Java 9 New Feature
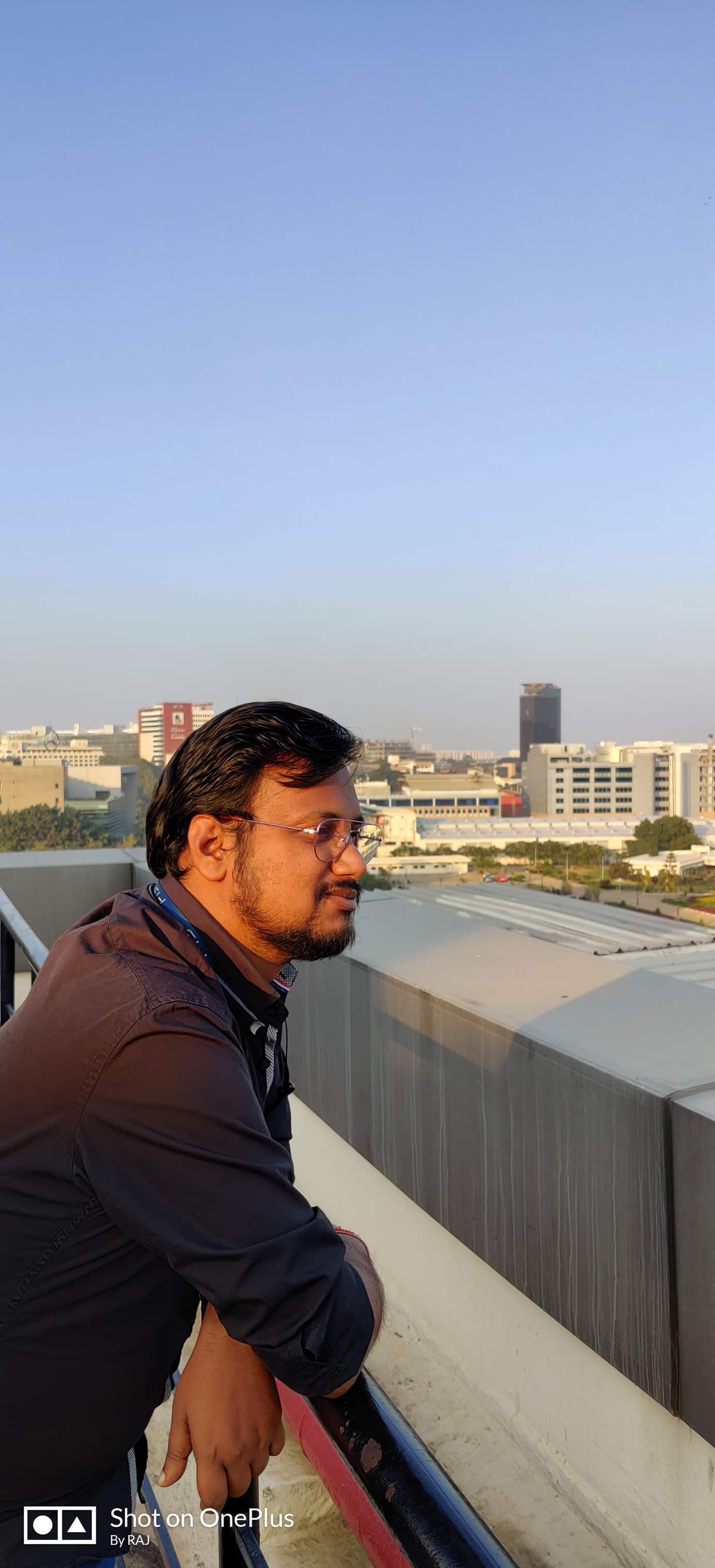

Java 9 introduced several features and enhancements to the language. Here's an overview of the key features along with examples:
1. Module System (Project Jigsaw):
Java 9 introduced a module system to address the challenges of modularity in large-scale applications.
Example: Creating a Module
// Module definition file (module-info.java)
module com.example.greeting {
exports com.example.greeting;
}
2. JShell: Interactive Java Shell:
JShell is an interactive REPL (Read-Eval-Print Loop) tool for quick experimentation and learning.
Example: Using JShell
$ jshell
| Welcome to JShell -- Version 9
| For an introduction type: /help intro
jshell> int x = 5;
x ==> 5
jshell> int square = x * x;
square ==> 25
jshell> System.out.println("Square: " + square);
Square: 25
3. HTTP/2 Client:
Java 9 introduced a new HTTP client that supports HTTP/2 and WebSocket.
Example: HTTP/2 Client
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class HttpClientExample {
public static void main(String[] args) throws Exception {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(new URI("https://jsonplaceholder.typicode.com/posts/1"))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println("Response Code: " + response.statusCode());
System.out.println("Response Body: " + response.body());
}
}
4. Process API Updates:
Java 9 enhanced the Process API to provide more control over native processes.
Example: ProcessHandle
import java.util.Optional;
public class ProcessHandleExample {
public static void main(String[] args) {
ProcessHandle currentProcess = ProcessHandle.current();
System.out.println("Current Process ID: " + currentProcess.pid());
Optional<String> info = currentProcess.info().command();
info.ifPresent(command -> System.out.println("Command: " + command));
}
}
5. Private Methods in Interfaces:
Java 9 allows private methods in interfaces for code reuse among default and static methods.
Example: Private Methods in Interface
interface MyInterface {
default void publicMethod() {
System.out.println("Public Method");
privateMethod();
}
private void privateMethod() {
System.out.println("Private Method");
}
}
public class InterfaceExample implements MyInterface {
public static void main(String[] args) {
InterfaceExample example = new InterfaceExample();
example.publicMethod();
}
}
6. Try-With-Resources Enhancement:
Java 9 allows effectively final variables to be used in try-with-resources statements.
Example: Try-With-Resources Enhancement
public class TryWithResourcesExample {
public static void main(String[] args) {
Resource resource = new Resource();
// No need to declare resource variable outside try
try (resource) {
resource.doSomething();
} catch (Exception e) {
e.printStackTrace();
}
}
}
class Resource implements AutoCloseable {
public void doSomething() {
System.out.println("Doing something");
}
@Override
public void close() throws Exception {
System.out.println("Resource closed");
}
}
7. Diamond Operator Enhancement:
Java 9 allows the diamond operator (<>
) to be used with anonymous classes.
Example: Diamond Operator Enhancement
import java.util.*;
public class DiamondOperatorExample {
public static void main(String[] args) {
// Without diamond operator enhancement (Java 8)
List<String> list1 = new ArrayList<String>();
// With diamond operator enhancement (Java 9)
List<String> list2 = new ArrayList<>() {
{
add("Item1");
add("Item2");
}
};
System.out.println(list1);
System.out.println(list2);
}
}
8. Stream API Enhancements:
Java 9 introduced new methods in the Stream API for more concise and readable code.
Example: Stream API Enhancements
import java.util.List;
import java.util.stream.Collectors;
public class StreamApiEnhancementsExample {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", "Charlie");
// TakeWhile: Take elements while the condition is true
List<String> result1 = names.stream().takeWhile(name -> name.length() < 5).collect(Collectors.toList());
// DropWhile: Drop elements while the condition is true
List<String> result2 = names.stream().dropWhile(name -> name.length() < 5).collect(Collectors.toList());
System.out.println(result1); // Output: [Alice, Bob]
System.out.println(result2); // Output: [Charlie]
}
}
These are just some of the key features introduced in Java 9. Each feature is designed to improve the language's modularity, developer productivity, and code readability.
Subscribe to my newsletter
Read articles from Navnit Raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
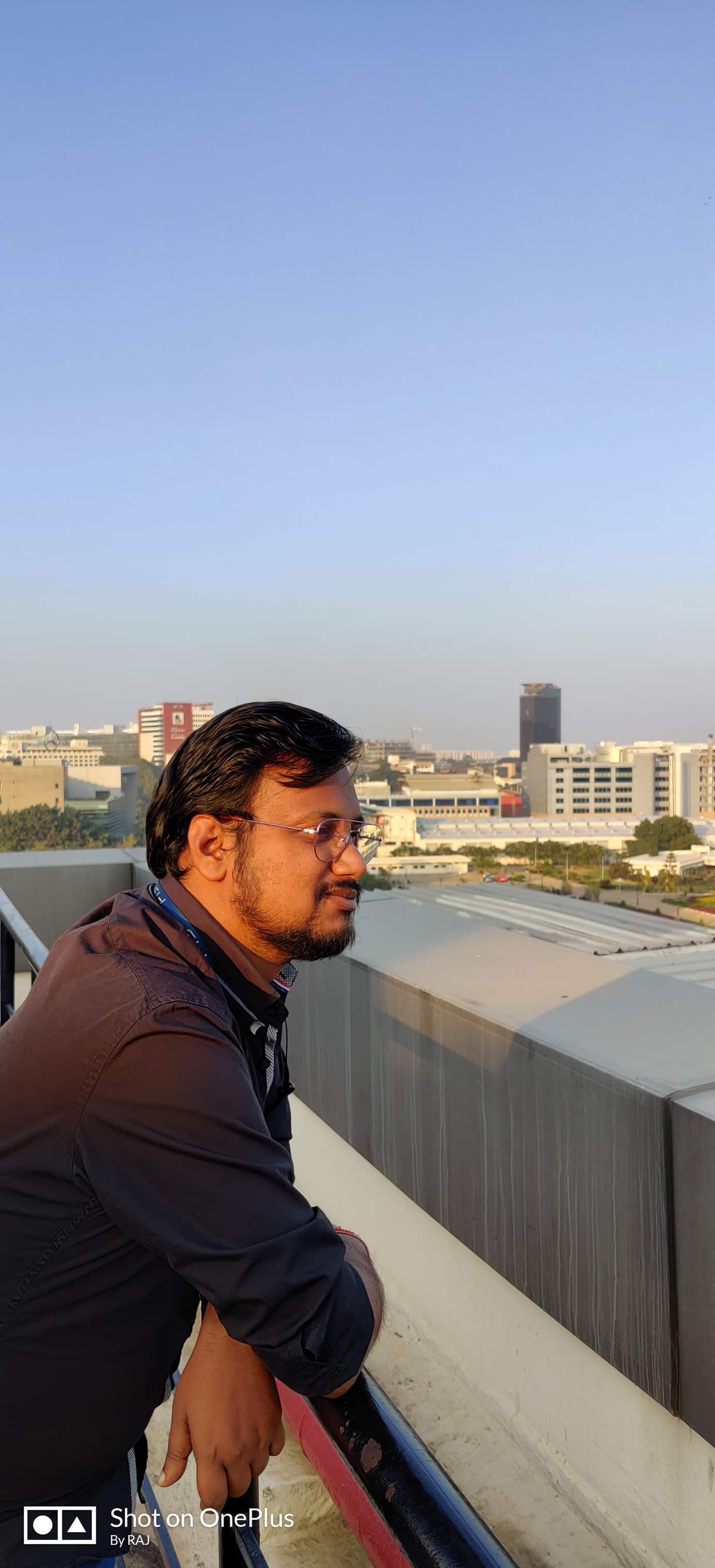