Day3 of #BashBlazeChallenge
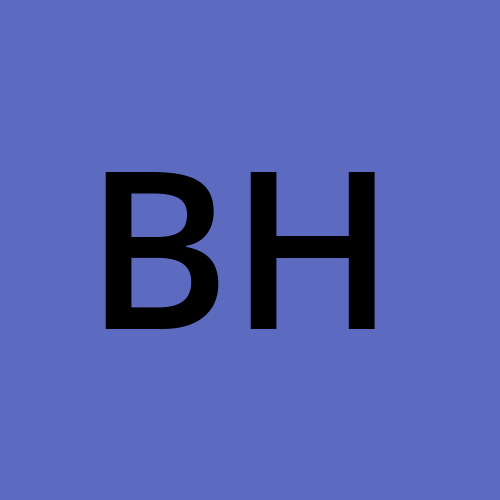
4 min read
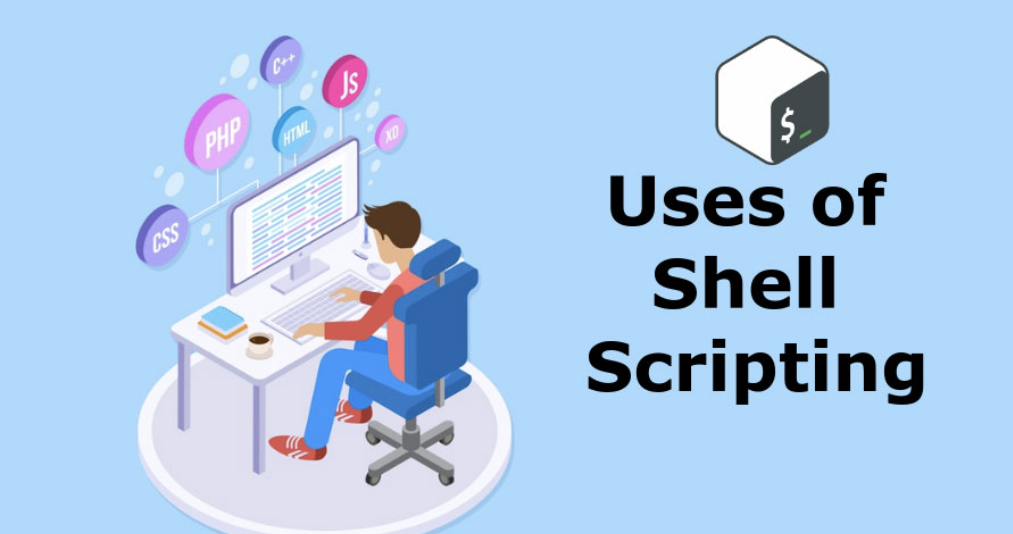
Summary:
I've successfully completed the #BashBlazeChallenge day3. The script I created helps users to effortlessly manage user accounts on a Linux system. It covers :
✨ Account Creation: Create new accounts with ease.
✨ Account Deletion: Say goodbye to unwanted accounts securely.
✨ Password Reset: Keep it secure with a straightforward password reset.
✨ List User Accounts: A quick overview of all users on the system.
Code :
#!/bin/bash
<<COMMENT1
The script I created helps users to effortlessly manage user accounts on a Linux system.
it covers :
Account Creation: Create new accounts.
Account Deletion: Deleting a existing user.
Password Reset: Password reset of a existing user.
List User Accounts: A quick overview of all users on the system.
COMMENT1
# Display function
function display_menu {
echo "Usage: $0 [OPTIONS]"
echo "Options:"
echo " -c, --create Create a new user account."
echo " -d, --delete Delete an existing user account."
echo " -r, --reset Reset password for an existing user account."
echo " -l, --list List all user accounts on the system."
echo " -h, --help Display this help and exit."
}
# User create function
function create_user {
read -p "Enter user name: " username # -p = prompt message
# Check if the user exists
count=($(sudo grep -c $username /etc/passwd))
if [ $count -eq 0 ];then
read -p "Enter password for user: " password
sudo useradd -m -p $password $username
echo "User created successfully."
else
echo "User already exists."
fi
}
# User delete function
function delete_user {
read -p "Enter user name: " username
# Check if the user exists
count=($(sudo grep -c $username /etc/passwd))
if [ $count -eq 1 ];then
sudo del -r $username
echo "User deleted successfully."
else
echo "User doesn't exists."
fi
}
# Password reset function
function reset_password {
read -p "Enter user name: " username
# Check if the user exists
count=($(sudo grep -c $username /etc/passwd))
if [ $count -eq 1 ];then
read -p "Enter new password: " new_password
echo "$username:$new_password" | sudo chpasswd
echo "User password changed successfully."
else
echo "User doesn't exists."
fi
}
# List user function
function list_users {
echo "List of users"
cat /etc/passwd | awk -F ':' '{print NR, "- " $1 " (UID:- " $3 " )"}'
}
if [ $# -eq 0 ] || [ $1 = "-h" ] || [ $1 = "--help" ];then
display_menu
exit 0
fi
while [ $# -gt 0 ];do
case $1 in
-c | --create)
create_user
;;
-d | --delete)
delete_user
;;
-r | --reset)
reset_password
;;
-l | --list)
list_users
;;
-h | --help)
display_menu
;;
*)
echo "Invalide input, give appropriate Options"
;;
esac
shift # Shifts the command line argument to left by 1
done
Output :
ubuntu@ip-172-31-1-222:~/scripts$ ./user_management.sh -h
Usage: ./user_management.sh [OPTIONS]
Options:
-c, --create Create a new user account.
-d, --delete Delete an existing user account.
-r, --reset Reset password for an existing user account.
-l, --list List all user accounts on the system.
-h, --help Display this help and exit.
ubuntu@ip-172-31-1-222:~/scripts$ ./user_management.sh -c -l -r -d
Enter user name: New_user
Enter password for user: new_user
User created successfully.
List of users
1 - root (UID:- 0 )
2 - daemon (UID:- 1 )
3 - bin (UID:- 2 )
4 - sys (UID:- 3 )
5 - sync (UID:- 4 )
6 - games (UID:- 5 )
7 - man (UID:- 6 )
8 - lp (UID:- 7 )
9 - mail (UID:- 8 )
10 - news (UID:- 9 )
11 - uucp (UID:- 10 )
12 - proxy (UID:- 13 )
13 - www-data (UID:- 33 )
14 - backup (UID:- 34 )
15 - list (UID:- 38 )
16 - irc (UID:- 39 )
17 - gnats (UID:- 41 )
18 - nobody (UID:- 65534 )
19 - systemd-network (UID:- 100 )
20 - systemd-resolve (UID:- 101 )
21 - messagebus (UID:- 102 )
22 - systemd-timesync (UID:- 103 )
23 - syslog (UID:- 104 )
24 - _apt (UID:- 105 )
25 - tss (UID:- 106 )
26 - uuidd (UID:- 107 )
27 - tcpdump (UID:- 108 )
28 - sshd (UID:- 109 )
29 - pollinate (UID:- 110 )
30 - landscape (UID:- 111 )
31 - fwupd-refresh (UID:- 112 )
32 - ec2-instance-connect (UID:- 113 )
33 - _chrony (UID:- 114 )
34 - ubuntu (UID:- 1000 )
35 - lxd (UID:- 999 )
36 - User1 (UID:- 1001 )
37 - New_user (UID:- 1002 )
Enter user name: New_user
Enter new password: new_password
User password changed successfully.
Enter user name: New_user
sudo: del: command not found
User deleted successfully.
0
Subscribe to my newsletter
Read articles from Bharat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
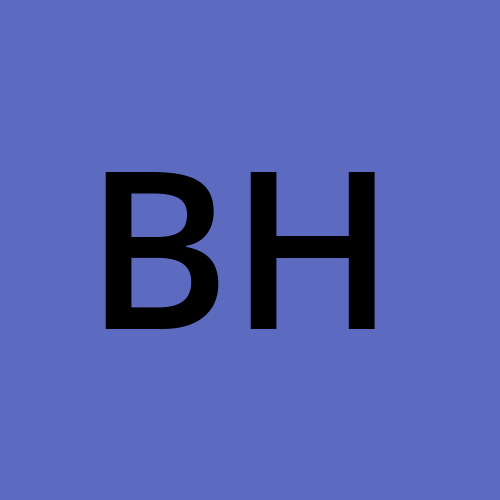