Functions (Function Declaration, Function Expression, Arrow Functions)
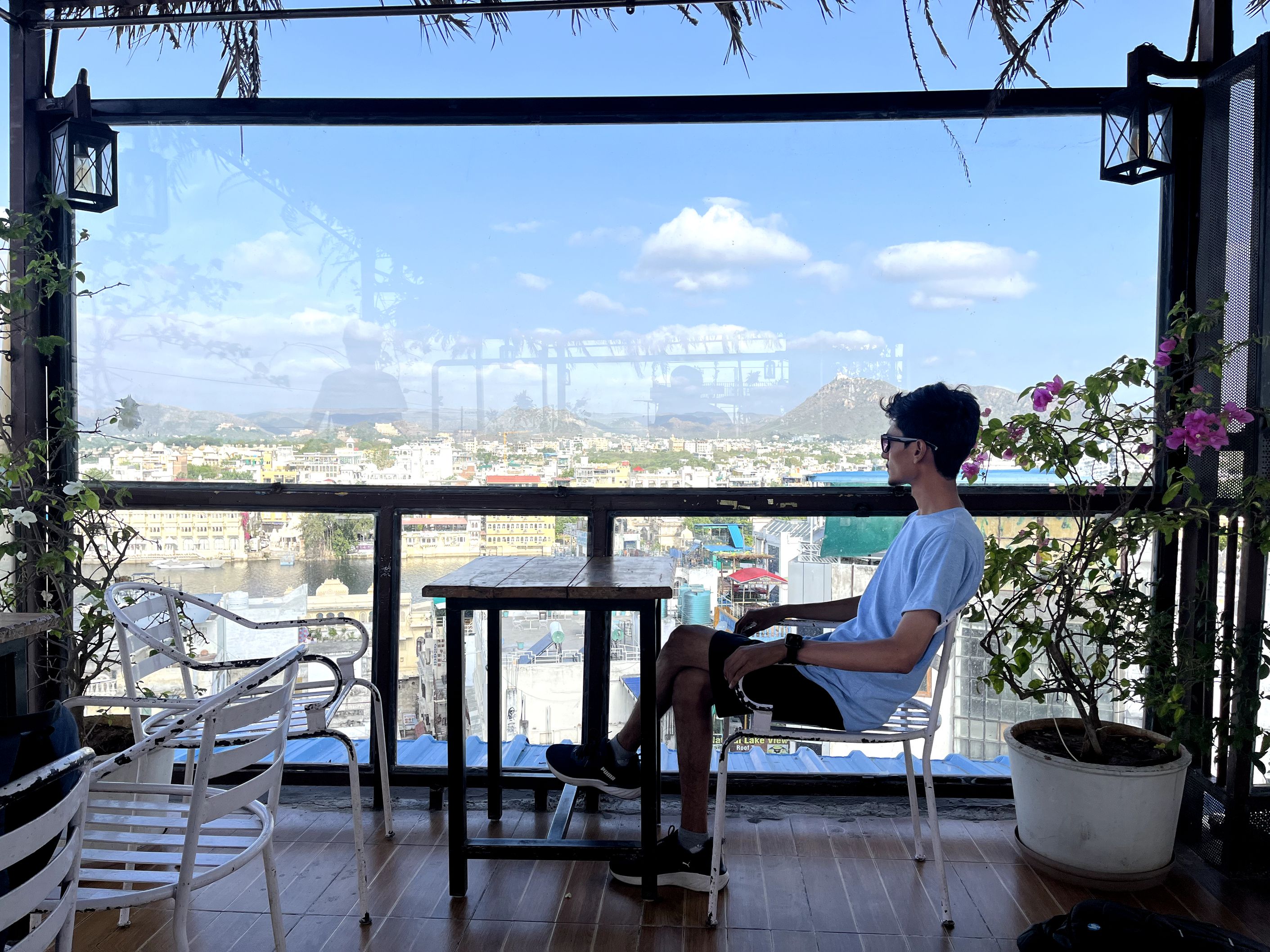
Expert-Level Explanation
Functions in JavaScript are blocks of code designed to perform a particular task.
Function Declaration: Defines a named function. Syntax:
function name(parameters) { //code }
. A function declaration is hoisted, allowing it to be used before it is defined in the code.Function Expression: A function defined within an expression. Syntax:
const myFunction = function(parameters) { //code };
. Function expressions are not hoisted.Arrow Functions: Introduced in ES6, they provide a concise way to write functions. Syntax:
const myFunction = (parameters) => { //code };
. Arrow functions do not have their ownthis
context.
Creative Explanation
Think of functions as kitchen appliances:
Function Declaration: Like a classic oven that's always ready to bake. You know it by a specific name and can use it even if you decide what to bake later (hoisting).
Function Expression: Like a blender that you set up when you need it. It's not ready until you declare it (no hoisting).
Arrow Functions: Like a modern, compact microwave. Quick and efficient for small tasks, but it doesn't have some features of the larger appliances (no own
this
context).
Practical Explanation with Code
// Function Declaration
function greet(name) {
return `Hello, ${name}!`;
}
// Function Expression
const square = function(number) {
return number * number;
};
// Arrow Function
const add = (a, b) => a + b;
Real-world Example
Function Declaration: Like having a specific tool for a job, such as a screwdriver for screws.
Function Expression: Like setting up a tent. It's not usable until it's fully set up.
Arrow Functions: Like using a quick-fix solution like duct tape for small repairs.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
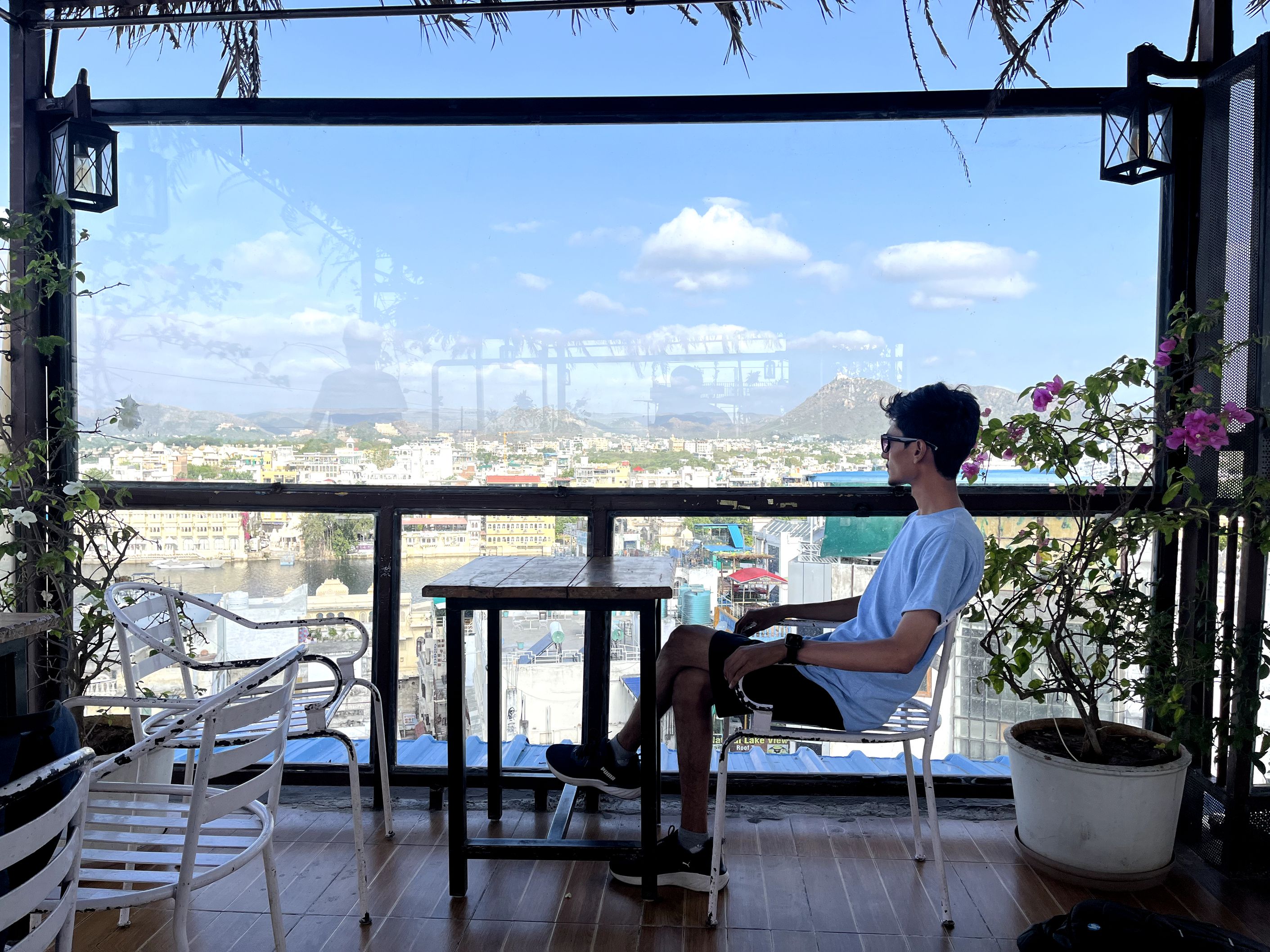
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.