"Optimizing React Performance: A Deep Dive into the Differences Between useMemo and React.memo"
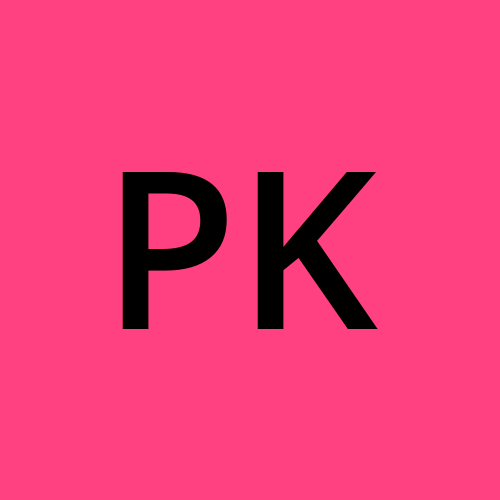
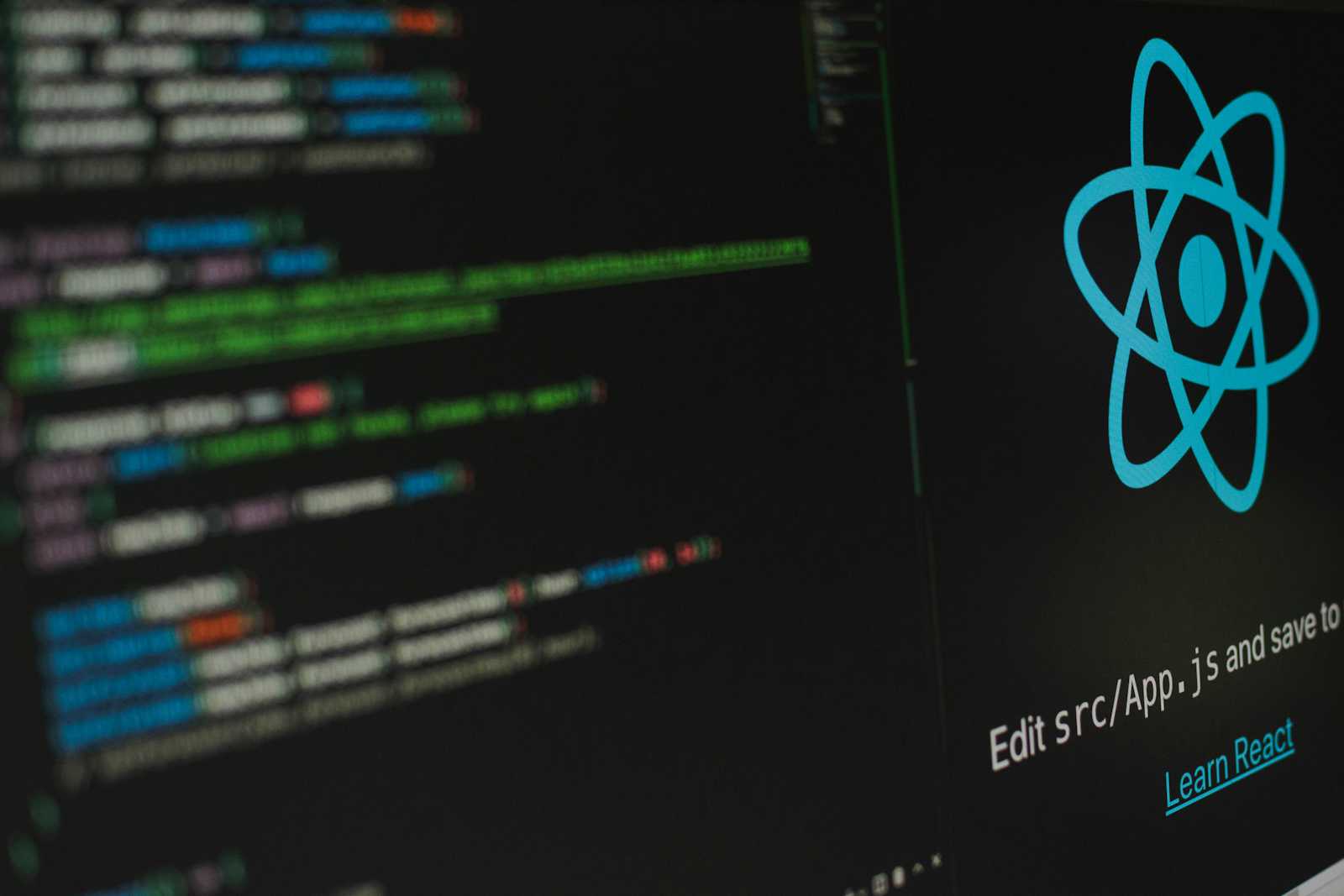
React, a popular JavaScript library for building user interfaces, provides developers with a variety of tools to optimize performance. Two such tools are useMemo
and React.memo
. While they share a similar goal of improving performance by memoizing values, they serve different purposes and are applied in distinct scenarios. In this article, we will delve into the differences between useMemo
and React.memo
in React.
Understanding useMemo
useMemo
is a React hook designed to memoize the result of expensive computations. It is particularly useful when dealing with calculations or operations that are computationally intensive and do not need to be recalculated on every render. By memoizing the result, useMemo
helps prevent unnecessary recalculations, thus optimizing the overall performance of the React application.
Here's a basic example of useMemo
in action:
jsxCopy codeimport React, { useMemo } from 'react';
const MyComponent = ({ data }) => {
const processedData = useMemo(() => {
// Expensive computation based on data
return processData(data);
}, [data]);
return <div>{processedData}</div>;
};
In this example, useMemo
takes a function as its first argument, which represents the expensive computation, and an array of dependencies as its second argument. The memoized result is recalculated only when any of the dependencies in the array change.
Exploring React.memo
On the other hand, React.memo
is a higher-order component (HOC) that is used to memoize the rendering of a functional component. It is particularly helpful when dealing with components that receive the same props but do not need to re-render on every parent render.
Consider the following example:
jsxCopy codeimport React from 'react';
const MyComponent = React.memo(({ data }) => {
// Component logic using data
return <div>{data}</div>;
});
In this case, React.memo
wraps the functional component, and it automatically prevents re-rendering if the data
prop remains unchanged. It performs a shallow comparison of props to determine whether the component should update.
Key Differences
Now that we have a basic understanding of both useMemo
and React.memo
, let's explore the key differences between them:
1. Application
useMemo
is applied within functional components to memoize the result of an expensive computation. It focuses on optimizing the performance of calculations within the component itself.React.memo
is applied to functional components to memoize the rendering of the component based on prop changes. It optimizes the rendering process by preventing unnecessary renders when props remain unchanged.
2. Usage
useMemo
is used inside the body of a functional component and takes a function as its argument. It returns the memoized result of the computation.React.memo
is used as a higher-order component that wraps around a functional component. It automatically memoizes the rendering of the component based on prop changes.
3. Dependencies
useMemo
requires an array of dependencies as its second argument. It recalculates the memoized value only when the dependencies change.React.memo
performs a shallow comparison of props by default. It re-renders the component only if the props change, similar to theshouldComponentUpdate
lifecycle method in class components.
4. Use Cases
useMemo
is beneficial when dealing with expensive calculations or operations within a component that depend on specific values.React.memo
is useful when optimizing the rendering of a component that receives the same props but does not need to re-render on every parent render.
Conclusion
In conclusion, both useMemo
and React.memo
play crucial roles in optimizing the performance of React applications, but they are designed for different purposes. While useMemo
focuses on memoizing the result of expensive computations within functional components, React.memo
is dedicated to memoizing the rendering of functional components based on prop changes.
Understanding when and how to use each of these tools is essential for React developers striving to build efficient and performant applications. By leveraging useMemo
and React.memo
appropriately, developers can strike a balance between computation optimization and rendering efficiency in their React projects.
Subscribe to my newsletter
Read articles from Pavan Kulkarni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
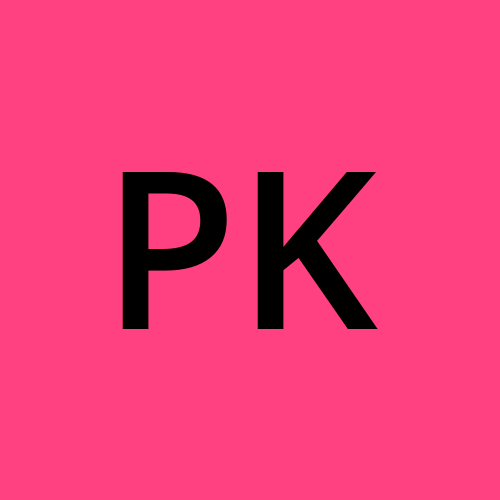
Pavan Kulkarni
Pavan Kulkarni
Devops Engineer with five years experience in managing CI/CD Pipelines, Bring cost optimization and efficiency in utilization of Cloud resources