PHP Directory Functions
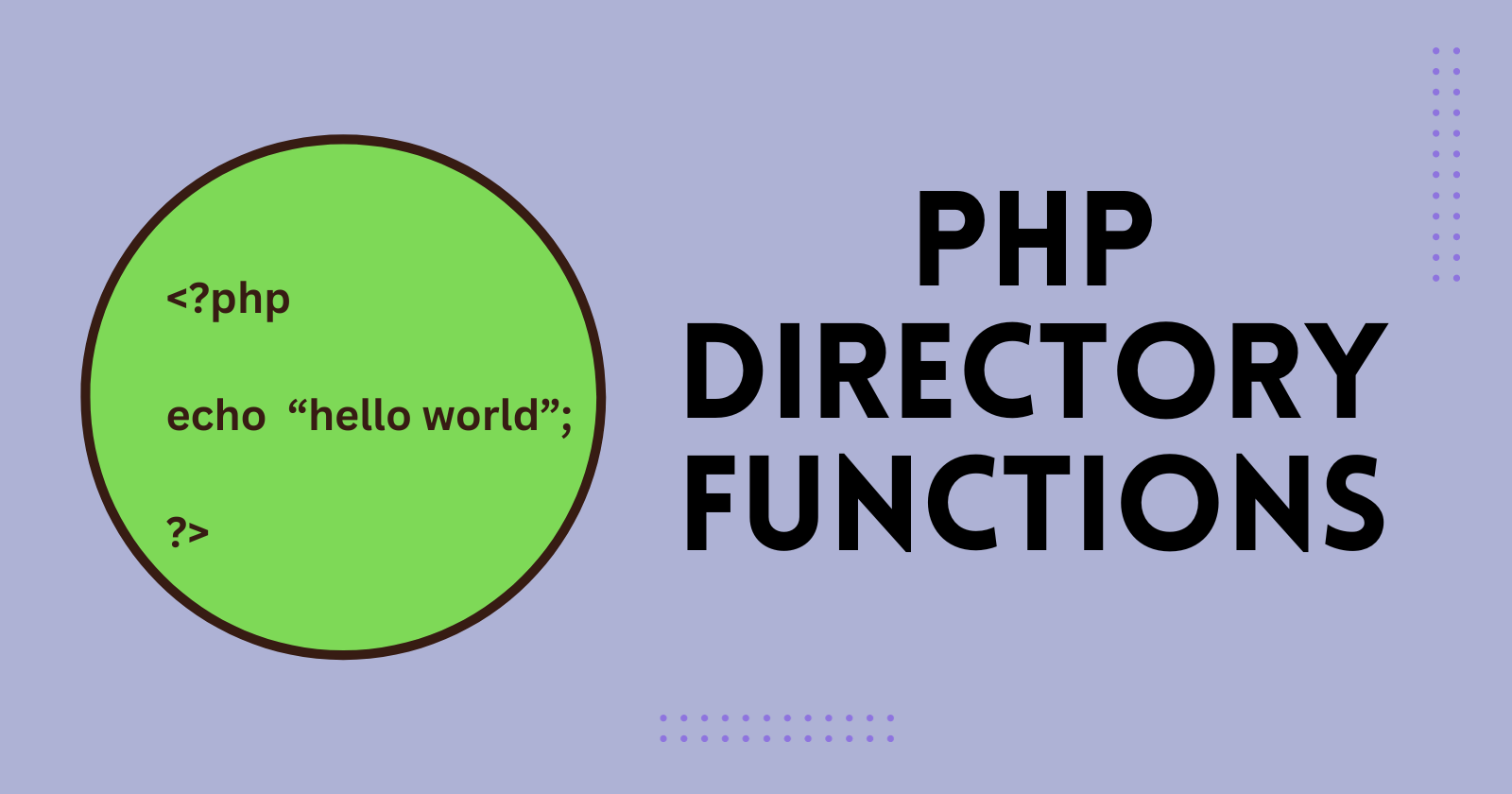
The directory functions allow you to retrieve information about directories and their contents. In this blog, you get an overall discussion about it. This is a core concept of php. Official Documentation.
When you work with files and directories in php you need to first open your directory then read it and lastly close your directory after execution. PHP directory functions do the same.
getcwd()
- function returns the current working directory.echo getcwd(); // Return your directory. exmple: C:/xampp/php
dir()
- function returns an instance of the Directory class.$d = dir("/xampp/php"); echo "Handle: " . $d->handle . "\n"; echo "Path: " . $d->path . "\n"; while (false !== ($entry = $d->read())) { echo "filename: " . $entry."\n"; } $d->close(); // Return like: Handle: Resource id #2 Path: /xampp/php filename: . filename: .. filename: index2.php filename: myFile.docx filename: new.txt filename: catalog.php
opendir()
function opens a directory handle.readdir()
function returns the name of the next entry in a directory. The entries are returned in the order in which they are stored by the filesystem.closedir()
Close directory handle. Closes the directory stream indicated bydir_handle
. The stream must have previously been opened byopendir().
// Basic structure of these functions. $dir = "/images/"; // Open a directory, and read its contents if (is_dir($dir)){ if ($dh = opendir($dir)){ while (($file = readdir($dh)) !== false){ echo "filename:" . $file . "<br>"; } closedir($dh); } }
scandir() -
Returns an array of files and directories from thedirectory
.$dir = '/tmp'; $files1 = scandir($dir); $files2 = scandir($dir, SCANDIR_SORT_DESCENDING); print_r($files1); print_r($files2); // Output Array ( [0] => . [1] => .. [2] => bar.php [3] => foo.txt [4] => somedir ) Array ( [0] => somedir [1] => foo.txt [2] => bar.php [3] => .. [4] => . )
This article is associated with PHP File Handling article. Happy learning.
Subscribe to my newsletter
Read articles from Md. Moinul Hossain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Md. Moinul Hossain
Md. Moinul Hossain
A Passionate web Application developer and DevOps enthusiast from Bangladesh.