JavaScript Events (Part-1): Events, their types, and handlers in JavaScript in-depth
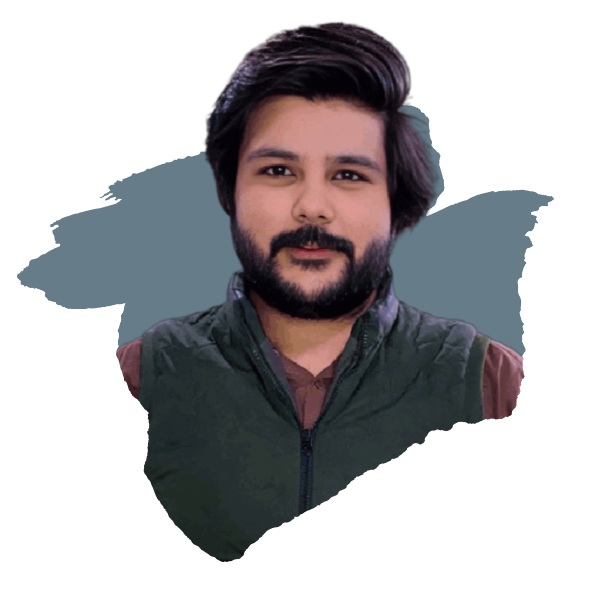
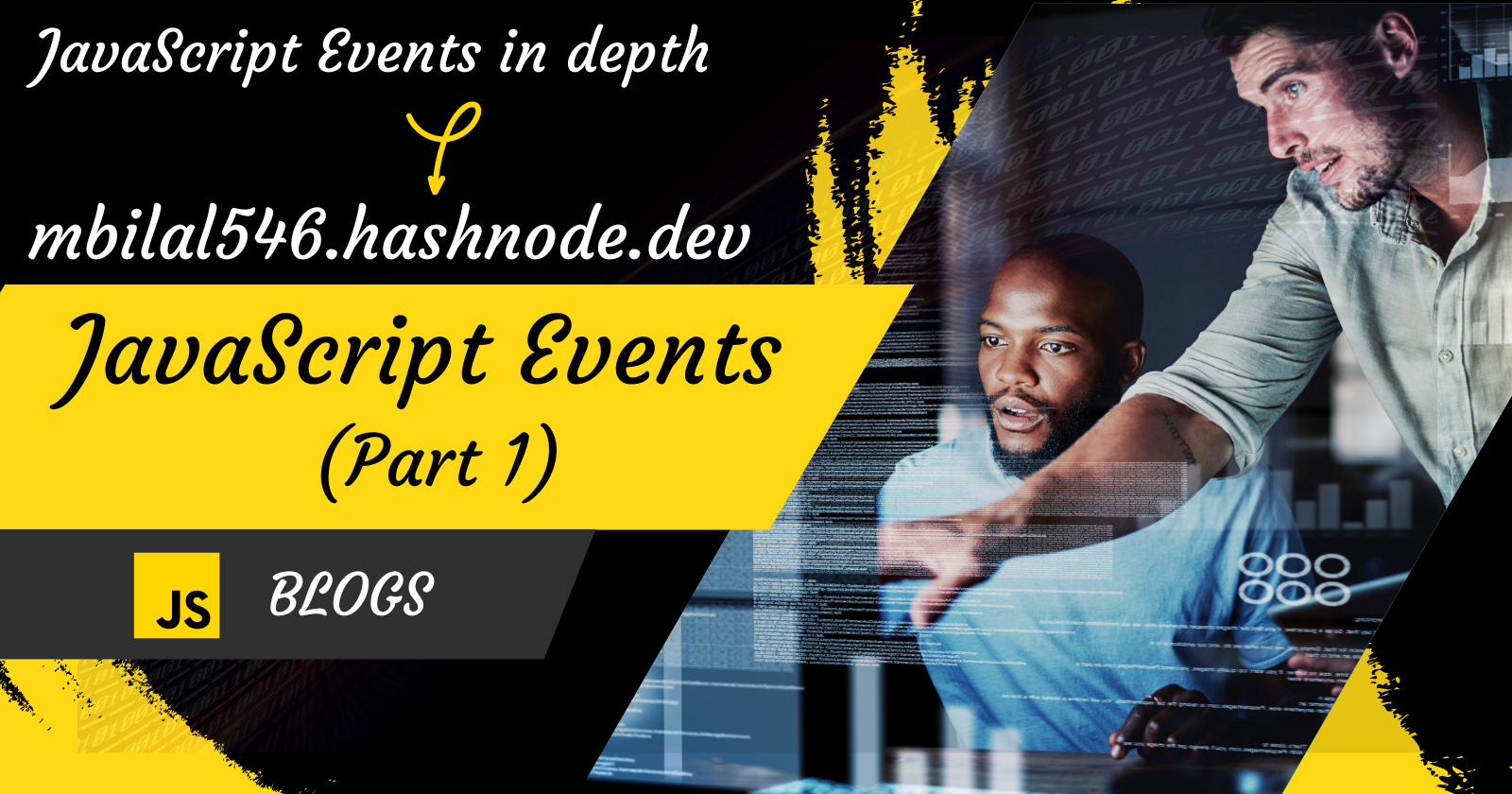
What are events in JavaScript?
Events are actions or occurrences that happen in the browser environment, such as user interactions (clicks, keypresses, form submissions), page loading, data fetching, or system-level changes. JavaScript allows you to react to these events and execute code in response, making your web pages interactive and dynamic. Events are fired by specific elements in the browser, known as event sources. Such as HTML elements (buttons, forms, images, etc.), the browser window itself, and the document elements (the entire HTML document).
What are event handlers in JavaScript?
Event handlers are JavaScript functions that are attached to event sources. They define the code that should be executed when a particular event occurs.
You can assign event handlers in two ways:
Inline attributes:
<button onclick="myFunction()">Click me</button>
JavaScript code:
button.addEventListener("click", myFunction(){});
Bypassing the reference to the function.JavaScript code:
button.addEventListener("click", myFunction);
The second and third ones are the better approaches.
Let's talk about the types of events
There are the following types of events:
Mouse Events
click
In JavaScript, you can handle mouse-click events using the click
event. This event is triggered when the user clicks the left mouse button.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect a mouse click on an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
</head>
<body>
<h3 id="para">
This is the paragraph
</h3>
<button id="btn">Click Me To change Bg Color & Color</button>
</body>
</html>
If you click the given button, then the background and the color are changed by using the JavaScript code given below
let button = document.getElementById("btn");
function clickBtn() {
document.body.style.backgroundColor = "#212121";
document.body.style.color = "#ffffff";
}
button.addEventListener("click", clickBtn);// Passing function's reference
dblclick
In JavaScript, thedblclick
event is triggered when a user double-clicks on an element using the mouse.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect a mouse double-click on an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
</head>
<body>
<h3 id="para">
This is the paragraph
</h3>
<button id="btn">DblClick Me To change Bg Color & Color</button>
</body>
</html>
If you double-click the given button, then the background and the color are changed by using the JavaScript code given below
let button = document.getElementById("btn");
function dblClickBtn() {
document.body.style.backgroundColor = "red";
document.body.style.color = "white";
}
button.addEventListener("dblclick", dblClickBtn);// Passing function's reference
mousedown
In JavaScript, themousedown
event is triggered when a mouse button is pressed down over an element. This event is commonly used to detect when a user initiates a click-or-drag operation.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect a mouse down on an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
</head>
<body>
<h3 id="para">
This is the paragraph
</h3>
<button id="btn">Press Click To change Bg Color & Color</button>
</body>
</html>
If you press the mouse button (whether it is the right or left button), then the background and the color are changed by using the JavaScript code given below
let button = document.getElementById("btn");
function mouseDown() {
document.body.style.backgroundColor = "red";
document.body.style.color = "white";
}
button.addEventListener("mousedown", mouseDown);// Passing function's reference
mouseup
In JavaScript, themouseup
event is triggered when a mouse button is released over an element. This event is commonly used to detect when a user has finished clicking or interacting with an element through a mouse button.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect a mouse-up event on an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
</head>
<body>
<h3 id="para">
This is the paragraph
</h3>
<button id="btn">Press Click and release To change Bg Color & Color</button>
</body>
</html>
If you release the mouse button (whether it is the right or left button), then the background and the color are changed by using the JavaScript code given below
let button = document.getElementById("btn");
function mouseUp() {
document.body.style.backgroundColor = "red";
document.body.style.color = "white";
}
button.addEventListener("mouseup", mouseUp);// Passing function's reference
mouseover
In JavaScript, themouseover
event is triggered when the mouse pointer enters the area covered by an element on a web page. This event is commonly used to implement interactive features or provide feedback when the user interacts with elements on the page.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect a mouseover event on an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
</head>
<body>
<h3 id="para">
This is the paragraph
</h3>
<button id="btn">MouseOver me To change Bg Color & Color</button>
</body>
</html>
If you enter the mouse in a specific area, like a button, then the background and the color are changed by using the JavaScript code given below
let button = document.getElementById("btn");
function mouseOver() {
document.body.style.backgroundColor = "red";
document.body.style.color = "white";
}
button.addEventListener("mouseover", mouseOver);// Passing function's reference
mouseout
Themouseout
event in JavaScript is triggered when the mouse pointer moves out of an element. It is often used in combination with themouseover
event to implement functionality when the mouse enters or leaves a particular element.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect a mouse-out event on an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
</head>
<body>
<h3 id="para">
This is the paragraph
</h3>
<button id="btn">MouseOut from me To change Bg Color & Color</button>
</body>
</html>
If you enter the mouse in a specific area like a button and then move your mouse out to that area, then the background and the color are changed by using the JavaScript code given below
let button = document.getElementById("btn");
function mouseOut() {
document.body.style.backgroundColor = "#212121";
document.body.style.color = "#ffffff";
}
button.addEventListener("mouseout", mouseOut);// Passing function's reference
mouseenter
In JavaScript, themouseenter
event is triggered when the mouse pointer enters the boundaries of a specific HTML element. This event is often used in conjunction with themouseover
event, but it differs in that it doesn't bubble up through descendant elements.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect a mouse-enter event on an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
</head>
<body>
<h3 id="para">
This is the paragraph
</h3>
<button id="btn">Enter the mouse To change Bg Color & Color</button>
</body>
</html>
If you enter the mouse in a specific area, like a button, then the background and the color are changed by using the JavaScript code given below
let button = document.getElementById("btn");
function mouseEnter() {
document.body.style.backgroundColor = "#212121";
document.body.style.color = "#ffffff";
}
button.addEventListener("mouseenter", mouseEnter);// Passing function's reference
mouseleave
In JavaScript, you can use the mouseleave
event to detect when the mouse leaves an element. Themouseleave
event is triggered when the mouse pointer leaves the boundaries of an element.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect a mouse-leave event on an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
</head>
<body>
<h3 id="para">
This is the paragraph
</h3>
<button id="btn">Enter and then leave the mouse To change Bg Color & Color</button>
</body>
</html>
If you enter the mouse in a specific area like a button and then leave the mouse in that area, then the background and the color are changed by using the JavaScript code given below
let button = document.getElementById("btn");
function mouseLeave() {
document.body.style.backgroundColor = "#212121";
document.body.style.color = "#ffffff";
}
button.addEventListener("mouseleave", mouseLeave);// Passing function's reference
contextmenu
In JavaScript, you can handle thecontextmenu
event to capture when the right mouse button is clicked, triggering the context menu.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect a context menu event on an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
</head>
<body>
<h3 id="para">
This is the paragraph
</h3>
<button id="btn">Right click the mouse To change Bg Color & Color</button>
</body>
</html>
If you right-click the mouse in a specific area, like a button, then the background and the color are changed by using the JavaScript code given below
let button = document.getElementById("btn");
function contextMenu() {
document.body.style.backgroundColor = "#212121";
document.body.style.color = "#ffffff";
}
button.addEventListener("contextmenu", contextMenu);// Passing function's referen
mousemove
In JavaScript, you can use the mousemove
event to detect when the mouse pointer moves over an element on a web page in the x-axis and y-axis.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect a mouse move event on an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
<style>
body{
margin: 0;
padding: 0;
box-sizing: border-box;
display: flex;
justify-content: center;
background-color: #212121;
}
#main{
width: 70%;
height: 100vh;
background-color: black;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
#btn{
width: 30%;
}
</style>
</head>
<body>
<div id="main">
<h1 style="color: yellow;">This is main div</h1>
<button id="btn">Drag in div to see how many px cursor cover</button>
</div>
</body>
</html>
If you drag the mouse in a specific area, like a div, then it will tell how many pixels it moves in the x-axis and y-axis by using the JavaScript code given below.
let main = document.getElementById("main");
function mouseMoveDetails(e){
console.log(e);
}
main.addEventListener("mousemove", mouseMoveDetails); // This provide details
// of mousemove event of both x-axis and y-axis when you move the mouse.
function mouseMove(e){
console.log(e.clientX+"px");
console.log(e.clientY+"px");
}
main.addEventListener("mousemove", mouseMove);// Passing function's reference
// it will tell how many px it moves in the x-axis and y-axis
// We can also use x and y in place of clientX and clientY
Keyboard Events
There are some keyboard events; let's discuss them one by one
keydown
Theonkeydown
event occurs when a key on the keyboard is pressed down. It can be attached to any HTML element, and when the specified key is pressed, the associated event handler function is executed.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect anonkeydown
event:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
<style>
body{
margin: 0;
padding: 0;
box-sizing: border-box;
display: flex;
justify-content: center;
background-color: black;
}
#screen{
width: 50%;
background-color: #212121;
color: white;
padding: 100px 20px;
display: flex;
flex-direction: column;
align-items: center;
}
#myInput{
width: 50%;
height: 30px;
padding: 5px;
font-size: 18px;
}
</style>
</head>
<body>
<div id="screen">
<h1 style="font-size: 60px;">onkeydown Event</h1>
<input type="text" id="myInput">
</div>
</body>
</html>
If you press any key in the input tag, then it shows that key in the alert box, and after pressing OK, that key shows in your output by using the JavaScript code given below.
let input = document.getElementById('myInput');
input.onkeydown = function(event) {
alert(`The key released by keyboard is: ${event.key}`);
};
keyup
In JavaScript, theonkeyup
event is triggered when a key is released (pressed down and then released) on the keyboard. This event is commonly used to perform actions or execute code in response to user input.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect anonkeyup
event:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
<style>
body{
margin: 0;
padding: 0;
box-sizing: border-box;
display: flex;
justify-content: center;
background-color: black;
}
#screen{
width: 50%;
background-color: #212121;
color: white;
padding: 100px 20px;
display: flex;
flex-direction: column;
align-items: center;
}
#myInput{
width: 50%;
height: 30px;
padding: 5px;
font-size: 18px;
}
</style>
</head>
<body>
<div id="screen">
<h1 style="font-size: 60px;">onkeyup Event</h1>
<input type="text" id="myInput">
</div>
</body>
</html>
If you press any key in the input tag, then it shows that key in the alert box, and input tag immediately by using the JavaScript code given below.
let input = document.getElementById('myInput');
input.onkeyup = function(event) {
alert(`The key pressed by keyboard is: ${event.key}`);
};
We can do the same thing with addEventListner
which is the proficient method.
let input = document.getElementById('myInput');
function onKeyUp(event) {
alert(`The key pressed by keyboard is: ${event.key}`);
};
document.addEventListener('keyup', onKeyUp);
This event has the following use cases:
Real-time input validation:
- You can use it to validate user input in real time as they type.
Autocomplete Suggestions:
- Implementing autocomplete features where suggestions are provided based on user input.
Dynamic Content Loading:
- Triggering content updates or fetching data from a server as the user types.
Shortcut Key Detection:
- Recognizing specific key combinations or shortcuts.
keypress
Theonkeypress
event in JavaScript is triggered when a key is pressed and held down. This event is associated with the keyboard, and it can be used to capture and respond to user input related to key presses.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect anonkeypress
event:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
<style>
body{
margin: 0;
padding: 0;
box-sizing: border-box;
display: flex;
justify-content: center;
background-color: black;
}
#screen{
width: 50%;
background-color: #212121;
color: white;
padding: 100px 20px;
display: flex;
flex-direction: column;
align-items: center;
}
#myInput{
width: 50%;
height: 30px;
padding: 5px;
font-size: 18px;
}
</style>
</head>
<body>
<div id="screen">
<h1 style="font-size: 60px;">onkeypress Event</h1>
<input type="text" id="myInput">
</div>
</body>
</html>
If you press any key in the input tag, then it shows that key in the alert box, and after pressing OK, that key shows in your output by using the JavaScript code given below.
let input = document.getElementById('myInput');
function onKeyPress(event) {
alert(`The key pressed by keyboard is: ${event.key}`);
console.log(event); // in console it will show you properties
// like key and some other properties
};
document.addEventListener('keypress', onKeyPress);
Note:
If you press any key in the input tag, then it shows that key in the alert box, and after pressing OK, that key shows in your output. After reading this, you are thinking that onkeydown
&onkeypress
are the same but they are different because onkeydown
consider( ctrl
, shift
, andbackspace
) these as keys.
Now let's talk about some event object properties of all of these by using onkeyup
key
Theevent.key
property is part of the KeyboardEvent interface in JavaScript. It is commonly used to retrieve the value of the key pressed during a keyboard event.
Here's a basic example using HTML and JavaScript to demonstrate how you can detect ankey property
event:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Understanding DOM Events</title>
<style>
body{
margin: 0;
padding: 0;
box-sizing: border-box;
display: flex;
justify-content: center;
background-color: black;
}
#screen{
width: 50%;
background-color: #212121;
color: white;
padding: 100px 20px;
display: flex;
flex-direction: column;
align-items: center;
}
#myInput{
width: 50%;
height: 30px;
padding: 5px;
font-size: 18px;
}
</style>
</head>
<body>
<div id="screen">
<h1 style="font-size: 60px;">key Property Event</h1>
<input type="text" id="myInput">
</div>
</body>
</html>
If you press any key in the input tag, then it shows that key by using the JavaScript code given below.
let input = document.getElementById('myInput');
function onKeyUp(event) {
alert(`The key pressed by keyboard is: ${event.key}`);
};
document.addEventListener('keyup', onKeyUp);
keyCode
Theevent.keyCode
property is part of theKeyboardEvent
object in JavaScript, and it represents the Unicode value of a key that was pressed during the event. It is important to note that thekeypress
event is deprecated, and it is recommended to use the keydown
orkeyup
events instead.
If you press any key in the alert box, then it shows the Unicode of the key by using the JavaScript code given below.
let input = document.getElementById('myInput');
function onKeyUp(event) {
alert(`The key pressed by keyboard is: ${event.keyCode}`);
};
document.addEventListener('keyup', onKeyUp);
which
Theevent.which
property is used to retrieve the Unicode character code of the pressed key.
If you press any key in the alert box, then it shows the Unicode of the key by using the JavaScript code given below.
let input = document.getElementById('myInput');
function onKeyUp(event) {
alert(`The key pressed by keyboard is: ${event.which}`);
};
document.addEventListener('keyup', onKeyUp);
ctrlKey
Theevent.ctrlKey
property is a boolean property. It indicates whether the Ctrl key (or the Command key on Mac) is pressed or not when a keyboard event occurs.
If you press the ctrl
key then in the alert box it shows the true
or false
by using the JavaScript code given below.
let input = document.getElementById('myInput');
function onKeyUp(event) {
alert(`The key pressed by keyboard is: ${event.ctrlKey}`);
};
document.addEventListener('keyup', onKeyUp);
Same as for shiftKey
and altKey
and others.
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
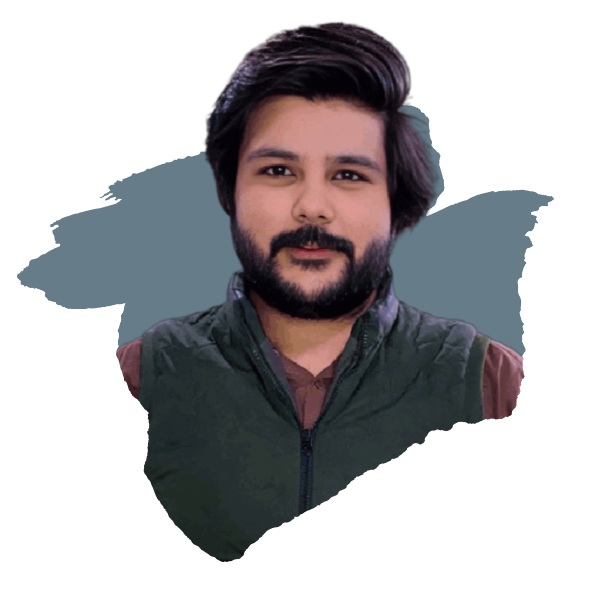
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. 📈 Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. 🔊 Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub 🖥 Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. ✨ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. 🎓 Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. 👥 Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.