2nd Attempt DAY 1
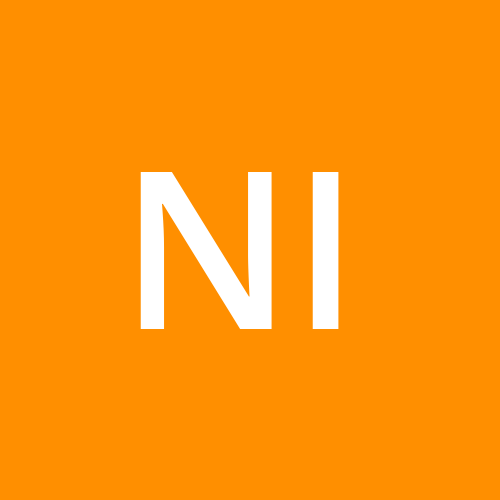
Problem Statement (string : Largest number with given sum) easy question.
Geek lost the password of his super locker. He remembers the number of digits N as well as the sum S of all the digits of his password. He know that his password is the largest number of N digits that can be made with given sum S. As he is busy doing his homework, help him retrieving his password.
Input:
N = 5, S = 12
Output:
93000
Explanation:
Sum of elements is 12. Largest possible
5 digit number is 93000 with sum 12.
we have to write a function that returns string which is a number.
Approach is like for every digit we check all 10 number from 9 to 0 while comparing the sum.
code
string largestNumber(int n, int sum)
{
string ans="";
while(n--)
{
if(sum==0)
{
ans=ans+to_string(0);
continue;
}
for(int i=9;i>=0;i--)
{
if(sum>=i)
{
ans=ans+to_string(i);
sum=sum-i;
break;
}
}
}
return (sum==0)?ans:"-1";
}
Problem Statement : String's Count(easy + important)
Given a length n, count the number of strings of length n that can be made using a, b and c with at-most one b and two c allowed.
Input: n = 1
Output: 3
Explanation: Possible strings are: "a",
"b" and "c"
countA
: Represents the count of strings with only 'a' at each position (1 possibility).countB
: Represents the count of strings with only 'b' at each position (n possibilities).countC
: Represents the count of strings with only 'c' at each position (n possibilities).countAB
: Represents the count of strings with two distinct characters, considering 'a' and 'b' (n * (n - 1) possibilities).countAC
: Represents the count of strings with two distinct characters, considering 'a' and 'c' (countAB / 2 possibilities).countBC
: Represents the count of strings with two distinct characters, considering 'b' and 'c' (countAC * (n - 2) possibilities).total
: Sum of all the counts, representing the total number of valid strings of lengthn
with at most one 'b' and two 'c'.
long long int countStr(long long int n) {
const long long res = 2 * n + (3 * (n - 1) * n) / 2 + (n * (n - 1) * (n - 2)) / 2 + 1;
return res;
}
PODT Problem Statement : Sequence of Sequence(DP medium problem)
Given two integers m and n, try making a special sequence of numbers seq of length n such that
seqi+1 >= 2*seqi
seqi \> 0
seqi <= m
Your task is to determine total number of such special sequences possible.
Input:
m = 10
n = 4
Output:
4
Explaination:
There should be n elements and
value of last element should be at-most m.
The sequences are {1, 2, 4, 8}, {1, 2, 4, 9},
{1, 2, 4, 10}, {1, 2, 5, 10}.
int numberSequence(int m, int n){
if(m<n)
return 0;
if(n==0)
return 1;
return numberSequence(m/2,n-1) + numberSequence(m-1,n);
}
Finally outdoor activity was running 2 km .
Subscribe to my newsletter
Read articles from Nitishkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
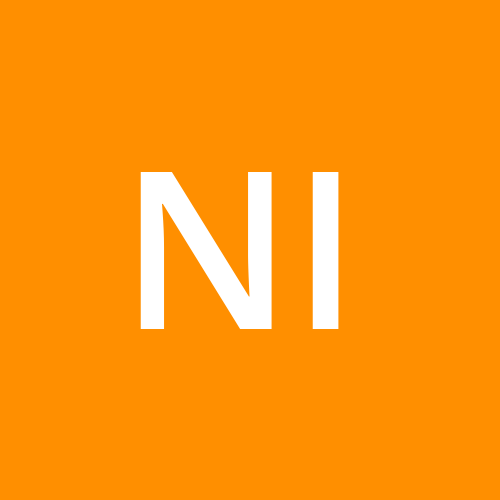