GitHub CICD Create_EC2_via_Terraform
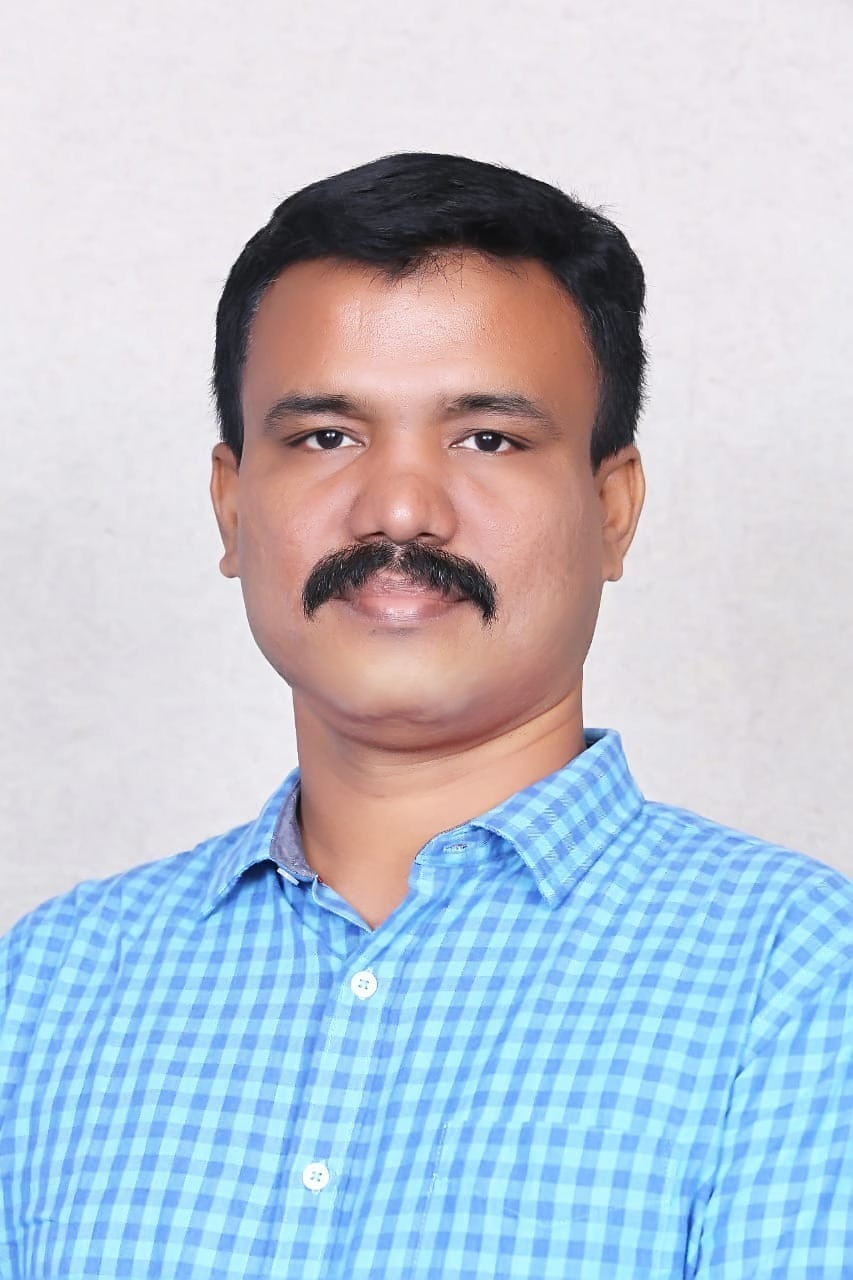
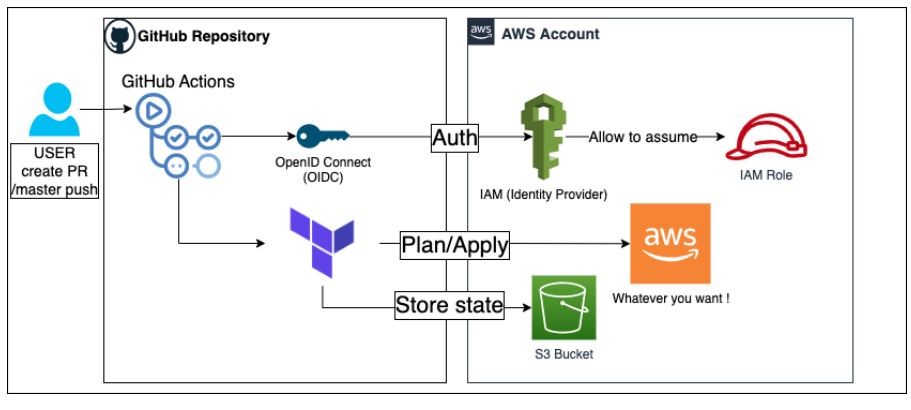
Step 1: Local SSH Pub Key Generation and GitHub Setup
1.1. Open a terminal and generate a new SSH key pair:
bashCopy codessh-keygen -t rsa -b 4096 -C "your_email@example.com"
1.2. Copy the public key to the clipboard:
cat ~/.ssh/id_rsa.pub | pbcopy
1.3. Go to GitHub, navigate to "Settings" > "SSH and GPG keys" > "New SSH key", and paste the copied key.
Step 2: S3 Bucket Creation for Terraform Backend
2.1. Open the AWS Management Console and navigate to S3.
2.2. Create a new S3 bucket:
aws s3api create-bucket --bucket my-terraform-backend-bucket --region us-east-1
Step 3: IAM Role Creation for GitHub Actions
3.1. Create a new IAM role for GitHub Actions with the necessary permissions. Use AWS CLI or AWS Console.
Step 4: GitHub Repository and Local Terraform Setup
4.1. Create a new GitHub repository and clone it to your local machine:
git clone https://github.com/suresh-subramanian2013/GitHub-CICD-Creating-EC2-Instances-with-Terraform.git
4.2. Create an ec2.tf
file with your Terraform configuration.
provider "aws" {
region = var.region
}
resource "aws_instance" "myec2" {
ami = "ami-0cff7528ff583bf9a"
instance_type = "t2.micro"
}
terraform {
backend "s3" {}
}
4.3. Push the changes to GitHub:
bashCopy codegit add .
git commit -m "Initial Terraform configuration"
git push origin main
Step 5: GitHub Action YAML Files
5.1. In your GitHub repository, create a directory named .github/workflows
.
5.2. Inside this directory, create YAML files for Apply and Destroy workflows:
name: 'Terraform'
on:
push:
branches:
- main
jobs:
terraform:
name: 'Terraform'
runs-on: ubuntu-latest
permissions:
id-token: write
contents: read
steps:
- name: Checkout
uses: actions/checkout@v3
- name: Configure AWS Credentials
uses: aws-actions/configure-aws-credentials@v1
with:
aws-region: us-east-2
role-to-assume: arn:aws:iam::xxxxxxx:role/github-action-role
# role-session-name: GithubActionsSession
- run: aws sts get-caller-identity
- name: Terraform Init
run: terraform init -backend-config="xxxxxxxxxxxxxx" -backend-config="key=${{ github.repository }}/terraform.tfstate" -backend-config="region=us-east-2"
- name: Terraform Validate
run: terraform validate
- name: terraform plan
run: terraform plan
- name: Terraform Apply
if: github.ref == 'refs/heads/main' && github.event_name == 'push'
run: terraform apply -auto-approve -input=true
Step 6: Local Code Update and Push
6.1. Modify your ec2.tf
file to change the instance type.
provider "aws" {
region = var.region
}
resource "aws_instance" "myec2" {
ami = "ami-0cff7528ff583bf9a"
instance_type = "t2.large"
}
terraform {
backend "s3" {}
}
6.2. Push the changes to GitHub:
bashCopy codegit add .
git commit -m "Update instance type"
git push origin main
Step 7: Destroy from GitHub Actions Workflow Trigger
7.1. Create a pull request to trigger the Destroy workflow.
name: Terraform Destroy
on:
workflow_dispatch:
jobs:
tf-destroy:
runs-on: ubuntu-latest
permissions:
id-token: write
contents: read
steps:
- name: Checkout
uses: actions/checkout@v2
- name: Configure AWS Credentials
uses: aws-actions/configure-aws-credentials@v1
with:
aws-region: us-east-2
role-to-assume: arn:aws:iam::xxxxxxx:role/github-action-role
# role-session-name: GithubActionsSession
- run: aws sts get-caller-identity
- name: Terraform Init
id: init
run: terraform init -backend-config="bucket=xxxxxxxxxx" -backend-config="key=${{ github.repository }}/terraform.tfstate" -backend-config="region=us-east-2"
- name: Show Destroy plan
run: terraform plan -destroy
continue-on-error: true
- name: Terraform destroy
id: destroy
run: terraform destroy -auto-approve
7.2. Once the pull request is merged or closed, the Destroy workflow will automatically execute, tearing down the resources.
Now, you have a detailed guide with hands-on commands for setting up GitHub and Youtube https://youtu.be/KsOV3GfJs5s .
I'm glad to help, but I don't have the ability to follow blogs, subscribe to channels, or connect on LinkedIn https://www.linkedin.com/in/suresh-kumar-s-profile/.
However, if you have any more questions or need assistance with anything else, feel free to ask!
Subscribe to my newsletter
Read articles from Suresh Kumar S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
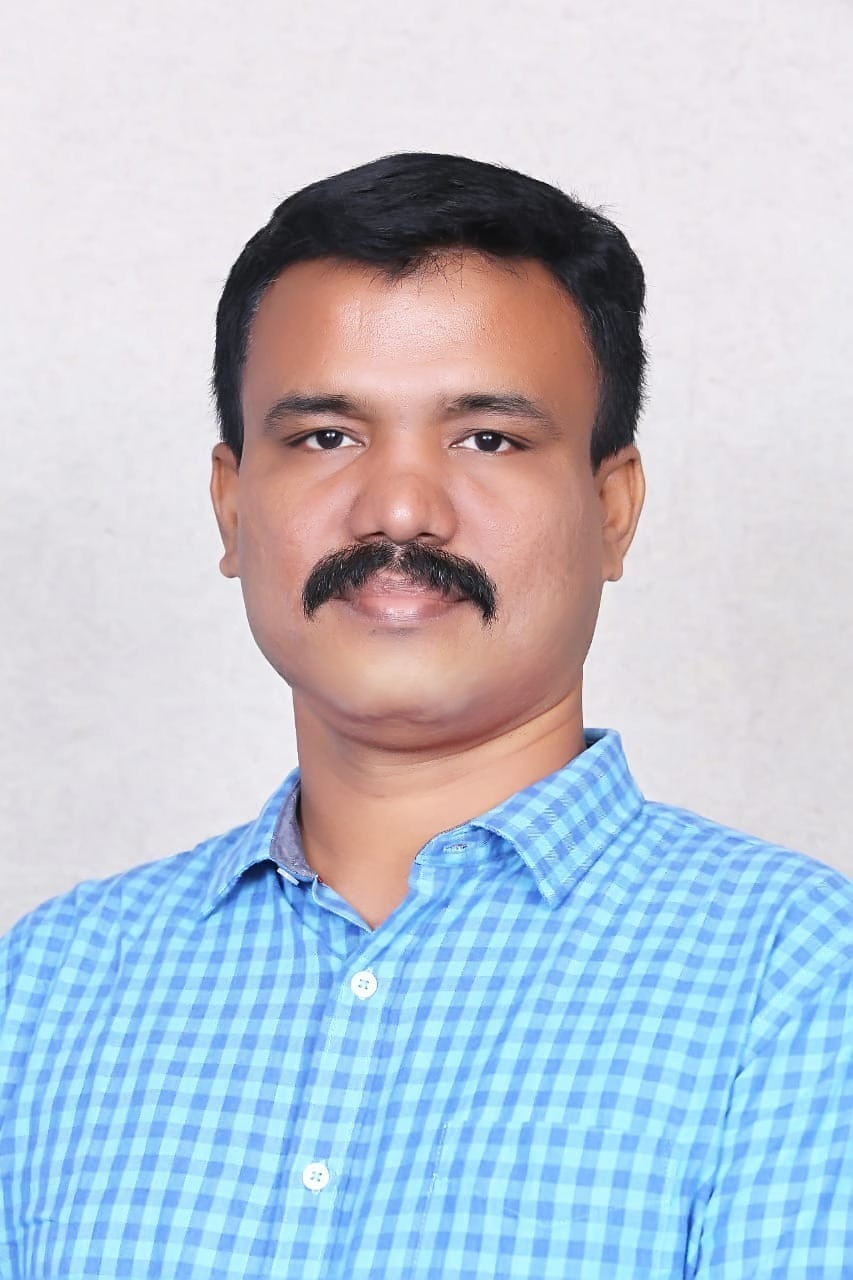
Suresh Kumar S
Suresh Kumar S
Experienced AWS DevOps Engineer with 9+ years in IT, specializing in AWS for over 5 years. Holder of 5 AWS certifications, adept at optimizing infrastructure and driving efficiency.