Array Questions(Program)
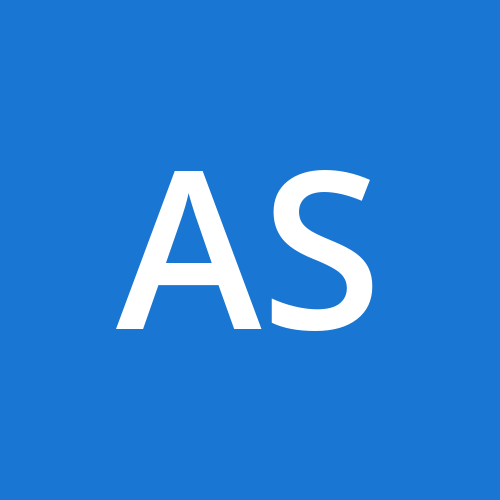
Basic Level Questions
Binary Search
Given a sorted array of size N and an integer K, find the position(0-based indexing) at which K is present in the array using binary search.
Input:
N = 5
arr[] = {1 2 3 4 5}
K = 4
Output: 3
Explanation: 4 appears at index 3.
int binarysearch(int arr[], int n, int k) {
// code here
int i =0, j = n-1;
int m;
while(i<=j)
{
m=(i+j)/2;
if(arr[m] == k)
return m;
else if(arr[m] > k)
j= m-1;
else
i = m+1;
}
return -1;
}
Power of 2
Given a non-negative integer N. The task is to check if N is a power of 2. More formally, check if N can be expressed as 2x for some integer x. Return true if N is power of 2 else return false.
bool isPowerofTwo(long long n){
if (n == 0)
return false;
if(n == 1)
return true;
while(n != 1)
{
if(n%2!=0)
return false;
n = n / 2;
}
return true;
}
Union of two arrays
Given two arrays a[] and b[] of size n and m respectively. The task is to find the number of elements in the union between these two arrays.
Union of the two arrays can be defined as the set containing distinct elements from both the arrays. If there are repetitions, then only one occurrence of element should be printed in the union.
int doUnion(int a[], int n, int b[], int m) {
//code here
set<int> val;
for(int i=0;i<n;i++)
val.insert(a[i]);
for(int i=0;i<m;i++)
val.insert(b[i]);
return val.size();
}
Reverse a String
You are given a string s. You need to reverse the string.
string reverseWord(string str){
for(int i=0, j=str.size()-1; i <j ; i++, j--)
{
char ch = str[i];
str[i] = str[j];
str[j] = ch;
}
return str;
}
Check if two arrays are equal or not
Given two arrays A and B of equal size N, the task is to find if given arrays are equal or not. Two arrays are said to be equal if both of them contain same set of elements, arrangements (or permutation) of elements may be different though.
Note : If there are repetitions, then counts of repeated elements must also be same for two array to be equal.
bool check(vector<ll> A, vector<ll> B, int N) {
//code here
if (A.size()== B.size())
{
sort(A.begin(), A.end());
sort(B.begin(), B.end());
for(int i=0;i<A.size();i++)
{
if(A.at(i)!= B.at(i))
{
return 0;
}
}
return 1;
}
return 0;
}
Reverse array in groups
Given an array arr[] of positive integers of size N. Reverse every sub-array group of size K.
Note: If at any instance, there are no more subarrays of size greater than or equal to K, then reverse the last subarray (irrespective of its size). You shouldn't return any array, modify the given array in-place.
void reverseInGroups(vector<long long>& arr, int n, int k){
// code here
int i;
for(int i =0;i<n;i+=k)
{
if(i+k>n)
reverse(arr.begin()+i, arr.end());
else
reverse(arr.begin()+i, arr.begin()+i+k);
}
}
Cyclically rotate an array by one
Given an array, rotate the array by one position in clock-wise direction.
void rotate(int arr[], int n)
{
int ele = arr[n-1];
for(int i=n-1;i>0;i--)
arr[i] =arr[i-1];
arr[0]= ele;
}
Subscribe to my newsletter
Read articles from Akshima Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
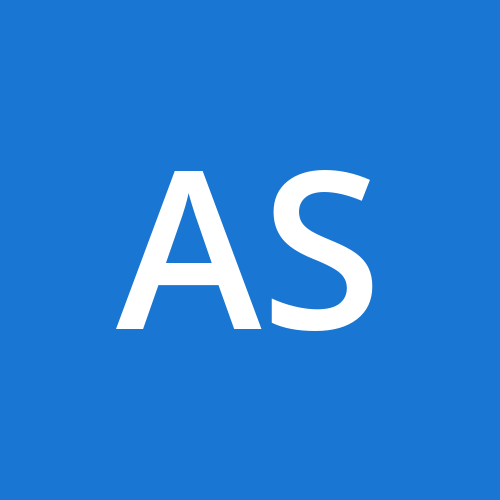