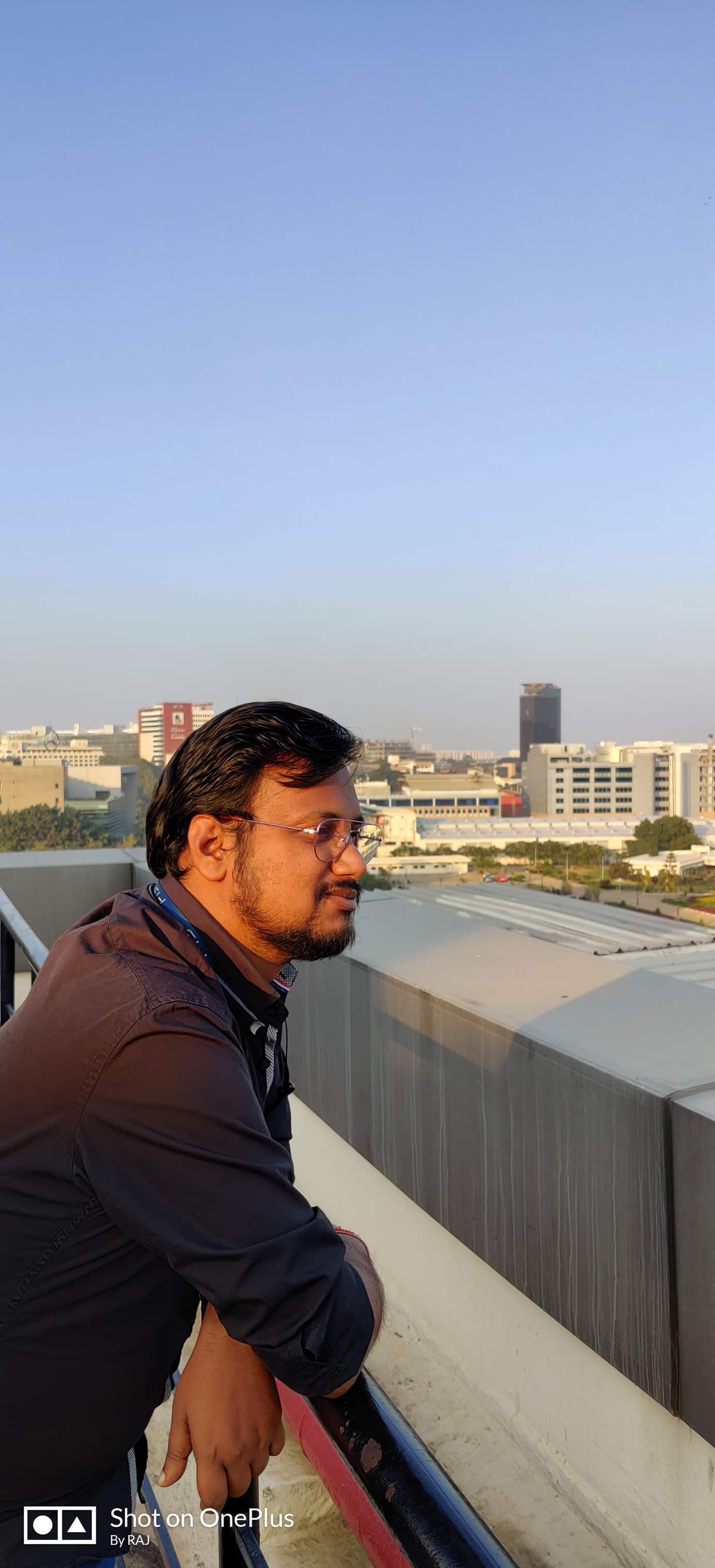
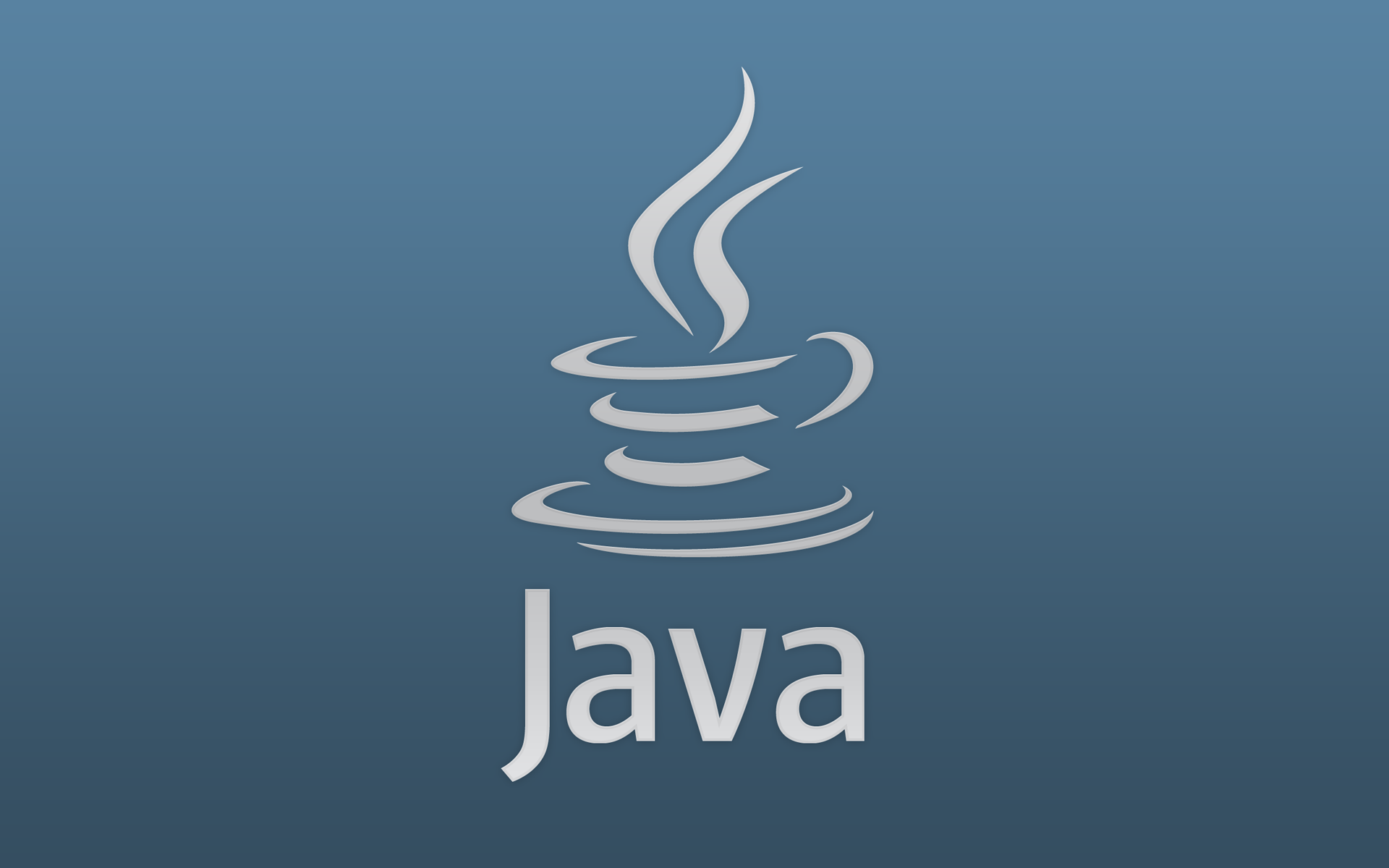
The Optional
class in Java was introduced in Java 8 to address the problem of handling potentially null values more effectively. It provides a container that may or may not contain a non-null value. Optional
is designed to encourage a more explicit and safer way of handling null values, reducing the likelihood of NullPointerExceptions
. The class encourages developers to consider the possibility of a value being absent and forces them to handle such cases explicitly.
Common Methods in Optional
Class:
of(T value)
:- Creates an
Optional
containing a non-null value.
- Creates an
Optional<String> optional = Optional.of("Hello, Optional!");
ofNullable(T value)
:- Creates an
Optional
containing a value if it's non-null; otherwise, returns an emptyOptional
.
- Creates an
String message = "Hello, Optional!";
Optional<String> optional = Optional.ofNullable(message);
empty()
:- Returns an empty
Optional
.
- Returns an empty
Optional<String> emptyOptional = Optional.empty();
isPresent()
:- Returns
true
if theOptional
contains a value, otherwise returnsfalse
.
- Returns
Optional<String> optional = Optional.of("Hello, Optional!");
if (optional.isPresent()) {
// Value is present, do something
}
get()
:- Returns the value if present; otherwise, throws
NoSuchElementException
.
- Returns the value if present; otherwise, throws
Optional<String> optional = Optional.of("Hello, Optional!");
String value = optional.get();
orElse(T other)
:- Returns the value if present; otherwise, returns the specified default value.
Optional<String> optional = Optional.empty();
String result = optional.orElse("Default Value");
orElseGet(Supplier<? extends T> other)
:- Returns the value if present; otherwise, returns the result produced by the supplying function.
Optional<String> optional = Optional.empty();
String result = optional.orElseGet(() -> "Default Value");
orElseThrow(Supplier<? extends X> exceptionSupplier)
:- Returns the value if present; otherwise, throws an exception produced by the supplying function.
Optional<String> optional = Optional.empty();
String result = optional.orElseThrow(() -> new NoSuchElementException("Value is absent"));
Example Usage:
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
// Creating Optional with a non-null value
Optional<String> messageOptional = Optional.of("Hello, Optional!");
// Creating Optional with a nullable value
String nullableMessage = null;
Optional<String> nullableOptional = Optional.ofNullable(nullableMessage);
// Creating an empty Optional
Optional<String> emptyOptional = Optional.empty();
// Checking if a value is present
if (messageOptional.isPresent()) {
System.out.println(messageOptional.get());
}
// Using orElse to provide a default value
String defaultValue = nullableOptional.orElse("Default Value");
System.out.println(defaultValue);
// Using orElseGet with a Supplier
String result = emptyOptional.orElseGet(() -> "Another Default");
System.out.println(result);
// Using orElseThrow to handle absence with an exception
try {
String value = emptyOptional.orElseThrow(() -> new RuntimeException("Value is absent"));
System.out.println(value);
} catch (RuntimeException e) {
System.err.println(e.getMessage());
}
}
}
In this example:
Optional
is used to wrap values, and various methods are demonstrated to handle the presence or absence of values.orElse
,orElseGet
, andorElseThrow
are used to provide default values or handle the absence of values gracefully.The use of
isPresent
andget
methods shows how to check for the presence of a value before accessing it.
By using Optional
, developers can create more robust and predictable code, reducing the likelihood of NullPointerExceptions
and making the handling of absent values more explicit.
Subscribe to my newsletter
Read articles from Navnit Raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
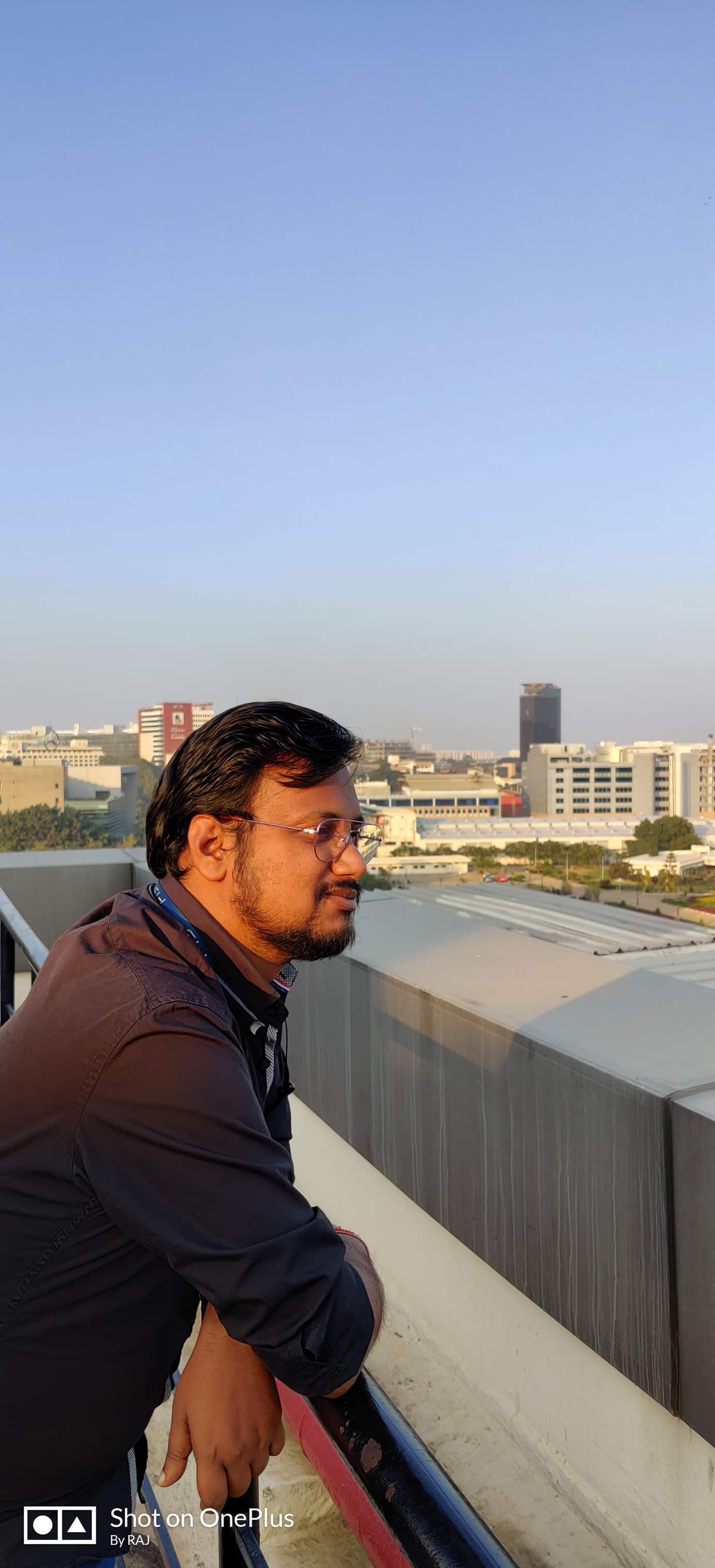