What is the difference between Java and JavaScript?

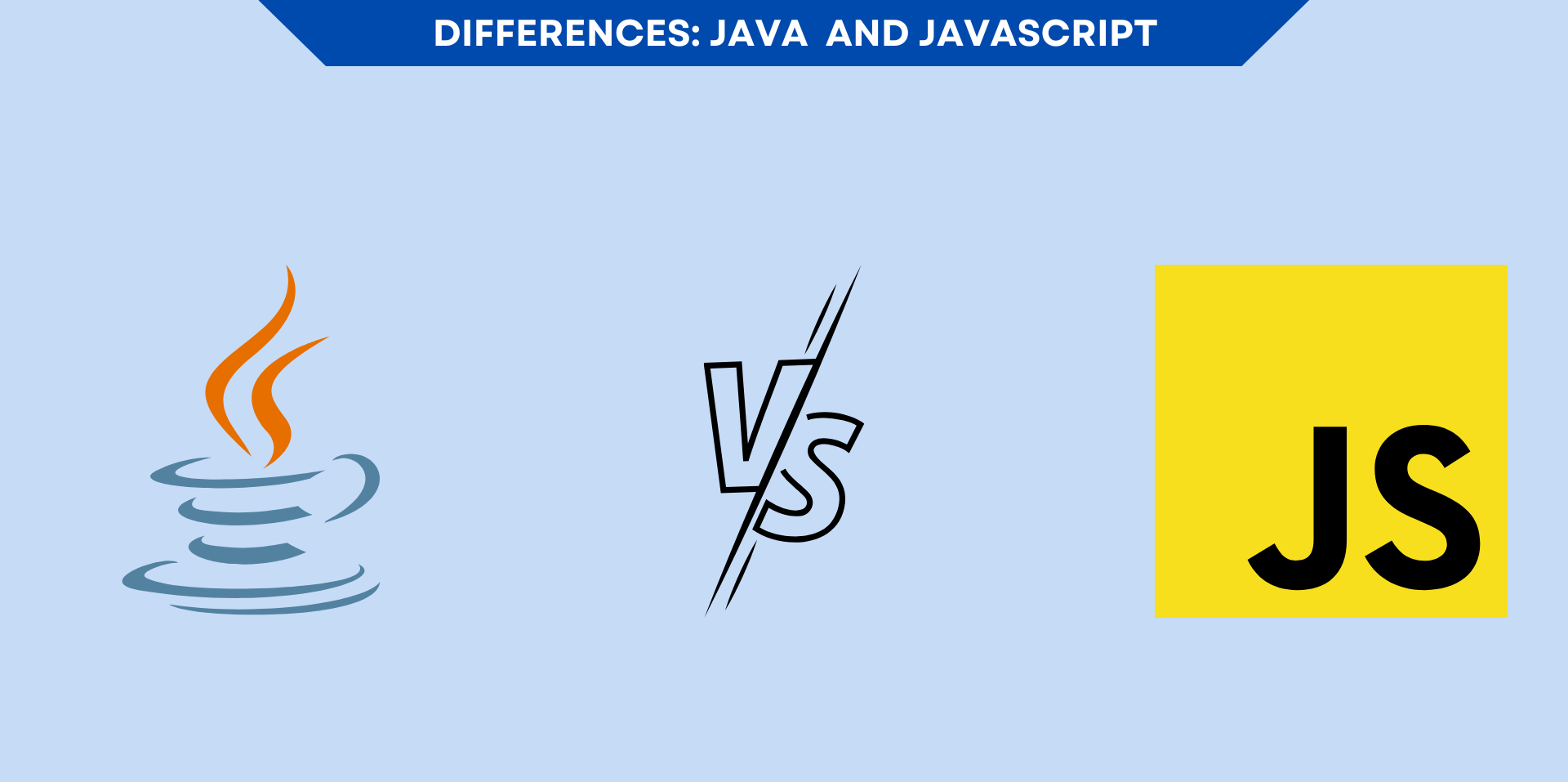
Java and JavaScript are two different programming languages that serve different purposes and are used in different contexts. Here are some key differences between Java and JavaScript:
Purpose:
Java: Java is a general-purpose, object-oriented programming language that can be used for a wide range of applications, from desktop to mobile to enterprise-level software.
JavaScript: JavaScript is a scripting language primarily used for front-end web development. It enables interactive and dynamic behavior in web browsers.
Platform:
Java: Java is a standalone programming language that can be used to develop applications that run on a variety of platforms, thanks to its "Write Once, Run Anywhere" (WORA) philosophy. Java applications are typically compiled into bytecode and run on the Java Virtual Machine (JVM).
JavaScript: JavaScript is primarily used in web browsers to make web pages interactive. It is a client-side scripting language that runs directly in the browser.
Execution:
Java: Java code is typically compiled into bytecode, which is then executed on the JVM. This provides a level of abstraction from the underlying hardware and operating system.
JavaScript: JavaScript code is executed by the browser's JavaScript engine directly, without the need for compilation. Modern browsers have their own JavaScript engines, such as V8 in Chrome and SpiderMonkey in Firefox.
Object Model:
Java: Java uses a class-based object-oriented programming model. Objects are instances of classes, and the language supports concepts like inheritance and polymorphism.
JavaScript: JavaScript uses a prototype-based object model. Objects can inherit properties directly from other objects, and there is no strict concept of classes in the traditional sense.
Concurrency:
Java: Java has built-in support for multithreading and concurrency. This makes it suitable for developing applications that require parallel processing.
JavaScript: JavaScript is single-threaded, but it supports asynchronous programming through features like callbacks, promises, and async/await. This is crucial for handling events and performing non-blocking operations in the browser.
Usage:
Java: Java is used in a wide range of applications, including enterprise-level server-side applications, Android mobile app development, and large-scale distributed systems.
JavaScript: JavaScript is primarily used for front-end web development to enhance the interactivity and user experience of websites. It is also used on the server side (Node.js) for building scalable and real-time applications.
Language Origin:
Java: Developed by Sun Microsystems in the mid-1990s. It follows the "Write Once, Run Anywhere" philosophy.
JavaScript: Created by Netscape in the mid-1990s for client-side scripting in web browsers. It has since evolved and is now supported by all major browsers.
Syntax Style:
Java: Has a syntax similar to languages like C++ and C#. It uses braces
{}
for block statements and requires a semicolon;
to terminate statements.JavaScript: Has a syntax influenced by languages like Java and C. It uses curly braces
{}
for blocks but doesn't always require semicolons (they are optional, though recommended).
Java Example:
// Java Example - Hello World
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
This is a classic "Hello, World!" example in Java. The program defines a class named HelloWorld
with a main
method. When executed, it prints the string "Hello, World!" to the console.
JavaScript Example:
// JavaScript Example - Hello World
console.log("Hello, World!");
In JavaScript, the same "Hello, World!" example is much simpler. This script uses the console.log
function to output the string "Hello, World!" to the browser's console. This could be part of an HTML file or executed in a browser's developer console.
It's important to note that the environments in which these examples run are different. The Java example would typically be compiled and run on the Java Virtual Machine (JVM), while the JavaScript example is executed directly by a JavaScript engine in a web browser or a server-side environment like Node.js.
Subscribe to my newsletter
Read articles from Bharat Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bharat Kumar
Bharat Kumar
I am a frontend developer.