A Guide For Developing React Native Offline First Apps
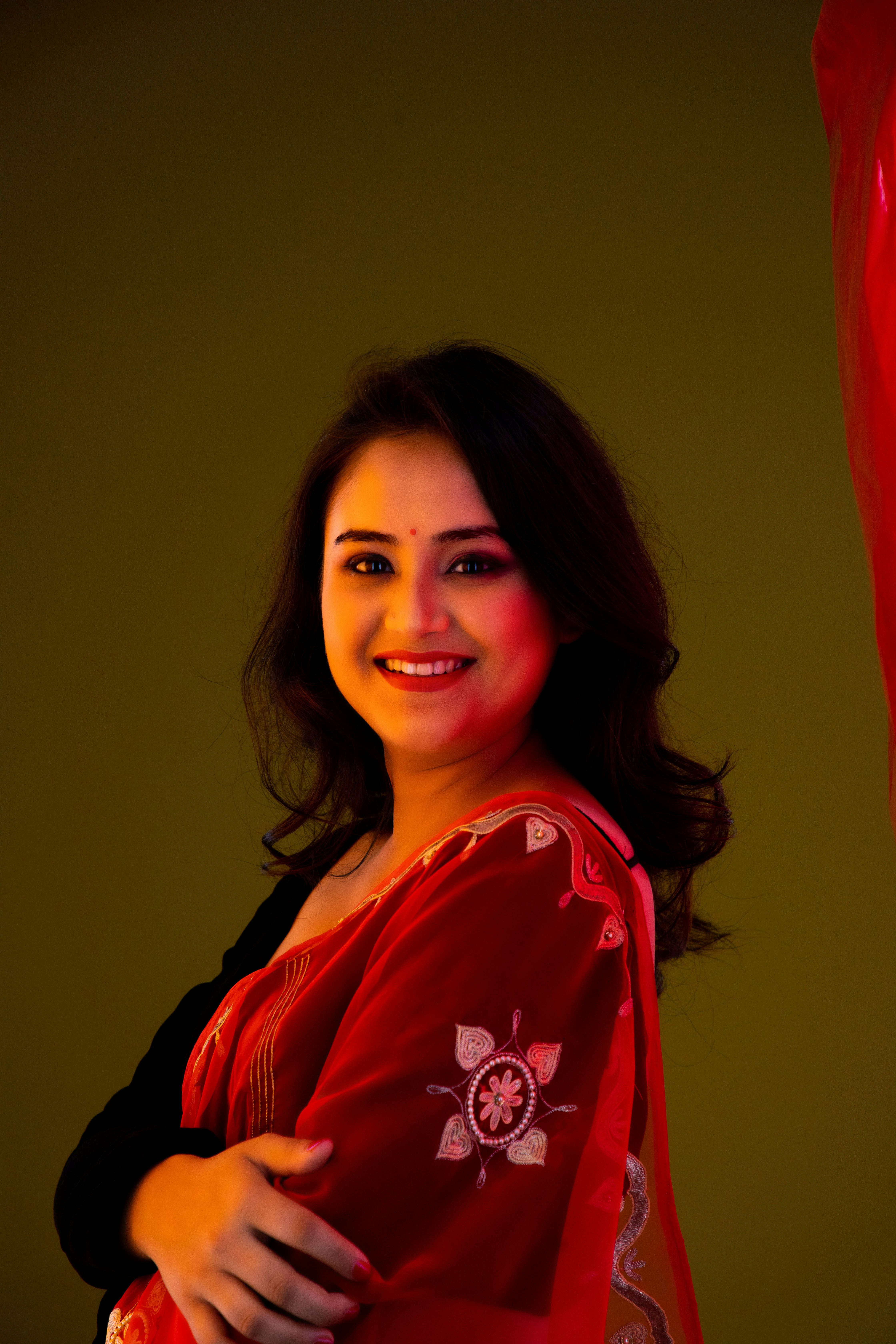
The increasing use of mobile phones have resulted in the reliance on the mobile apps for daily activities like paying utility bills, booking cab rides and more. Most of these functionalities can only be performed with a reliable internet connection.
But what if someone doesn't have internet connectivity? Well, then you can use an offline-first app. Although most of the apps and activities nowadays require you to have internet connectivity, offline-first apps allow you to perform certain activities with limited access in many cases.
These applications have offline capabilities thanks to the support of React Native technology. It is also one of the most preferred options for business owners as well as developers to build an offline-first app. Before we dive into the process of developing a React Native offline-first app, let us explore the concept in detail.
#Understanding Offline-First Applications in React Native
The native applications that can be used without an internet connection are called offline-first applications. Such apps are built using the React native technology, which is called React Native offline first applications. However, now most mobile phone users have access to the internet. So, why would one want to use such apps?
What if the internet connection is poor or has limited accessibility? In addition to that, offline first apps are more user-friendly in comparison to the ones that need the internet. Offline first apps were designed as a solution to the restricted functionality problem of online apps. Data storage and management is handled at the local level in the offline apps. It's fast and easy.
So, due to restricted functionality, the users will experience interruptions even with the internet connections. And because offline apps can load pages and content quickly, users' actions are completed quickly. They also allow you to save and sync data locally. So, it can be used later.
So, even without an internet connection, the users can enjoy the same experience with the responsive user interface of React Native offline first apps.
#Workflow for Offline-First Applications
Before we discuss how React native offline first app works, it is necessary to go through the working of the offline first application, then we can see how React native offline first app works.
When opened, the first thing the app will do is to check whether the device has access to the internet or not. After verifying that there is no internet connection, the app keeps the user activities in an offline database that can not be accessed by other app users.
When the app gets internet connectivity, the local database is connected with an online server. In this stage, the data is shared with other app users. To know it better, look at the architectural flow of the offline first app below:
#Implementing Offline Support in React Native: Methods
#Usage Restrictions
In this method, the app will display a message on the screen stating that because the app is currently unable to connect to the internet, some features that need internet connection to function, will not be functioning.
Here is how you can implement the usage restrictions in an offline first React native app that provides offline notifications. Step: 1 Use NPM and install the @react-native-community/netinfo dependency: npm install @react-native-community/netinfo Step: 2 (iOS) If you are using iOS then enter the command shown below:
cd ios pod install
Step: 2 (Android) To support Android, you have to modify your android/build.gradle configuration. . buildscript { ext { buildToolsVersion = “28.0.3” minSdkVersion = 16 compileSdkVersion = 28 targetSdkVersion = 28 supportLibVersion = “28.0.0” } }
Step: 3 You have to create Offline.Notice.js component in your components or apps.
import React from ‘react’; import { View, Text, Image, StyleSheet } from ‘react-native’; import { useNetInfo } from ‘@react-native-community/netinfo’; const OfflineNotice = () => { const netInfo = useNetInfo(); if (netInfo.type !== ‘unknown’ && netInfo.isInternetReachable === false) return ( <View style={styles.container}> <Image style={styles.image} source={require(‘../assets/images/offline.png’)} /> <Text style={styles.text}>No Internet Connection</Text> </View> ); return null; }; const styles = StyleSheet.create({ container: { alignItems: ‘center’, justifyContent: ‘center’, height: ‘100%’, width: ‘100%’, zIndex: 1, }, image: { height: 500, width: 500, }, text: { fontSize: 25, }, }); export default OfflineNotice;
Step: 4 Import the OfflineNotice component in your app to utilize it.
import React, { useState } from ‘react’; import { StyleSheet, SafeAreaView } from ‘react-native’; import LandingScreen from ‘./Screens/LandingScreen’; import userApi from ‘./api/user’; import OfflineNotice from ‘./components/OfflineNotice’; const App = () => { const [users, setUsers] = useState([]); const getUser = async () => { const tempUser = await userApi.getUsers(); setUsers([…users, tempUser]); }; return ( <> <OfflineNotice /> <SafeAreaView style={styles.container}> <LandingScreen getUser={getUser} users={users} /> </SafeAreaView> </> ); }; const styles = StyleSheet.create({ container: { flex: 1, }, });
export default App;
# Caching
Caching enables the app to download the answers given by the server to the local device storage. So, when you cannot access the internet, you will still be able to retrieve and render the required data from your device’s local storage.
The downloading capabilities of YouTube and other similar apps show how caching works. Redux With Caching Redux caching is implemented in your React Native applications by using redux-persist. Step: 1 Run the command given below to run all essential dependencies:
npm install redux react-redux redux-saga redux-persist @redux-devtools/extension @react-native-async-storage/async-storage Step: 2 For updating the Redux store configuration, you have to add the following code in your app/store/index.js.
import { createStore, applyMiddleware } from ‘redux’; import { composeWithDevTools } from ‘@redux-devtools/extension’; import createSagaMiddleware from ‘redux-saga’; import { persistStore, persistReducer } from ‘redux-persist’; import AsyncStorage from ‘@react-native-async-storage/async-storage’; import reducers from ‘../reducer’; import sagas from ‘../sagas’; const sagaMiddleware = createSagaMiddleware(); const persistConfig = { key: ‘root’, storage: AsyncStorage, }; const persistedReducer = persistReducer(persistConfig, reducers); export const store = createStore( persistedReducer, composeWithDevTools(applyMiddleware(sagaMiddleware)) ); sagaMiddleware.run(sagas); export const persistor = persistStore(store);
Step: 3 App component is wrapped using the index.js’s Persistgate component.
import React from ‘react’; import { AppRegistry } from ‘react-native’; import { Provider } from ‘react-redux’; import { PersistGate } from ‘redux-persist/integration/react’; import App from ‘./app/App’; import { name as appName } from ‘./app.json’; import { store, persistor } from ‘./app/store’; const ConnectedApp = () => { return ( <Provider store={store}> <PersistGate persistor={persistor} loading={null}> <App /> </PersistGate> </Provider> ); }; AppRegistry.registerComponent(appName, () => ConnectedApp);
Combining redux with caching is effectively applied in your React Native app using the steps given above with the help of the redux-persist. The PersistGate component also helps you cache and retrieve data without facing any difficulties. This is to make sure that your Redux store is hydrated and persists between app runs.
# Request Queue
It stores all the feature requests while the app is offline and implement them all when the app is connected to the internet. The offline edit function of Google Docs is a fine example of a request queue along with all the note-taking software available in the market that allows you to make and implement changes even without an internet connection. Generally, the online apps save changes to the server.
In your React Native offline-first app, you can implement Request Queue using the redux-offline library. Step: 1 Install the @redux-offline/redux-offline dependency using the following command: npm install @redux-offline/redux-offline
Step: 2 In the store.js settings, add the code given below for your redux store.
import { applyMiddleware, createStore, compose } from ‘redux’; import { offline } from ‘@redux-offline/redux-offline’; import offlineConfig from ‘@redux-offline/redux-offline/lib/defaults’; const store = createStore( reducer, compose( applyMiddleware(middleware), offline(offlineConfig) ) );
Step: 3 The offline metadata redefines your redux architecture. You are allowed to make the following changes in the SetUsers action:
const setUsers = users => ({ type: ‘SET_USERS’, payload: users, meta: { offline: { effect: { url: ‘/api/save-data’, method: ‘POST’, json: { users } }, commit: { type: ‘SAVE_USERS_COMMIT’, meta: { users } }, rollback: { type: ‘SAVE_USERS_ROLLBACK’, meta: { users } } } } });
The offline metadata is included in the code's meta attribute of the action. If the effect is successful, you have to dispatch the commit property action. But if the network action fails, you have to dispatch the rollback property action. To save your modifications, you have to imply the effect property.
All the network activities queued in the Request Queue will be carried out once the app reconnects with the internet. But if the network activity fails permanently, the changes in the state are undone with rollback action.
<uses-permission android:name=”android.permission.ACCESS_NETWORK_STATE” />
# React Native Offline App Development Best Practices
To lower the bounce rate and maintain user engagement in your app, using React Native is critical for your offline application. The best practices discussed below will help you build React Native offline-first apps with maximized performance.
Use Local Databases Utilizing local databases such as WatermelonDB, Realm, SQLite, and more allows your app to function without an internet connection. This can help you save and manage the data. And it can be synced with the server once the app is reconnected with the internet.
Optimizing Performance Offline-first apps built using React Native are seen to have fewer server queries, use less data, and perform better. It lowers the server latency and minimizes the volume of data transacted on the network which helps make your app faster.
Prioritize User Needs When you are building an offline-first app, you have to consider how the users are going to interact with your app. This helps you deliver a seamless user experience when the app is offline.
Implement Sync Mechanism
Although, in offline use, the data is stored in the local device database and when the app is reconnected with the internet, the data is updated on the server. For that, you need effective synchronization methods that utilize advanced strategies like polling servers, serverless architectures, WebSockets, and more.
Cache Matters Caching is a process that allows your app to store the app data in the local database without internet connectivity. It makes sure that your app works fine without internet connectivity. Your offline-first app handles the cached data using AsyncStorage, React Native Cache, and other caching libraries.
Offline Error Handlin
Handling errors is an important aspect of every app development process. While working with an offline-first approach, error handling is required for tasks such as bill payments, purchases, filling forms, and more that can only be done online.
The end user must get a feedback message or notification to understand the problem and take necessary action to restore the internet connectivity. 7. Comprehensive Testing Conducting a thorough test for each component of your app helps you ensure that the UX is not affected negatively in any way. Running offline tests in different environments and with poor internet connection helps you offer a seamless user experience under these situations.
#The Significance of Offline-First Applications
Data Cost Reduction
Offline first apps have either no internet usage or limited. When materials are cached on devices instead of the internet, users can save money on their data plan which is very crucial to them. So, the use of offline-first apps saves data costs for the users.
Enhanced Sync
Apps that are designed to run offline, come with a synchronization algorithm that updates the app data whenever an internet connection is available. This helps maintain the user data accuracy and prevents data loss.
Enhanced Access Rural and remote areas have little or no internet accessibility. So, online apps aren't suitable for them, but they can easily access the React Native offline first app. Increasing the number of app users and providing them unrestricted access becomes an easy task.
Boosting Efficiency Offline first apps come with a faster and more responsive UX. They have server requests that improve the app performance and reduce the latency.
Improved User Experience
Two things a user wouldn't have to worry about while using an offline first app. First, the app will function seamlessly without any glitch when offline. And second is that it will be faster and provide local data storing and management options, which will further improve the working of the app in the absence of an internet connection.
So, when the app doesn't have to go to the server every time to bring the user requests material and can get it right from the local device, the speed and performance of the app increases.
#Conclusion
To offer enhanced user experience, offline first functionality is crucial. And React native comes in handy for building it. This blog discussed some methods on how React native can help you build offline first functionality. In addition to that, we also went through a series of best practices that can help you deliver an improved user experience and app performance.
I hope this is helpful to you. Thanks for reading!
Subscribe to my newsletter
Read articles from Ishikha rao directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
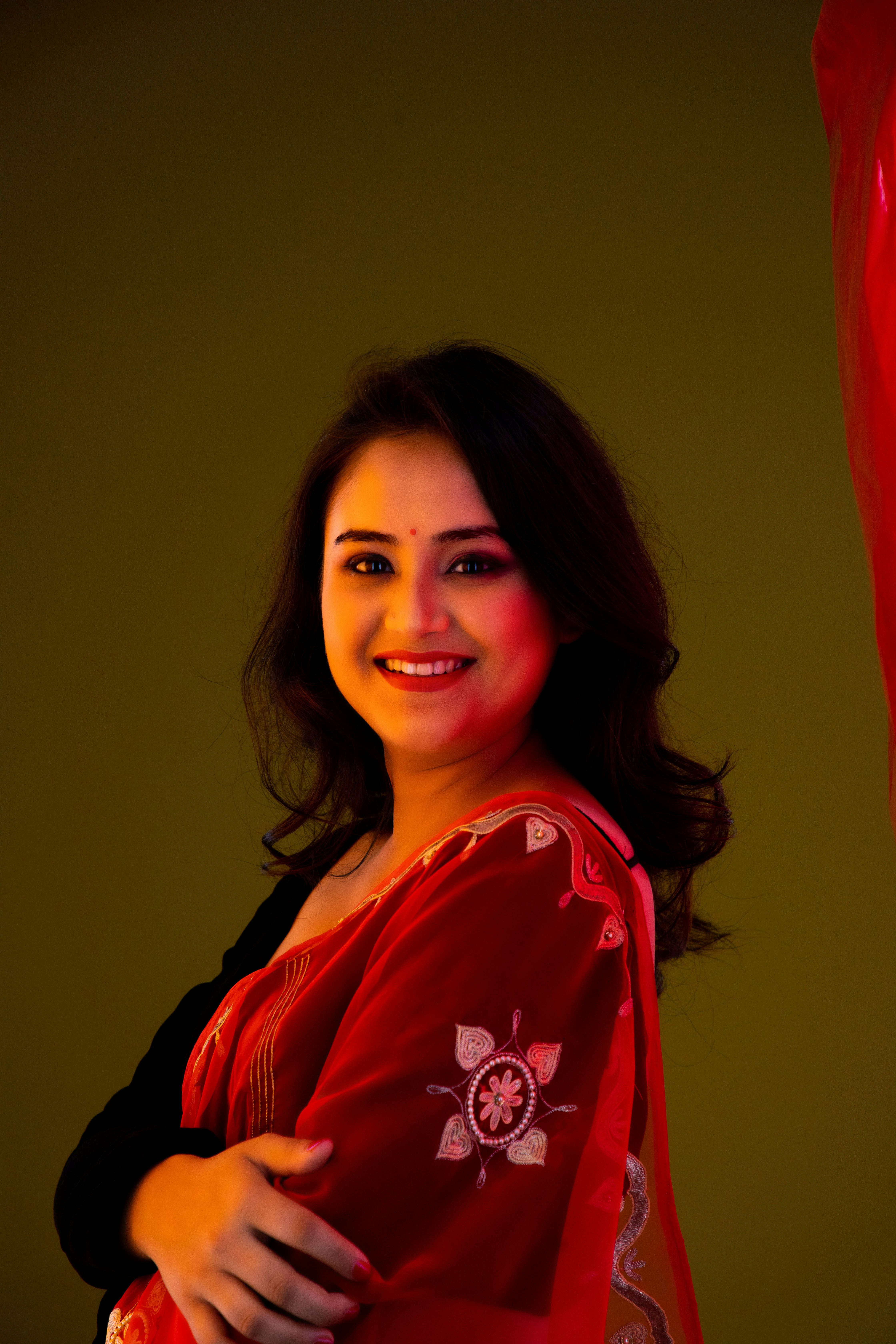
Ishikha rao
Ishikha rao
A Tech Geek by profession and Tech writer by passion.