How to configure TSConfig Path Aliases?

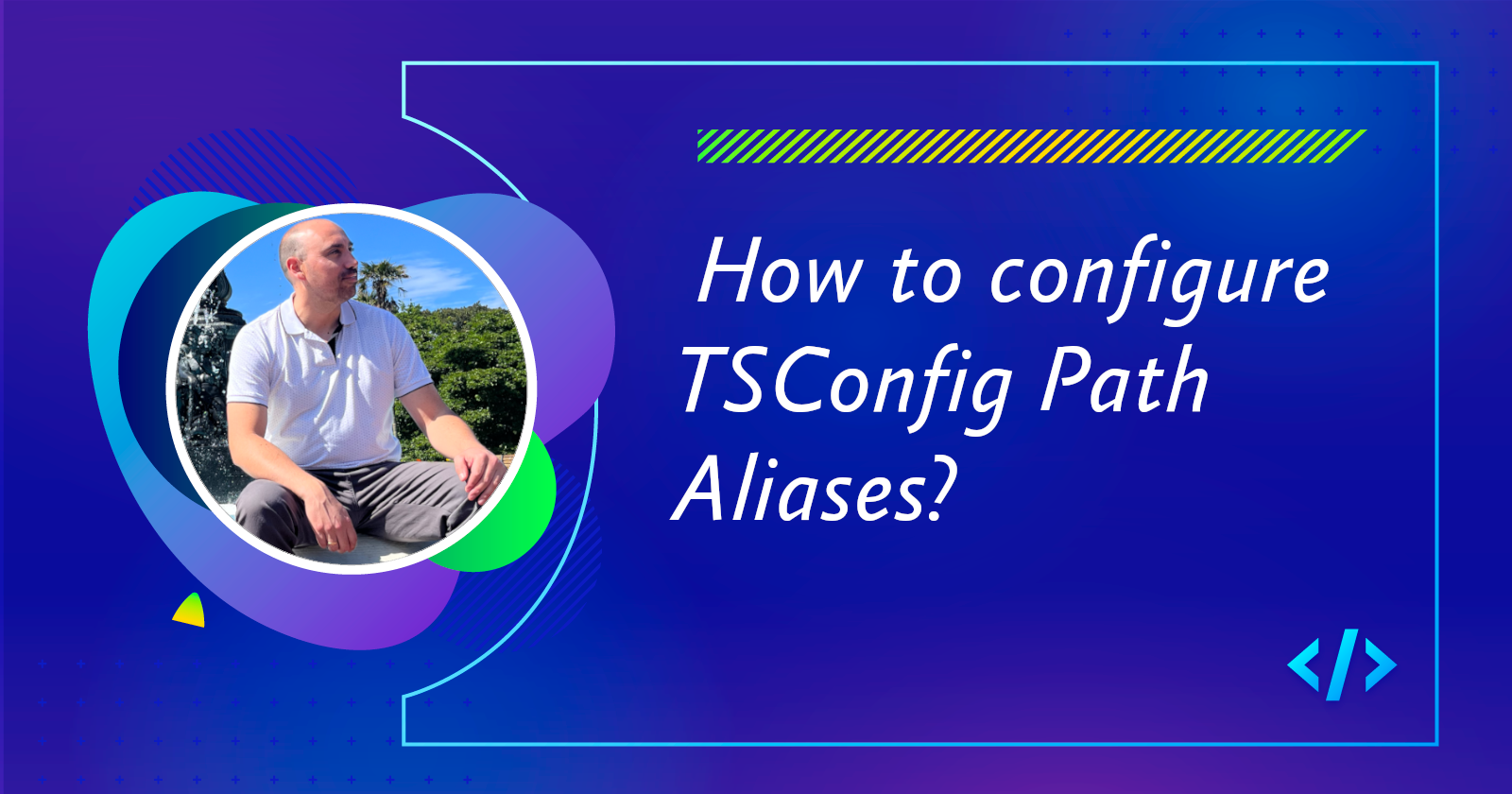
TSConfig path aliases in TypeScript allows us to define custom aliases for module imports in our project. This means we can create shorter and more meaningful names for our module imports, rather than using long and complex relative paths.
To set up path aliases, we need to update our tsconfig.json file to add the "paths" property:
{
"compilerOptions": {
...
"outDir": "./dist",
// Each path is relative to the baseUrl
"baseUrl": ".",
"paths": {
"@/controllers/*":[
"src/controllers/*"
]
}
}
}
In the previous configuration example, we have "@/controllers/" path alias which is an alias for the "src/controllers/" path. The asterisk (*) acts as a wildcard, allowing us to import any module within that folder using this alias.
Now, we can import modules using aliases instead of long relative paths:
//using paths
import { ExampleController } from "@/controllers/example.controller";
//without paths
import { ExampleController } from "../../controllers/example.controller";
Last but not least, to distribute our code we need to install tsc-alias package to map correctly the path aliases:
npm install -D tsc-alias
And also, update the scripts config section of the package.json file to add:
...
"scripts": {
"build": "tsc --build && tsc-alias",
},
...
build: Compile our typescript files and replace alias paths with relative paths after compilation.
Bonus
To recompile TypeScript code on the fly and reload the application whenever a file is changed install the following packages:
npm install -D ts-node-dev tsconfig-paths
And create a new command in your package.json:
{
"scripts": {
...
"start": "ts-node-dev --respawn -r tsconfig-paths/register ./src/app.ts",
},
Best Practices
When working with Path Aliases, it is important to follow a set of best practices to ensure clarity, maintainability, and flexibility:
Use meaningful alias names: Choose alias names that clearly represent the purpose of the path. Don't use names that are too general or vague as they could lead to mistakes or confusion.
Good alias names: @components, @images, @controllers, @views, @styles
Organize your aliases logically: Group related aliases together to make your project more organized and intuitive.
Keep aliases consistent: Ensure that all team members use the same set of aliases across the project to maintain codebase consistency.
Document it: Document the aliases and share them with your team to avoid confusion.
In conclusion, your code is easier to read and maintain when you use these aliases. In addition, if we need to change the folder structure of the project, we only need to update the "path aliases" in the tsconfig.json without modify any other import statements in the codebase.
See you in the next article.
Subscribe to my newsletter
Read articles from Max Martínez Cartagena directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Max Martínez Cartagena
Max Martínez Cartagena
I'm an enthusiastic Chilean software engineer in New Zeland. I mostly focus on the back-end of the systems. This is my site, Señor Developer, where I share my knowledge and experience.