Creating a Replica of "cout" & "cin"
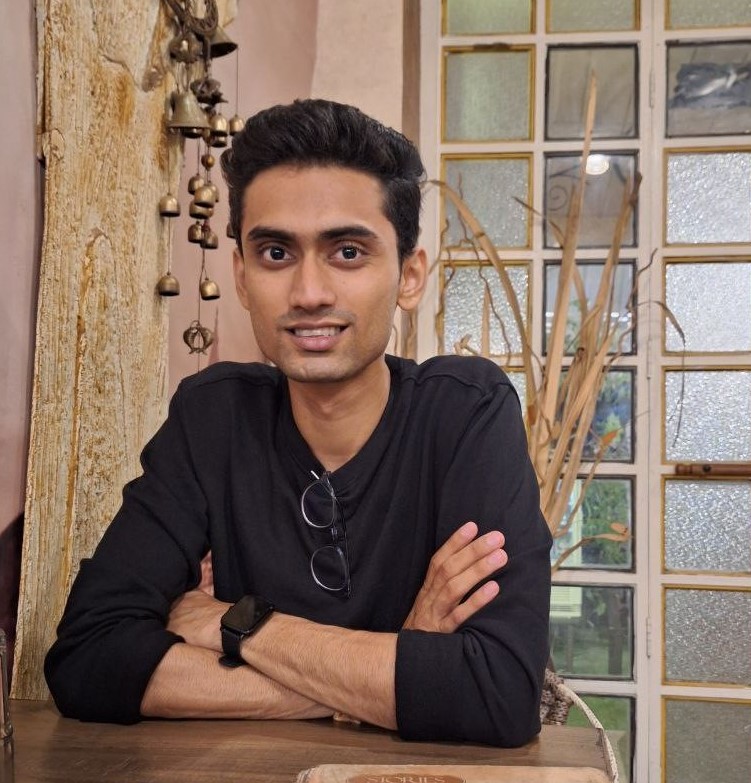
3 min read
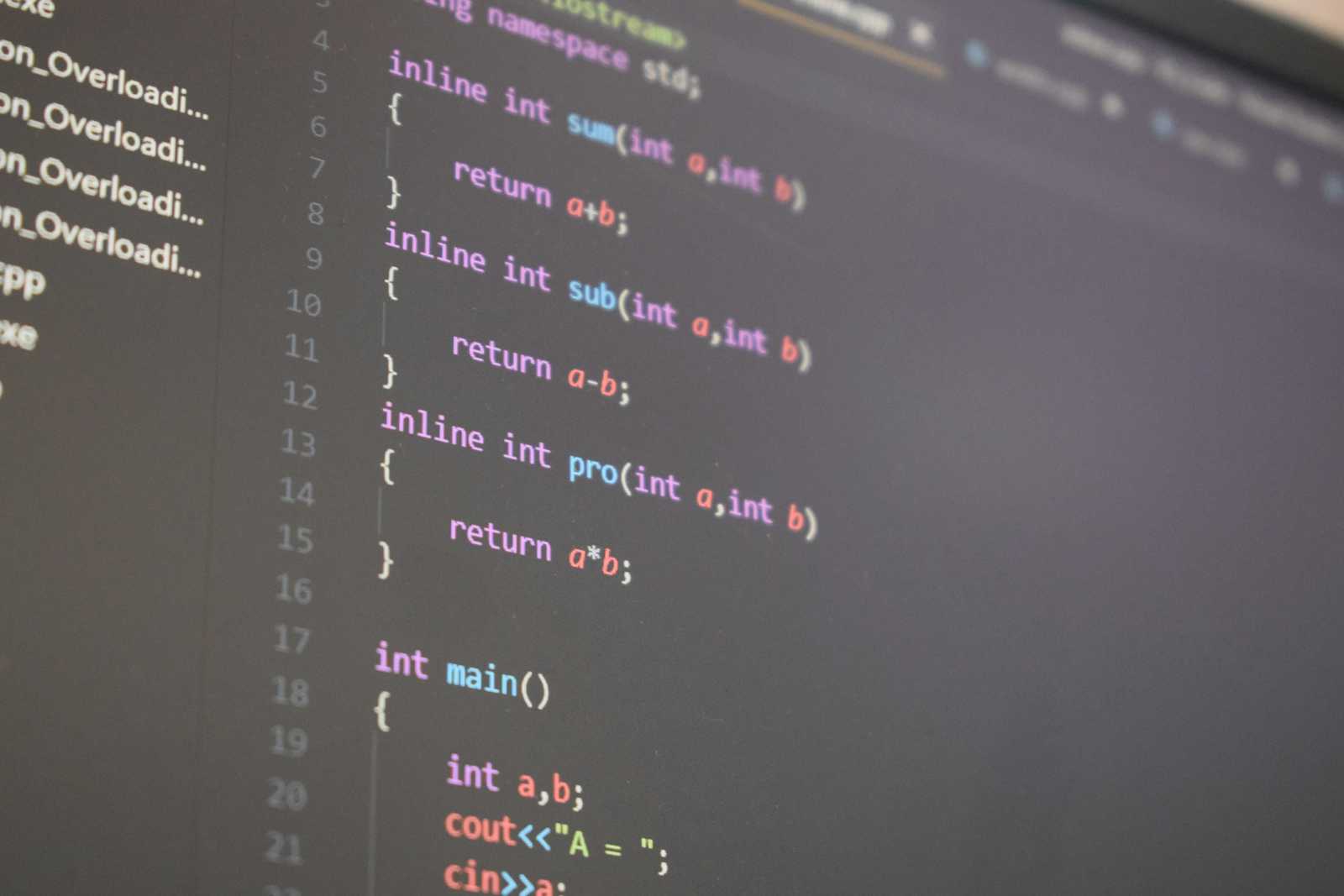
I have created a somewhat replica of the inbuilt objects 'cout' and 'cin' & the 'endl' function in C++
To achieve this thing :
1. I have created a namespace 'suraj_soni'
2. In that namespace I have created two classes 'Sout' & 'Sin'
3. 'Sout' class represents the printing functionality
4. 'Sin' class takes the input from the keyword
5. Also, I have created a function named as 'changeLine'
Do check it!
#include <stdio.h>
#include <string.h>
namespace suraj_soni
{
class Sout
{
public:
//operator<<(int) to print the int type data
Sout &operator<<(int x) //return type must of 'Sout&' to get the reference to this object only
{
printf("%d", x);
return *this; //returning the address of the object for which the method has been called
}
//operator<<(char) to print the char type data
Sout &operator<<(char x)
{
printf("%c", x);
return *this;
}
//operator<<(const char *x) to print the string type data
Sout &operator<<(const char *x)
{
printf("%s", x);
return *this;
}
//operator<<(double) to print the double type data
Sout &operator<<(double x)
{
printf("%lf",x);
return *this;
}
//operator<<(float) to print the float type data
Sout &operator<<(float x)
{
printf("%f",x);
return *this;
}
//operator<<(bool) to print the boolean type data
Sout &operator<<(bool x)
{
printf("%d",x);
return *this;
}
//operator<<(void (*p)()) to change the line
Sout &operator<<(void (*p)()) //the parameter 'p' here reprents the pointer to the function
{ //'p' can point to the function with no parameters and void return type
printf("\n");
return *this;
}
};
class Sin
{
public:
//operator>>(int &) will accept the int type data
int operator>>(int &x) //&x will act as the alias for the input feeded int type variable
{
scanf("%d", &x); //scanf will hault the program to accept the input from the keyboard
fflush(stdin);
return x;
}
//operator>>(char &) will accept the char type data
char operator>>(char &x)
{
scanf("%c", &x);
fflush(stdin);
return x;
}
//operator>>(char *) will accept the string type data
char* operator>>(char *x)
{
fgets(x,20,stdin);
x[strlen(x)-1]='\0';
fflush(stdin);
return x;
}
//operator>>(double &) will accept the double type data
double operator>>(double &x)
{
scanf("%lf", &x);
fflush(stdin);
return x;
}
//operator>>(float &) will accept the float type data
float operator>>(float &x)
{
scanf("%f", &x);
fflush(stdin);
return x;
}
//operator>>(bool &) will accept the boolean type data
bool operator>>(bool &x)
{
scanf("%d", &x);
fflush(stdin);
return x;
}
};
Sout sout; //sout object is somewhat similar to 'cout'
Sin sin; //sin is somewhat similar to 'cin'
void changeLine() //changeLine function is somewhat similar to the 'endl'
{
printf("\n");
}
}
using namespace suraj_soni;
int main()
{
sout << 10<<changeLine << 'A'<<changeLine << 20<<changeLine<<3.2f<<changeLine<<5.65<<changeLine<<true<<changeLine;
sout<<"Enter a number : ";
int x;
sin>>x;
sout<<'('<<x<<')'<<changeLine;
sout<<"Enter a char : ";
char xx;
sin>>xx;
sout<<'('<<xx<<')'<<changeLine;
sout<<"Enter a float : ";
float xe;
sin>>xe;
sout<<'('<<xe<<')'<<changeLine;
sout<<"Enter a double : ";
double xr;
sin>>xr;
sout<<'('<<xr<<')'<<changeLine;
sout<<"Enter a bool : ";
bool xw;
sin>>xw;
sout<<'('<<xw<<')'<<changeLine;
char xc[1000];
sout<<"Enter a string : ";
sin>>xc;
sout<<'['<<xc<<']'<<changeLine;
return 0;
}
0
Subscribe to my newsletter
Read articles from Suraj Soni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
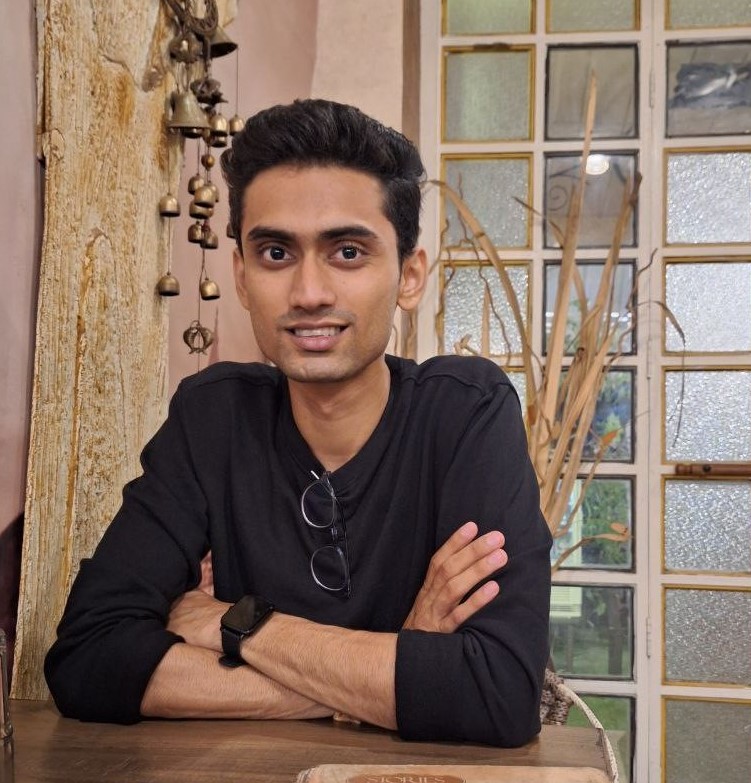
Suraj Soni
Suraj Soni
2023 Computer Science Grad. Interested in the technologies; C++, Python, AI/ML