Array Questions(Program)
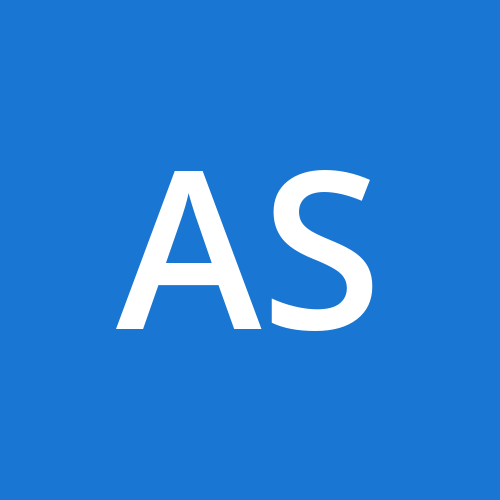
Find minimum and maximum element in an array
Given an array A of size N of integers. Your task is to find the minimum and maximum elements in the array.
pair<long long, long long> getMinMax(long long arr[], int n) {
pair <long, long> p1;
p1.first = p1.second = arr[0];
for(int i=0;i<n;i++)
{
if(p1.first > arr[i])
p1.first = arr[i];
if(p1.second < arr[i])
p1.second = arr[i];
}
return p1;
}
pair<long long, long long> getMinMax(long long a[], int n) {
pair<long long, long long> p;
long long max = a[0];
long long min = a[0];
for(int i=0;i<n;i++)
{
if(min > a[i])
min = a[i];
if(max < a[i])
max = a[i];
}
p.first = min;
p.second = max;
return p;
}
Largest Element in Array
Given an array A[] of size n. The task is to find the largest element in it.
class Solution
{
public:
int largest(vector<int> &arr, int n)
{
int max= arr[0];
for(int i=0;i<n;i++)
{
if(max < arr[i])
max = arr[i];
}
return max;
}
};
Immediate Smaller Element
Given an integer array Arr of size N. For each element in the array, check whether the right adjacent element (on the next immediate position) of the array is smaller. If next element is smaller, update the current index to that element. If not, then -1.
class Solution{
public:
void immediateSmaller(vector<int>&arr, int n) {
// code here
for(int i=0;i<n-1;i++)
{
if(arr[i] > arr[i+1])
arr[i]= arr[i+1];
else
arr[i] =-1;
}
arr[n-1]= -1;
}
};
First element to occur k times
Given an array of N integers. Find the first element that occurs at least K number of times.
class Solution{
public:
int firstElementKTime(int a[], int n, int k)
{
int max=a[0];
for(int i=0;i<n;i++)
{
if(max < a[i])
max = a[i];
}
int freq[max+1]={0};
for(int i=0;i<n;i++)
{
int index = a[i];
freq[index] +=1;
if (freq[index]>=k){
return a[i];
}
}
return -1;
}
};
Implement stack using array
Write a program to implement a Stack using Array. Your task is to use the class as shown in the comments in the code editor and complete the functions push() and pop() to implement a stack.
//Function to push an integer into the stack.
void MyStack :: push(int x)
{
// Your Code
if(top == 999)
return;
else
{
arr[++top] = x;
}
}
//Function to remove an item from top of the stack.
int MyStack :: pop()
{
// Your Code
if(top == -1)
return -1;
else
return arr[top--];
}
Implement Queue using array
Implement a Queue using an Array. Queries in the Queue are of the following type:
(i) 1 x (a query of this type means pushing 'x' into the queue)
(ii) 2 (a query of this type means to pop element from queue and print the poped element)
//Function to push an element x in a queue.
void MyQueue :: push(int x)
{
// Your Code
// if(rear == 100005)
// cout<<-1;
// if(front==0)
// front = 1;
arr[rear++]=x;
}
//Function to pop an element from queue and return that element.
int MyQueue :: pop()
{
// Your Code
if(front == rear)
return -1;
else
{
int elem = arr[front];
front++;
if(front > rear)
front=rear=0;
return elem;
}
}
Searching a number
Given an array Arr of N elements and a integer K. Your task is to return the position of first occurence of K in the given array.
class Solution{
public:
int search(int arr[], int n, int k) {
// code here
for(int i=0;i<n;i++)
{
if(arr[i] == k)
return i+1;
}
return -1;
}
};
Rotating an Array
Given an array of size N. The task is to rotate array by D elements where D ≤ N.
//User function template for C++
class Solution{
public:
void leftRotate(int arr[], int n, int d) {
// code here
int arr2[n], i, j;
for(i=d,j=0;i<n;i++)
arr2[j++]= arr[i];
for(i=0;i<d;i++)
arr2[j++] = arr[i];
for(i=0;i<n;i++)
arr[i]= arr2[i];
}
};
Binary Array Sorting
Given a binary array A[] of size N. The task is to arrange the array in increasing order.
int c0=0, c1=0;
for(int i=0;i<N;i++){
if(arr[i] == 0)
c0++;
else if(arr[i]==1)
c1++;
}
for(int i=0;i<c0;i++)
arr[i] =0;
for(int i=c0;i<c0+c1;i++)
arr[i] =1;
Third largest element
Given an array of distinct elements. Find the third largest element in it.
class Solution{
public:
int thirdLargest(int a[], int n)
{
//Your code here
if(n<=2)
return -1;
// int max = INT_MIN;
// for(int i=0;i<n;i++)
// {
// if (max < a[i])
// max = a[i];
// }
// int smax = INT_MIN;
// for(int i=0;i<n;i++)
// {
// if (smax < a[i] && a[i] < max )
// smax = a[i];
// }
// int tmax = INT_MIN;
// for(int i=0;i<n;i++)
// {
// if (tmax < a[i] && a[i] < smax )
// tmax = a[i];
// }
// return tmax;
// sort(a, a+n);
int max=INT_MIN, smax= INT_MIN, tmax = INT_MIN ;
for(int i=0;i<n;i++)
{
if(a[i] > max)
{
tmax = smax;
smax = max;
max = a[i];
}
else if(a[i] >smax)
{
tmax = smax;
smax = a[i];
}
else if(a[i]> tmax)
{
tmax = a[i];
}
}
return tmax;
}
};
Subscribe to my newsletter
Read articles from Akshima Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
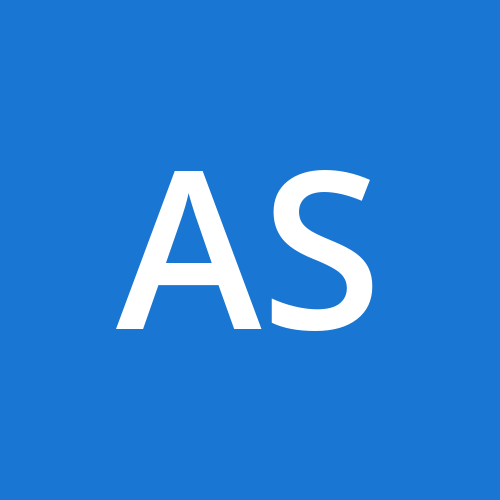