50 Git interview questions along with their answers for DevOps positions:
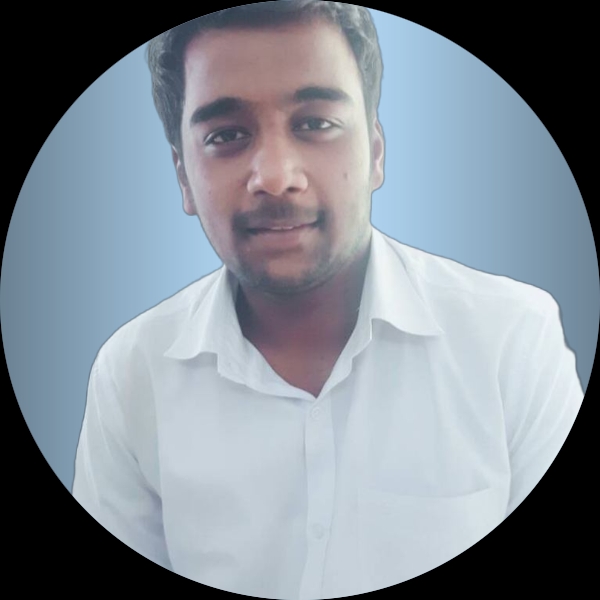

What is Git?
- Answer: Git is a distributed version control system used to track changes in files and coordinate work on those files among multiple people.
What is the difference between Git and SVN?
- Answer: Git is a distributed version control system, while SVN (Subversion) is a centralized version control system. In Git, every user has a local copy of the repository, allowing them to work offline and commit changes locally.
What is a repository in Git?
- Answer: A repository in Git is a data structure that stores metadata and objects representing a project. It contains all versions of the project's files and directories.
How do you create a new Git repository?
- Answer: You can create a new Git repository using the command
git init
followed by the project directory.
What is a commit in Git?
- Answer: A commit is a snapshot of changes made to a repository at a specific point in time. It includes a unique identifier, a commit message, and a pointer to the parent commit(s).
How do you commit changes in Git?
- Answer: To commit changes in Git, you use the command
git commit -m "commit message"
. This will record the changes in the local repository.
What is the purpose of the staging area (index) in Git?
- Answer: The staging area is where you prepare and review changes before committing them to the repository. It allows you to selectively include or exclude specific changes from a commit.
How do you add files to the staging area in Git?
- Answer: You can add files to the staging area using the command
git add filename
orgit add .
to add all modified files.
What is a branch in Git?
- Answer: A branch in Git is a separate line of development that allows you to work on features or bug fixes without affecting the main codebase.
How do you create a new branch in Git?
Answer: You can create a new branch using the command git checkout -b branch_name
.
How do you switch between branches in Git?
Answer: You can switch between branches using the command git checkout branch_name
.
What is a merge in Git?
Answer: A merge in Git combines the changes from one branch into another branch. It is used to integrate feature branches back into the main branch.
How do you merge branches in Git?
Answer: To merge branches in Git, you first switch to the target branch (git checkout target_branch
) and then use the command git merge source_branch
.
What is a conflict in Git?
Answer: A conflict in Git occurs when two branches have made changes to the same part of a file and Git is unable to automatically resolve the differences.
How do you resolve a merge conflict in Git?
Answer: To resolve a merge conflict, you need to manually edit the conflicting file(s), choose which changes to keep, and then commit the changes.
What is a remote repository in Git?
Answer: A remote repository in Git is a version of a project hosted on a server that allows collaboration between multiple contributors.
How do you clone a Git repository?
Answer: You can clone a Git repository using the command git clone repository_url
.
What is the difference between git pull
and git fetch
?
Answer: git pull
fetches and merges changes from a remote repository to the current branch, while git fetch
only downloads changes from the remote repository without merging them.
What is a tag in Git?
Answer: A tag in Git is a reference that points to a specific commit. It is used to mark specific points in history, such as release versions.
How do you create a tag in Git?
Answer: You can create a tag in Git using the command git tag tag_name
.
How do you push a tag to a remote repository?
Answer: You can push a tag to a remote repository using the command git push origin tag_name
.
What is a Git hook?
Answer: A Git hook is a script that Git executes before or after certain events, such as committing changes or merging branches.
What is Git bisect?
Answer: Git bisect is a command used for binary search of bugs. It helps you find the commit that introduced a bug.
What is Git rebase?
Answer: Git rebase is a command used to integrate changes from one branch into another by moving or combining commits.
What is the difference between a merge and a rebase in Git?
Answer: Merging creates a new commit that combines the changes from two branches, while rebasing moves or combines a sequence of commits to a new base commit.
How do you undo the last Git commit?
Answer: You can undo the last Git commit using the command git reset HEAD~1
.
What is Git cherry-pick?
Answer: Git cherry-pick is a command used to apply a specific commit from one branch to another.
What is Git stash?
Answer: Git stash is a command used to save changes that are not ready to be committed, allowing you to switch branches without losing your work.
How do you list all the branches in a Git repository?
Answer: You can list all branches in a Git repository using the command git branch
.
What is the purpose of the .gitignore
file?
Answer: The .gitignore
file is used to specify which files and directories Git should ignore, meaning they will not be tracked or committed to the repository.
How do you remove a file from Git without deleting it?
Answer: You can remove a file from Git without deleting it using the command git rm --cached filename
.
How do you rename a file in Git?
Answer: You can rename a file in Git using the command git mv old_filename new_filename
.
What is a Git submodule?
Answer: A Git submodule is a separate Git repository embedded within another Git repository. It allows you to include other projects as dependencies.
How do you update a Git submodule?
Answer: You can update a Git submodule using the command git submodule update --remote
.
What is Git flow?
Answer: Git flow is a branching model that defines a consistent way to organize branches and merges in a Git repository.
What is GitLab/GitHub Actions?
Answer: GitLab/GitHub Actions are Continuous Integration/Continuous Deployment (CI/CD) services provided by GitLab/GitHub for automating tasks like testing and deploying code.
How do you revert a commit in Git?
Answer: You can revert a commit in Git using the command git revert commit_sha
.
What is the difference between git rebase
and git merge
?
Answer: git rebase
integrates changes from one branch onto another by moving or combining commits, resulting in a linear history. git merge
creates a new commit that combines changes from two branches, resulting in a branch-like history.
How do you squash multiple commits into one?
Answer: You can squash multiple commits into one using the interactive rebase command: git rebase -i HEAD~n
, where n
is the number of commits you want to squash.
How do you amend the last commit message in Git?
Answer: You can amend the last commit message using the command git commit --amend
.
What is Git LFS (Large File Storage)?
Answer: Git LFS is an extension to Git that allows the versioning of large files by storing them on a remote server, while keeping lightweight references in the repository.
How do you configure Git user information?
Answer: You can configure Git user information using the commands: git config --global
user.name
"Your Name" git config --global
user.email
"
your.email@example.com
"
How do you show the commit history in Git?
Answer: You can show the commit history using the command git log
.
What is Git Cherry-Pick and when would you use it?
Answer: Git Cherry-Pick is a command used to apply a specific commit from one branch to another. It is useful when you want to apply a specific fix or feature to a different branch without merging the entire branch.
How do you create and apply patches in Git?
Answer: To create a patch, use the command git format-patch -1 <commit>
. To apply a patch, use the command git am <patch>
.
How do you view the changes between two Git commits?
Answer: You can view the changes between two Git commits using the command git diff commit1 commit2
.
What is Git reflog?
Answer: Git reflog is a log of all reference updates in a repository. It allows you to recover lost commits or branches.
What is Git blame?
Answer: Git blame is a command that shows information about who last modified each line of a file, and in which commit.
How do you delete a branch in Git?
Answer: You can delete a branch in Git using the command git branch -d branch_name
.
How do you force push in Git?
Answer: You can force push in Git using the command git push -f origin branch_name
.
Remember, these are just interview questions, and in a real-world scenario, understanding the concepts and being able to apply them is crucial.
Subscribe to my newsletter
Read articles from Shrishail Patil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
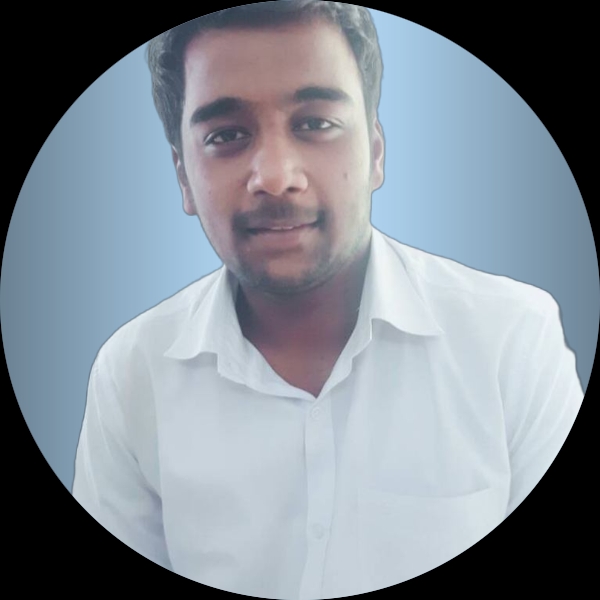
Shrishail Patil
Shrishail Patil
DevOps Enthusiast | Cloud & DevOps | Kubernetes | AWS | Ansible | GIT | Terraform | Gitlab | Docker | Python | Linux | Software Testing | Data Engineering